Best Practices for Writing Good Git Commit Messages

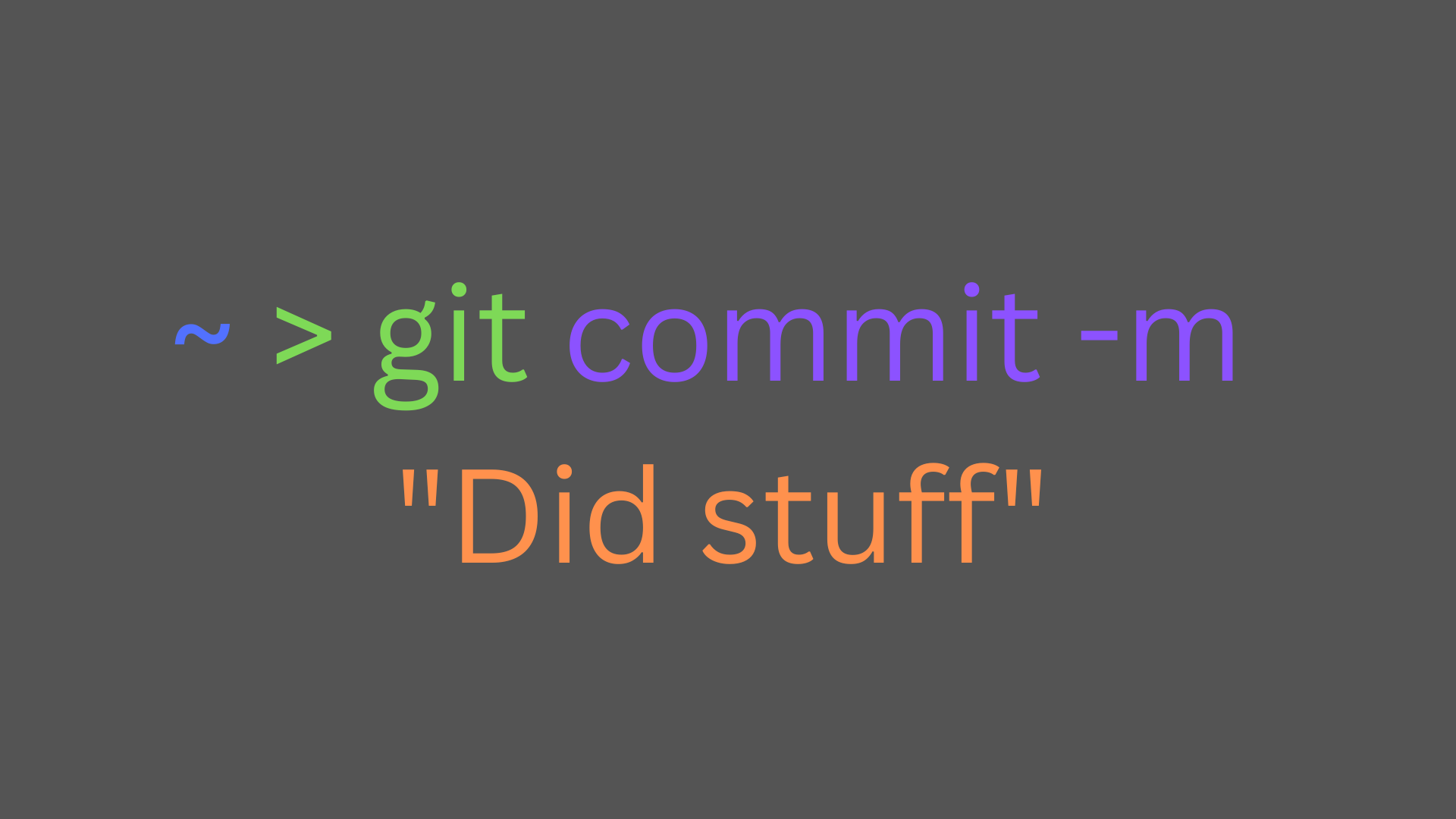
Introduction
In software development, commit messages are used to track changes to a codebase, making it easier to reference past versions when debugging or reviewing features. Well written commit messages matter because they’re essential not only for team collaboration but also for creating a strong impression on recruiters who may judge your coding practices based on your git commit history.
