How to Use Python for DevOps: Essential Skills and Tools for Beginners (Part-1)
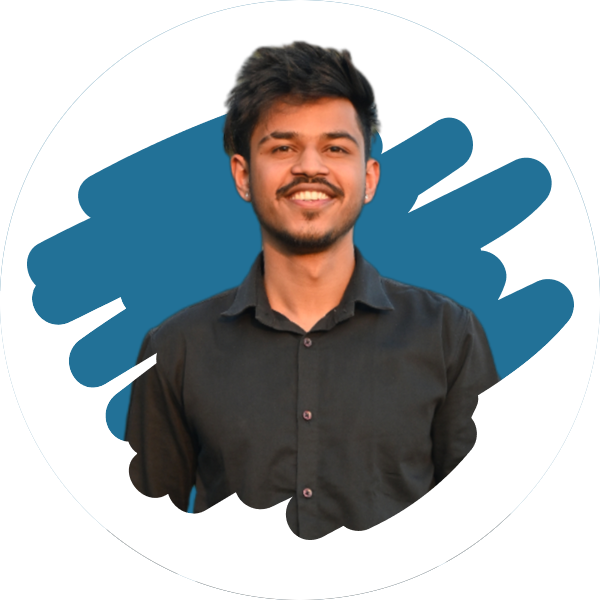
Table of contents
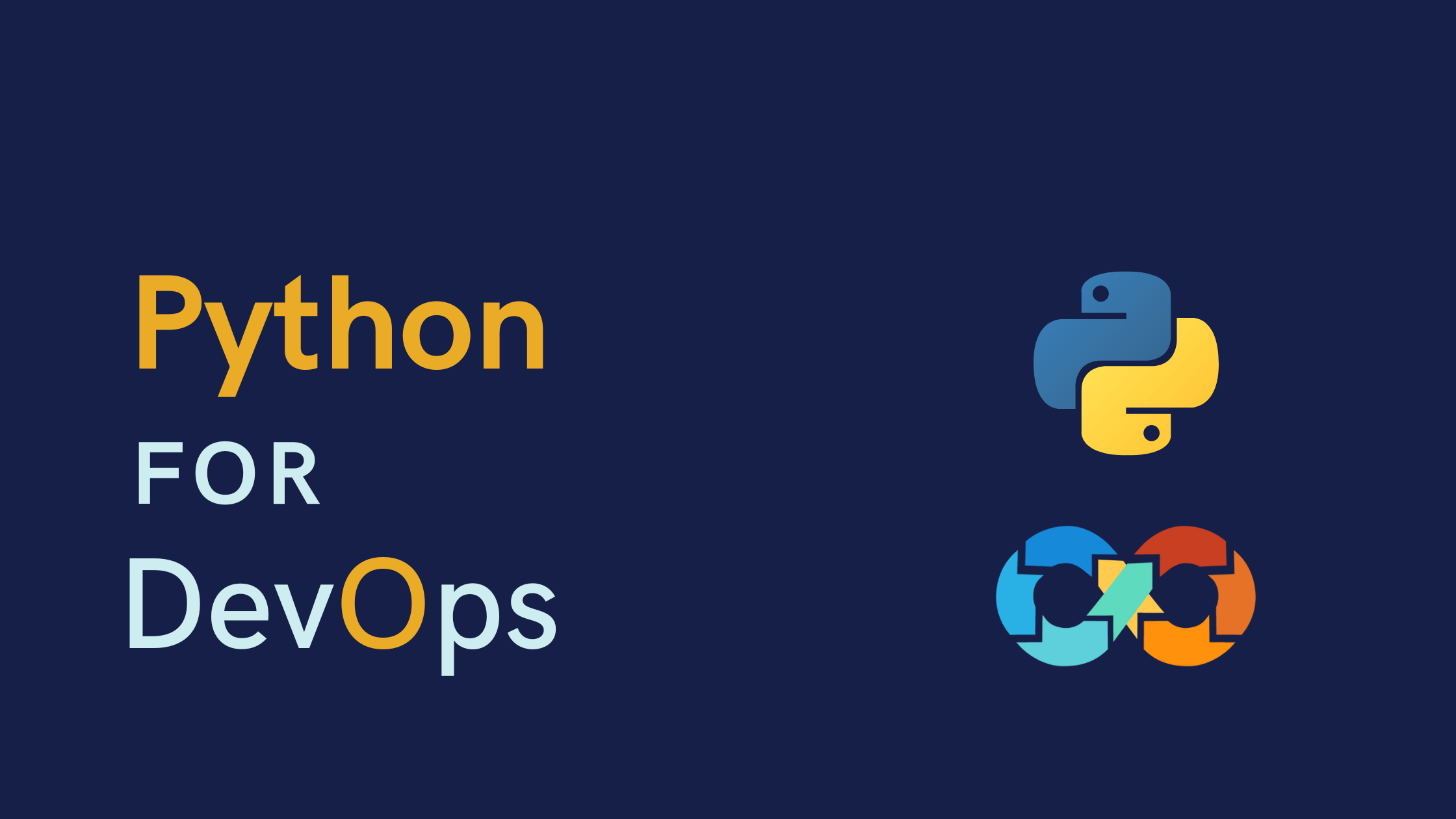
💡 Introduction
Welcome to the world of DevOps! Today, we're tackling one of the most frequently asked questions in the DevOps community: How much Python is needed for DevOps? This blog will take you through everything essential in Python, from beginner to advanced topics, to equip you with practical skills for DevOps tasks.
