Java Architect Scenario-Based Interview Questions and Answers.
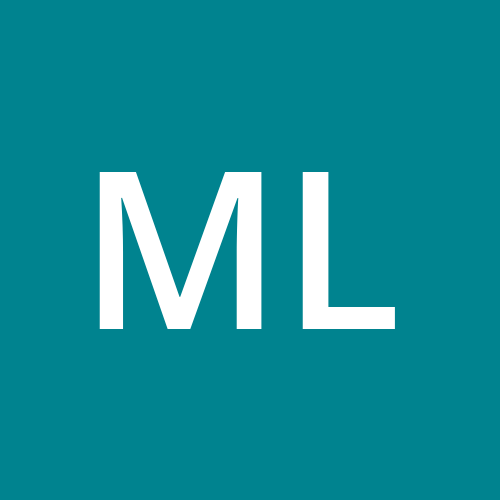
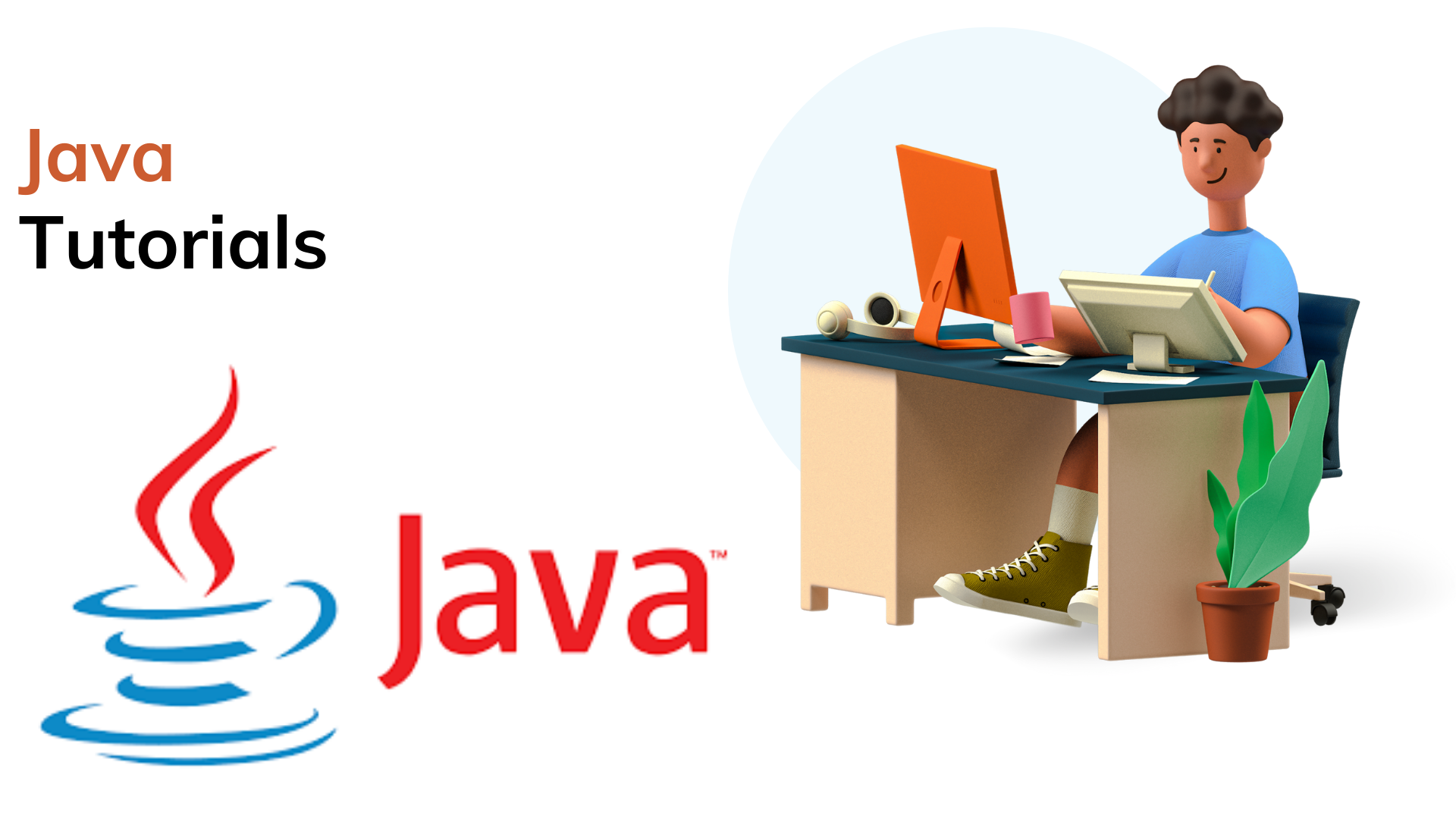
As a Java architect, you are expected to have deep knowledge of system design, architecture patterns, performance optimization, security, and integration. To help you prepare for a Java architect interview, here are some scenario-based questions along with detailed answers that test various skills such as design thinking, problem-solving, and the ability to handle complex software architecture situations.
