Pattern Program Questions in Java
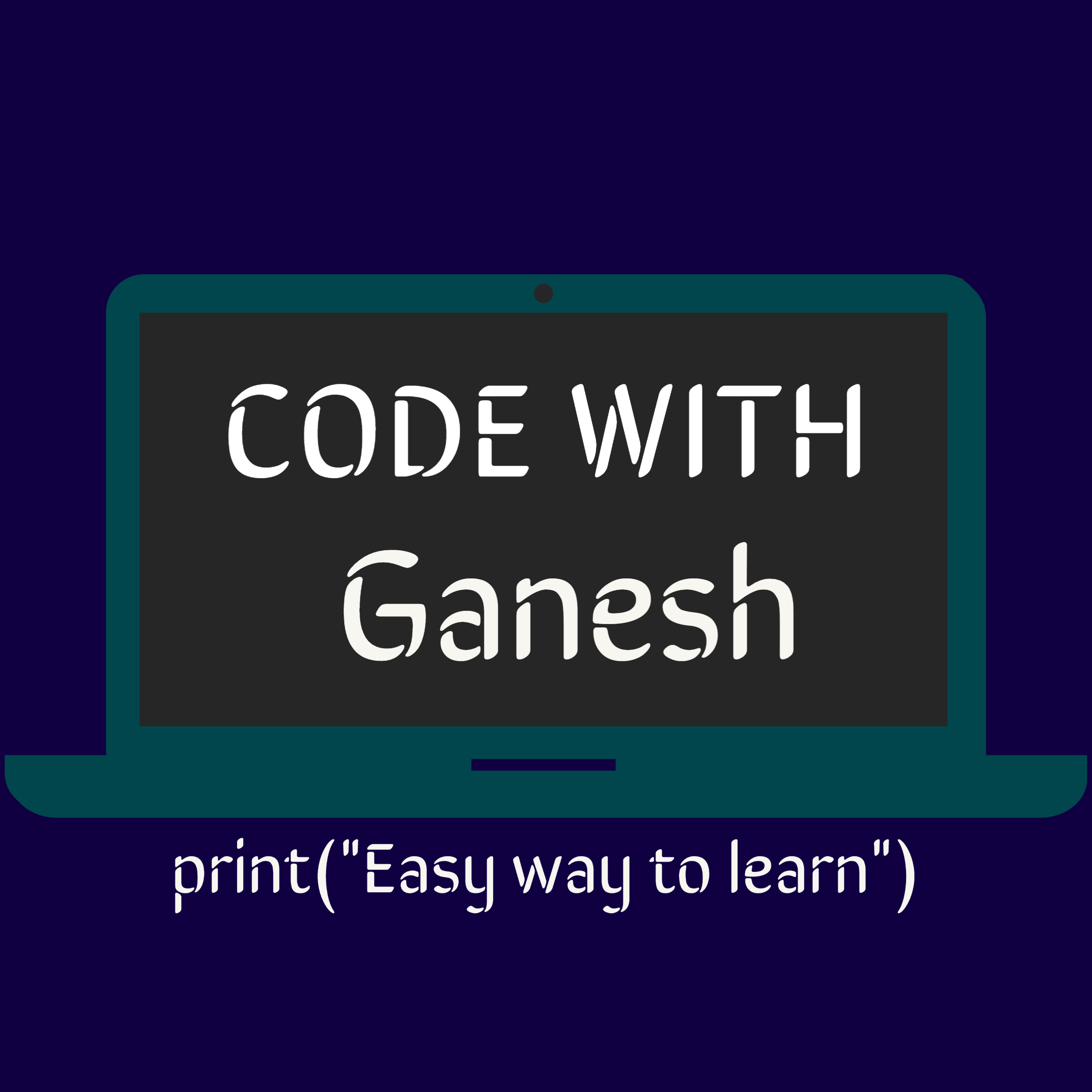
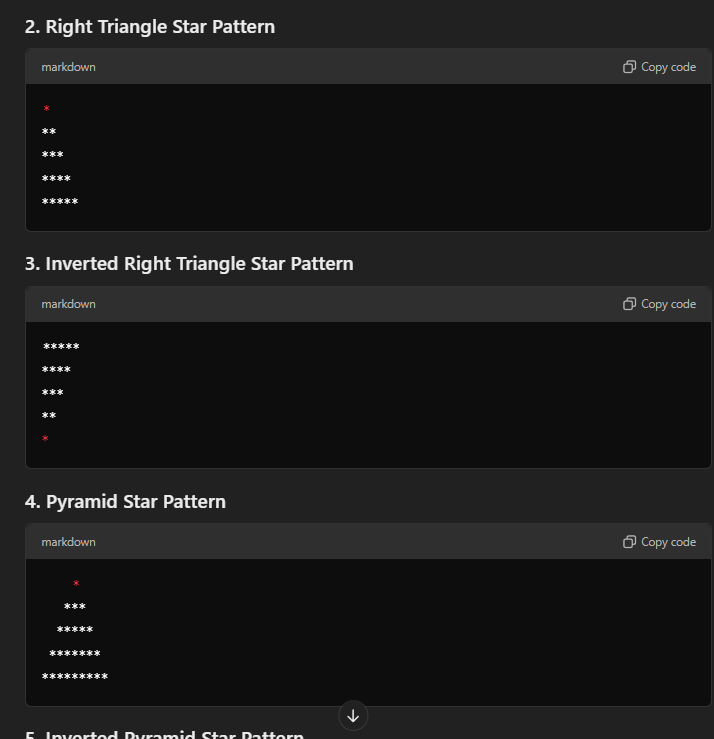
Pattern Program Problems in Java
Square Star Pattern
Problem Statement: Write a Java program to print a square star pattern of size
n
.Example (for ( n = 5 )):
***** ***** ***** ***** *****
Right Triangle Star Pattern
Problem Statement: Write a Java program to print a right triangle star pattern of height
n
.Example (for ( n = 5 )):
* ** *** **** *****
Inverted Right Triangle Star Pattern
Problem Statement: Write a Java program to print an inverted right triangle star pattern of height
n
.Example (for ( n = 5 )):
***** **** *** ** *
Pyramid Star Pattern
Problem Statement: Write a Java program to print a centered pyramid pattern of height
n
.Example (for ( n = 5 )):
* *** ***** ******* *********
Hint: Use spaces for alignment.
Inverted Pyramid Star Pattern
Problem Statement: Write a Java program to print an inverted centered pyramid pattern of height
n
.Example (for ( n = 5 )):
********* ******* ***** *** *
Hollow Pyramid Star Pattern
Problem Statement: Write a Java program to print a hollow pyramid of height
n
.Example (for ( n = 5 )):
* * * * * * * *********
Diamond Star Pattern
Problem Statement: Write a Java program to print a diamond star pattern of height
n
. The diamond should have2*n - 1
rows in total.Example (for ( n = 5 )):
* *** ***** ******* ********* ******* ***** *** *
Hollow Diamond Star Pattern
Problem Statement: Write a Java program to print a hollow diamond star pattern of height
n
.Example (for ( n = 5 )):
* * * * * * * * * * * * * * * *
Right Triangle Number Pattern
Problem Statement: Write a Java program to print a right triangle number pattern up to
n
rows.Example (for ( n = 5 )):
1 12 123 1234 12345
Pyramid Number Pattern
Problem Statement: Write a Java program to print a centered pyramid pattern with numbers. Each row should display numbers in increasing order starting from
1
.Example (for ( n = 5 )):
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5
Pascal's Triangle
Problem Statement: Write a Java program to print Pascal's Triangle of height
n
.Example (for ( n = 5 )):
1 1 1 1 2 1 1 3 3 1 1 4 6 4 1
Hint: Use the combination formula ( C(n, k) = \frac{n!}{k!(n-k)!} ).
