JSON Journeys: Navigating Data Formats!
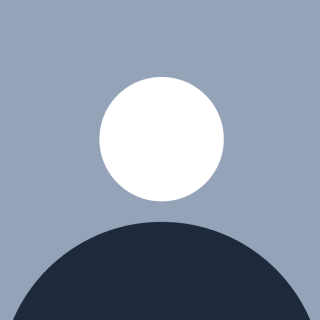
Table of contents
- What is JSON?
- Why is JSON Important?
- JSON Structure — The Map of Data
- Working with JSON Data
- Parsing and Stringifying JSON
- Navigating JSON Data
- Practical Applications of JSON
- Building a Simple JSON-Based Application
- Challenge — Create a Simple JSON-Based Application
- Mastering Data Formats with JSON
- Let’s dive into The Call Stack Chronicles!
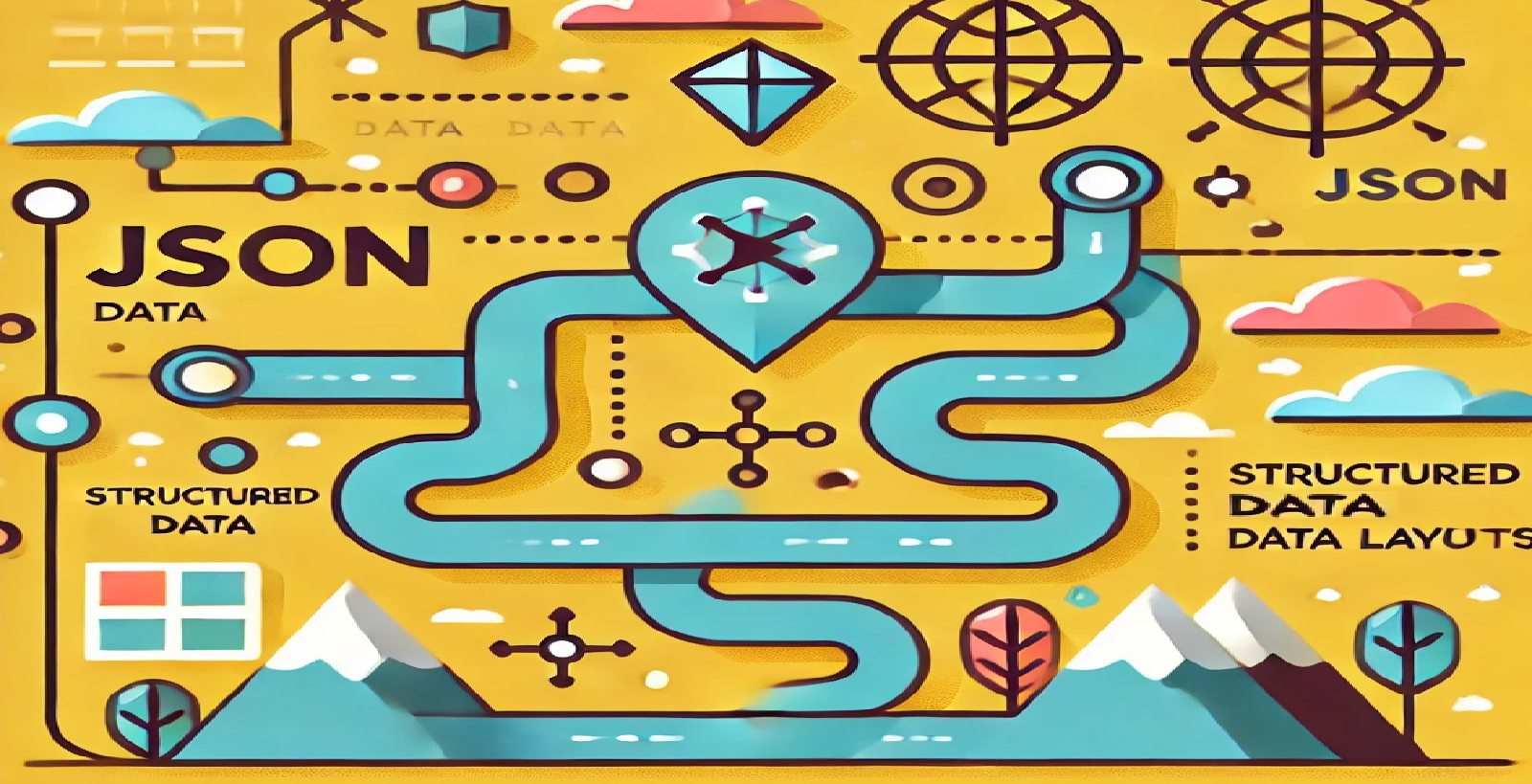
Imagine you're an explorer setting off on a journey to discover a hidden treasure of information. Along the way, you encounter maps and instructions that help you find, understand, and organize this treasure. In the world of web development, JSON (JavaScript Object Notation) is like that treasure map—it's one of the most popular formats for storing and exchanging data, especially between web servers and browsers.
