When (and When Not) to Use GraphQL in Your Rails App

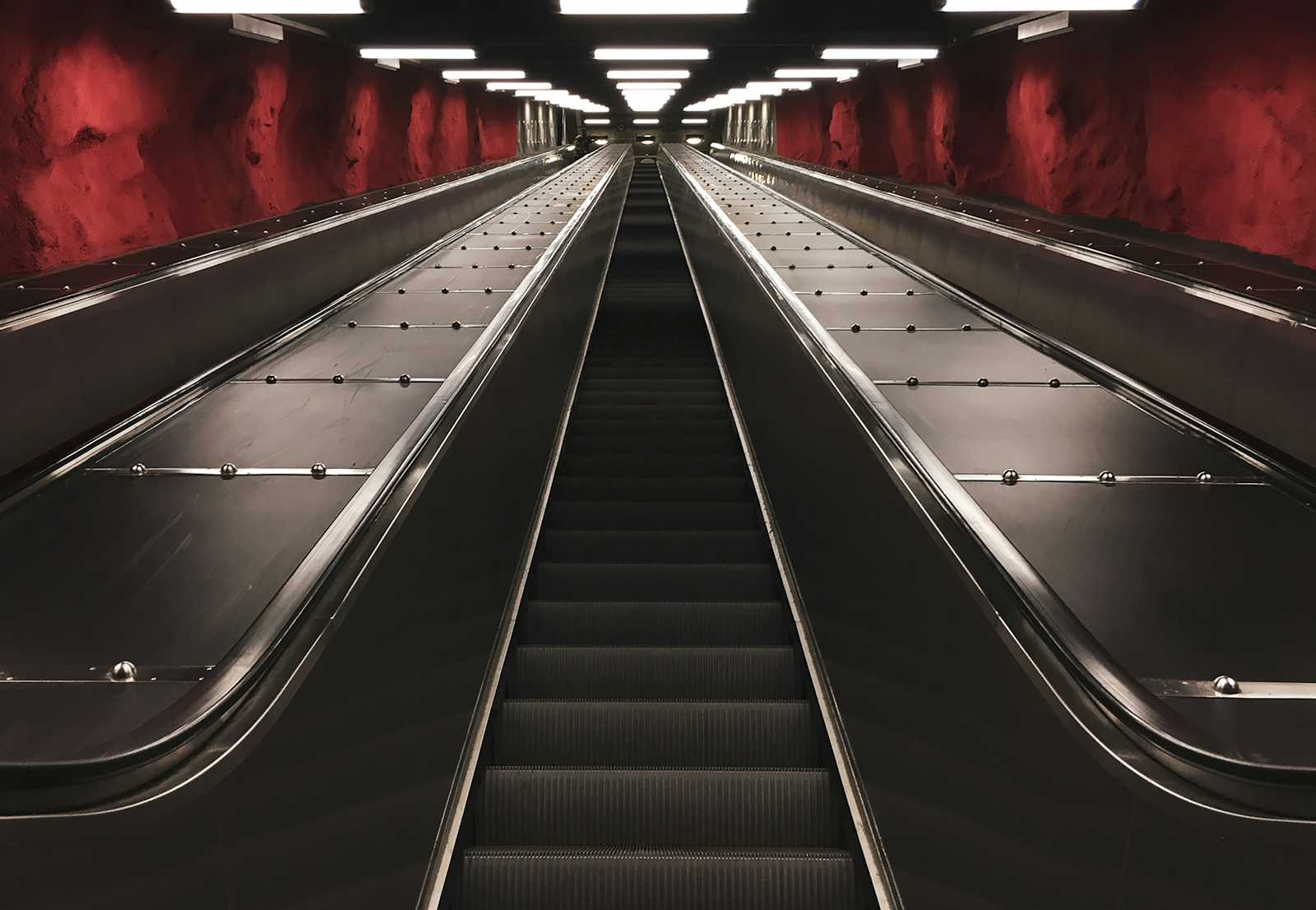
Introduction
GraphQL has emerged as a powerful alternative to REST APIs, offering developers more flexibility and efficiency in data fetching.
