Pre-Requisite of Routing in React JS
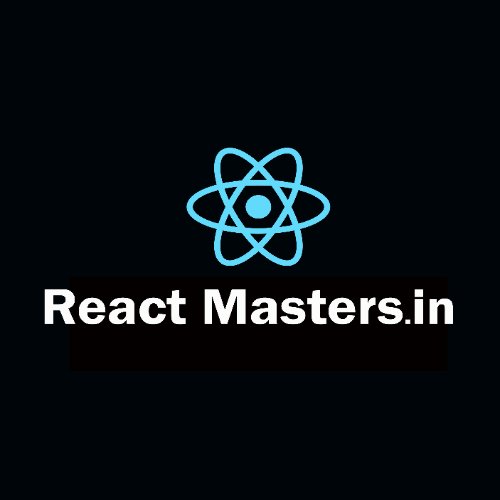
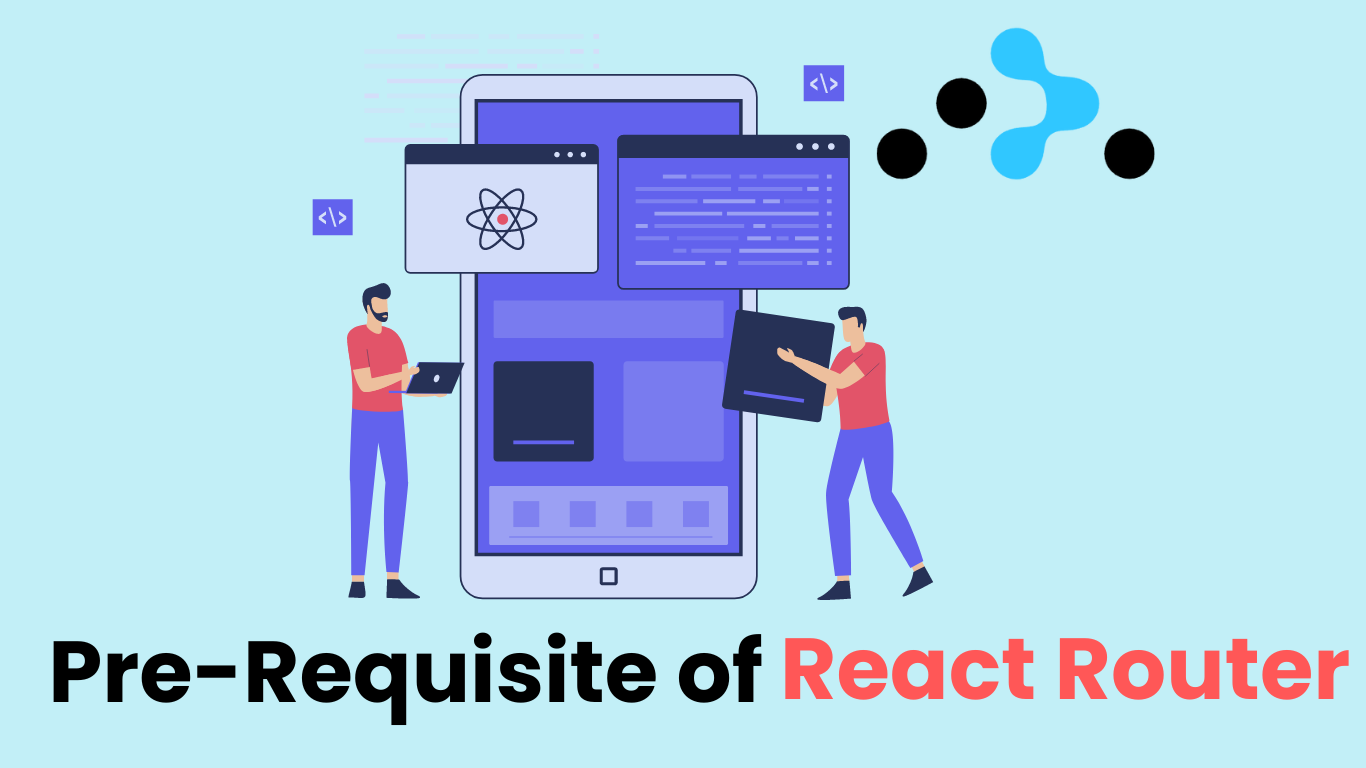
Understanding Routing in React JS and its Prerequisites:
Routing is an essential concept in web development that enables users to navigate different views or pages within a web application.
