Monitoring Disk Space in PowerShell with Automated Email Alerts

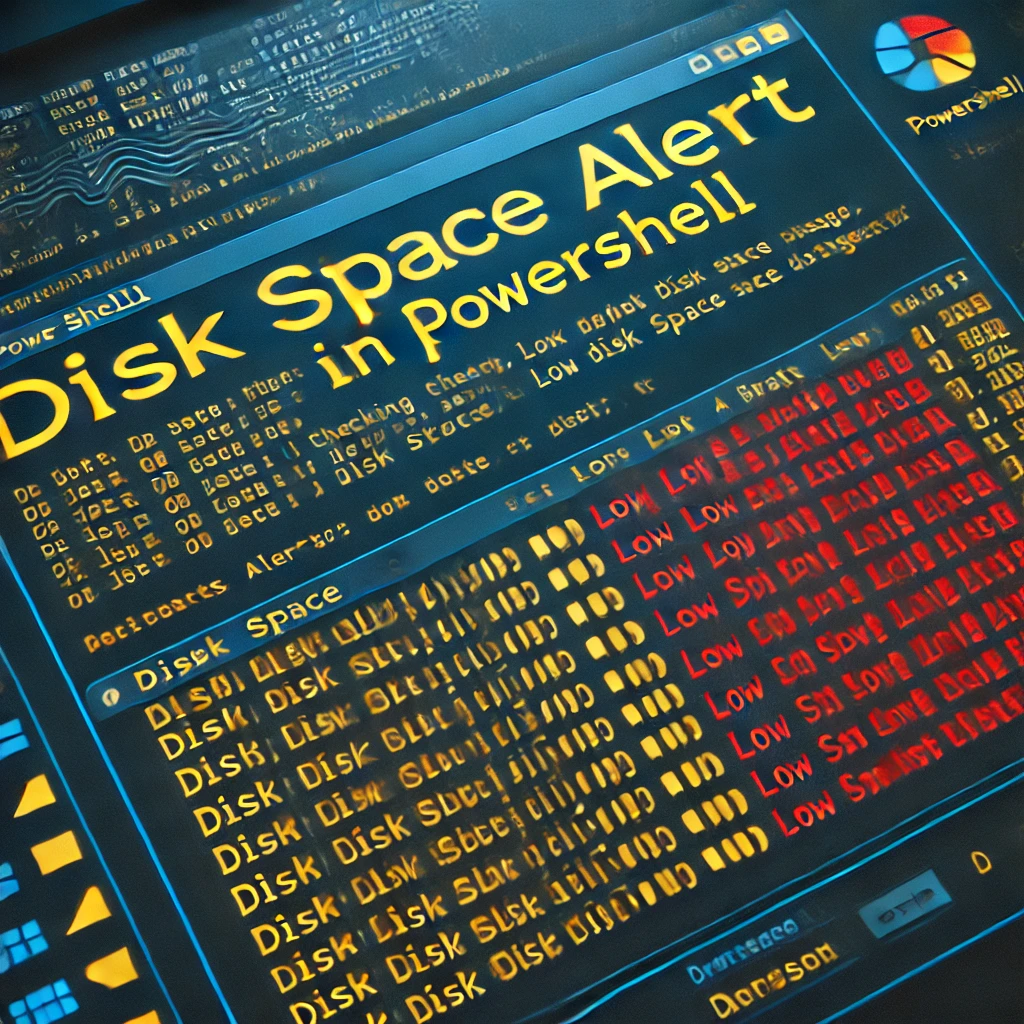
Keeping track of available disk space is crucial for system health and performance. In this blog, I'll walk you through creating a PowerShell script that checks disk space on a specific drive and sends an automated email alert when the free space falls below a defined threshold.
Prerequisites
Basic knowledge of PowerShell scripting
A Gmail account with SMTP access for email alerts
PowerShell installed on your Windows machine
Let's dive into setting up this script step-by-step.
