Breaking Out of the Root: A Deep Dive into React Portals
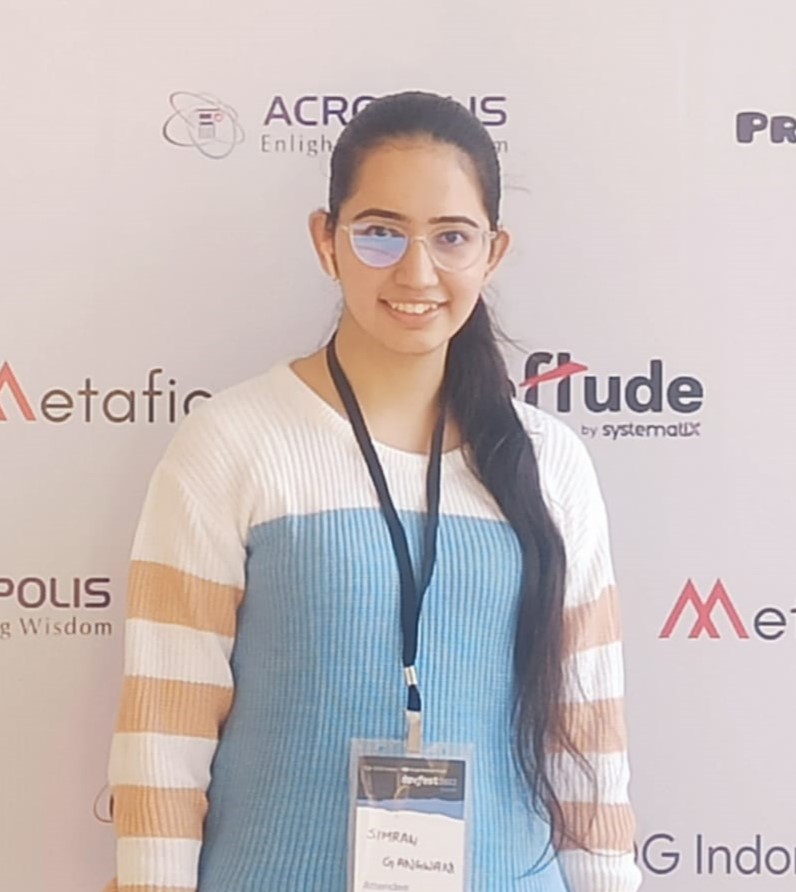
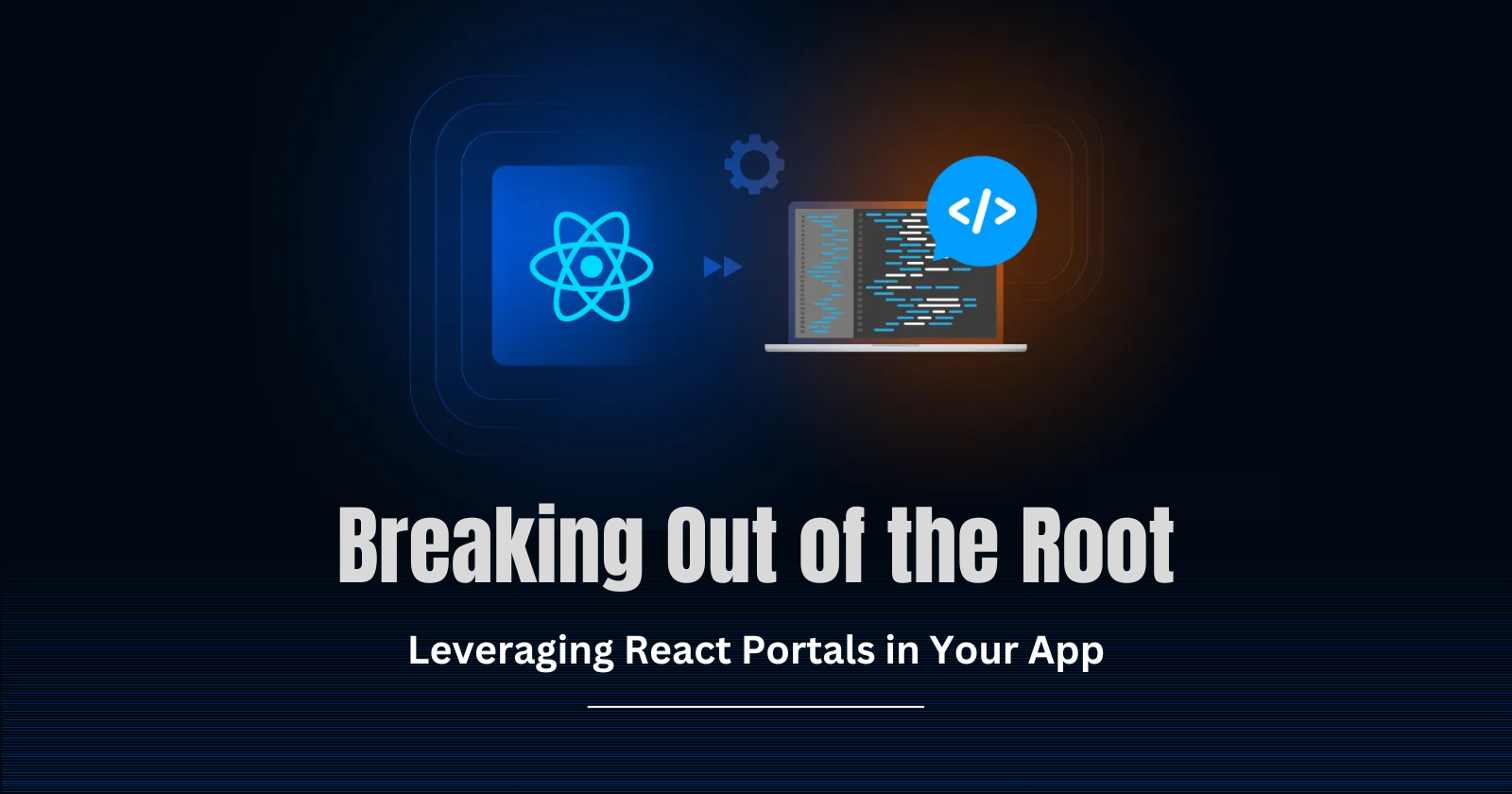
React is all about organizing components in a structured hierarchy, giving us the flexibility to create multiple components independently while rendering them within a single DOM node, typically called the “root”. But what if a component needs to step outside this boundary and render elsewhere in the DOM tree? React Portals allow us to break out of this conventional approach, enabling components to exist outside the root node while still maintaining complete control. Sounds intriguing, right? Join me as we uncover the magic behind React Portals!
Introduction
Imagine a scenario where you need to build a modal, pop-up, or tooltip that should appear on top of everything in your app. Within the traditional React DOM hierarchy, these components might feel a bit constrained. React Portals allow us to move beyond these limitations by rendering components outside the root DOM element, unlocking new potential for flexible UI design.
In simpler terms, React Portals give you the ability to render components outside of the root div
. They allow you to place children into a DOM node that exists outside the DOM hierarchy of the parent component.
What does this mean? Typically, React applications are mounted onto a single DOM element in the HTML file, often referred to as the root
. If you examine the DOM tree in your browser, you’ll see that all React components are nested within this root element. So, while all components in a React app are children of the main App
component, and the App
component itself is mounted to the root node, React Portals enable us to break out of this structure and mount components onto any DOM node of our choosing.
With React Portals, you can render a component onto a DOM node that exists outside the root element, bypassing the conventional DOM tree.
Why would we need this? Let’s dive into some use cases for React Portals to understand their value more fully.
Use Cases of React Portals
Now that we understand the capabilities of React Portals, the next question is: when should we use them? Here are some key use cases where portals can significantly enhance component behavior and styling flexibility:
Modals: Modals overlay the entire page to display information on top of all other elements, regardless of nesting depth. By rendering modals outside the main DOM hierarchy, we can avoid styling conflicts, particularly with
z-index
and positioning issues, ensuring they appear prominently as intended.Tooltips, Toasts & Popovers: These components often appear at specific positions on the page or in relation to other elements. Rendering them outside the standard component tree avoids potential conflicts in positioning, allowing them to display accurately without interference.
Overlays & Notifications: Elements like loading screens or notifications often need to display on top of everything else in the application. Rendering them outside the root div ensures they are not impacted by styling from other components, providing seamless visibility and functionality.
