Day 8 of 90DaysOfDevOps : Shell Scripting Challengeπ§

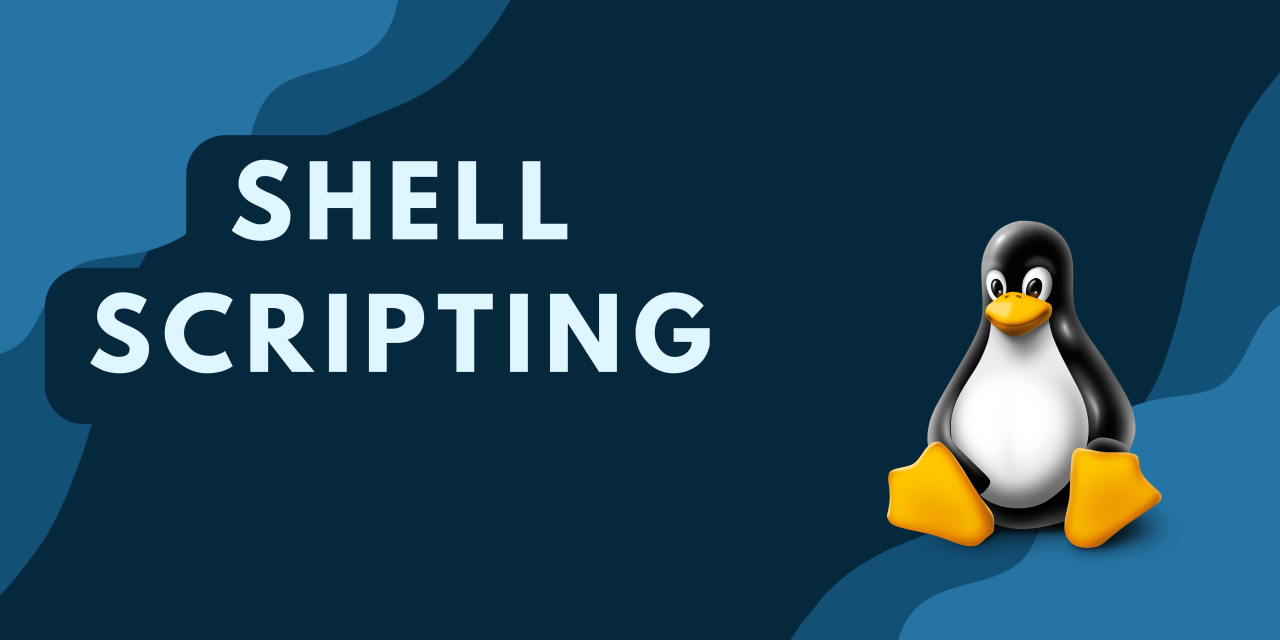
Task 1: Adding Comments in Bash Scripts π
Comments are notes added in code to explain what each part does, making it easier for others (and yourself) to understand later. Comments in bash scripts start with a #
.
#!/bin/bash
# This script greets the user
echo "Hello, user!"
In the above script:
# This script greets the user
is a comment and wonβt be executed.The
echo
command will display "Hello, user!"
Task 2: Displaying Messages with echo
π¬
The echo
command is used to print text to the terminal. This is great for providing messages, displaying results, or debugging.
#!/bin/bash
echo "Welcome to Bash scripting!"
When you run this script, youβll see:
Welcome to Bash scripting!
Task 3: Using Variables π¦
Variables store data like numbers, text, or filenames. You can create a variable by assigning it a value, which can then be referenced in the script.
#!/bin/bash
greeting="Hello"
name="Pooja"
echo "$greeting, $name!"
Output:
Hello, Pooja!
Task 4: Using Variables for Simple Math β
You can use variables to perform calculations in a script. In this example, weβll create a script that takes two numbers and prints their sum.
#!/bin/bash
num1=10
num2=20
sum=$((num1 + num2))
echo "The sum of $num1 and $num2 is $sum"
Output:
The sum of 10 and 20 is 30
Task 5: Built-in Variables for Useful Information π
Bash has built-in variables that hold useful system information. Here are three common ones:
$USER
: The current username.$HOME
: The path to the current userβs home directory.$PWD
: The current working directory.
#!/bin/bash
echo "User: $USER"
echo "Home Directory: $HOME"
echo "Current Directory: $PWD"
Output:
User: pooja
Home Directory: /home/pooja
Current Directory: /home/pooja/scripts
Task 6: Using Wildcards for Pattern Matching π
Wildcards are special characters like *
used to match patterns in filenames. For example, to list all .txt
files in a directory, we can use *
to match any file ending in .txt
.
#!/bin/bash
echo "Listing all .txt files in the directory:"
ls *.txt
If there are files like notes.txt
and data.txt
in the directory, the output will be:
Listing all .txt files in the directory:
notes.txt
data.txt
Summary π
Bash scripting lets you automate tasks efficiently. By mastering comments, echo
, variables, built-in variables, and wildcards, you can start building useful scripts to make your work easier!
Subscribe to my newsletter
Read articles from Pooja Naitam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pooja Naitam
Pooja Naitam
π Hello! I'm Pooja Naitam, a passionate DevOps fresher with a solid foundation in the field. I hold the AWS Certified Cloud Practitioner (CCP) certification, and I'm eager to apply my knowledge to real-world projects while continuously learning cutting-edge technologies. Let's connect and grow together in the exciting world of DevOps!