Step 1: Define Thresholds and Drive Configuration
In this script, we’ll check if the free space on the specified drive (e.g., C:
) is below a defined minimum size. Update the drive letter and size threshold according to your needs.
powershellCopy code# Define the drive to monitor and the minimum space threshold
$drives = @("C") # Drives to monitor (e.g., C:)
$minSize = 400GB # Minimum free space threshold
$minSizeGB = [math]::round($minSize / 1GB, 2) # Convert to GB for readability
Step 2: Configure SMTP Email Settings
Next, set up the SMTP email configuration. Ensure you replace the credentials with your Gmail SMTP server details without revealing your password here.
powershellCopy code# SMTP configuration
$email_username = "your_email@gmail.com"
$email_password = "your_password" # Generate an app password for Gmail
$email_smtp_host = "smtp.gmail.com"
$email_smtp_port = 587
$email_smtp_SSL = $true
$email_from_address = "your_email@gmail.com"
$email_to_addressArray = @("recipient_email@gmail.com") # List of recipients
Step 3: Iterate Over Drives and Check Free Space
Now, we’ll use a foreach
loop to check each drive’s available space and compare it to the minimum threshold.
powershellCopy codeforeach ($d in $drives) {
Write-Host "`r`n"
Write-Host "Checking drive $d …"
$disk = Get-PSDrive -Name $d
if ($disk.Free -lt $minSize) {
Write-Host "Drive $d has less than $minSizeGB GB free... sending e-mail…"
# Calculate used and free space in GB for readability
$usedGB = [math]::round($disk.Used / 1GB, 2)
$freeGB = [math]::round($disk.Free / 1GB, 2)
Step 4: Construct the Email Message in Table Format
To create a well-structured email body, let’s use HTML to format the information in a table. This is ideal for clear, easy-to-read alerts.
powershellCopy code # Prepare HTML formatted email message
$message = New-Object Net.Mail.MailMessage
$message.IsBodyHtml = $true # Enable HTML body format
$message.From = $email_from_address
foreach ($to in $email_to_addressArray) {
$message.To.Add($to)
}
$message.Subject = "[SPACE-ALERT] WARNING: $env:computername drive $d has less than $minSizeGB GB free"
$message.Body = @"
<h3>ALERT: Low Disk Space Detected!</h3>
<p>This is an automatic alert to inform you that <b>$env:computername</b> drive $d is running low on free space.</p>
<table border="1" cellpadding="5" cellspacing="0" style="border-collapse: collapse; width: 60%;">
<tr style="background-color: #f2f2f2;">
<th>Machine HostName</th>
<th>Drive</th>
<th>Used Space (GB)</th>
<th>Free Space (GB)</th>
<th>Threshold (GB)</th>
</tr>
<tr>
<td>$env:computername</td>
<td>$d</td>
<td>$usedGB</td>
<td>$freeGB</td>
<td>$minSizeGB</td>
</tr>
</table>
<p>This warning is triggered when the free space falls below $minSizeGB GB.</p>
<p>Best Regards,<br>Your Monitoring System</p>
"@
Step 5: Send the Email Alert
Using the SMTP client, we can send the email with the table format whenever the free space falls below the threshold. Ensure you handle potential errors with a try-catch block to log issues if the email fails to send.
powershellCopy code # Create and configure SMTP client
$smtp = New-Object Net.Mail.SmtpClient($email_smtp_host, $email_smtp_port)
$smtp.EnableSSL = $email_smtp_SSL
$smtp.Credentials = New-Object System.Net.NetworkCredential($email_username, $email_password)
try {
$smtp.Send($message)
Write-Host "… E-Mail sent!"
} catch {
Write-Host "Failed to send email:" $_.Exception.Message
}
$message.Dispose()
} else {
Write-Host "Drive $d has more than $minSize bytes free: nothing to do."
}
}
Summary
This PowerShell script is a straightforward way to automate disk space monitoring and receive email alerts in a clean, table-formatted report when space is running low. Here’s what we covered:
Setting Up Drive and Space Thresholds: Specify drives and minimum space.
SMTP Configuration: Configuring email for alerts.
Checking Drive Space: Loop through drives and monitor available space.
Formatting Email in Table Form: Use HTML for a clean, structured email body.
Sending Alerts: Use SMTP to send emails automatically when disk space is low.
This script helps you maintain a healthy system by proactively notifying you when you need to free up space. Try it out and tweak the configurations to fit your system requirements. Happy monitoring!
Subscribe to my newsletter
Read articles from Zahoor Farooq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Zahoor Farooq
Zahoor Farooq
Code enthusiastic fr