Optimizing Memory Usage in Laravel: How to Use chunk(), chunkById(), and Lazy Collections for Efficient Large Dataset Processing

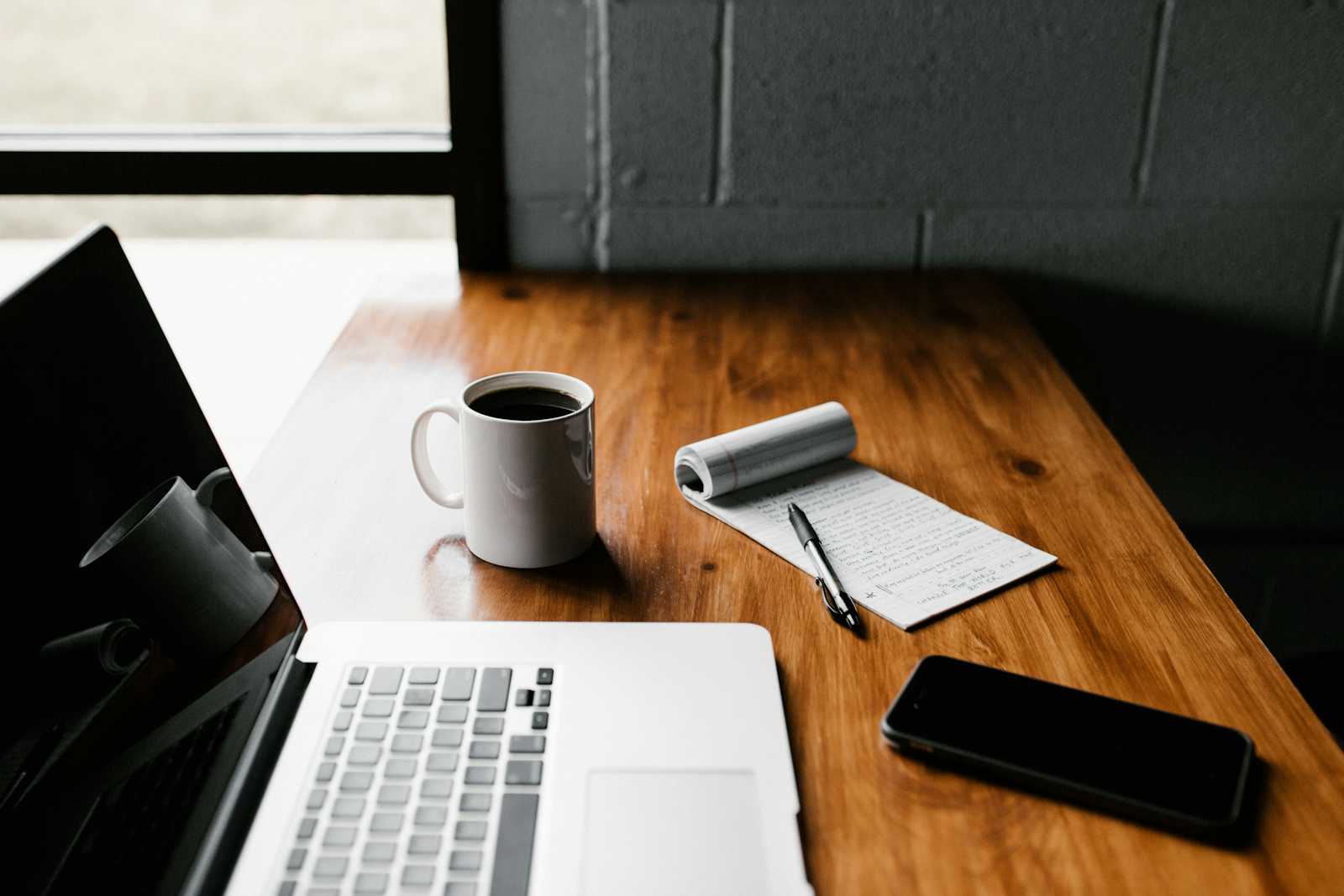
If you're working with large amounts of data in Laravel, you might run into issues with memory usage. For example, if you try to load 100,000 records into memory all at once, your application might run out of memory and crash. Luckily, Laravel provides a few helpful methods to work with large datasets efficiently, without using too much memory. These methods are chunk()
, chunkById()
, and Lazy Collections.