In this blog, we’ll cover:
Python Basics: An introduction to Python’s core features, including installation, configuration, and data types.
Key Programming Elements: Strings, numbers, variables, keywords, operators.
This is the Part-1 of the blog. Next part of the blog will cover:
Data Structures: if-else conditions, and loops, lists, dictionaries, and sets—essential for organizing and managing data.
Advanced Features for DevOps: Functions, modules and packages, environment variables, and CLI arguments.
Let’s dive in and explore how Python can simplify and empower DevOps practices for you!
💡 Pre-requisites
The best part about this blog? No prerequisites! All you need is a curiosity to learn Python and a willingness to explore how it fits into the DevOps ecosystem. Whether you're completely new to programming or have some experience, this guide is designed to help you get started from scratch. Let's embark on this learning journey together!
💡 Installation
According to the official Python documentation, Python is a “high-level, general-purpose programming language. Its design philosophy emphasizes code readability with significant indentation, and it’s dynamically typed.” Python's simplicity, flexibility, and extensive library support make it a strong choice for DevOps engineers.
First, let’s install Python on your system.
macOS: Use Homebrew to install Python:
brew install python3
Windows: Download the official installer from the Python website.
Linux: Use the following command to install a specific version of Python (replace
XX
with the version number):sudo apt install python3.XX
To confirm the installation, check the Python version:
python3 --version
💡 Why DevOps Engineers Use Python
As DevOps engineers, we often work on Linux-based systems (e.g., EC2 instances and virtual machines), which don’t typically come with a graphical interface. To operate in these environments, we use shell commands—commands for tasks like managing files, viewing system status, and more. When we organize these commands into a script (saved with a .sh
extension), we get a shell script.
While shell scripting is efficient, here are two reasons why Python is a valuable addition:
Cross-Platform Compatibility: Not all systems we work with are Linux-based. Some are Windows-based, and shell scripts often don’t work across both platforms. Python scripts, however, are cross-platform, making them highly adaptable. For instance, Ansible, a widely-used automation tool, is written in Python and runs on both Linux and Windows.
Simplified Complex Operations: For simple tasks like checking storage or memory, shell commands are quick and effective. However, for more complex operations, such as interacting with APIs (e.g., AWS's
Boto3
library), handling data, or filtering information, Python’s modules and libraries make these tasks easier and more manageable.
In short:
Use shell commands for simple, system-based operations.
Use Python for complex tasks requiring cross-platform compatibility, API interactions, or advanced data processing.
💡 Writing Your First Python Program
Let’s create our first Python file. Follow these steps:
Create a Python file named
main.py
and add this content:print("Hello User!")
Run the program:
python3 main.py
If everything is set up correctly, you should see:
Hello, User!
💡 Understanding Basic Data Types in Python
Data types categorize the kind of data that can be held in a variable. In programming, everything is data: for instance, text like "Pravesh"
, numbers like 29
, or booleans like True
. By defining data types, we help the compiler understand how to handle the data stored in our variables.
Here are some of the most common data types in Python:
Numeric Types:
int: Whole numbers, e.g.,
5
float: Numbers with decimals, e.g.,
3.14
complex: Numbers with a real and imaginary part, e.g.,
P = 9 + 5
Sequence Types:
str (String): Text sequences, e.g.,
"Hello, World!"
list: Ordered and mutable sequences, e.g.,
my_list = [1, 2, 3]
tuple: Ordered but immutable sequences, e.g.,
my_tuple = (1, 2, 3)
Mapping Types:
- dict (Dictionary): Stores data in key-value pairs, e.g.,
my_dict = {'name': 'Pravesh', 'age': 21}
- dict (Dictionary): Stores data in key-value pairs, e.g.,
Set Types:
set: Unordered sequences of unique elements, e.g.,
my_set = {1, 2, 3}
frozenset: Immutable sets, e.g.,
frozen = frozenset([1, 2, 3])
Boolean Type:
- bool: Represents
True
orFalse
, e.g.,is_added = True
- bool: Represents
None Type:
- None: Represents the absence of a value, or a null value, e.g.,
my_var = None
- None: Represents the absence of a value, or a null value, e.g.,
Let's Explore Some Data Types in Detail
Strings
Strings are sequences of characters enclosed in single ('...'