In this article, we’ll guide you through what JSON is, how to work with JSON data, and how to use JavaScript methods to navigate through and manipulate that data. Our theme is to think of yourself as a data explorer, learning how to read, extract, and store valuable information along the way.
What is JSON?
Let’s start at the beginning of our journey by answering a simple question: What is JSON?
JSON (JavaScript Object Notation) is a lightweight, easy-to-read format for structuring data. While the name has JavaScript in it, JSON is a universal data format that can be used in almost any programming language. It’s like a universal map that any explorer can use, regardless of where they’re from.
Simple Example of JSON
Here’s a small example of JSON data that represents a user profile:
code{
"name": "Alice",
"age": 25,
"email": "alice@example.com",
"isMember": true
}
Let’s break this down:
The structure consists of key-value pairs. Each key (like
"name"
,"age"
,"email"
) points to a value (like"Alice"
,25
, ortrue
).The keys are always strings, but the values can be a mix of strings (
"Alice"
), numbers (25
), booleans (true
), or even arrays and objects.
Think of this as a little information card about a user that can be sent across different systems, making it easy to read and use.
Why is JSON Important?
You might be wondering: Why do I need to know JSON?
Well, JSON is widely used to send and receive data between servers and web browsers. For example, if you’re browsing an online store and the page shows a list of products, there’s a good chance the product data (like names, prices, and descriptions) was sent from the server to your browser in JSON format.
As a developer, you’ll often deal with JSON when:
Fetching data from an API.
Sending data to a server.
Storing structured data in files or databases.
JSON Structure — The Map of Data
Now that you know what JSON is, let’s go deeper into how JSON is structured. Think of JSON as a map that helps you understand where each piece of information is stored.
JSON organizes data in two main ways:
Objects: Collections of key-value pairs (like the user profile example).
Arrays: Lists of values, which can include numbers, strings, or even other objects.
Flowchart: Visualizing JSON Structure
To help you visualize how JSON works, let’s look at a flowchart representing a more complex JSON object:
code{
"user": {
"name": "Alice",
"age": 25,
"preferences": {
"newsletter": true,
"theme": "dark"
}
}
}
Here’s how it looks broken down:
code[ Object ] -------> "user"
|
[ Object ] (user details)
|------> "name": "Alice"
|------> "age": 25
|------> [ Object ] "preferences"
|----> "newsletter": true
|----> "theme": "dark"
This flowchart shows how JSON can nest objects inside other objects. It’s like an explorer’s map, where you follow a path (keys) to find treasures (values).
Working with JSON Data
As a developer (or an explorer), the next part of your journey is working with JSON data. In JavaScript, JSON is widely used to send and receive data between a web server and a browser.
Where Do We Encounter JSON?
Here’s where you’re likely to run into JSON:
APIs: APIs (Application Programming Interfaces) are like ports where explorers can receive or send data from one system to another. The data they send and receive is often in JSON format.
Configuration Files: JSON is also used to store settings or configurations in files.
LocalStorage: You might store JSON in your browser’s storage to keep track of user preferences or session data.
