Comprehensive List of String Programming Questions

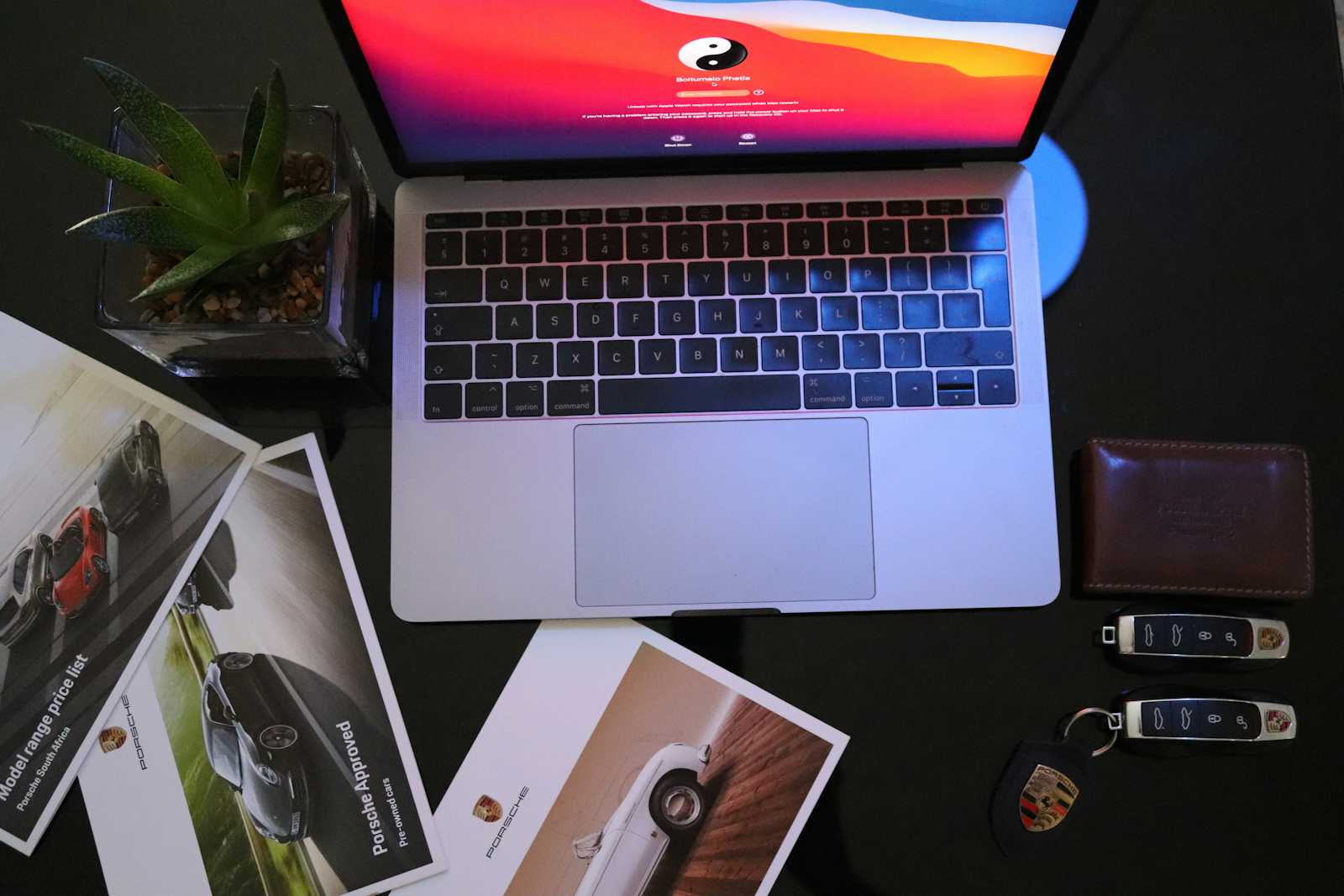
Write a program that checks if a given string is a palindrome. Ignore case and spaces. For example, "A man a plan a canal Panama" should return true
Write a program that finds the most frequently occurring character in a string. Ex: in "programming" frequent char is 'g'
Write method to return a reversed string
Write a program that finds the longest word in a given sentence. For example, in "The quick brown fox jumps over the lazy dog", the longest word is "jumps".
Convert DD/MM/YYYY to DD-MON-YYYY. Ex: 30/09/2010 -> 30-SEP-2010
Write a program that compresses a string by replacing consecutive repeating characters with the character followed by the count. For example, "aaabbcddd" should become "a3b2c1d3". If the "compressed" string is longer than the original, return the original string.
Write a program that takes a compressed string (e.g., "a3b2c1d3") and expands it back to its original form ("aaabbcddd"). Assume the input format is valid.
Write a program that finds the longest common prefix among an array of strings. For example, for ["flower", "flow", "flight"], the longest common prefix is "fl".
Write a program that encrypts and decrypts a string using a Caesar cipher with a given shift. For example, shifting "abc" by 2 results in "cde". Handle both uppercase and lowercase characters.