), double ("..."
), or triple quotes ('''...'''
). Strings are immutable, meaning we can’t change their content directly, but we can create new strings based on them.
Examples of String Operations:
# Basic string
my_string = "Hello, Pravesh!"
# Accessing individual characters
print(my_string[0]) # Output: H
# String functions
print(len(my_string)) # Length of the string, Output: 14
print(my_string.upper()) # Convert to uppercase, Output: "HELLO, PRAVESH!"
print(my_string.lower()) # Convert to lowercase, Output: "hello, pravesh!"
print(my_string.strip("!")) # Remove trailing '!', Output: "Hello, Pravesh"
print(my_string.replace("Pravesh", "User")) # Replace a substring, Output: "Hello, User!"
# Combining strings
greeting = "Hello, " + "User!" # Output: "Hello, User!"
# Slicing strings
print(my_string[7:14]) # Output: "Pravesh"
# Other methods
print(my_string.startswith("Hello")) # Output: True
print(" ".join(["Welcome", "to", "DevOps!"])) # Output: "Welcome to DevOps!"
Numeric Types
Python supports two main numeric types: int
for integers and float
for decimal numbers. These can be used for mathematical operations such as addition, subtraction, multiplication, and division.
Examples of Numeric Operations:
# Integers and floats
num1 = 10 # Integer
num2 = 3.5 # Float
# Arithmetic operations
print(num1 + num2) # Addition, Output: 13.5
print(num1 - num2) # Subtraction, Output: 6.5
print(num1 * num2) # Multiplication, Output: 35.0
print(num1 / num2) # Division, Output: 2.8571...
# Numeric functions
print(round(3.14159, 2)) # Round to 2 decimal places, Output: 3.14
print(abs(-5)) # Absolute value, Output: 5
These fundamental data types and operations will serve as building blocks for handling more complex tasks in Python, especially in automating DevOps workflows. With these basics, you’re well on your way to writing powerful Python scripts!
💡 Keywords and Variables in Python
Keywords
Keywords in Python are reserved words with specific meanings, and they cannot be used as variable names. Keywords help define the structure and flow of Python programs. Here is a list of keywords that you cannot use for variables:
and
, or
, not
, if
, else
, elif
, while
, for
, in
, try
, except
, catch
, finally
, def
, return
, class
, import
, from
, as
, True
, False
, None
, is
, lambda
, with
, global
, nonlocal
Variables
Variables are names used to store data. They allow us to make code more readable and flexible by holding values that can change or be reused throughout the program.
Example of Creating and Using Variables:
# Assigning a value to a variable
my_variable = 42
# Accessing the value of a variable
print(my_variable) # Output: 42
💡 Variable Scope and Lifetime
In Python, the scope of a variable determines where it can be accessed, and its lifetime determines how long it exists in memory. Python has two main types of variable scope:
Local Scope: Variables defined within a function have a local scope, meaning they can only be accessed inside that function.
Example of Local Scope:
def my_function(): x = 10 # Local variable print(x) my_function() print(x) # This will raise an error because 'x' is only accessible within my_function
Global Scope: Variables defined outside of any function have a global scope and can be accessed throughout the entire program.
Example of Global Scope:
y = 20 # Global variable def another_function(): print(y) # This will access the global variable 'y' another_function() print(y) # Output: 20
Variable Lifetime: The lifetime of a variable depends on its scope:
Local variables exist only while the function is being executed.
Global variables exist for the entire duration of the program.
💡 Naming Conventions for Variables
When creating variable names, consider these conventions to keep your code readable and clear:
Descriptive names: Choose names that explain the purpose of the variable.
Lowercase and underscores: Use lowercase letters, and separate words with underscores (
_
), e.g.,add_user
.Avoid reserved words: Do not use keywords or reserved words.
Meaningful names: Choose names that convey the variable's purpose.
