Rest Parameters vs. Spread Syntax: Simplified Explanation-

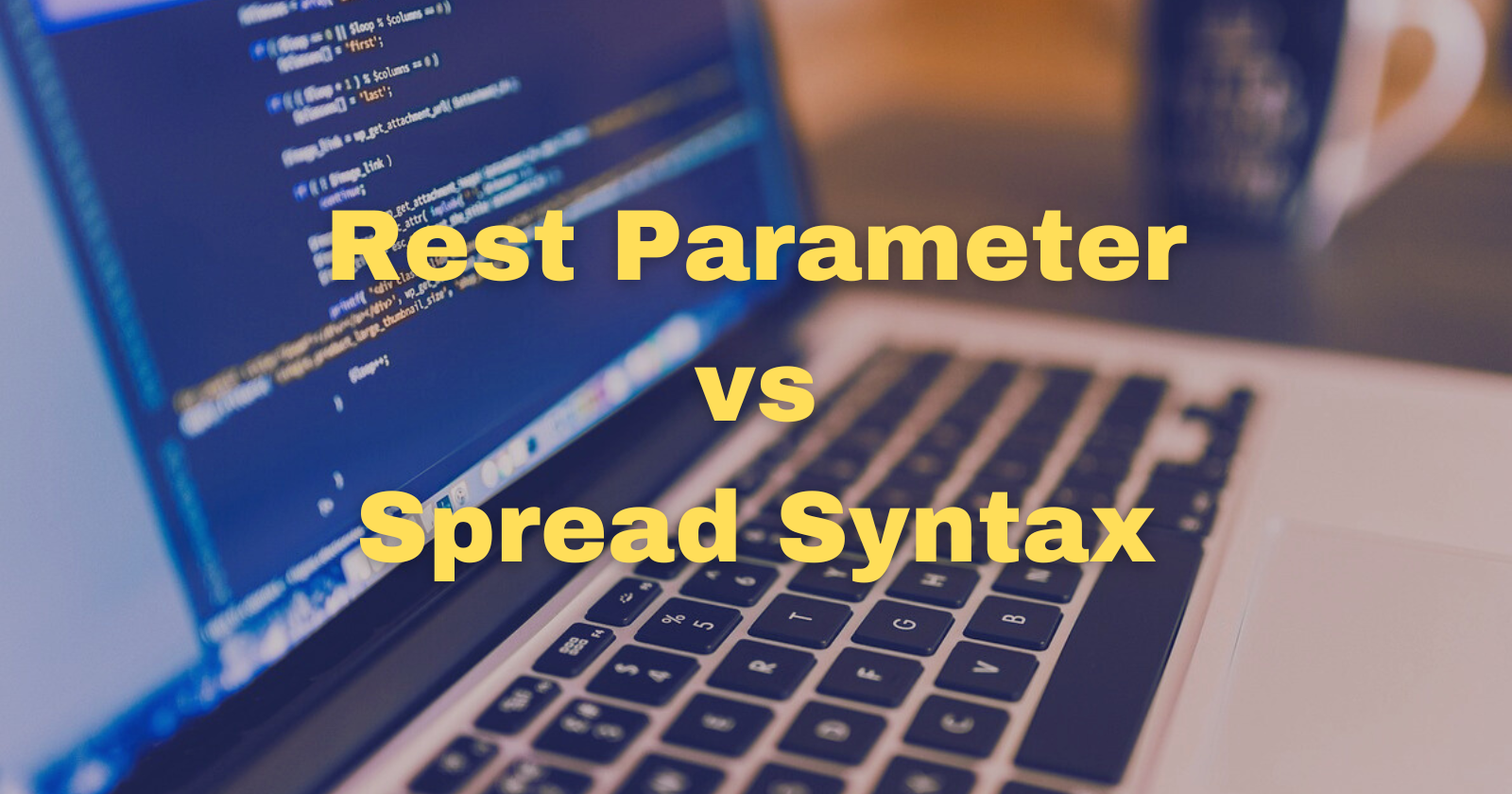
Hi π fellow developers! My name is Sandeep Singh, and I am an aspiring web developer. Today, I am back with an interesting and useful JavaScript concept: Rest Parameters and Spread Syntax. In this article, I will aim to make these two concepts clear by keeping the explanations concise and to the point. By the end of this article, you will understand the difference between the two and be able to use them in your daily JavaScript code.
Rest Parameter
The "Rest" parameter in JavaScript allows you to represent an indefinite number of arguments as an array. This is useful when you want to work with multiple arguments in a function without knowing how many there will be. Sometimes we encounter a situation where we donβt know how many arguments our function will take,in such cases, the rest parameter comes in handy.
lets understand this with an example !
function sum (a ,b ){
return a+b;
}
// calling the function sum with multiple arguments
const result = sum(1,2,3,4,5,6,7,8);
console.log(result);
// output: 3
In the example above, we are taking two parameters in our function sum
, but calling it with multiple arguments. The value of a
will be 1 and b
will be 2. So, what happens to the rest of the arguments? Where did they go?
Well, in JavaScript, we can pass as many arguments as we want to a function.
Now here comes the use of the rest parameter. We can use the rest parameter to collect the remaining arguments in an array.
We can use Rest Parameter to collect the rest of the arguments in an array.
so ,the remaining argument can be stored in an array like this-
function sum (a ,b , ...args ){ // args is the name of the array and it will..
// store the remaining argumnets
//lets log args
console.log(args) // it will log [3,4,5,6,7,8]
return a+b;
}
// calling the function sum with multiple arguments
const result = sum(1,2,3,4,5,6,7,8);
console.log(result);
// output: 3
From the above example, it can be understood that we can use the rest parameter (...args
) to store the remaining arguments into an array, and we can use that array as we want.
Rest parameters are useful when we have to deal with multiple arguments .
Note: The rest parameter must come at the end of the parameter list.
Spread Syntax
We used the rest parameter to collect arguments into an array, but what if we want to use array elements as individual arguments? This is where the spread syntax comes in, which allows us to spread the array elements into arguments.
lets understand this with an example
function sum(a, b, ...rest) {
return a + b;
}
let arr = [1, 2, 3, 4, 5, 6, 7, 8];
// Passing the array (arr) as arguments to the function sum
let result = sum(...arr);
console.log(result); // Output: 3
In the above code example, we are passing the array elements as arguments to the function sum
. For that, we used the spread syntax: sum(...arr)
.
Both the rest parameter and the spread syntax start with three dots (...
), but the difference is clear:
We use the rest parameter to collect arguments into an array.
We use the spread syntax to pass array elements as individual arguments to a function.
Besides passing array elements as arguments to a function, the spread syntax can be used for other purposes as well.
For example:
copying array elements
const arr1= [1,2,3];
const arr2=[ ...arr1 ];
console.log(arr2) ; // output: [1,2,3]
Merging multiple arrays
const arr1=[1,2,3];
const arr2=[4,5,6];
const arr3=[7,8,9];
const array=[ ...arr1 , ...arr2 , ...arr3 ];
console.log(array) ; // [1,2,3,4,5,6,7,8,9]
Copying objects
const user ={
name:"Sandeep",
age:21,
};
const person={...user};
console.log(person);
I hope the above article has made it clear for you to understand the uses of the rest parameter and spread syntax, as well as the difference between them.
Practice the code examples above for better understanding!
See you next time with another informative article. π
Subscribe to my newsletter
Read articles from Sandeep Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