Code Snippet: Fetching JSON Data from an API
Imagine you’re on a mission to get data from a remote location (an API). JavaScript provides a way to fetch data from APIs, and often, the data returned is in JSON format. Here’s how it’s done:
codefetch("https://api.example.com/data") // Step 1: Make a request to the API
.then(response => response.json()) // Step 2: Convert the response into JSON
.then(data => {
console.log(data); // Step 3: Log the data (the JSON) to the console
})
.catch(error => console.log("Error fetching data: ", error));
Breaking it down:
fetch()
: This is like sending out a request to get data from an API. In this case, the API at"
https://api.example.com/data
"
..then(response => response.json())
: Once the API responds, this step converts the raw data into JSON format so that you can work with it..then(data => { ... })
: Once you have the JSON data, you can do something with it—like display it on your webpage or log it to the console..catch(error)
: If something goes wrong (like a failed network request), thecatch
block will handle the error.
Think of fetching data like an explorer communicating with a distant land, receiving information from far away to use in their journey.
Parsing and Stringifying JSON
As you explore JSON data, you’ll encounter two key tasks:
Parsing JSON: When you receive JSON data as a string, you need to convert it into a JavaScript object that you can work with.
Stringifying JSON: When you need to send data to a server or store it in a file, you need to convert a JavaScript object back into a JSON string.
Code Snippet: JSON Methods (Parsing and Stringifying)
Example 1: Parsing JSON Data
Imagine you receive data from a server, but it’s in the form of a string. To work with this data in JavaScript, you’ll use JSON.parse()
.
let jsonString = '{"name": "Alice", "age": 25, "isMember": true}';
let user = JSON.parse(jsonString);
console.log(user.name); // Output: Alice
Explanation:
JSON.parse()
takes the string form of JSON and converts it into a JavaScript object.Now, you can access properties like
user.name
just like you would with any regular JavaScript object.
Example 2: Stringifying JavaScript Data
Let’s say you have a JavaScript object and want to send it to a server or store it. You’ll need to convert it to a JSON string using JSON.stringify()
.
let user = {
name: "Alice",
age: 25,
isMember: true
};
let jsonString = JSON.stringify(user);
console.log(jsonString); // Output: {"name":"Alice","age":25,"isMember":true}
Explanation:
JSON.stringify()
converts a JavaScript object into a JSON string. This is the format you would use to send data to a server.
Navigating JSON Data
In this first part of our JSON journey, we explored what JSON is, its structure, and how you can fetch, parse, and stringify JSON data in JavaScript. With this foundational knowledge, you’re now ready to navigate JSON data like a skilled explorer.
In the next part, we’ll dive deeper into practical applications of JSON, including building a simple JSON-based application to reinforce what you’ve learned.
Practical Applications of JSON
Previously, we laid the groundwork for understanding JSON and how to work with it in JavaScript. Now, let’s explore where JSON is used in real-world applications.
As a data explorer, your job doesn’t end with just reading maps—you also have to make decisions and interact with the world around you. JSON allows you to do just that in the digital world, providing a bridge between your application and external data sources, such as servers, APIs, and databases.
Here are some common uses of JSON:
APIs: JSON is the most popular format for transferring data between clients (like browsers) and servers. APIs return data in JSON format that can be easily processed by JavaScript.
Configuration Files: Many web applications use JSON for configuration settings. Instead of hardcoding values, JSON files store information that the application can read and modify as needed.
Data Storage: JSON is often used to store structured data in databases or even in local storage within the browser.
Let’s take a deeper look at each use case, starting with APIs, where JSON is often the star player.
Building a Simple JSON-Based Application
Now that you’ve become comfortable with the structure and methods of JSON, let’s build a simple application that uses JSON to fetch, display, and manipulate data.
Example: Creating a Simple To-Do List App Using JSON
We’ll build a To-Do List app that allows users to add tasks, store them as JSON, and retrieve them. This example will help reinforce your understanding of how to fetch and manipulate JSON data.
Here’s how we’ll approach the project:
Fetch existing tasks from a mock API (we'll simulate this with hardcoded JSON data).
Add new tasks and update the task list in real-time.
Store the tasks as a JSON string and display them in the browser.