Dropdown Menus & Drawers: Dropdowns and drawers often need to be displayed over other content without being clipped or hidden by
overflow: hidden;
settings or adjacent component styling. By rendering these components separately with portals, they are free from parent constraints, allowing them to position properly and remain visible across the app.
Using React Portals in these scenarios helps streamline the user experience, ensures positioning accuracy, and minimizes the chance of styling conflicts.
Benefits of Using React Portals
React Portals offer a range of benefits, particularly when handling complex UI requirements. Here are some key advantages:
Enhanced Styling Control with Fewer Conflicts: By rendering components outside the typical DOM hierarchy, portals help to minimize CSS conflicts with parent and sibling elements. This allows for precise component placement and improved control over styling, making it easier to avoid issues related to positioning and layering.
Improved Performance: Portals enhance application efficiency by minimizing unnecessary re-renders. Since components rendered through portals operate outside the main component tree, they can update independently, minimizing the impact on the rest of the application.
Streamlined Code Organization: Portals allow the separation of UI and logic in the component tree, improving the clarity and maintainability of the code. This separation supports a more organized code structure, making it easier for developers to understand and maintain.
Improved Accessibility: By positioning UI elements like modals and tooltips at the top level of the DOM, portals enhance accessibility, making it easier for screen readers to navigate and interpret the content accurately. This contributes to a more inclusive user experience across the application.
Implementing Portals
Portals are created using the createPortal()
method provided by ReactDOM
since React 16. This method accepts two arguments:
The JSX to render, which could be string, number, elements, or components.
The DOM node where this JSX will be mounted.
Let’s walk through creating a modal using React Portals.
Step 1: Setting Up the Portal DOM Node
To start, we need a DOM node outside the root element to act as a container for the portal. Add a new div
element below the root element in the index.html
file.
<!-- index.html -->
<body>
<div id="root"></div>
<div id="modal-root"></div> <!-- Portal container for modal -->
</body>
Step 2: Creating the Modal Component
Next, we’ll create the Modal
component. We’ll use ReactDOM.createPortal()
to render this component outside the main React DOM hierarchy.
// Modal.js
import React from "react";
import ReactDOM from "react-dom";
const Modal = ({ isOpen, onClose, children }) => {
if (!isOpen) return null;
return ReactDOM.createPortal(
<div className="overlay">
<div className="my-modal">
<button onClick={onClose} className="modal-close">
✕
</button>
{children}
</div>
</div>,
document.getElementById("modal-root")
);
};
export default Modal;
In this example, document.getElementById('modal-root')
is passed as the second argument, instructing React to render the modal within the modal-root
container defined in index.html
.
Step 3: Using the Modal Component in the App Component
We can now use the Modal
component within the App
component. Here, we’ll set up a state to control the modal’s visibility and toggle it with a button click.
// App.js
import "./modal.css";
import React, { useState } from "react";
import Modal from "./Modal";
const App = () => {
const [isModalOpen, setModalOpen] = useState(false);
return (
<div className="App">
<button onClick={() => setModalOpen(true)}>View Modal</button>
<Modal isOpen={isModalOpen} onClose={() => setModalOpen(false)}>
<h2>Modal's Heading</h2>
<p>Here comes the content of your modal.</p>
</Modal>
</div>
);
};
export default App;
When the "View Modal" button is clicked, isModalOpen
is set to true
, rendering the modal component.
Step 4: Styling our Modal
Finally, let’s add basic styling for the modal.
/* modal.css */
.overlay {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.7);
display: flex;
align-items: center;
justify-content: center;
}
.my-modal {
background: white;
padding: 16px;
border-radius: 0.5rem;
width: 50vw;
position: relative;
}
.modal-close {
position: absolute;
top: 10px;
right: 10px;
border: none;
background-color: transparent;
cursor: pointer;
}
Digging Deep into the Dev Tools
In the browser, you’ll still see the button and modal displayed as expected. However, if you inspect the elements, you’ll notice that the modal component now falls under the modal-root
DOM node instead of the conventional root
DOM node.
In a React application, while all components are typically children of the root component (mounted on the root DOM node), React Portals provide the flexibility to render components outside this main structure. This allows you to mount any component onto a DOM node of your choice, offering greater control over your app's DOM structure.