Floyd’s Triangle
Problem Statement: Write a Java program to print Floyd's Triangle of height
n
.Example (for ( n = 5 )):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
Square Number Pattern
Problem Statement: Write a Java program to print a square number pattern with numbers from
1
ton
. Each row should start from the first number of the sequence.Example (for ( n = 4 )):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
Hollow Square Pattern with Border
Problem Statement: Write a Java program to print a hollow square pattern of size
n
, with a border of stars.Example (for ( n = 5 )):
***** * * * * * * *****
Zigzag Number Pattern
Problem Statement: Write a Java program to print a zigzag number pattern of height
n
, with numbers increasing row by row.Example (for ( n = 5 )):
1 2 3 6 5 4 7 8 9 10 15 14 13 12 11
Each problem encourages practicing loops, conditionals, and alignment using spaces in Java.
Solution
public class patterns {
public static void main (String[] args) {
System.out.println ("\nProblem Statement: Write a Java program to print a square star pattern of size n.\n");
squareStarPattern(5);
System.out.println ("\nProblem Statement: Write a Java program to print a right triangle star pattern of height n.\n");
rightTriangleStar (5);
System.out.println ("\nProblem Statement: Write a Java program to print a Inverted right triangle star pattern of height n.\n");
invertedRightTriangleStarPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a Pyramid Star Pattern star pattern of height n.\n");
pyramidStarPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a Inverted Pyramid Star Pattern star pattern of height n.\n");
InvertedPyramidStarPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a Diamond Star Pattern star pattern of height n.\n");
diamondStarPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a hollow Pyramid pattern of height n.\n");
hollowPyramid (5);
System.out.println ("\nProblem Statement: Write a Java program to print a hollow diamond Pyramid pattern of height n.\n");
hollowDiamondPyramid (5);
System.out.println ("\nProblem Statement: Write a Java program to print a right triangle number pattern up to n rows.\n");
rightTriangleNumberPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print Pyramid Number Triangle of height n.\n");
pyramidNumberPattern1 (5);
System.out.println ("\nProblem Statement: Write a Java program to print Pascal's Triangle Triangle of height n.\n");
PascalsTriangle (5);
System.out.println ("\nProblem Statement: Write a Java program to print Floyd's Triangle of height n.\n");
floydsTriangle (5);
System.out.println ("\nProblem Statement: Write a Java program to print a square number pattern with numbers from 1 to n. Each row should start from the first number of the sequence.\n");
squareNumberPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a hollow square pattern of size n, with a border of stars.\n");
hollowSquarePattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print a zigzag number pattern of height n, with numbers increasing row by row.n");
zigzagNumberPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print k Triangle of height n.\n");
kPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print right Pascals Triangle of height n.\n");
rightPascalsTriangle (5);
System.out.println ("\nProblem Statement: Write a Java program to print right Butterfly Star Pattern of height n.\n");
butterflyStarPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print right Stop watch Star Pattern of height n.\n");
stopWatchPattern (5);
System.out.println ("\nProblem Statement: Write a Java program to print right Stop watch Hollow Star Pattern of height n.\n");
stopWatchHollowPattern (5);
}
/*
Problem Statement: Write a Java program to print a square star pattern of size n.
Example (for ( n = 5 )):
*****
*****
*****
*****
*****
* */
public static void squareStarPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j <n; j++) {
System.out.print("* ");
}
System.out.println ();
}
}
/*
Right Triangle Star Pattern
Problem Statement: Write a Java program to print a right triangle star pattern of height n.
