Android Architecture
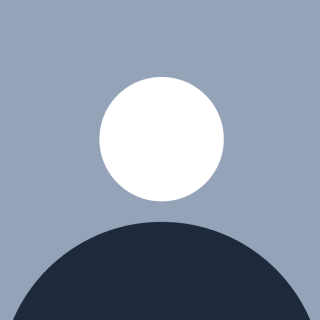
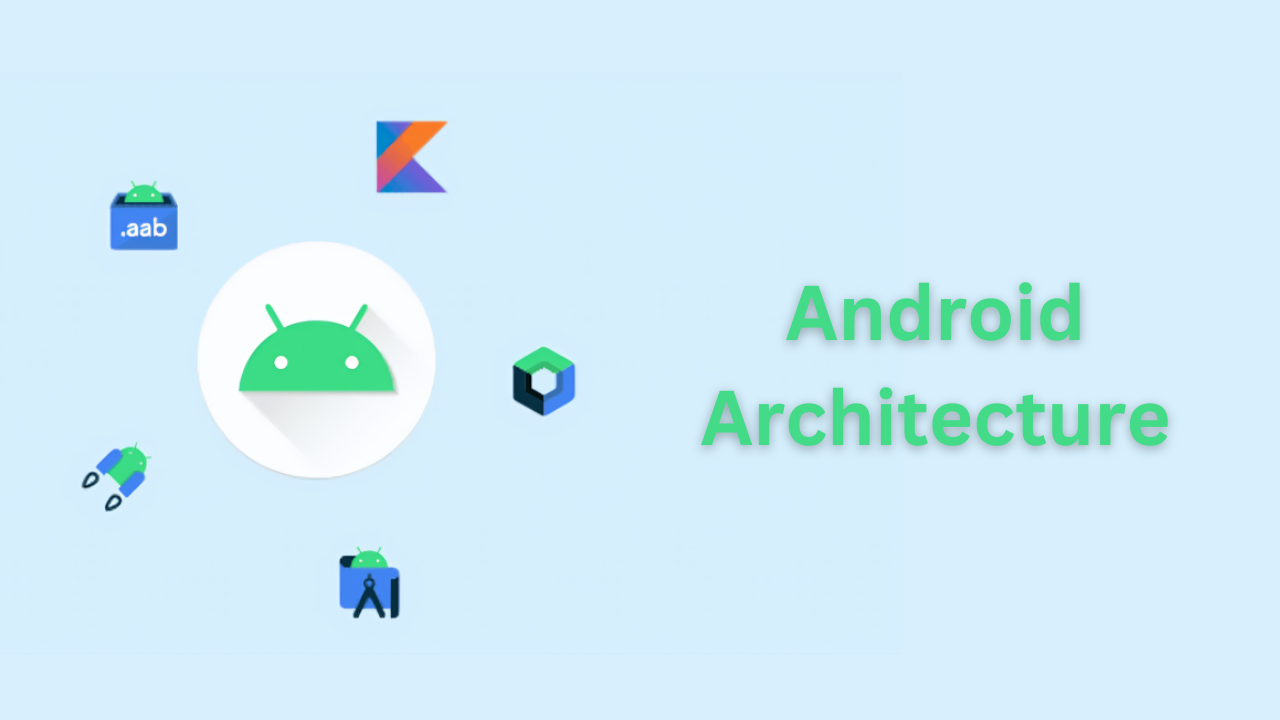
AOSP, or Android Open Source Project, is an open-source software stack and development platform for mobile devices, led by Google. It provides the foundational code and tools necessary for building Android operating systems, allowing manufacturers and developers to create customized versions of the Android OS for a wide range of devices.
Definitions for Key Terms in AOSP
Android app
An app created using only the public Android API. These apps can be downloaded from platforms like Google Play Store and may also be preinstalled by manufacturers for core device functionality.Privileged app
An app that uses both the Android API and system APIs. These apps must be preinstalled on the device and have elevated privileges compared to regular apps.Device manufacturer app
An app developed using a combination of Android APIs, system APIs, and direct access to Android framework implementation. These apps must be preinstalled by the device manufacturer and can only be updated with system software updates.System API
A set of APIs available exclusively to Android partners and OEMs for integration into preinstalled apps. These APIs are marked with@SystemApi
annotations in the code.Android API
The publicly available API that third-party developers use to build Android apps. It is documented and accessible for general use.Android framework
A collection of Java classes and other precompiled code that allows developers to build apps. Some portions are publicly accessible through the Android API, while others are available only to OEMs through system APIs.System services
Modular components such assystem_server
, SurfaceFlinger, and MediaService that expose functionality for interacting with Android’s underlying hardware and system capabilities.Android runtime (ART)
The Java runtime environment provided by AOSP, responsible for translating app bytecode into processor-specific instructions for execution on the device.Hardware abstraction layer (HAL)
A layer that standardizes interfaces for hardware vendors to implement, allowing Android to be agnostic to the underlying hardware. HALs allow developers to write higher-level code without needing to modify lower-level hardware drivers.Native daemons and libraries
Native daemons likeinit
,healthd
,logd
, andstoraged
interact directly with the kernel, providing essential system functionality. Native libraries likelibc
,liblog
, andlibutils
also interface directly with the kernel and provide core services.Kernel
The core part of the operating system, managing the hardware and acting as an intermediary between the hardware and software. The Android kernel is modular, with some parts hardware-agnostic and others specific to the vendor.
