Day-22 : Getting Started with Jenkins
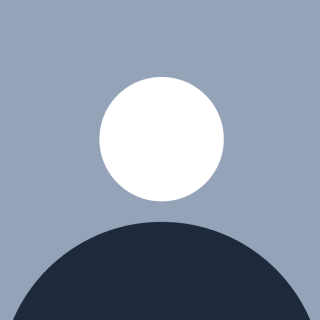
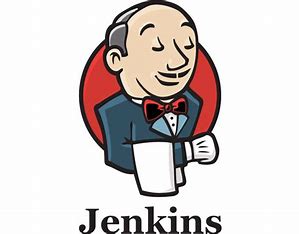
What is Jenkins?
Jenkins is an open-source automation server used to automate parts of software development, including building, testing, and deploying code. It’s commonly used in DevOps to help teams deliver software more quickly and efficiently by implementing Continuous Integration (CI) and Continuous Delivery (CD).
Jenkins is highly extensible and can be integrated with many tools in the software development ecosystem. Through a plugin-based architecture, Jenkins supports different stages of the development lifecycle, such as version control (e.g., Git), build tools (e.g., Maven, Gradle), testing frameworks, deployment tools, and more.
Key Features of Jenkins
Continuous Integration: Jenkins automates the integration of code changes, allowing teams to identify issues early.
Continuous Delivery/Deployment: Jenkins can automate the deployment process, enabling faster releases and consistent environments.
Pipeline Automation: Jenkins supports complex workflows and pipelines with a series of steps, like building, testing, and deployment.
Extensible with Plugins: Jenkins has a vast plugin library that allows you to integrate almost any tool or perform specific tasks within the pipeline.
Distributed Builds: Jenkins can offload tasks to multiple agents (nodes) for better performance, scalability, and security.
How to Use Jenkins
1. Install Jenkins
You can install Jenkins on multiple platforms, including Windows, macOS, and Linux.
For local development, you can also use Docker to run Jenkins in a container.
2. Set Up Jenkins
After installation, access Jenkins through a web browser.
Perform the initial setup, which involves installing recommended plugins and creating an admin user.
3. Create Your First Job
Jenkins organizes work in jobs (also called projects). Jobs define the tasks you want Jenkins to perform, such as building code, running tests, or deploying applications.
To create a job:
Go to Dashboard and click on New Item.
Enter a name, select a job type (typically a Freestyle project for simple tasks or a Pipeline project for advanced workflows), and click OK.
Configure the job settings, including:
Source Code Management (SCM): Link a Git or SVN repository.
Build Triggers: Set up conditions for triggering builds (e.g., on code commits, on schedule).
Build Steps: Define tasks like compiling code, running tests, or packaging the application.
Click Save to save the job configuration.
4. Run and Monitor Jobs
You can start a job manually by clicking Build Now.
View job history and details in the Build History section.
Monitor logs and see the output of each step by clicking on the job and then clicking Console Output for individual builds.
5. Using Pipelines for Complex Workflows
For more advanced automation, Jenkins provides Pipeline projects.
A pipeline is a series of stages and steps, defined in a Jenkinsfile (a file written in Groovy syntax).
Pipelines allow for more complex workflows, such as branching, parallel execution, and more robust error handling.
Example Jenkinsfile:
groovyCopy codepipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
sh 'make build'
}
}
stage('Test') {
steps {
echo 'Testing...'
sh 'make test'
}
}
stage('Deploy') {
steps {
echo 'Deploying...'
sh 'make deploy'
}
}
}
}
6. Set Up Notifications
- Jenkins can send notifications on build success or failure via email, Slack, or other channels. You can configure these notifications as post-build actions in your job or as part of your pipeline.
7. Manage Plugins and Agents
Plugins: Jenkins has thousands of plugins to extend its functionality, which you can install via Manage Jenkins > Manage Plugins.
Distributed Builds: Set up multiple agents (nodes) to distribute the workload and offload tasks from the Jenkins master server. This setup improves performance and scalability.
8. Use Jenkins for Continuous Integration/Continuous Deployment (CI/CD)
For CI/CD, Jenkins can automate the entire software release process.
Set up automated testing, building, and deployment of applications whenever code changes are committed to your repository.
This automation ensures that every change is verified and tested before reaching production, improving software quality and reducing the risk of bugs.
Typical Use Cases for Jenkins
Automated Testing: Run unit tests, integration tests, and end-to-end tests automatically.
Code Quality Analysis: Use plugins for static code analysis and code quality reports.
Building Artifacts: Compile code into deployable artifacts (e.g., JAR files, Docker images).
Deployment: Automate the deployment process to different environments (e.g., development, staging, production).
Scheduled Tasks: Run tasks periodically, such as data backups, security scans, or reporting.
Reporting and Monitoring: Generate reports and send notifications for build status, code quality, and test coverage.
Jenkins plays a crucial role in automating the build, test, and deployment processes, making it an essential tool for implementing Continuous Integration (CI) and Continuous Delivery/Deployment (CD) in modern software development practices. Here’s how Jenkins contributes to each stage and why it's so valuable:
1. Automating the Build Process
In traditional development workflows, building software from source code can be time-consuming and prone to errors when done manually. Jenkins automates this by enabling continuous integration:
Automatic Builds on Code Changes: Jenkins can automatically trigger a build whenever code is committed to a version control repository (like Git, SVN). This allows developers to see immediately if their changes are compatible with the main codebase.
Build Configuration: Jenkins allows you to configure different build steps (e.g., compiling code, packaging binaries) and execute these steps in a consistent, repeatable way. This eliminates human error and ensures the builds are standardized.