Example (for ( n = 5 )):
*
**
***
****
*****
*/
public static void rightTriangleStar(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j <=i; j++) {
System.out.print("* ");
}
System.out.println ();
}
}
public static void invertedRightTriangleStarPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
System.out.print("* ");
}
System.out.println ();
}
}
/*
*
***
*****
*******
*********
*/
public static void pyramidStarPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
System.out.print(" ");
}
for (int j = 0; j < (2*i)+1; j++) {
System.out.print("*");
}
System.out.println ();
}
}
public static void InvertedPyramidStarPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
for (int j = 0; j < (2*(n-i))-1; j++) {
System.out.print("*");
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a diamond star pattern of height n
*
***
*****
*******
*********
*******
*****
***
*
* */
public static void diamondStarPattern2(int n){
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
System.out.print(" ");
}
for (int j = 0; j < (2*i)+1; j++) {
System.out.print("*");
}
System.out.println ();
}
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < i+2; j++) {
System.out.print(" ");
}
for (int j = 0; j < (2*(n-i-1))-1; j++) {
System.out.print("*");
}
System.out.println ();
}
}
public static void diamondStarPattern3(int n){
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
System.out.print(" ");
}
for (int j = 0; j < (2*i)+1; j++) {
System.out.print("*");
}
System.out.println ();
}
for (int i = n - 2 ; i >= 0; i++) {
for (int j = 0; j < n - i -1; j++) {
System.out.println (" ");
}
for (int j = 0; j < 2*i + 1; j--) {
System.out.println ("*");
}
}
}
public static void diamondStarPattern(int n){
for (int i = 0; i < n; i++) {
System.out.print (" ".repeat (n-i-1));
System.out.print ("*".repeat (2*i+1));
System.out.println ();
}
for (int i = n - 2 ; i >= 0; i--) {
System.out.print (" ".repeat (n-i-1));
System.out.print ("*".repeat (2*i+1));
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a hollow pyramid of height n.
*
* *
* *
* *
*********
*/
public static void hollowPyramid(int n){
for (int i = 0; i < n; i++) {
for (int j = n - i; j >= 0; j--) {
System.out.print (" ");
}
for (int j = 0; j < 2*i+1; j++) {
if((j == 0 || j == 2*i || i == n-1)){
System.out.print ("*");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a hollow diamond star pattern of height n.
*
* *
* *
* *
* *
* *
* *
* *
*
*/
public static void hollowDiamondPyramid(int n){
for (int i = 0; i < n; i++) {
for (int j = n - i-2; j >= 0; j--) {
System.out.print (" ");
}
for (int j = 0; j < 2*i+1; j++) {
if((j == 0 || j == 2*i)){
System.out.print ("*");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
for (int i = n-2; i >= 0; i--) {
for (int j = 0; j < n-i-1; j++) {
System.out.print (" ");
}
for (int j = 0; j < 2*i+1; j++) {
if((j == 0 || j == 2*i)){
System.out.print ("*");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
}
/*
Right Triangle Number Pattern
Problem Statement: Write a Java program to print a right triangle number pattern up to n rows.
1
12
123
1234
12345
*/
public static void rightTriangleNumberPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j <= i; j++) {
System.out.print (j+1);
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print Pascal's Triangle of height n.
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
*/
public static void PascalsTriangle(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j < n-i-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <=i; j++) {
int p = factorial (i)/(factorial (j)*factorial ((i-j)));
System.out.print (" "+p);
}
System.out.println ();
}
}
private static int factorial(int n){
if (n ==0){
return 1;
}
int fact = 1;
for (int i = 1; i <=n ; i++) {
fact*=i;
}
return fact;
}
/*
Problem Statement: Write a Java program to print Pascal's Triangle of height n.
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
*/
public static void pyramidNumberPattern(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j < n-i-1; j++) {
System.out.print (" ");
}
int count =1 ;
for (int j = 0; j < 2*i+1; j++) {
if(j%2 == 0){
System.out.print (count);
count++;
}else {
System.out.print(" ");
}
}
System.out.println ();
}
}
public static void pyramidNumberPattern1(int n){
for (int i = 0; i < n; i++) {
for (int j = 0; j < n-i-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <=i; j++) {
int count = j+1;
System.out.print (" " + count);
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print Floyd's Triangle of height n.
