Git: Essential Commands and Creating an Emergency Commit Alias
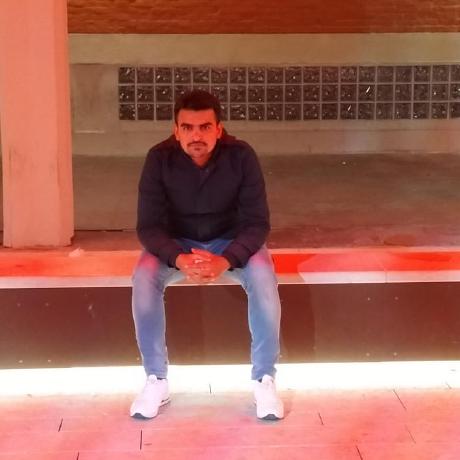

Git is a powerful, distributed version control system widely used by developers to track changes in their codebase, collaborate with others, and manage projects efficiently. Understanding how to use Git effectively can greatly enhance your development workflow. In this article, we’ll explore the most useful Git commands, how to clone a repository using SSH keys, and how to create an emergency commit alias for quick commits.
Cloning a Git Repository with SSH Key
When working with Git repositories, you often need to clone them to your local machine. Cloning a repo means downloading a copy of the repository from a remote server (e.g., GitHub, GitLab, Bitbucket) to your local environment. This enables you to make changes, track versions, and collaborate with others.
To clone a repository securely using SSH (which avoids the need for entering your username and password repeatedly), follow these steps:
Steps to Clone a Git Repository Using SSH:
Generate SSH Key Pair (if you haven't already): First, you need to generate an SSH key on your local machine. Open your terminal and run:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This creates a new SSH key pair (private and public) in the
~/.ssh
directory by default.Add SSH Key to SSH Agent: Ensure the SSH agent is running and add your key:
eval "$(ssh-agent -s)" ssh-add ~/.ssh/id_rsa
Add SSH Key to Git Hosting Service: Copy the content of your public SSH key (e.g.,
~/.ssh/id_
rsa.pub
) and add it to your Git service (GitHub, GitLab, etc.) under the SSH key settings.Clone the Repository Using SSH: Once your SSH key is added, you can now clone a repository using the SSH URL. For example, to clone a repository from GitHub:
git clone git@github.com:username/repository.git
This will clone the repository to your local machine without needing to enter your username or password every time you interact with the remote repository.
Most Useful Git Commands
Here are some of the most commonly used Git commands that every developer should be familiar with:
1. git init
This command initializes a new Git repository in the current directory. It creates the .git
folder which will hold the repository data.
git init
2. git clone
As mentioned earlier, this command is used to create a local copy of a remote repository.
git clone <repository-url>
3. git status
The git status
command is one of the most important commands. It shows the current state of the repository, including staged, unstaged, and untracked files.
git status
4. git add
The git add
command stages changes (i.e., prepares them to be committed). You can add specific files or all changes.
git add <filename> # Add a specific file
git add . # Add all changes in the current directory
5. git commit
Once changes are staged, use git commit
to save them. Each commit requires a message explaining the changes.
git commit -m "Your commit message"
6. git push
The git push
command uploads your local changes to the remote repository.
git push origin main # Push changes to the main branch
7. git pull
This command fetches and merges changes from the remote repository into your current branch.
git pull origin main # Pull the latest changes from the main branch
8. git branch
Use this command to list, create, or delete branches in your repository.
git branch # List all branches
git branch <branch-name> # Create a new branch
git branch -d <branch-name> # Delete a branch
9. git merge
This command merges another branch into the current branch.
git merge <branch-name>
10. git log
The git log
command shows the commit history of the repository.
git log
11. git reset
You can use git reset
to undo changes, either by un-staging changes or by completely resetting a commit.
git reset <commit-hash> # Reset to a specific commit
git reset HEAD <file> # Unstage a file
12. git diff
To see the differences between your working directory and the index (staged files), or between two commits, use git diff
.
git diff # Show unstaged changes
git diff --cached # Show staged changes
Creating an Emergency Commit Alias in Git
Sometimes, during intense development or critical bug fixes, you might need to commit changes quickly, especially when working with large teams. The process of staging, committing, and pushing changes can take precious time in an emergency situation. One way to speed up the process is by creating an alias that allows you to execute a series of Git commands with a single shortcut.
Creating an "Emergency Commit All" Alias
Here’s how to create an alias for a one-liner that stages, commits, and pushes changes all at once. This can be helpful in scenarios where you need to quickly commit everything and push it to the remote repository.
Edit your Git Configuration: You can add an alias to your Git configuration file (
~/.gitconfig
). Open the file in a text editor or modify it directly via the terminal.Run:
git config --global alias.commitall "!git add . && git commit -m 'Emergency commit' && git push"
This command creates a new alias
git commitall
that performs the following tasks in sequence:Adds all changes (
git add .
)Commits them with a default message ('Emergency commit')
Pushes the changes to the remote repository
Use the Alias: After configuring the alias, you can quickly commit all changes by running:
git commitall
Customizing the Commit Message
You can even customize the commit message for the commitall
alias. For example, if you want to specify a custom message when running commitall
, you can modify the alias as follows:
git config --global alias.commitall "!f() { git add . && git commit -m \"$1\" && git push; }; f"
Now, when you run git commitall "Your custom message"
, it will use the custom message you provide.
Notes on the Alias:
This is a dangerous alias because it commits everything in the repository without prompting for file selections or reviewing changes. It's meant for emergency situations, where speed is more important than thoroughness.
Always double-check the changes you are committing to avoid accidentally pushing unwanted changes.
Conclusion
Git is an essential tool for version control, and mastering its commands is crucial for efficient software development. Whether you're cloning a repository using SSH, working with different branches, or committing your changes, knowing the right Git commands can streamline your workflow.
Creating an emergency commit alias can save you time in urgent situations, allowing you to quickly commit and push changes with a single command. However, it’s important to use such aliases responsibly to avoid pushing unreviewed or incomplete code.
By combining the power of Git’s basic commands with handy aliases, you’ll become more efficient and organized in your development tasks.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
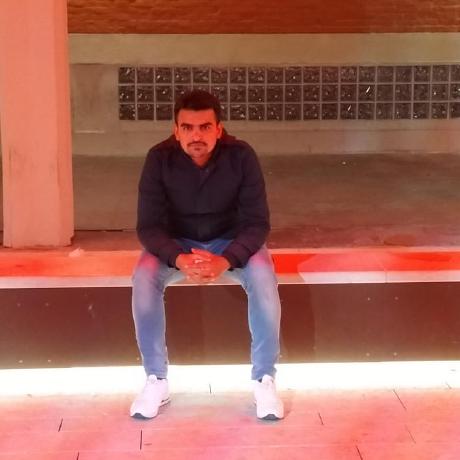
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.