1. Scenario: Designing a Highly Scalable Microservices Architecture
Question:
You have been asked to design a microservices-based architecture for an e-commerce application. The application will have several modules like user management, product catalog, order processing, payment service, and inventory management. Each module will be developed and deployed independently. How would you approach designing this system to ensure high scalability, reliability, and maintainability?
Answer:
To design a scalable and reliable microservices-based system for e-commerce, I would follow these steps:
Define the Service Boundaries:
Each service should be responsible for a single business function (e.g., user management, order processing, etc.). This ensures loose coupling and high cohesion within services.
Use Domain-Driven Design (DDD) principles to model bounded contexts for each microservice.
Communication Between Services:
Prefer asynchronous communication (e.g., Kafka, RabbitMQ) for scalability and reliability, particularly for actions like order placement or payment processing.
For synchronous communication, RESTful APIs or gRPC can be used.
API Gateway:
- Use an API Gateway (e.g., Kong, Zuul, Spring Cloud Gateway) to manage service routing, load balancing, and authentication.
Service Discovery:
- Implement service discovery (e.g., Netflix Eureka, Consul) to manage dynamically registered services in a scalable environment.
Data Management:
Adopt the Database per Service pattern where each service owns its data and has its database (e.g., MySQL for the user service, MongoDB for the product catalog).
Use Event Sourcing and CQRS (Command Query Responsibility Segregation) patterns to handle complex transactional consistency and data retrieval.
Resilience and Fault Tolerance:
Implement circuit breakers (e.g., Hystrix, Resilience4j) to prevent cascading failures.
Use retries and fallbacks in case of temporary failures.
Scalability and Load Balancing:
Use containerization (e.g., Docker) and orchestration platforms (e.g., Kubernetes) to scale services horizontally.
Configure horizontal scaling for services based on load.
Monitoring and Logging:
Implement centralized logging using tools like ELK Stack (Elasticsearch, Logstash, Kibana) or EFK Stack (Fluentd).
Use Prometheus and Grafana for system monitoring and alerting.
Security:
Use OAuth2 or JWT tokens for secure authentication and authorization.
Protect APIs and services using SSL/TLS encryption and implement API rate limiting.
2. Scenario: Legacy System Integration
Question:
Your company has a legacy monolithic application, and you need to integrate it with a new microservices-based architecture. The legacy application is critical, and you cannot completely rewrite it immediately. How would you approach integrating the legacy system with the new microservices?
Answer:
Integrating a legacy monolithic system with a new microservices architecture requires careful planning. Here’s how I would approach it:
Assess the Legacy System:
Understand the key components of the legacy system that need to be integrated with the new system.
Identify the most critical data flows and interactions that need to be maintained during the transition.
Strangler Pattern:
- Use the Strangler Pattern to gradually replace parts of the legacy system with microservices. This allows the old system to remain functional while the new system is being built incrementally.
Expose Legacy System Data via APIs:
Create APIs or use an existing API layer to expose critical functionalities of the legacy system (e.g., via REST or SOAP).
Use an API Gateway to manage routing between legacy services and new microservices.
Adapter Layer:
- Implement an adapter layer between the legacy system and the new microservices to handle communication. This adapter will translate data and protocols, allowing seamless interaction.
Data Synchronization:
- Use an event-driven approach for data synchronization. For instance, use an enterprise service bus (ESB) or message brokers (e.g., Kafka) to sync data between the legacy system and the new microservices.
Incremental Migration:
- Gradually migrate functionalities from the monolithic system to microservices. Start with less critical services and move toward more critical ones once the microservices architecture stabilizes.
Monitoring and Logging:
- Implement centralized logging and monitoring for both the legacy system and the new microservices to detect and diagnose issues early.
Security Considerations:
- Ensure that security is consistently applied across the integration layer. If the legacy system uses older authentication methods, consider implementing a security gateway or service mesh to standardize authentication for both systems.
3. Scenario: High Traffic Handling
Question:
Your Java-based system is expected to handle a high volume of traffic, with thousands of requests per second. How would you design the system to ensure high availability, low latency, and efficient resource utilization?
Answer:
To handle high traffic efficiently, I would consider the following strategies:
Load Balancing:
Use a load balancer (e.g., Nginx, HAProxy, or cloud-based load balancers) to distribute traffic across multiple instances of your application.
Implement sticky sessions if necessary to maintain session consistency.
Horizontal Scaling:
Use horizontal scaling with containerization (e.g., Docker) and Kubernetes to manage microservices and scale dynamically based on traffic loads.