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
*/
public static void floydsTriangle(int n){
int count = 1;
for (int i = 0; i < n; i++) {
for (int j = 0; j <= i; j++) {
System.out.print (" "+count++);
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a square number pattern with numbers from 1 to n. Each row should start from the first number of the sequence.
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
*/
public static void squareNumberPattern(int n){
int count = 1;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print (count++ + " ");
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a hollow square pattern of size n, with a border of stars.
*****
* *
* *
* *
*****
*/
public static void hollowSquarePattern (int n) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (j == 0 || j == n-1 || i == 0 || i == n -1 ){
System.out.print ("* ");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print a zigzag number pattern of height n, with numbers increasing row by row.
1
2 3
6 5 4
7 8 9 10
15 14 13 12 11
*/
public static void zigzagNumberPattern (int n) {
int count = 1;
for (int i = 0; i < n; i++) {
for (int j = 0; j <= i; j++) {
if (i != 0 && i%2 == 0){
System.out.print (count + " ");
count--;
}else {
System.out.print (count + " ");
count++;
}
}
if (i % 2 != 0){
count = count + i+1;
}else if(i!=0){
count += i+2;
}
System.out.println ();
}
}
/*
K Pattern
* * * * *
* * * *
* * *
* *
*
* *
* * *
* * * *
* * * * *
*/
public static void kPattern(int n){
for (int i = n; i > 0; i--) {
for (int j = 0; j <i; j++) {
System.out.print ("* ");
}
System.out.println ();
}
for (int i = 1; i < n; i++) {
for (int j = 0; j <=i; j++) {
System.out.print ("* ");
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print right Pascals Triangle of height n.
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
*/
public static void rightPascalsTriangle(int n){
for (int i = 0; i < 2*n; i++) {
for (int j = 0; i < n && j <=i; j++) {
System.out.print ("* ");
}
for (int j = n-1; i >= n && j > i - n; j--) {
System.out.print ("* ");
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print right Butterfly Star Pattern of height n.
* *
* * * *
* * * * * *
* * * * * * * *
* * * * * * * * * *
* * * * * * * * * *
* * * * * * * *
* * * * * *
* * * *
* *
*/
public static void butterflyStarPattern (int n) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < 2*n; j++) {
if(j <= i || j >= 2*n-i-1){
System.out.print ("* ");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
for (int i = n - 1; i >= 0; i--) {
for (int j = 0; j < 2*n; j++) {
if(j <= i || j >= 2*n-i-1){
System.out.print ("* ");
}else {
System.out.print (" ");
}
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print right Stop watch Star Pattern of height n.
* * * * * * * * *
* * * * * * *
* * * * *
* * *
*
* * *
* * * * *
* * * * * * *
* * * * * * * * *
*/
public static void stopWatchPattern(int n){
for (int i = n -1 ; i >= 0; i--) {
for (int j = 0; j < n-(i)-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <= 2*i; j++) {
System.out.print("* ");
}
System.out.println ();
}
for (int i = 1 ; i <= n-1; i++) {
for (int j = 0; j < n-(i)-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <= 2*i; j++) {
System.out.print("* ");
}
System.out.println ();
}
}
/*
Problem Statement: Write a Java program to print right Stop watch Hollow Star Pattern of height n.
