Creating RESTful APIs with Wix: A Step-by-Step Guide for Integrators

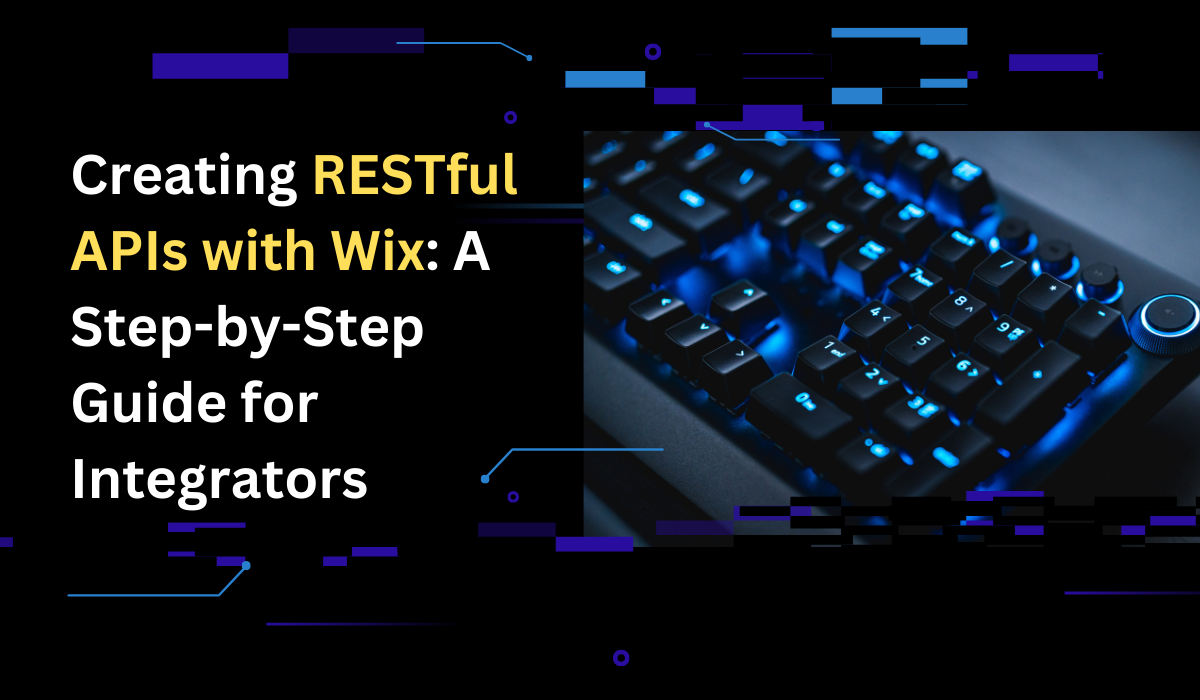
APIs represent the back muscles of modern web development today, offering seamless and myriad inter-application communication in the digital landscape. Although positioned more or less as a nifty website builder, Wix also provides robust serverless capabilities through its platform named Velo Wix with the help of which developers can create RESTful APIs effectively. Through this documentation, you will be guided step by step on how to create a RESTful API in Wix, making it even more achievable for those new to web development.
What is a RESTful API?
A RESTful API (Representational State Transfer) is an architecture of style that relies on the standard HTTP methods (GET, POST, PUT, DELETE) so that any client, like a browser, can communicate with a server. RESTful APIs are also stateless; that is, each request from a client contains all the information the server needs to fulfill that request.
Why Wix for Your API Requirements?
Advantages of using Wix for building APIs:
Server Management: There's no server management when building your API, thanks to Wix managing the server infrastructure.
Development Environment: The Velo development environment gives you tools, libraries, and a code editor all right in Wix.
Approachable User Interface: With its powerful abilities, Wix still stays approachable, which helps for rapid development.
Step 1: Setting Up Your Wix Site
Before you begin building your API, you will need a Wix account and have or create at least one site to perform the work on your API.
Create your Wix account and sign in
Choose a template or start a blank page. For this tutorial, a simple website will be utilized
Enable Velo: Once you have your site built, click on the toggle for "Dev Mode", located underneath the top menu bar, to enable Velo
Step 2: Determine Your API Needs
Describe what your API will do. For instance, if you wanted to have a simple task management application API where it would allow users to do the following.
