Handling Time Zone Differences in Java: Ensuring Consistent Dates Across Regions

Table of contents
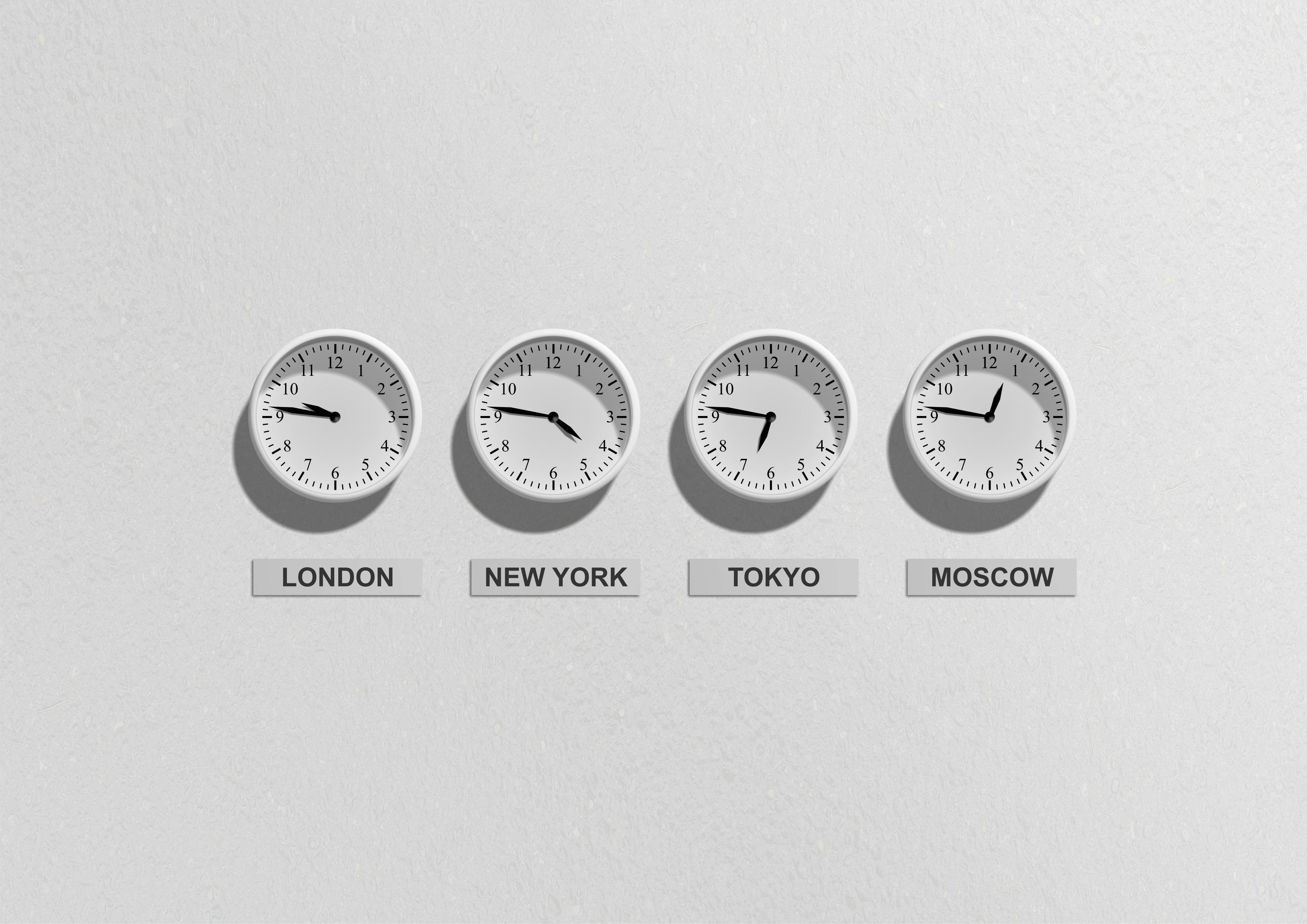
When developing applications that cater to users across various time zones, ensuring consistent date and time handling is crucial. Time zone differences can lead to discrepancies where users see different dates or times, even though they're interacting with the same event or data. In this article, I’ll walk you through how to manage these differences effectively using Java, with a focus on understanding time zones, using Java's time libraries.
1. Understanding the Time Zone Issue
Let’s say you have users in New York and Tokyo interacting with the same system, where events are set to start on specific dates and times. Because New York is in Eastern Standard Time (EST) and Tokyo in Japan Standard Time (JST), a given time in New York will appear as a different time in Tokyo. This can lead to confusion, especially if both users are viewing shared events like meetings or deadlines.
To solve this, we need to (1) standardize the time format across all users and (2) ensure that date and time are displayed correctly in each user’s local time zone.
2. Solution Approach: Using java.time Package
Java’s java.time package, introduced in Java 8, provides robust tools for working with time zones. By storing time in a standard time zone (usually UTC) and converting it to users’ local time zones only when needed, we can maintain consistency across regions.
Here’s how I typically handle it:
Step 1: Storing Dates in UTC
Coordinated Universal Time (UTC) is the common standard for storing time data. By saving dates in UTC, we eliminate the risk of time zone issues when users access the data from different regions.
import java.time.LocalDateTime;
import java.time.ZoneOffset;
import java.time.ZonedDateTime;
public class TimeZoneExample {
public static void main(String[] args) {
// Get the current time in UTC
ZonedDateTime utcTime = ZonedDateTime.now(ZoneOffset.UTC);
System.out.println("Stored UTC Time: " + utcTime);
}
}
Step 2: Converting UTC to User's Local Time Zone
When displaying time, convert the UTC-stored time to the user’s local time zone. This can be done by retrieving the user’s time zone from their profile or the device's settings, then adjusting the UTC time accordingly
import java.time.ZoneId;
public class TimeZoneExample {
public static void main(String[] args) {
// Get the current UTC time
ZonedDateTime utcTime = ZonedDateTime.now(ZoneOffset.UTC);
// Assume user is in Tokyo
ZoneId userTimeZone = ZoneId.of("Asia/Tokyo");
ZonedDateTime userTime = utcTime.withZoneSameInstant(userTimeZone);
System.out.println("UTC Time: " + utcTime);
System.out.println("User's Local Time (Tokyo): " + userTime);
}
}
In this code, I use withZoneSameInstant() to adjust utcTime to the user’s time zone without altering the instant it represents.
3. Ensuring Consistency with Instant for Backend Operations
For backend logic, Instant can be helpful. An Instant represents a timestamp in UTC without time zone details, making it perfect for scheduling and comparing dates on the backend.
import java.time.Instant;
public class TimeZoneExample {
public static void main(String[] args) {
Instant timestamp = Instant.now();
System.out.println("Instant timestamp (UTC): " + timestamp);
}
}
Conclusion
By storing times in UTC and converting them to the local time zone only, when necessary, we can prevent the confusion caused by time zone differences. Java’s java.time package offers a straightforward, reliable way to handle these conversions and display consistent date and time information to users worldwide.
Subscribe to my newsletter
Read articles from Fred Achi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