What is a Commit Message?
A commit message is a brief description of a change made to a codebase. Each commit captures one meaningful change, helping to build the project step by step.
Now, imagine you’re building a triangle, which has three sides. For each side, you’d make a separate commit to track your progress, like this:
feat: “Add Triangle Side 1”
feat: “Add Triangle Side 2”
feat: “Add Triangle Side 3”
Together, these commits represent the complete project of building the triangle, demonstrating how small changes accumulate to form the whole triangle.
How To Commit
Instead of using the common command git commit -m "message" for a quick commit, it’s best practice to include both a subject and a body in your commit message, separated by a blank line. To do this, you use the -m flag twice—Git will treat the first -m as the subject and the second -m as the body.
For example, if I just implemented a login feature, my commit message would look like this:
git commit -m "Add login feature"
-m "Implements user login functionality using JWT authentication. This enables users to log in securely and maintain session state across pages."
Top Six Best Practices In Commits
1. Keep it Short but Descriptive
Rule: Write a brief subject line (50 characters or less) and use the body for further details if necessary.
Good ✅:
git commit -m "Add JWT authentication to login feature"
-m "This update implements JWT authentication, enabling secure user sessions across different pages. It replaces the previous session-based auth system."
Bad (too long) ❌:
git commit -m "This commit adds JSON Web Token (JWT) based authentication to the login feature and will help secure user sessions better."
2. Use the Imperative Mood
Rule: Write the subject line as a command, which helps standardize messages and match Git’s style.
Good ✅:
git commit -m "Fix login bug that blocked user access"
Bad (Used past tense over imperative) ❌:
git commit -m "Fixed login bug that blocked user access"
3. Separate the Subject and Body with a Blank Line
Rule: Use a blank line between the subject and body to improve readability.
git commit -m "Add new user registration feature"
-m "Implements new user registration with email and password validation. Includes form error handling and adds password strength meter."
OR using a text editor:
git commit
In the editor:
Add new user registration feature
Implements new user registration with email and password validation.
Includes form error handling and adds password strength meter.
4. Explain the Why, Not Just the What
Rule: In the body, explain why you made the change, providing context for future developers.
Good ✅:
git commit -m "Refactor auth middleware"
-m "Optimizes middleware to reduce response times by caching user sessions. This change addresses latency issues reported by users on slower networks."
Bad (Not explicit enough) ❌:
git commit -m "Refactor auth middleware for better performance"
5. Reference Issues and Tickets
Rule: If relevant, include issue or ticket IDs to link your change to a specific bug or feature request.
Good ✅:
git commit -m "Fix login bug in mobile view (#452)"
-m "Corrects an alignment issue in the login form for mobile devices. Closes issue #452 reported by the QA team."
Bad (no traceability) ❌:
git commit -m "Fix login bug in mobile view"
6. Use Consistent Prefixes for Types of Changes
Rule: Use prefixes to clarify the type of change. Common prefixes include fix, feat, chore, docs, style, refactor, and test.
Type | Description | Example |
feat | Adds a new feature or functionality. | feat: Add password reset option to login page |
fix | Fixes a bug or issue. | fix: Resolve crash on logout button click |
chore | Routine tasks, maintenance, or project setup changes. | chore: Update dependencies in package.json |
docs | Updates or changes to documentation. | docs: Update README with API usage examples |
style | Code style changes (formatting) that don’t affect logic. | style: Apply consistent indentation in all JS files |
refactor | Code restructuring without changing functionality. | refactor: Simplify user authentication flow |
test | Adds or updates tests. | test: Add unit tests for login component |
Conclusion
Writing clear, meaningful commit messages is one of those small habits that make a big difference. By keeping messages concise yet descriptive, using the imperative mood, separating the subject and body, and explaining why a change was made, you’re not just documenting code—you’re building a story for your project. These practices make your work easier to follow, help teammates understand your contributions, and even leave a positive impression on recruiters reviewing your git commit history. Keep practicing, and quality commits will become second nature.
Subscribe to my newsletter
Read articles from Daniel Olasupo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Daniel Olasupo
Daniel Olasupo
software engineer | Learner | Technical Writing Blogger