Retrieve existing tasks
Add new tasks
Update existing ones
Delete some of them
Example Task Structure
{
"id": "1",
"title": "Sample Task",
"completed": false
}
Step 3: Create a Database Collection
Here, using Wix, you can create a collection to keep and manage the data. I will
Navigate to the left sidebar and click on the icon "Database"
click on the button "New Collection" and name it "Tasks"
Define fields for the collection "Tasks"
title (Text)
completed (Boolean)
Step 4: Your API Endpoints
Now let's create your API endpoints.
In Velo, you would create API endpoints using the wix-http-functions
module, so your Wix site can know how to respond to HTTP requests.
Go to the Site Structure panel and scroll down to the Backend section.
Create a new
.js
file such astaskApi.js
.
Sample Code for the Task API
Here is what it should look like:
import { ok, notFound, badRequest } from 'wix-http-functions';
import wixData from 'wix-data';
export function get_tasks(request) {
return wixData.query("Tasks")
.find()
.then(results => {
return ok({ tasks: results.items });
})
.catch((error) => {
console.error(error);
return badRequest("Failed to retrieve tasks.");
});
}
export function post_tasks(request) {
return request.body
.then((body) => {
const { title, completed } = body;
if (!title) {
return badRequest("Task title is required.");
}
const toInsert = {
title: title,
completed: completed || false,
};
return wixData.insert("Tasks", toInsert)
.then((result) => {
return ok({ task: result });
})
.catch((error) => {
console.error(error);
return badRequest("Failed to add task.");
});
});
}
export function put_tasks(request) {
return request.body
.then((body) => {
const { id, title, completed } = body;
if (!id) {
return badRequest("Task ID is required.");
}
const toUpdate = {
_id: id,
title: title,
completed: completed,
};
return wixData.update("Tasks", toUpdate)
.then((result) => {
return ok({ task: result });
})
.catch((error) => {
console.error(error);
return badRequest("Failed to update task.");
});
});
}
export function delete_tasks(request) {
const id = request.path[0]; // The first path parameter is the ID
return wixData.remove("Tasks", id)
.then((result) => {
if (result) {
return ok({ message: "Task deleted successfully." });
} else {
return notFound("Task not found.");
}
})
.catch((error) => {
console.error(error);
return badRequest("Failed to delete task.");
});
}
Code Breakdown
GET
/tasks
: Fetches all tasks from theTasks
collection.POST
/tasks
: Creates a new task in theTasks
collection.PUT
/tasks
: Updates an existing task by its ID.DELETE
/tasks/{id}
: Deletes a task by its ID.
Step 5: Testing Your API
You should test your API after you create it. You can send HTTP requests using Postman or URL to test your API.
Get All Tasks:
Method: GET
URL:
https://<your-wix-site>.
wixsite.com/_functions/tasks
Create a New Task:
Method: POST
URL:
https://<your-wix-site>.
wixsite.com/_functions/tasks
Body:
{
"title": "New Task",
"completed": false
}
Update a Task:
Method: PUT
URL:
https://<your-wix-site>.
wixsite.com/_functions/tasks
Body:
{
"id": "1",
"title": "Updated Task",
"completed": true
}
Delete a Task:
Method: DELETE
URL:
https://<your-wix-site>.
wixsite.com/_functions/tasks/1
Step 6: Security of Your API
Any part of an API is not exempted from security. Wix allows you to add authentication and authorization support for your API. Consider limiting access to your API using the Wix built-in user login functionality or even an authentication provider such as Auth0.
Use Wix Users API: Only service your API endpoints to authenticated users.
Add Validation: Validate incoming data always, and ensure that the API you build deals with invalid requests efficiently.
Building RESTful API using Wix's Velo is a pretty simple process, taking advantage of the strength of Wix's infrastructure and providing considerable customization at the same time. As you've read this guide, you can build a fully functional API that'll act as the backend for many applications. Thus, the ease of use combined with pretty powerful features makes this such an attractive option for Wix developers in the process of building web applications quickly and efficiently.
This is the beauty of your API: considering you now have one, you are good at going and adding whatever functionality to it, integrating it with other services, and scaling your application when necessary. Happy coding!
Subscribe to my newsletter
Read articles from Saurabh Dhariwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Saurabh Dhariwal
Saurabh Dhariwal
Saurabh is a qualified engineer with more than a decade of proven experience in solving complex problems with scalable solutions, harnessing the power of PHP frameworks/CMS with a keen eye on UX.