Examples of Good Naming Practices:
# Good variable naming
user_name = "John"
total_items = 42
# Avoid using reserved words
class = "Python" # This will raise an error
# Choose meaningful names
a = 10 # Less clear
num_of_students = 10 # More descriptive
💡 Functions, Modules, Packages, and Virtual Environments in Python
In Python, functions, modules, packages, and virtual environments provide ways to organize, reuse, and manage your code effectively. Here’s a look at each of these concepts:
💡 Functions
A function is a block of code that performs a specific task. Functions make code reusable and organized. In Python, functions are defined using the def
keyword, and they can accept arguments to operate on different values.
Example of a Simple Function:
def greet(name):
print("Hello " + name)
greet("Pravesh") # Output: "Hello Pravesh"
In this example, name
is a parameter in the greet
function, and "Pravesh"
is an argument passed to it when calling the function.
💡 Modules
A module is a Python script (file) containing Python code, such as functions, classes, and variables, that can be used in other scripts. Modules help in organizing and modularizing code for better readability and maintenance.
Creating and Using a Module: Suppose we have a file named my_
module.py
:
# my_module.py
def cube(x):
return x ** 3
pi = 3.14159265
We can use this module in another script by importing it:
import my_module
result = my_module.cube(5)
print(result) # Output: 125
print(my_module.pi) # Output: 3.14
💡 Packages
Packages are collections of related modules organized into directories. Each package contains a special file named __init__.py
, which indicates that the directory should be treated as a package.
Example of Package Structure:
my_package/
__init__.py
module1.py
module2.py
To use functions or variables from modules within a package:
from my_package import module1
result = module1.some_function()
You can import an entire module or specific functions/variables from it:
# Import the entire math module
import math
# Use a function from the math module
result = math.sqrt(16)
print(result) # Output: 4.0
# Import a specific function/variable from the math module
from math import pi
print(pi) # Output: 3.141592653589793
💡 Virtual Environments
A virtual environment is an isolated environment that allows you to manage dependencies for different Python projects separately. By using virtual environments, you can avoid conflicts between dependencies needed by different projects.
Creating and Activating a Virtual Environment
You can create a virtual environment using venv
(built-in) or virtualenv
(a third-party tool). Here’s how to create and activate a virtual environment using venv
:
# Create a virtual environment named 'myenv'
python3 -m venv myenv
# Activate the virtual environment (Linux/macOS)
source myenv/bin/activate
# On Windows
myenv\Scripts\activate
Once activated, you work in an isolated workspace where installed libraries and dependencies won’t affect the system’s global environment.
💡 Command Line Arguments (CLI Args) and Environment Variables (Env Vars)
💡 Why Use CLI Arguments?
CLI (Command Line Interface) arguments allow us to provide input to a program at runtime, which is especially useful in dynamic applications. For example, in a calculator program, instead of hardcoding values, you can accept user inputs for numbers and operators to perform calculations.
Using CLI Arguments
In Python, sys.argv
is used to handle CLI arguments. Each CLI argument is stored in an indexed list, starting from 0. Let’s look at an example:
import sys
# Access the first CLI argument
arg1 = sys.argv[1]
print(arg1)
Running the Script with CLI Args:
python3 main.py Pravesh
Output:
Pravesh
In this case, "Pravesh"
is passed as a CLI argument and stored in sys.argv[1]
, which prints it when the script runs.
💡 Environment Variables (Env Vars)
Environment variables are a secure way to store sensitive information, such as passwords, API keys, tokens, or certificates, outside of your code. This keeps sensitive data safe and accessible only during runtime.
Setting an Environment Variable
To create an environment variable in the terminal, you can use export
:
export PASSWORD="peorshqesnvmrw"
This creates an environment variable named PASSWORD
. By convention, environment variables are written in ALL_CAPS.
Accessing Environment Variables in Python
To use environment variables in Python, you can use the os
module:
import os
# Access the environment variable
password = os.getenv("PASSWORD")
print(password) # This will print the value of PASSWORD, if set
💡 Why Use CLI Args and Env Vars?
CLI Args allow for flexibility, as they enable users to input different values each time the script is run without changing the code.
Environment Variables securely store sensitive data, protecting your program from exposure of confidential information within the code.
Both CLI arguments and environment variables add flexibility and security, especially in DevOps, where scripts often require dynamic input and access to secure credentials.
💡 Operators in Python
Operators in Python are symbols or keywords used to perform operations on values and variables. They are essential for manipulating data and implementing logic in programs. Here’s a breakdown of the main types of operators in Python:
1. Arithmetic Operators
These operators perform basic mathematical operations on numbers:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)**
: Exponentiation (power)//
: Floor division (returns the integer part of division)
Example:
a = 10
b = 3
print(a + b) # Output: 13
print(a - b) # Output: 7
print(a * b) # Output: 30
print(a / b) # Output: 3.3333
print(a % b) # Output: 1
print(a ** b) # Output: 1000
print(a // b) # Output: 3
2. Assignment Operators
These operators assign values to variables.