* * * * * * * * *
* *
* *
* *
*
* *
* *
* *
* * * * * * * * *
*/
public static void stopWatchHollowPattern(int n){
for (int i = n -1 ; i >= 0; i--) {
for (int j = 0; j < n-(i)-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <= 2*i; j++) {
if(j == 0 || j == 2*i || i == 0 || i == n-1){
System.out.print("* ");
}else {
System.out.print(" ");
}
}
System.out.println ();
}
for (int i = 1 ; i <= n-1; i++) {
for (int j = 0; j < n-(i)-1; j++) {
System.out.print (" ");
}
for (int j = 0; j <= 2*i; j++) {
if(j == 0 || j == 2*i || i == 0 || i == n-1){
System.out.print("* ");
}else {
System.out.print(" ");
}
}
System.out.println ();
}
}
}
Subscribe to my newsletter
Read articles from Nethula Ganesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
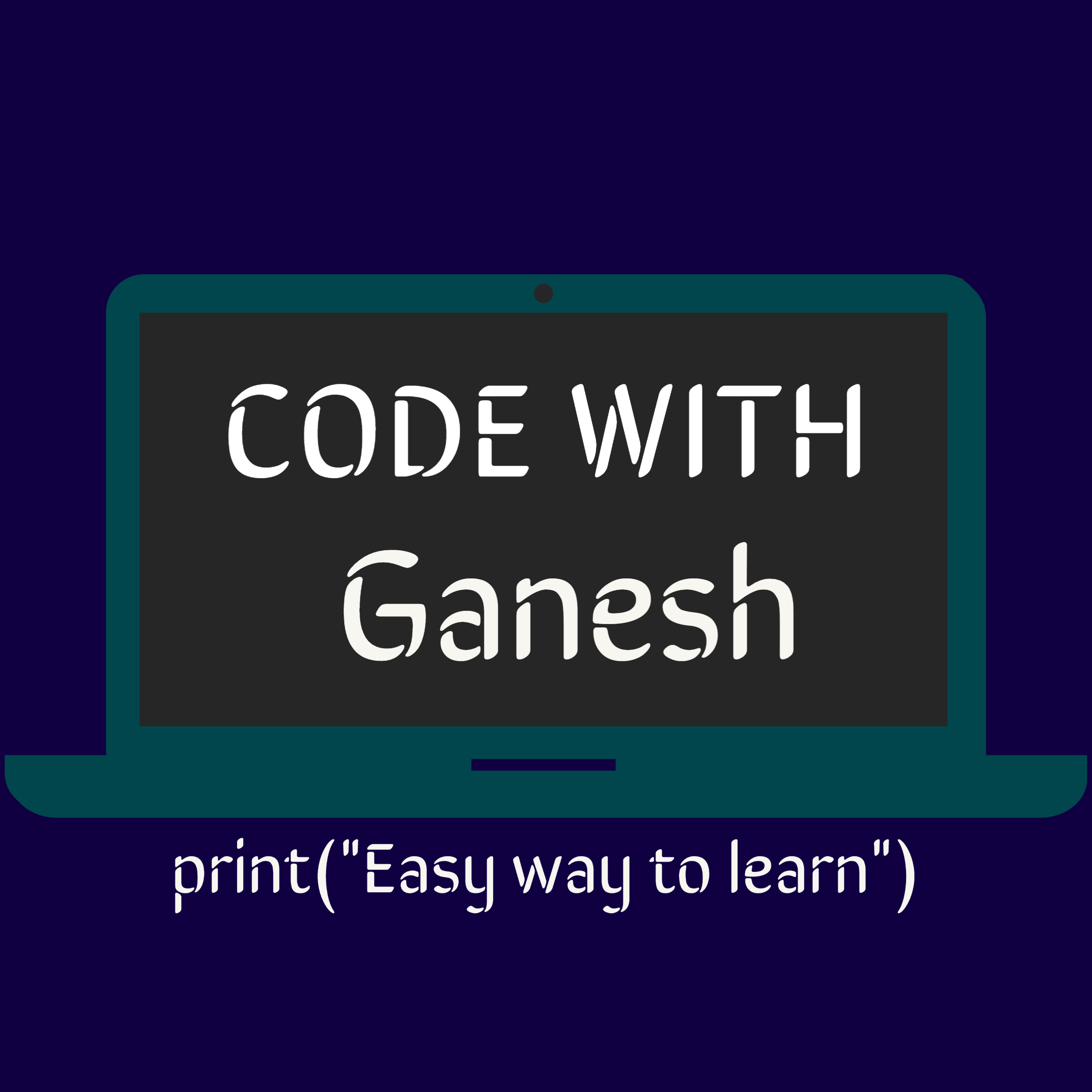