In React, routing plays a crucial role in building single-page applications (SPAs) where users can interact with other parts of the application without needing to refresh or reload the page.
By understanding React Router and how to implement routing, developers can create seamless, dynamic, and efficient user experiences.
What is Routing in React JS?
Routing in React JS refers to defining and handling the navigation paths within a React application.
React, a library focused on creating user interfaces does not natively support routing functionalities.
However, with the help of an external library called React Router, developers can efficiently manage routing and build an interactive, multi-view application.
React Router provides a set of components that enable the dynamic navigation of URLs and ensure that specific content is displayed based on the path specified in the browser.
When a user navigates to a different URL, the React Router intercepts the request, determines the corresponding component to render, and displays it without reloading the page.
This approach enhances user experience by minimizing loading times and creating a more seamless transition between views.
Some key features of React Router include:
Dynamic Routing: React Router allows components to update and render based on the current route, supporting a real-time, interactive experience.
Nested Routes: It enables nested navigation, allowing components within components, particularly useful for building complex applications with multiple view levels.
URL Parameters: React Router supports URL parameters, making it possible to pass values or IDs directly within the URL, which is especially useful in applications that require dynamic data fetching.
By using React Router, developers can manage paths like “/home,” “/about,” or “/product/
” efficiently, displaying different components or content for each path.
Prerequisites for Using Routing in React JS
To use routing in a React application, developers should be familiar with a few fundamental concepts and tools to ensure a smooth implementation:
1. Basic Understanding of React
- Familiarity with core React concepts such as components, props, and state is essential before diving into routing. Knowing how to build and structure components helps in creating a layout that works seamlessly with routes.
2. React Router Library
- Installing and using React Router is a prerequisite for implementing routing in React. You can install it using npm or yarn by running:
npm install react-router-dom
- React Router provides components like
<BrowserRouter>
,<Route>
,<Link>
, and<Switch>
, which are essential for defining routes, setting up navigation, and controlling the display of components.
3. Component-Based Architecture
Routing in React relies heavily on components. Each route usually corresponds to a specific component, making it important to structure your application into modular components.
This helps organize your routes and allows you to display specific components for each path easily.
4. JavaScript ES6 Syntax
- A solid understanding of ES6 JavaScript syntax is beneficial, as features like destructuring, arrow functions, and modules are commonly used when working with React Router.
5. Understanding of Single-Page Applications (SPAs)
Knowing the fundamentals of SPAs is helpful since React Router is primarily used to build SPAs.
Unlike traditional multi-page applications, SPAs update content dynamically without reloading the entire page, and routing enables navigation within these applications.
Implementing Routing in React
After meeting the prerequisites, you can start by setting up routing with React Router. Wrap the root component with <BrowserRouter>
, define routes with <Route>
, and use <Link>
for navigation. Here’s a basic example:
import { BrowserRouter as Router, Route, Switch, Link } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
</nav>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
</Switch>
</Router>
);
}
Conclusion
Routing in React JS is a powerful way to create dynamic, multi-page experiences within single-page applications.
With React Router, developers can manage paths, create nested routes, and enhance user experience through seamless navigation.
Having a strong foundation in React, understanding SPAs, and knowing JavaScript basics are crucial prerequisites to working with routing effectively.
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
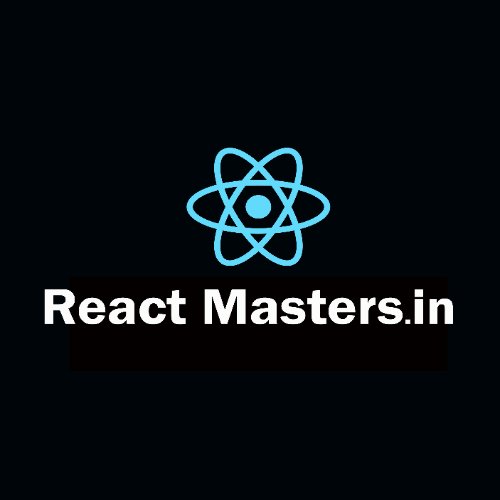
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.