=
: Assigns the value on the right to the variable on the left.+=
: Adds the right operand to the left operand and assigns the result to the left.-=
: Subtracts the right operand from the left operand and assigns the result to the left.Similarly,
*=
,/=
,%=
,**=
,//=
can be used for other operations.
Example:
x = 5
x += 3 # Equivalent to x = x + 3
print(x) # Output: 8
3. Relational Operators
These operators compare two values and return a boolean (True
or False
).
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
Example:
a = 5
b = 3
print(a == b) # Output: False
print(a > b) # Output: True
4. Logical Operators
Logical operators combine multiple conditions.
and
: ReturnsTrue
if both statements are true.or
: ReturnsTrue
if at least one statement is true.not
: Inverts the boolean value.
Example:
x = True
y = False
print(x and y) # Output: False
print(x or y) # Output: True
print(not x) # Output: False
5. Identity Operators
These operators check if two variables refer to the same object in memory.
is
: ReturnsTrue
if two variables are the same object.is not
: ReturnsTrue
if two variables are not the same object.
Example:
a = [1, 2, 3]
b = a
c = [1, 2, 3]
print(a is b) # Output: True (b points to the same object as a)
print(a is c) # Output: False (c is a different object, even if values are same)
6. Membership Operators
These operators check if a value is present in a sequence (e.g., list, string).
in
: ReturnsTrue
if the value is found in the sequence.not in
: ReturnsTrue
if the value is not found in the sequence.
Example:
my_list = [1, 2, 3, 4]
print(3 in my_list) # Output: True
print(5 not in my_list) # Output: True
7. Bitwise Operators
These operators perform bit-level operations on binary numbers.
&
: Bitwise AND|
: Bitwise OR^
: Bitwise XOR~
: Bitwise NOT<<
: Left shift>>
: Right shift
Example:
a = 4 # Binary: 100
b = 5 # Binary: 101
print(a & b) # Output: 4 (100)
print(a | b) # Output: 5 (101)
print(a ^ b) # Output: 1 (001)
print(~a) # Output: -5 (Two's complement: -100 in binary)
Each of these operators enables you to perform specific tasks with Python variables and values, ranging from simple calculations to complex logical operations, crucial for effective DevOps scripting and automation.
💡 Conclusion
Congratulations on making it this far! You've now covered some fundamental concepts in Python, from data types and variables to functions, modules, and operators. Each of these elements is foundational for building automation scripts and handling DevOps tasks efficiently.
But we’re just scratching the surface! In the upcoming Part 2, we'll dive even deeper, covering advanced topics and implementing a full-fledged Python project tailored for DevOps. This will help solidify your knowledge and show how these concepts apply to real-world scenarios.
Stay tuned for Part 2, coming soon!
🚀 For more informative blog, Follow me on Hashnode, X(Twitter) and LinkedIn.
Till then, Happy Coding!!
Subscribe to my newsletter
Read articles from Pravesh Sudha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
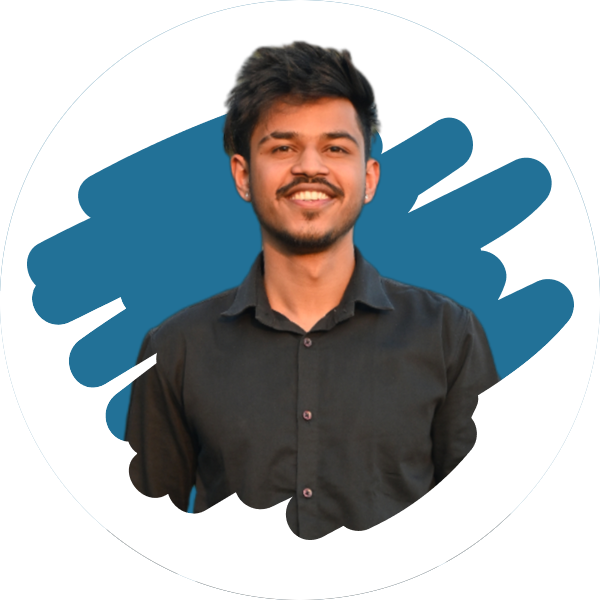
Pravesh Sudha
Pravesh Sudha
I am a Self Taught developer from India with major interest in open source, devops and Coding. Pursuing a Bachelors in Philosophy has helped me a lot to drive right in my career. Landed in tech, now I am exploring tech blogging for fun