String programs
Write a program that checks if a given string is a palindrome. Ignore case and spaces. For example, "A man a plan a canal Panama" should return true
package ganesh.nethula.strings;
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (mostFrequentOccurringChar ("A man a plan a canal Panama"));
}
public static boolean isPalindrome (String string) {
// Checking edge cases
if (string == null) {
return false;
}
string = string.trim ().toLowerCase ();
if (string.isEmpty ()){
return false;
}
string = string.replaceAll (" ","");
int start = 0;
int end = string.length () - 1;
while (start < end){
if (string.charAt (start) != string.charAt (end)){
return false;
}
start++;
end--;
}
return true;
}
}
Write a program that finds the most frequently occurring character in a string. Ex: in "programming" frequent char is 'g'
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (mostFrequentOccurringChar ("A man a plan a canal Panama"));
}
// most frequent occurring words in the string
public static char mostFrequentOccurringChar(String string){
if (string == null || string.isEmpty () || string.isBlank ()){
return '\0';
}
if (string.length () == 1){
return string.charAt (0);
}
int maxCharCount = 1;
char maxCountChar = string.charAt (0);
boolean[] visited = new boolean[string.length ()];
for (int i = 0; i < string.length (); i++) {
int charCount = 1;
for (int j = i; j < string.length (); j++) {
if (!visited[j] && string.charAt (i) == string.charAt (j)){
charCount++;
visited[i] = true;
}
if (charCount > maxCharCount){
maxCharCount = charCount;
maxCountChar = string.charAt (i);
}
}
}
return maxCountChar;
}
}
Write method to return a reversed string
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (reverse("code with ganesh"));
}
public static String reverse(String str){
if(str == null || str.length () == 1){
return str;
}
String reverse = "";
for (int i = str.length() - 1; i >= 0; i--) {
reverse += str.charAt (i);
}
return reverse;
}
}
Write a program that finds the longest word in a given sentence. For example, in "The quick brown fox jumps over the lazy dog", the longest word is "jumps".
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (longestWord("he quick brown fox jumps over the lazy dog, the longest word is jumps"));
}
public static String longestWord(String string){
if (string == null) {
throw new NullPointerException ("String is null");
}
if (string.isEmpty () || string.isBlank ()){
return null;
}
string = string.trim ();
String[] words = string.split (" ");
int maxLength = words[0].length ();
String longestWord = words[0];
for (String str : words){
str = str.trim ();
if (str.length () > maxLength){
maxLength = str.length ();
longestWord = str;
}
}
return longestWord;
}
}
Convert DD/MM/YYYY to DD-MON-YYYY. Ex: 30/09/2010 -> 30-SEP-2010
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (formatedDate ("30/09/2010"));
}
public static String formatedDate (String input) {
String []months = {"JAN","FEB","MAR","APR","MAY","JUN","JUL","AUG","SEP","OCT","NOV","DEC"};
String[] dateArray = input.split ( "/" );
String date = dateArray[0];
String mon = months[Integer.parseInt ( dateArray[1] ) - 1];
String year = dateArray[2];
return date + "-" + mon + "-" + year;
}
}
Write a program that compresses a string by replacing consecutive repeating characters with the character followed by the count. For example, "aaabbcddd" should become "a3b2c1d3". If the "compressed" string is longer than the original, return the original string.
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (compressString("aaabbeeeeccddd"));
}
public static String compressString(String string){
if (string == null) {
throw new NullPointerException ("Strings shouldn't be null");
}
if (string.isBlank () || string.isEmpty ()){
return string;
}
String compressedString = "";
for (int i = 0; i < string.length (); i++) {
int count = 1;
char ch = string.charAt (i);
for (int j = i +1 ; j < string.length (); j++) {
if (ch == string.charAt (j)){
count++;
i = j;
}else {
break;
}
}
compressedString += String.valueOf (ch) + count;
}
return compressedString;
}
}
Write a program that takes a compressed string (e.g., "a3b2c1d3") and expands it back to its original form ("aaabbcddd"). Assume the input format is valid.
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (deCompressString("a3b2c1d3"));
}
public static String deCompressString(String string){
if (string == null) {
throw new NullPointerException ("Strings shouldn't be null");
}
if (string.isBlank () || string.isEmpty ()){
return string;
}
String decompressedString = "";
for (int i = 0; i < string.length (); i++) {
char ch = string.charAt (i);
int count = string.charAt (++i) - '0';
for (int j = 0; j < count; j++) {
decompressedString += String.valueOf (ch);
}
}
return decompressedString;
}
}
Write a program that finds the longest common prefix among an array of strings. For example, for ["flower", "flow", "flight"], the longest common prefix is "fl".
public class StringProgramsExample {
public static void main (String[] args) {
String[] strings = {"flower", "flow", "flight"};
System.out.println (longestCommonPrefix(strings));
}
public static String longestCommonPrefix(String[] strings){
if (strings == null){
throw new NullPointerException ("String shouldn't be null");
}
String longestPrefix = strings[0];
for (int i = 0; i < strings.length; i++) {
while (strings[i].indexOf (longestPrefix) != 0){
longestPrefix = longestPrefix.substring (0,longestPrefix.length ()-1);
}
if (longestPrefix.isEmpty ()){
return "";
}
}
return longestPrefix;
}
}
Write a program that encrypts and decrypts a string using a Caesar cipher with a given shift. For example, shifting "abc" by 2 results in "cde". Handle both uppercase and lowercase characters.
public class StringProgramsExample {
public static void main (String[] args) {
System.out.println (encryptOrDecrypt("abc",2));
}
public static String encryptOrDecrypt(String string,int shifts){
if (string == null) {
throw new NullPointerException ("String is null");
}
if (string.isEmpty () || string.isBlank ()){
return string;
}
String rotateString = "";
shifts = shifts % 26;
for (int i = 0; i < string.length (); i++) {
if(string.charAt (i) >='A' && string.charAt (i) <= 'Z'){
rotateString += (char)((string.charAt (i) - 'A' + shifts)%26 + 'A');
}else if (string.charAt (i) >='a' && string.charAt (i) <= 'z'){
rotateString += (char)((string.charAt (i) - 'a' + shifts)%26 + 'a');
}else {
rotateString += string.charAt (i);
}
}
return rotateString;
}
}
Subscribe to my newsletter
Read articles from Ganesh Nethula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
