Flutter App for UI automation
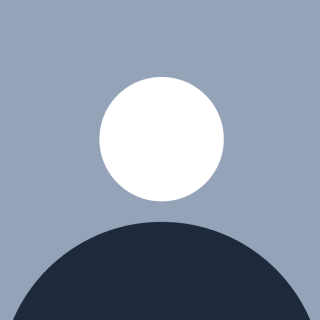
2 min read
Enabling APP for UI automation
Install Appium Flutter Driver: Appium Flutter Driver is a test automation tool for Flutter apps on multiple platforms. You can install it via npm:
npm install -g appium-flutter-driver
Enable Flutter Driver: You need to enable Flutter Driver extension in your app. In your main.dart
file, add these lines:
import 'package:flutter_driver/driver_extension.dart';
void main() { enableFlutterDriverExtension(); runApp(MyApp()); }
Build the app
# Android
flutter build apk --debug
# IOS
flutter build ios --debug --no-codesign -t lib/main.dart
Output: Built /Users/sid/build/ios/iphoneos/Runner.app.
# To have app deployed in phone
flutter run -v -t lib/main.dart
Bonus: creating .ipa file
Copy Runner.app to a a newly created folder. Say desktop
Rename folder to Payload
Zip the file to Payload.zip
Rename Payload.zip to Payload.ipa
Alternatively use,
xcrun -sdk iphoneos PackageApplication -v "Runner.app" -o "$(pwd)/Runner.ipa"
Script for running test
Install below required packages
APPIUM_SKIP_CHROMEDRIVER_INSTALL=true appium driver install --source=npm appium-xcuitest-driver@5.14.0
APPIUM_SKIP_CHROMEDRIVER_INSTALL=true appium driver install --source=npm appium-flutter-driver@2.4.2
APPIUM_SKIP_CHROMEDRIVER_INSTALL=true appium driver install --source=npm appium-uiautomator2-driver@2.42.1
# Should show below
[Appium] Available drivers:
[Appium] - uiautomator2@2.45.1 (automationName 'UiAutomator2')
[Appium] - flutter@2.4.2 (automationName 'Flutter')
[Appium] - xcuitest@5.14.0 (automationName 'XCUITest')
[Appium] Available plugins:
[Appium] - relaxed-caps@1.0.6
pip install Appium-Flutter-Finder
python code for running UI test
from appium.webdriver import Remote
from appium.options.ios.xcuitest.base import XCUITestOptions
from appium_flutter_finder.flutter_finder import FlutterElement, FlutterFinder
options = XCUITestOptions()
options.automation_name = 'flutter'
options.platform_name = 'ios'
options.set_capability('platformVersion', '17.0')
options.set_capability('deviceName', 'iPhone 15 Pro Max')
#options.set_capability('app', f'{os.path.dirname(os.path.realpath(__file__))}/../sample2/IOSFullScreen.zip')
options.set_capability('app', '/Users/sid/Payload.ipa')
options.set_capability('newCommandTimeout', 10000)
options.set_capability('appium:autoAcceptAlerts', 'true')
driver = Remote('http://localhost:4723', options=options)
finder = FlutterFinder()
text_finder = finder.by_text('Sign in')
text_element = FlutterElement(driver, text_finder)
text_element.click()
# Swipe down to bottom page
driver.switch_to.context("NATIVE_APP")
driver.swipe(0, 100, 0, 0)
driver.save_screenshot('Homepage_bottom.png')
0
Subscribe to my newsletter
Read articles from Siddartha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by