Android Architecture
Application Layer (System Apps):
- This layer includes both built-in and third-party applications. These applications are what users interact with directly on their devices.
Application Framework Layer (Java API Framework):
These are applications developed using the Android API. They are typically distributed through the Google Play Store, although other distribution methods exist. Some Android apps may be preinstalled by device manufacturers to support core device functionalities.
This layer provides developers with the APIs needed to develop applications. It is written in Java and includes several key components:
Activity Manager: Manages the lifecycle of applications and provides a common navigation backstack.
Location Manager: Provides APIs to determine the location of the device.
Package Manager: Manages the applications installed on the device.
Notification Manager: Allows applications to display alerts and notifications to the user.
Resource Manager: Provides access to non-code embedded resources such as strings, color settings, and user interface layouts.
Telephony Manager: Provides information about the telephony services on the device.
Window Manager: Manages the windowing system on the device.
Content Providers: Allows applications to publish and share data with other applications.
View System: An extensible set of views used to create application user interfaces.
Libraries and Android Runtime Layer:
C/C++ Libraries: These libraries are written in C or C++ and include:
Webkit: Provides a web browser engine used to display web content.
OpenMAX AL: Provides a standardized interface for audio, video, and image codecs.
Libc: Provides a subset of the standard C library (libc), used by the majority of C-based applications.
OpenGL|ES: Provides an interface between software and graphics acceleration hardware.
Media Framework: Provides a unified interface for playing and recording audio and video.
SQLite: Provides a lightweight relational database engine used to access a variety of relational database systems.
SSL: Provides a secure sockets layer (SSL) implementation used to access a variety of secure communications protocols.
Android Runtime:
ART/Dalvik Virtual Machine: A virtual machine optimized for mobile devices, allowing multiple instances of the Virtual Machine to run efficiently in a limited amount of memory. Each Dalvik application runs in its own process, with its own instance of the Dalvik Virtual Machine, and is executed in a separate Linux process. Note: Dalvik Virtual Machine is deprecated in Android 4.4 KitKat and replaced by Android Runtime (ART). ART uses Ahead-Of-Time (AOT) compilation, making it faster than Dalvik's Just-In-Time (JIT) compilation.
Core Library: Provides most of the functionality of the Java programming language required by the Java API framework and the apps running on Android.
Hardware Abstraction Layer (HAL):
The HAL provides standard interfaces that expose device hardware capabilities to the higher-level Java API framework. It acts as the interface between the Operating System Kernel and the hardware. The source code of the HAL is not available in the AOSP to protect the Intellectual Property rights of hardware manufacturers. It provides a virtual hardware platform for the operating system, ensuring hardware independence and portability across devices.
Linux Kernel:
Android's core system services are based on Linux and are written in C/C++. The Linux kernel is responsible for managing core system services such as security, memory management, process management, network stack, and driver model. It also provides an abstraction layer between the hardware and the rest of the software stack.
Structure of the Android Source Repository
Android Source Repository Structure
art
Android Runtime (ART) and related components.
Purpose: Contains the core runtime system for Android, replacing the older Dalvik VM. ART improves performance with features like JIT (Just-in-Time) and AOT (Ahead-of-Time) compilation.bionic
Android's custom C library.
Purpose: Provides the C library for Android, offering essential system-level APIs, POSIX implementations, and Android-specific system calls.bootable/
Boot process-related components.
Sub-directories:bootable/recovery
: Code related to the recovery partition of devices.bootable/bootloader
: Code for bootloader functionality, handling the device boot process.
build/
Android build system files.
Purpose: Contains configuration files and build scripts for Android’s build system. This includes both legacymake
-based files and more modern Blueprint (Android.bp
) files.cts
Compatibility Test Suite.
Purpose: Contains tests ensuring Android devices adhere to the Compatibility Definition Document (CDD), verifying they meet Android's compatibility standards.dalvik
(Deprecated in favor of ART).
Purpose: Legacy Dalvik Virtual Machine code, now largely replaced by ART for improved performance, but kept for backward compatibility.developers/
Resources for Android developers.
Purpose: Contains documentation, scripts, and tools designed to help Android developers with coding and platform integration.development/
Development tools and utilities.
Purpose: Contains tools for Android development, focusing on aspects like debugging, building, and integration.device/
Device-specific configurations and files.
Purpose: This directory contains device-specific code, such as configurations, kernel files, proprietary binaries, and device integration settings.external/
Third-party and external libraries.