Use auto-scaling groups in cloud environments (e.g., AWS, GCP) to scale resources as traffic increases.
Caching:
Implement caching mechanisms to reduce the load on your databases and application servers. Use distributed caching solutions like Redis or Memcached for frequently accessed data.
Consider CDN (Content Delivery Network) caching for static content.
Database Optimization:
Optimize database queries by indexing frequently queried columns and using proper normalization techniques.
Use database replication and sharding to distribute database loads and prevent bottlenecks.
Asynchronous Processing:
Offload time-consuming tasks (e.g., email sending, report generation) to background jobs or message queues (e.g., Kafka, RabbitMQ).
Use worker pools to manage background task processing and ensure responsiveness.
Microservices Design:
Design microservices to be stateless, enabling easy horizontal scaling without session-related issues.
Use event-driven architectures where possible, allowing decoupled services to communicate asynchronously.
Optimizing JVM Performance:
Tune JVM parameters (e.g., heap size, garbage collection settings) to optimize memory usage and minimize latency.
Use tools like JVisualVM, JProfiler, or Prometheus to monitor JVM performance.
Rate Limiting:
- Implement rate limiting and throttling to protect the backend from DDoS attacks and to ensure fair usage of resources.
Monitoring and Alerts:
Set up comprehensive monitoring for application performance, server health, and traffic patterns using tools like Prometheus, Grafana, or New Relic.
Implement automated alerts for anomalous behaviors such as high latency or resource exhaustion.
4. Scenario: Fault Tolerance in Distributed Systems
Question:
Your application is built using multiple distributed services, and you need to ensure that the system remains functional even if one or more services fail. How would you implement fault tolerance in this system?
Answer:
To implement fault tolerance in a distributed system, I would consider the following strategies:
Circuit Breaker Pattern:
- Implement the Circuit Breaker pattern using libraries like Hystrix or Resilience4j. This ensures that if a service fails, subsequent calls to that service are short-circuited to prevent cascading failures.
Retries with Backoff:
- Use retry mechanisms with exponential backoff for transient failures. This ensures that temporary network issues or service unavailability don’t cause permanent failures.
Graceful Degradation:
- Design services to gracefully degrade under heavy load or failure conditions. For instance, when a critical service is unavailable, the system can fallback to providing limited functionality or return cached data.
Failover Mechanisms:
Implement failover mechanisms by having redundant instances of critical services running in different availability zones or regions.
Use tools like Kubernetes for self-healing, which will automatically restart failed pods.
Event Sourcing and CQRS:
- Use Event Sourcing to ensure that the system state is always consistent and can be rebuilt in case of failure. CQRS can help separate read and write concerns, optimizing for failures in specific parts of the system.
Distributed Transactions:
- Use eventual consistency for distributed transactions to prevent blocking. Implement patterns like Saga or Compensation for handling distributed transactions across services.
Monitoring and Alerting:
- Implement robust monitoring and alerting to quickly detect failures and performance bottlenecks. Tools like Prometheus, Grafana, and ELK Stack can help with this.
Service Replication:
- Use replication for stateful services to ensure that data is not lost in case of failures. Databases like Cassandra or services like Redis support replication for fault tolerance.
Conclusion
These scenario-based interview questions for a Java architect focus on the ability to handle complex real-world situations involving system design, integration, scalability, performance, and fault tolerance. As a Java architect, it's crucial to balance trade-offs between different approaches, understand various architectural patterns, and make decisions that align with both business goals and technical requirements. These answers provide a foundation for tackling challenges in high-traffic, distributed, and evolving systems, preparing you to excel in an interview for a Java architect role.
More such articles:
https://www.youtube.com/@maheshwarligade
Subscribe to my newsletter
Read articles from Maheshwar Ligade directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
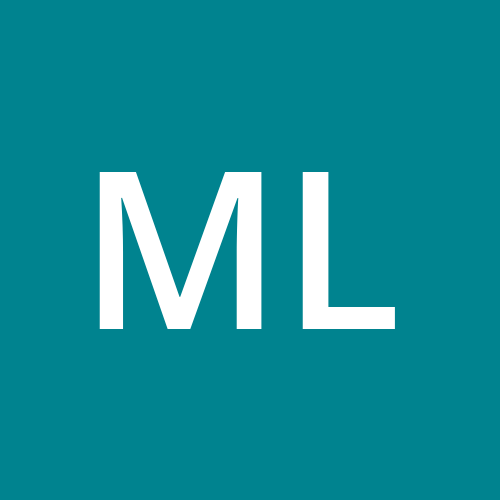
Maheshwar Ligade
Maheshwar Ligade
Learner, Love to make things simple, Full Stack Developer, StackOverflower, Passionate about using machine learning, deep learning and AI