Build Artifacts: Jenkins can package built code into artifacts (e.g., JAR files, Docker images) that are ready for testing or deployment. These artifacts are stored for later use, providing a reliable source of application versions.
Parallel Builds and Distributed Builds: For larger projects, Jenkins can distribute builds across multiple agents (machines) to reduce the time taken to complete a build. This ensures that even large codebases are built quickly, maintaining the development velocity.
2. Automating Testing
Automated testing is critical in any CI/CD pipeline to catch issues early and maintain code quality. Jenkins integrates with a variety of testing frameworks and tools to automate different types of testing, such as unit testing, integration testing, and end-to-end testing:
Trigger Tests Automatically: Jenkins can be configured to trigger tests automatically after each build. This ensures that every change is tested without requiring manual intervention.
Testing Stages in Pipelines: Using Jenkins Pipelines, you can create multiple testing stages. For example, you might have an initial unit test stage followed by integration tests and, finally, end-to-end tests. Each stage can pass or fail independently, and you can configure Jenkins to stop the pipeline if any stage fails, saving time and resources.
Parallel Test Execution: Jenkins can run tests in parallel across multiple agents or nodes, significantly speeding up the testing process. This is especially useful for large test suites.
Reports and Feedback: Jenkins provides detailed test reports and logs, allowing developers to quickly diagnose and fix issues. It can also integrate with tools like JUnit, TestNG, and others to provide test summaries, failure reports, and visual feedback, making it easy to track test results over time.
Automated Regression Testing: Jenkins allows you to configure automated regression tests that run every time changes are made to the codebase. This prevents bugs from being reintroduced into previously working code.
3. Automating Deployment
In the context of Continuous Delivery and Continuous Deployment, Jenkins plays a significant role in automating the deployment process, allowing software to be quickly and reliably released to various environments:
Continuous Delivery: Jenkins can automate the deployment process to a staging environment, where the application can be manually tested or reviewed. The final deployment to production is manual, but Jenkins ensures that the application is always ready for deployment.
Continuous Deployment: For teams practicing full automation, Jenkins can deploy to production automatically after passing all tests. This enables quick feedback and shortens the time between development and release, allowing businesses to respond faster to user needs.
Environment-Specific Configurations: Jenkins supports the use of environment variables, parameterized builds, and separate stages for different environments (development, testing, staging, production). This ensures that deployments are correctly configured for each environment.
Rollback Mechanisms: Jenkins can be configured to automatically rollback to the previous stable version if a deployment fails. This is crucial for minimizing downtime and ensuring that faulty code doesn’t affect the end-users.
Deployment to Various Platforms: Jenkins supports deploying to various environments, such as virtual machines, containers (Docker/Kubernetes), cloud platforms (AWS, Azure, GCP), or specific application servers. It integrates with numerous deployment tools, making it highly versatile.
Approval Gates: In larger organizations or for critical releases, Jenkins can be configured to require manual approvals at certain points. For example, Jenkins can stop before deploying to production and wait for a manager’s approval.
Advantages of Jenkins in CI/CD Automation
Speed: Automating build, test, and deployment processes reduces the time developers spend on repetitive tasks, increasing productivity and allowing faster feedback loops.
Reliability: Jenkins ensures that processes are consistent and repeatable, reducing the risk of human error. Automated testing and deployment catch issues early, improving software quality.
Scalability: Jenkins can scale horizontally by adding more agents or nodes, making it possible to handle multiple projects and large codebases efficiently.
Collaboration and Transparency: Jenkins provides visibility into the build, test, and deployment status for the entire team. This transparency encourages collaboration and accountability.
Cost Efficiency: By automating repetitive tasks, Jenkins reduces the manual labor involved, lowering operational costs and allowing resources to focus on more critical tasks.
Example of a Jenkins CI/CD Pipeline
Here’s an example of a Jenkins pipeline that automates build, test, and deployment stages:
groovyCopy codepipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building...'
sh 'mvn clean package'
}
}
stage('Test') {
steps {
echo 'Testing...'
sh 'mvn test'
}
}
stage('Deploy to Staging') {
steps {
echo 'Deploying to staging...'
sh 'scp target/app.jar user@staging-server:/path/to/app'
}
}
stage('Deploy to Production') {
when {
branch 'main'
}
steps {
echo 'Deploying to production...'
sh 'scp target/app.jar user@prod-server:/path/to/app'
}
}
}
}
In this pipeline:
Build Stage: Compiles and packages the code.
Test Stage: Runs automated tests.
Deploy to Staging: Deploys the application to a staging environment.
Deploy to Production: Only deploys to production if the branch is
main
.
Task : Create a Freestyle Pipeline to Print "Hello World"
Create a freestyle pipeline in Jenkins that:
Prints "Hello World"
Prints the current date and time
Clones a GitHub repository and lists its contents
Configure the pipeline to run periodically (e.g., every hour).
Conclusion
Jenkins is a powerful tool for automating the build, test, and deployment stages, enabling teams to implement CI/CD workflows efficiently. By automating these processes, Jenkins helps organizations achieve faster delivery, better quality, and increased reliability, all while reducing manual effort. In today’s fast-paced software development environments, Jenkins is an essential tool for DevOps and CI/CD practices.
Subscribe to my newsletter
Read articles from Shivani Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Shivani Singh
Shivani Singh
"Passionate about simplifying the complexities of DevOps, I bring 5 years of hands-on experience in supporting development and operations teams to achieve faster, more reliable software delivery. I thrive on optimizing CI/CD pipelines, automating workflows, and troubleshooting challenges across diverse cloud environments. My expertise spans across infrastructure management, continuous integration, and performance monitoring—ensuring stability and efficiency at every stage of the software lifecycle. Always eager to learn, collaborate, and innovate, I’m committed to driving impactful change in the DevOps space."