Purpose: Includes external libraries or tools integrated into Android, such as WebRTC, OpenSSL, and other open-source dependencies.frameworks/
Core Android framework code.
Sub-directories:frameworks/base
: Core system services, APIs, and UI components.frameworks/native
: Native Android services and system libraries.frameworks/opt
: Optional frameworks and libraries that extend Android functionality.
hardware/
Hardware Abstraction Layer (HAL).
Purpose: Contains code for abstracting hardware, enabling Android to work across different devices and hardware platforms without needing changes to core system code.kernel/
Linux kernel source.
Purpose: Contains the Linux kernel and device-specific configurations and patches required for Android devices.libcore
Core Java libraries.
Purpose: Provides essential Java libraries (java.lang
,java.util
, etc.) that are foundational to Android's functionality.libnativehelper
JNI helper functions.
Purpose: Contains helper functions that simplify working with the Java Native Interface (JNI), facilitating communication between Java code and native code.packages/
Stock Android apps and providers.
Purpose: Contains the system apps (e.g., Settings, Launcher) and various core applications packaged with the Android platform.pdk
Platform Development Kit.
Purpose: Tools, documentation, and libraries to aid the development of platform extensions, customizations, and device-specific code.platform_testing/
Platform validation tools and tests.
Purpose: Contains testing utilities and scripts to ensure Android platform components are stable, compatible, and function as expected.prebuilts/
Prebuilt binaries and tools.
Purpose: Contains precompiled binaries (e.g., toolchains, libraries) used by the Android build system to reduce build times and improve efficiency.sdk/
Software Development Kit tools and libraries.
Purpose: Contains components required for Android application development, including SDK management tools, ADB, and fastboot utilities.system/
Core Android system libraries and services.
Purpose: Includes lower-level system components and libraries for managing key platform services like memory, file systems, and security.test/
Android tests.
Purpose: Contains unit tests, integration tests, and validation scripts for verifying Android components and ensuring platform stability.toolchain/
Build toolchain components.
Purpose: Contains the toolchains and build-related components (e.g., C/C++ compilers, linker scripts) used in the Android build process.tools/
Android development tools.
Purpose: Includes various utilities for Android development, such as debugging tools, profiling utilities, and other diagnostic tools.trusty/
Trusted Execution Environment (TEE).
Purpose: Contains code related to the Trusted Execution Environment, enabling secure processing and isolation of sensitive data and applications.
Top-Level Files
.gitattributes
Defines Git attributes for the repository.
Purpose: Configures Git settings such as line endings or diff behaviors for specific file types..gitmodules
Defines submodules.
Purpose: Specifies information about any Git submodules in the repository, detailing their location and configuration..supermanifest
Manifest for managing large projects.
Purpose: Tracks metadata for repositories that are part of larger super-repos, helping manage AOSP components.Android.bp
Blueprint build system configuration.
Purpose: Defines modules and build rules using the Blueprint build system, which has replaced many of the oldermake
-based files for parts of the Android system.BUILD
make
build system configuration.
Purpose: Legacy build configuration file for parts of Android still using the traditionalmake
-based system. Some parts of the platform still rely onBUILD
files.WORKSPACE
Used by the Bazel build system.
Purpose: Defines the project's workspace and manages external dependencies for builds using Bazel, Android's new build system for large-scale projects.bootstrap.bash
Script for bootstrapping the build environment.
Purpose: Initializes the Android build environment by configuring paths and setting up necessary environment variables.lk_inc.mk
Makefile for kernel or low-level boot components.
Purpose: Used in kernel or boot processes to manage low-level includes and build configuration.
Key Updates
Transition to Blueprint and Bazel:
The Android build system now uses Blueprint and Bazel for better scalability, flexibility, and efficiency.Android.bp
files are widely used for modularizing the Android codebase.Modularization:
Android's codebase is increasingly modular, with components split into smaller, reusable libraries and modules for easier maintenance and updates.Deprecation of Dalvik:
Dalvik has been largely replaced by ART (Android Runtime), though Dalvik code is still included for legacy support.Device-Specific Focus:
Thedevice/
andhardware/
directories have become even more central to Android’s ecosystem, focusing on device-specific configurations and hardware abstraction layers (HAL) for better platform portability.
Conclusion
In conclusion, the Android architecture is a comprehensive and layered system that provides a robust framework for developing mobile applications. It consists of several key components, including the Application Layer, Application Framework Layer, Libraries and Android Runtime Layer, Hardware Abstraction Layer, and the Linux Kernel. Each layer plays a crucial role in ensuring the smooth operation and functionality of Android devices. The Android Open Source Project (AOSP) offers a flexible platform for manufacturers and developers to create customized Android experiences, while the structured source repository supports efficient development and maintenance. Understanding this architecture is essential for anyone involved in Android development, as it provides the foundation for building innovative and efficient mobile applications.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer