Chapter 2: Loops and Strings in Go – Creating Brilliant Code with Style 🚀

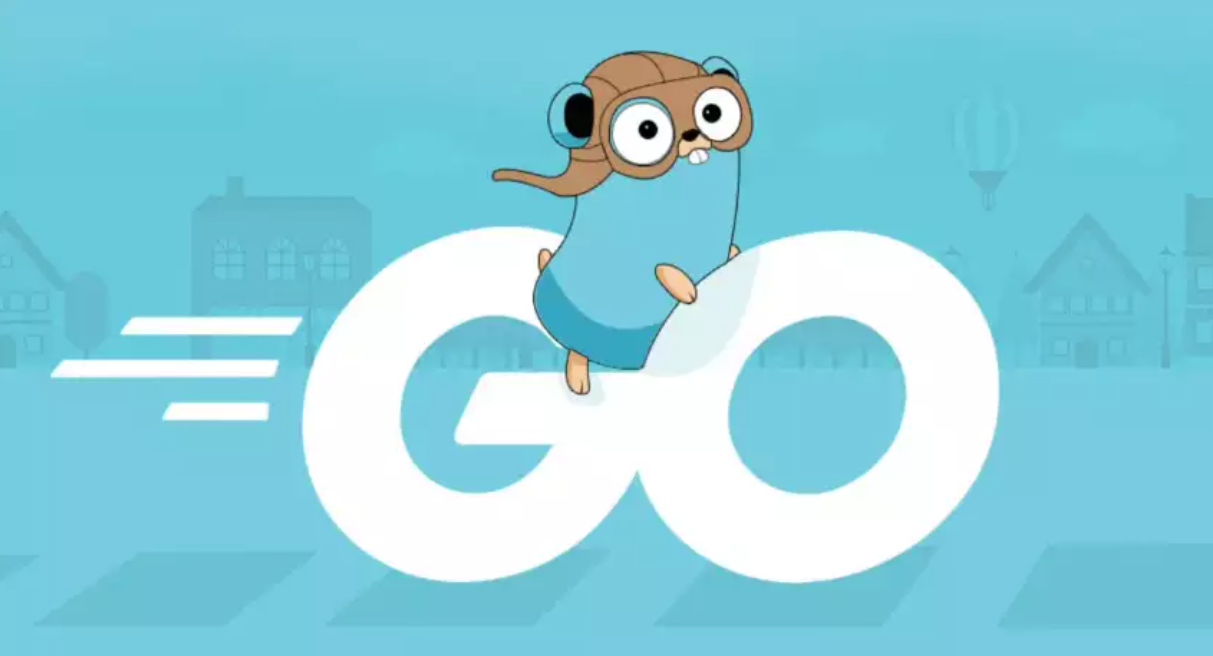
Welcome back, Go explorers! Ready to dive deeper into Go? So, it is time for our next chapter of Go programming. Today, we’re diving into two fundamental tools in coding: loops and strings. These might sound a bit trivial but these are the real big players when it comes to writing clean, well optimised code. And that is where loops and strings come to rescue either when automating chores or when improving the readability of your code. Ready? Let’s get started!
🎢 Loops in Go: The Power of Repetition
Loops are best described as the “repeat” of coding, and as coded procedures are repetitive in nature, then the logical step is to copy them in a routine. As you would have noticed, loops get you to do the same task again and again with only a few lines of code. In Go, loops are versatile and simple with just one main loop: the for loop. However, as you will see throughout this tutorial, with a little imagination, it will do just about everything you can throw at it!
1. The Simple for
Loop: Starting Easy
Here’s your classic for
loop: it stretches from a certain point and up to a certain point and has a limit that marks its fulfillment. Let us analyze it with an example of a friendly greeting!
package main
import "fmt"
func main() {
for i := 1; i <= 5; i++ {
fmt.Println("Hello, Go adventurers! 👋")
}
}
What a simple and short loop wrote this? Five calls “hello” was repeated in it. Fortunately, you do not have to write it out yourself as the loop takes care of it for you! Let's break it down:
i := 1
: We start at 1.i<= 5
: It will repeat the loop for 5 times and then stops.i++
: A new value of 1 toi
after each run thus taking it to five.
2. Loops That Count Down: Building Suspense
Counting down helps to create tension and has another benefit — timers. Here’s how to build a countdown:
package main
import "fmt"
func main() {
for i := 5; i > 0; i-- {
fmt.Printf("Countdown: %d...\n", i)
}
fmt.Println("🚀 Liftoff!")
}
3. Go’s Magic range
Loop: Perfect for Collections
It is when you need to segregate the things you have one box or container from the other one. Yes, range loops are your best friend in this case! Let’s loop through a list of friends and greet each of them:
package main
import "fmt"
func main() {
friends := []string{"Alex", "Jordan", "Taylor"}
for index, name := range friends {
fmt.Printf("Hello, %s! You’re friend number %d 🎉\n", name, index+1)
}
}
4. The Infinite Loop – Until You Say Stop 🛑
At other times, just like waiting for a given input, you may require an unlimited loop. Here’s how it works:
package main
import (
"fmt"
"time"
)
func main() {
i := 1
for {
fmt.Printf("Looping forever... %d\n", i)
time.Sleep(1 * time.Second) // Pause for 1 second
i++
if i > 3 { // Stop after three times
fmt.Println("Alright, time to exit 🚪")
break
}
}
}
In this case, it loops three times and then exits with break
.
5. Nested Loops: Perfect for Tables and Grids
Loops within other loops are possible and anytime there is structure, such as grids or tables of data, it can be useful. Let’s build a simple multiplication table:
package main
import "fmt"
func main() {
size := 3
fmt.Println("Multiplication Table:")
for i := 1; i <= size; i++ {
for j := 1; j <= size; j++ {
fmt.Printf("%d\t", i*j)
}
fmt.Println() // Newline after each row
}
}
This nested loop creates a 3x3 multiplication table. Awesome for developing grids or tables which makes development very easy.
🎨 Strings in Go: Unlocking the Magic of Text
Strings are how we manage text in Go. They look like simple, but the right tools can do impressive things; search, format and transform your text using the code making it both playful and practical.
1.Accessing Characters: Digging Into the Text
Need to get one or several themed characters from a string? Here’s how you can do it with indexing:
package main
import "fmt"
func main() {
greeting := "Hello, Go!"
fmt.Printf("First letter: %c\n", greeting[0])
fmt.Printf("Last letter: %c\n", greeting[len(greeting)-1])
}
2.Iteration Through Every Character in a String
Suppose, for instance, you would like to compare each character in isolation. You can do this by looping through the string with range:
package main
import "fmt"
func main() {
greeting := "Hello, Go!"
for index, char := range greeting {
fmt.Printf("Character at index %d: %c\n", index, char)
}
}
Each character is displayed with its position, helping you see exactly what’s going on.
3.Building Sentences
In Go, joining strings is really simple and we can simply concatenate two strings in Go. Let’s say you’re building a sentence from words:
package main
import (
"fmt"
"strings"
)
func main() {
words := []string{"Go", "is", "awesome!"}
sentence := strings.Join(words, " ")
fmt.Println(sentence)
}
4.Extracting Substrings (Without Special Functions)
But if to pull out part of a string, For pull it out of itself there must be some path. In Go, you can convert the string to a slice of bytes and use slicing:
package main
import "fmt"
func main() {
str := "Learning Go is fun!"
substr := str[9:11] // From index 9 to 10 (inclusive)
fmt.Println("Substring:", substr)
}
Here, "Go"
is extracted from "Learning Go is fun!"
by specifying the start and end indexes.
5.Comparing Strings in Go
String comparison in Go is simple and exact—Go’s comparisons are case-sensitive:
package main
import "fmt"
func main() {
str1 := "Go"
str2 := "go"
if str1 == str2 {
fmt.Println("The strings are identical!")
} else {
fmt.Println("The strings are different.")
}
}
Since Go treats uppercase and lowercase differently, “Go” and “go” aren’t considered the same.
6.Overview of String Searching – Does It Contain?
There are generally situations where you only want to see if a word or letter is in a string or not. Go’s strings package has built-in helpers for this:
package main
import (
"fmt"
"strings"
)
func main() {
phrase := "Go is powerful!"
fmt.Println(strings.Contains(phrase, "powerful"))
fmt.Println(strings.HasPrefix(phrase, "Go"))
fmt.Println(strings.HasSuffix(phrase, "!"))
}
Contains
checks for a substringHasPrefix
indicates if the beginning of a string matches a given text.HasSuffix
determines whether or not it terminates with some string.
7.Breaking Strings Down: Split
For the Split toolkit, what is most obviously useful is the capability of splitting a sentence or a phrase into words. Here’s an example:
package main
import (
"fmt"
"strings"
)
func main() {
sentence := "Go is fun to learn"
words := strings.Split(sentence, " ")
fmt.Println(words)
}
By applying using Split
we are now able to take the sentence and split it down into words. Thus, each word is an element in the slice to be ready for use as needed.
8.Conversion of the Upper and Lower case
Making strings all uppercase or lowercase is simple with these functions:
package main
import (
"fmt"
"strings"
)
func main() {
phrase := "Go is Great!"
fmt.Println(strings.ToUpper(phrase)) // "GO IS GREAT!"
fmt.Println(strings.ToLower(phrase)) // "go is great!"
}
This is especially useful when you want uniform case in text or handling any case sensitive task.
**Wrapping Up: Join Me on This Journey!**🌟
Amazing!!! 🙌🙌 You have successfully enhance your Go programming knowledge a notch higher! You have found the basics of loops
and strings
and discovered how even these basic workhorses of programming can help automate, format output, and manipulate text.
In the next chapter, we will extend our knowledge and explore such topics as data structures
, collections
and other forms of data organization. We will bring our skill level to the next level to help you face more complex data and challenges.
If you found this chapter helpful, please consider subscribing to my blog for more insights and tutorials! 🌟 Your support means a lot and helps me continue sharing knowledge and resources.
Also, if you enjoyed this content, please leave a like ❤️! Your feedback is invaluable and encourages me to keep creating more valuable content.
Keep experimenting with the code, and don’t hesitate to modify and play around with it. Happy coding!💻🚀
Subscribe to my newsletter
Read articles from Denish directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