However, like any technology, it's not a one-size-fits-all solution.
This article explores when GraphQL is the right choice for your Rails application and when you might want to stick with traditional REST endpoints.
Understanding GraphQL's Value Proposition
Before diving into specific use cases, let's understand what makes GraphQL unique:
Client-Driven Data Fetching: Clients can request exactly the data they need, no more, no less.
Single Endpoint: All queries go through a single endpoint, simplifying API management.
Strong Typing: The schema provides clear contracts between client and server.
Real-time Updates: Built-in support for subscriptions enables real-time features.
Efficient Data Loading: Reduces over-fetching and under-fetching of data.
When to Use GraphQL
1. Complex Data Requirements
GraphQL shines when your application has:
Multiple related models with complex relationships
Different views requiring varying data shapes
Nested data structures that would require multiple REST endpoints
2. Mobile Applications
Mobile apps particularly benefit from GraphQL because:
Network bandwidth is often limited
Battery life is affected by network calls
Different screen sizes and devices need different data shapes
Offline functionality requires precise data synchronization
3. Microservices Architecture
GraphQL serves as an excellent aggregation layer when:
Multiple microservices need to be queried
Data needs to be combined from different sources
Service boundaries are complex
Client needs to access multiple services efficiently
4. Rapid Frontend Development
Consider GraphQL when:
Frontend teams need to move quickly without backend changes
Multiple teams are working on different parts of the application
UI requirements change frequently
Prototyping needs to be fast and flexible
5. Real-time Features
GraphQL subscriptions are valuable for:
Live updates in collaborative features
Real-time dashboards
Chat applications
Live notifications
When Not to Use GraphQL
1. Simple CRUD Applications
Traditional REST might be better when:
Your application primarily performs basic CRUD operations
Data relationships are straightforward
You have few models and endpoints
Client requirements are simple and stable
2. File Upload Heavy Applications
REST is often more suitable when:
Your application handles large file uploads
You need simple binary data transfers
File processing is a core feature
You need direct CDN integration
3. Small Teams with Limited Resources
Stick to REST if:
Your team is small and learning curve is a concern
You need to deliver quickly with familiar tools
You don't have GraphQL expertise
Maintenance resources are limited
4. Highly Cached Public APIs
REST might be preferable when:
You need aggressive caching
Your API is public-facing with many consumers
CDN caching is crucial for performance
You have predictable data patterns
Implementing GraphQL in Rails 8
Let's look at three practical examples of implementing GraphQL in a Rails 8 application.
Example 1: Basic Setup and Query
First, let's set up a basic GraphQL endpoint in Rails 8 with a simple query:
# Gemfile
source 'https://rubygems.org'
gem 'rails', '~> 8.0.0'
gem 'graphql', '~> 2.2.0'
# app/graphql/types/query_type.rb
module Types
class QueryType < Types::BaseObject
field :articles, [Types::ArticleType], null: false do
description 'Get all articles'
argument :limit, Integer, required: false
end
def articles(limit: nil)
articles = Article.all
articles = articles.limit(limit) if limit
articles
end
end
end
# app/graphql/types/article_type.rb
module Types
class ArticleType < Types::BaseObject
field :id, ID, null: false
field :title, String, null: false
field :content, String, null: false
field :author, Types::UserType, null: false
field :comments_count, Integer, null: false
def comments_count
object.comments.count
end
end
end
# app/controllers/graphql_controller.rb
class GraphqlController < ApplicationController
def execute
result = BlogSchema.execute(
params[:query],
variables: ensure_hash(params[:variables]),
context: { current_user: current_user },
operation_name: params[:operationName]
)
render json: result
rescue StandardError => e
raise e unless Rails.env.development?