Step 1: Simulating a JSON API for To-Do Tasks
To simulate fetching tasks from an API, we’ll start with some hardcoded JSON data. Normally, you’d fetch this from a real API, but for this example, we’ll simulate the process.
code// Simulating fetching tasks from an API
let taskData = `[
{"task": "Buy groceries", "completed": false},
{"task": "Read a book", "completed": true}
]`;
function fetchTasks() {
return JSON.parse(taskData);
}
let tasks = fetchTasks();
console.log(tasks);
Explanation:
We simulate an API call by hardcoding the JSON response inside a string (
taskData
).JSON.parse()
is used to convert the JSON string into a JavaScript array of objects, allowing us to work with it in the app.
Step 2: Displaying Tasks in the Browser
Once we have our JSON data, we’ll display the tasks in a simple list in the browser. Each task will be rendered as a list item, and we’ll display whether the task is complete or not.
<ul id="taskList"></ul>
<script>
function displayTasks(tasks) {
let taskList = document.getElementById("taskList");
taskList.innerHTML = ""; // Clear the current list
tasks.forEach(task => {
let listItem = document.createElement("li");
listItem.textContent = `${task.task} - ${task.completed ? "Completed" : "Incomplete"}`;
taskList.appendChild(listItem);
});
}
displayTasks(tasks);
</script>
Explanation:
We dynamically create list items (
<li>
) for each task and append them to the<ul>
element (taskList
).Each list item shows the task name and whether it is completed.
Step 3: Adding New Tasks
Next, we’ll add functionality to allow users to input new tasks and update the task list.
<input type="text" id="newTaskInput" placeholder="Enter new task">
<button onclick="addTask()">Add Task</button>
<script>
function addTask() {
let newTaskInput = document.getElementById("newTaskInput").value;
let newTask = {
task: newTaskInput,
completed: false
};
tasks.push(newTask); // Add new task to the task array
displayTasks(tasks); // Refresh the task list
document.getElementById("newTaskInput").value = ""; // Clear input field
}
</script>
Explanation:
The
addTask()
function reads the user input, creates a new task object, adds it to the tasks array, and then redisplays the updated task list.The input field is cleared after adding the new task.
Step 4: Storing the Task List as JSON
Finally, we’ll convert the tasks array into a JSON string and display it on the page. This simulates saving the task list in JSON format for later use (e.g., saving to local storage or sending it to a server).
<button onclick="saveTasks()">Save Tasks as JSON</button>
<pre id="jsonOutput"></pre>
<script>
function saveTasks() {
let jsonOutput = JSON.stringify(tasks, null, 2); // Convert to JSON
document.getElementById("jsonOutput").textContent = jsonOutput;
}
</script>
Explanation:
JSON.stringify()
is used to convert the tasks array into a JSON string.The output is displayed in a
<pre>
tag, which preserves the formatting of the JSON data.
Now, you’ve built a simple To-Do List app that uses JSON to fetch, display, and store tasks. You can extend this app by adding functionality to edit or delete tasks, mark them as completed, and save them in localStorage or send them to a real API.
Challenge — Create a Simple JSON-Based Application
Now that we’ve built a basic JSON-powered app, here’s a challenge to test your skills:
Challenge Instructions:
Create a simple app that stores user preferences (such as theme or language) using JSON.
Allow users to select their preferences and save them as a JSON object.
Retrieve and display the saved preferences whenever the user revisits the page.
Here’s a starter idea:
Create a form that allows users to select a theme (e.g., dark or light) and a preferred language (e.g., English, Spanish).
When the user submits their preferences, save them as a JSON object and display a confirmation message.
When the page reloads, load the preferences from localStorage and apply them to the page.
Mastering Data Formats with JSON
As we conclude our journey, let’s reflect on what we’ve learned about JSON:
JSON is the language of data exchange: Whether you're fetching data from an API or storing settings in a file, JSON is the most common format for transferring structured data.
JavaScript provides powerful tools for working with JSON: Methods like
JSON.parse()
andJSON.stringify()
make it easy to convert between JSON strings and JavaScript objects.Practical applications of JSON: From APIs to configuration files, JSON is used everywhere in web development. Being comfortable with JSON is an essential skill for modern developers.
Let’s dive into The Call Stack Chronicles!
In the next article, we’ll dive into the inner workings of JavaScript by exploring the call stack—a key concept that explains how JavaScript executes functions and handles memory. Get ready for The Call Stack Chronicles, where we’ll uncover how your code runs behind the scenes!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by