Limitations with Portal
While React Portals provide powerful functionality and flexibility, there are a few key limitations and considerations to keep in mind:
Event Bubbling: Although portals can render components outside the root DOM node, they still behave like typical React children in many respects, including event bubbling. This means that events triggered inside a portal will propagate up through the React tree, even if the component’s actual position in the DOM differs from its parent.
Cross-Browser Compatibility: While portals work seamlessly in most modern browsers, they may introduce inconsistencies in older browsers.
Server-Side Rendering (SSR): Since portals render components outside the component hierarchy, they can present challenges in server-side rendering scenarios. Conditional rendering is often required to manage these issues effectively.
Testing Complexity: When testing components that use portals, additional configuration is often necessary to handle unit and integration tests. This added complexity can make testing more intricate.
Conclusion
React Portals bring a new level of flexibility to component placement, enabling elements to step outside the traditional DOM hierarchy and appear exactly where they’re needed. While there are unique considerations, such as accessibility and styling, portals empower developers to build dynamic, layered interfaces that enhance user experience without sacrificing maintainability. By embracing portals, developers can go beyond conventional boundaries and leverage the entire DOM to create seamless, user-focused applications.
Remember, sometimes the most effective place for a component is outside the usual box.
» NOTE: For a practical implementation of a modal using React Portals, you can refer to my GitHub Repository along with the live link: Modal Using Portal This example provides code and insights into creating modals that render outside the main DOM hierarchy, perfect for accessible and flexible UI components.
Subscribe to my newsletter
Read articles from Simran Gangwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
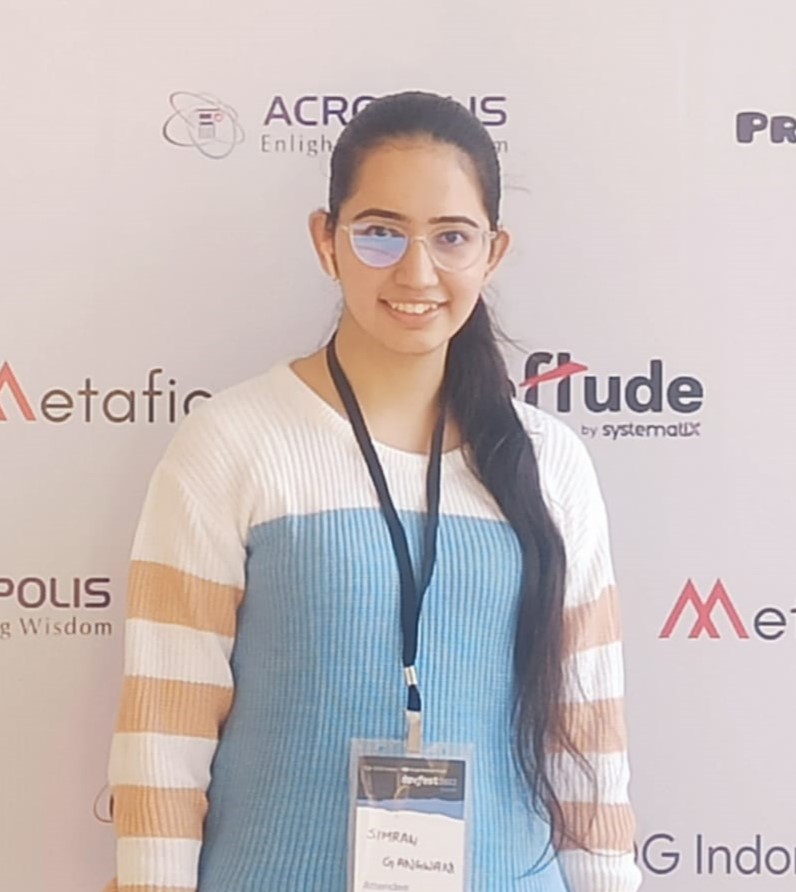
Simran Gangwani
Simran Gangwani
Frontend developer | ReactJs | UI Designer | Graphic Designer | Frontend developer Intern @MekmihansTechnologies | Ex Technical Content Writer @ScalerAcademy & @Codedamn | Web Dev Lead @TedX DAVV | GDSC Campus Lead'23 & Tech Lead'23