handle_error_in_development(e)
end
end
This example shows the basic setup of a GraphQL endpoint in Rails 8. It includes:
Basic schema setup
A query type for fetching articles
Field definitions with types
A controller to handle GraphQL requests
Example 2: Complex Mutations with Input Types
Let's implement a more complex mutation with input validation:
# app/graphql/types/article_input_type.rb
module Types
class ArticleInputType < Types::BaseInputObject
argument :title, String, required: true
argument :content, String, required: true
argument :category_ids, [ID], required: false
argument :tags, [String], required: false
end
end
# app/graphql/mutations/create_article.rb
module Mutations
class CreateArticle < BaseMutation
argument :article_input, Types::ArticleInputType, required: true
field :article, Types::ArticleType, null: true
field :errors, [String], null: false
def resolve(article_input:)
article = Article.new(
title: article_input.title,
content: article_input.content,
author: context[:current_user]
)
if article_input.category_ids
article.categories = Category.where(id: article_input.category_ids)
end
if article_input.tags
article.tags = article_input.tags.map do |tag_name|
Tag.find_or_create_by(name: tag_name)
end
end
if article.save
{
article: article,
errors: []
}
else
{
article: nil,
errors: article.errors.full_messages
}
end
rescue ActiveRecord::RecordInvalid => e
{
article: nil,
errors: e.record.errors.full_messages
}
end
end
end
This example demonstrates:
Input type definitions
Complex mutation handling
Error handling
Association management
Input validation
Example 3: Subscriptions for Real-time Updates
Here's how to implement real-time updates using GraphQL subscriptions:
# app/graphql/types/subscription_type.rb
module Types
class SubscriptionType < Types::BaseObject
field :article_updated, Types::ArticleType, null: false do
argument :id, ID, required: true
description 'Subscribe to article updates'
end
field :new_comment, Types::CommentType, null: false do
argument :article_id, ID, required: true
description 'Subscribe to new comments on an article'
end
def article_updated(id:)
article = Article.find(id)
# Return the article object which will be used as the subscription payload
article
end
def new_comment(article_id:)
article = Article.find(article_id)
# Return the newly created comment
article.comments.last
end
end
end
# config/initializers/graphql_subscriptions.rb
class BlogSchema < GraphQL::Schema
use GraphQL::Subscriptions::ActionCableSubscriptions
subscription(Types::SubscriptionType)
end
# app/channels/graphql_channel.rb
class GraphqlChannel < ApplicationCable::Channel
def subscribed
@subscription_ids = []
end
def execute(data)
result = BlogSchema.execute(
query: data["query"],
context: {
channel: self,
current_user: current_user
},
variables: ensure_hash(data["variables"]),
operation_name: data["operationName"]
)
payload = {
result: result.to_h,
more: result.subscription?
}
@subscription_ids << result.context[:subscription_id] if result.context[:subscription_id]
transmit(payload)
end
def unsubscribed
@subscription_ids.each do |sid|
BlogSchema.subscriptions.delete_subscription(sid)
end
end
end
This example shows:
Subscription type definition
Action Cable integration
Real-time updates handling
Channel setup for WebSocket communication
Subscription management
Best Practices for GraphQL in Rails
1. Performance Optimization
Batch Loading with graphql-batch: Instead of loading records one by one (causing N+1 queries), graphql-batch allows you to load multiple records in a single database query, significantly improving performance.
Connection Types for Pagination: Helps manage large datasets by implementing cursor-based pagination, ensuring efficient data loading and better user experience.
Caching Complex Resolvers: Store results of computationally expensive resolvers in cache to reduce database load and improve response times.
Monitor N+1 Queries: Keep track of unnecessary repeated database queries that can slow down your application. Tools like bullet gem can help identify these issues.
Fragment Caching: Cache portions of your GraphQL responses to avoid recalculating frequently requested data.
2. Security Considerations
Proper Authentication: Ensure users are who they claim to be, typically using JWT tokens or session-based authentication.
Field-level Authorization: Control access to specific fields based on user permissions, not just entire queries.
Rate Limiting: Prevent abuse by limiting how many queries a client can make in a given time period.
Query Complexity Limits: Set maximum complexity scores for queries to prevent resource-intensive operations.
Input Validation: Thoroughly validate all input data to prevent security vulnerabilities and ensure data integrity.
3. Testing
Schema Tests: Verify your GraphQL schema is correctly defined and maintains backward compatibility.
