Styling React Components: Options

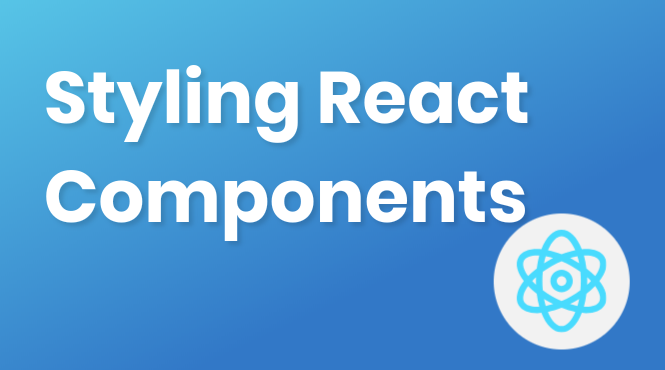
💡 Styling React Components: Flexibility at Its Best 💡
When it comes to styling in React, there’s no single “correct” way. Unlike some frameworks, React doesn’t dictate how you should style your components. Why? Because React is a library, not a framework, it leaves styling decisions entirely up to us.
Here are some popular options for styling React apps:
Inline styles (CSS written as JavaScript objects)
External CSS/SASS files
CSS Modules
Styled Components
Tailwind CSS
🎨 Inline Styling in React Inline styling in React is similar to using the style attribute in HTML. However, in JSX, it’s slightly different. We define our inline styles using JavaScript objects.
Here’s an example:
const style = { color: 'red', fontSize: '48px' };
<h1 style={style}>Styled with inline CSS</h1>
Notice how CSS properties use camelCase (e.g., fontSize), and values must be strings.
📦 External CSS If inline styles become too cumbersome, we can use external CSS files. The process is the same as traditional web development:
Import your CSS file into the React component using import './styles.css';
Use className instead of class in JSX because class is a reserved JavaScript keyword.
For example:
import './styles.css';
<h1 className="header">Styled with external CSS</h1>
💡 Global vs Component-Specific Styles Currently, when we import CSS files, the styles are global, meaning they apply across the entire app. However, as projects scale, it’s better to adopt solutions like CSS Modules or Styled Components to scope styles to individual components.
What’s your favorite way to style React components? Let me know in the comments.
Subscribe to my newsletter
Read articles from Zeeshan Safdar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zeeshan Safdar
Zeeshan Safdar
An accomplished front-end developer with a strong background in creating visually stunning and user-friendly websites and web applications. My deep understanding of web development principles and user-centered design allows me to deliver projects that exceed client expectations. I have a proven track record of success in delivering projects on time and to the highest standards, and I am able to work well in a team environment and am always looking for new challenges to take on.