Constructor in Java

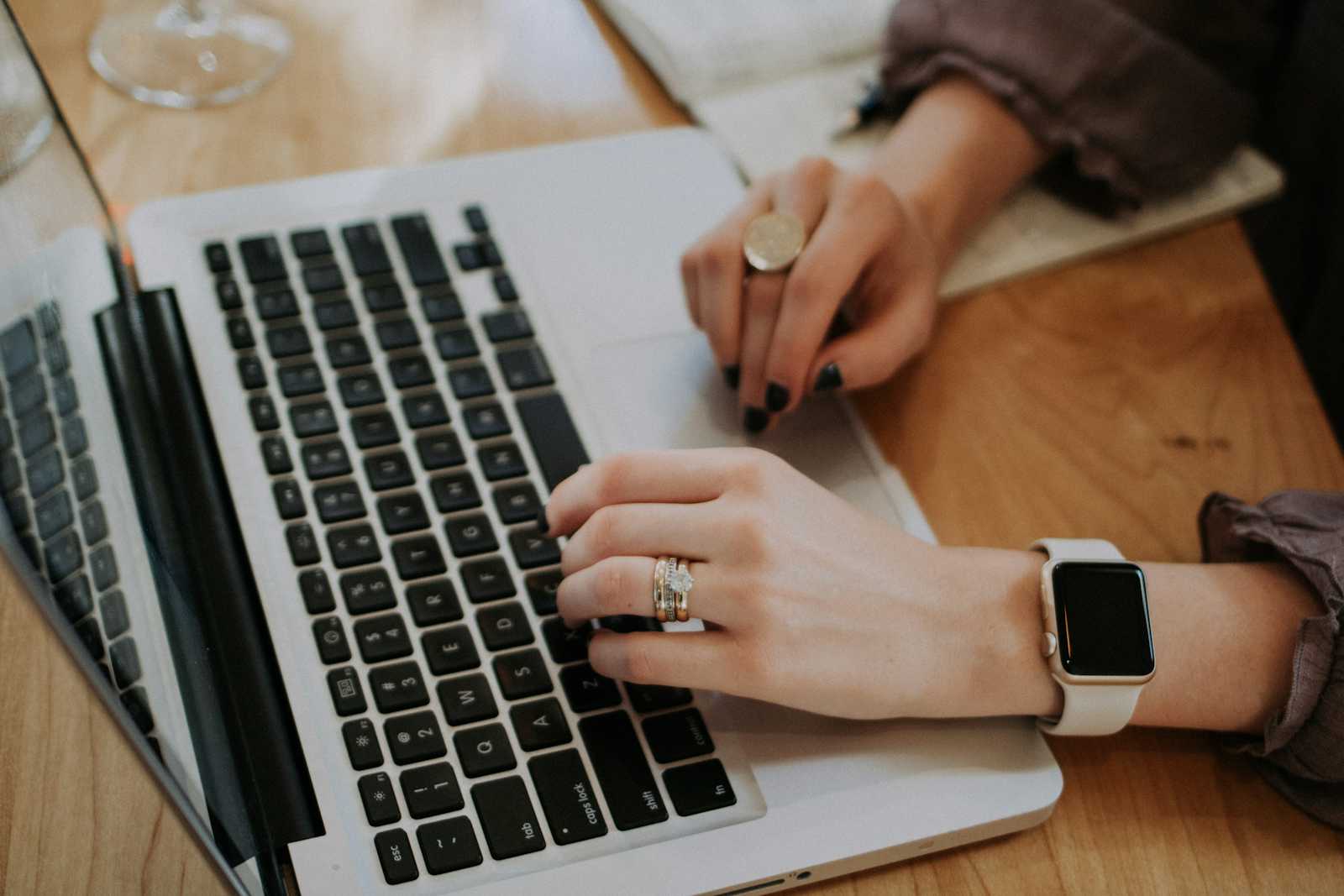
What is Constructor ?
Constructor is a type of method which gets executed when an object is created. Constructor name must be similar to class name. There are two types of constructors.
1. Constructor with out arguments (Default constructor).
2. Constructor with arguments.
Purpose of Constructor
The purpose of Constructor is to create an instance of a class and initialise it's instance variable.
Example for Default constructor in Java
import java.io.*;
class Demo
{
Public Demo()
{
System.out.println("Demo constructor");
}
public static void main (String [] args)
{
Demo d = new Demo();
}
}
Example for Constructor with arguments
import java.io.*;
class Demo
{
String name;
int rollno;
public Demo(String name,int rollno)
{
this.name=name;
this.rollno=rollno;
}
}
class Demo1
{
public static void main (String [] args)
{
Demo d = new Demo ("ABC", 30);
System.out.println("Demo Name :" + d.name + " and rollno :" + d.rollno);
}
}
Constructor Overloading
Developing multiple constructors with different arguments is called constructor Overloading. The purpose of Constructor overloading is to create same class object in multiple ways. In case of multiple constructors there might be code repetition, which must be avoided otherwise it leads to maintenance problem.
Example for constructor overloading
class Student
{
String name;
int rollno:
//Default constructor
public Student()
{
name = "unknown";
rollno =0;
}
//Constructor with parameters
public Student(String studentName,int studentRollno)
{
name = studentName;
rollno = studentRollno;
}
public void details()
{
System.out.println("Name: "+name+",Rollno: "+ rollno);
}
public static void main (String [] args)
{
Student s1 = new Student();
Student s2 = new Student("sree",20);
s1.details();
s2.details();
}
}
Constructor chaining
Invoking the constructor from another constructor is called constructor chaining. Inorder to avoid code repetition and achieve code reusability we use constructor chaining. Constructor chaining can be achieved by using call to “this” statement.
Example for constructor chaining
class Student
{
String name;
int id;
public Student(String name)
{
this.name = name;
}
public Student(String name,int id)
{
this(name);
this.id = id;
}
public void details ()
{
System.out.println("Name: " + name);
System.out.println("Id: " + id);
}
public static void main (String [] args)
{
Student s1 = new Student("Sree");
Student s2 = new Student("Joe",16);
s1.details();
s2.details();
}
}
Subscribe to my newsletter
Read articles from Sravya Talabathula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