Independent Resolver Testing: Test each resolver in isolation to ensure proper data handling.
Complex Query Integration Tests: Test how different parts of your schema work together in real-world scenarios.
Error Scenario Testing: Verify your application handles errors gracefully and returns appropriate error messages.
Subscription Testing: Ensure real-time updates work correctly and maintain connection stability.
4. Documentation
Clear Field Descriptions: Each field should have clear, concise descriptions of its purpose and usage.
Thorough Mutation Documentation: Detail what each mutation does, its inputs, possible outputs, and error cases.
Example Queries: Provide working example queries to help developers understand how to use your API.
Up-to-date Schema Docs: Keep documentation synchronized with schema changes.
Meaningful Naming: Use clear, consistent naming conventions for types, fields, and mutations.
Transitioning from REST to GraphQL
1. Gradual Migration Strategy
New Features First: Start by implementing new features in GraphQL rather than modifying existing ones.
Gradual Endpoint Migration: Convert REST endpoints to GraphQL queries/mutations one at a time.
Parallel Running: Maintain both REST and GraphQL APIs during transition to minimize disruption.
Performance Monitoring: Track how the transition affects application performance.
Team Training: Ensure team members understand GraphQL concepts and best practices.
2. Common Challenges
Schema Design: Making decisions about type organization and relationship modeling.
N+1 Problems: Managing efficient data loading patterns.
Caching: Implementing effective caching strategies for GraphQL queries.
Auth Implementation: Adapting authentication and authorization for GraphQL context.
Learning Curve: Managing team adaptation to new GraphQL concepts and patterns.
3. Migration Tools
Schema Generators: Tools that help convert REST endpoints to GraphQL schemas.
REST Adapters: Libraries that help bridge REST and GraphQL during transition.
Monitoring Tools: Solutions for tracking GraphQL performance and usage.
Doc Generators: Tools that automatically generate API documentation.
Testing Utils: Specialized testing tools for GraphQL implementations.
Monitoring and Maintenance
1. Performance Monitoring
Resolver Timing: Track how long different resolvers take to execute.
Query Complexity: Monitor the complexity of incoming queries.
N+1 Detection: Continuously watch for and address N+1 query issues.
Cache Analysis: Track cache effectiveness and hit rates.
Memory Usage: Monitor application memory consumption patterns.
2. Error Tracking
Resolver Errors: Track and analyze errors occurring in resolvers.
Failed Queries: Monitor and understand why queries fail.
Validation Errors: Track input validation failures and patterns.
Timeout Monitoring: Watch for and address query timeout issues.
Error Analysis: Analyze error patterns to identify systemic issues.
3. Documentation Maintenance
Schema Updates: Keep schema documentation current with changes.
Breaking Changes: Document any changes that could break client applications.
Changelog: Maintain a detailed log of API changes.
Query Examples: Update example queries as the schema evolves.
Best Practices: Document and update recommended usage patterns.
These practices help ensure a robust, performant, and maintainable GraphQL implementation in your Rails application.
Each aspect requires ongoing attention and refinement as your application grows and evolves.
Conclusion
GraphQL in Rails 8 offers powerful capabilities for building flexible and efficient APIs.
While it's not suitable for every project, it excels in complex applications with varying data requirements and real-time features.
The key to success lies in careful evaluation of your project's needs and proper implementation of GraphQL features.
Remember that the decision to use GraphQL should be based on your specific requirements, team expertise, and project constraints.
When implemented correctly, GraphQL can significantly improve your API's flexibility and efficiency, but it's essential to weigh the benefits against the added complexity and learning curve.
Consider starting with a hybrid approach if you're unsure, implementing GraphQL for new features while maintaining existing REST endpoints. This allows your team to gain experience with GraphQL while minimizing risk to existing functionality.
Subscribe to my newsletter
Read articles from Chetan Mittal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mittal
Chetan Mittal
I stumbled upon Ruby on Rails beta version in 2005 and has been using it since then. I have also trained multiple Rails developers all over the globe. Currently, providing consulting and advising companies on how to upgrade, secure, optimize, monitor, modernize, and scale their Rails apps.