In this post, I’ll explain how each of these methods works and show you how to use them with simple examples.
What is the chunk()
Method?
The chunk()
method allows you to retrieve and process records in smaller pieces, or "chunks". Instead of loading all your data into memory at once, you can tell Laravel to load just a small part (like 100 or 200 records) at a time, process it, and then load the next batch. This saves memory and keeps your app running smoothly.
Example:
Let’s say you have a User
model with thousands of records, and you want to update each user’s status. Instead of loading all the users at once, you can use chunk()
to process them in smaller groups.
use App\Models\User;
User::chunk(200, function ($users) {
foreach ($users as $user) {
// Process each user here
$user->update(['status' => 'active']);
}
});
In this example, User::chunk(200)
will fetch 200 users at a time, process them, and then fetch the next 200 users. This continues until all users are processed.
Why Use chunk()
?
Reduces Memory Usage: It only loads a small batch of records at a time.
Scalable: It helps your app handle large datasets without crashing.
What is the chunkById()
Method?
chunkById()
is similar to chunk()
, but it’s more reliable when you need to update records while processing them. The main difference is that chunkById()
makes sure the records are processed in the correct order, based on their id
values.
This is especially helpful if you’re updating the records as you process them. Without it, you might accidentally skip records or process some records more than once.
Example:
If you need to mark all User
records as "active", you can use chunkById()
to make sure each record is processed in order, even if you're updating the data during the loop.
use App\Models\User;
User::chunkById(200, function ($users) {
foreach ($users as $user) {
// Update each user
$user->update(['status' => 'active']);
}
}, 'id');
In this example, chunkById(200)
retrieves the users in batches of 200, ordered by their id
. The id
ensures that the chunks are processed in the correct order, so you don’t miss any records or process them multiple times.
Why Use chunkById()
?
Order Matters: It keeps the order of records based on their
id
so you don’t skip or repeat records.Efficient for Updates: It’s the best choice when updating data during processing.
What are Lazy Collections?
Lazy Collections are a memory-efficient way to process records one by one, without loading the entire dataset into memory. The lazy()
method retrieves records as needed, without storing all of them in memory at once. This is helpful when you need to process a very large number of records, but you don’t need to process them in chunks.
Example:
If you just want to loop through each User
record one at a time and do something (like sending an email), you can use lazy()
.
use App\Models\User;
foreach (User::lazy() as $user) {
// Process each user
$user->update(['status' => 'active']);
}
In this example, User::lazy()
returns the users one by one, so only one user is in memory at a time. This keeps your app’s memory usage very low.
Why Use Lazy Collections?
Super Memory-Efficient: It processes one record at a time, keeping memory usage low.
Simpler for Large Datasets: It’s great when you don’t need to work with chunks but still want to avoid memory overload.
When Should You Use chunk()
vs. lazy()
?
Use
chunk()
: When you need to process a large set of records in batches (like 100 or 200 at a time). It’s perfect for tasks like updating, deleting, or transforming data in groups.Use
lazy()
: When you want to process each record one by one, without worrying about chunks. It’s ideal when you don’t need to perform batch operations, and you just need to process a large number of records one after the other.
Conclusion
In Laravel, there are a few powerful methods you can use to process large datasets without running into memory issues:
chunk()
: Processes records in batches, reducing memory usage when working with large datasets.chunkById()
: Similar tochunk()
, but more reliable when updating records while processing.Lazy Collections: Processes records one by one, without using much memory.
By using these methods, you can ensure that your Laravel application can handle large datasets efficiently, without running into performance problems or memory issues.
Subscribe to my newsletter
Read articles from Asfia Aiman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfia Aiman
Asfia Aiman
Hey Hashnode community! I'm Asfia Aiman, a seasoned web developer with three years of experience. My expertise includes HTML, CSS, JavaScript, jQuery, AJAX for front-end, PHP, Bootstrap, Laravel for back-end, and MySQL for databases. I prioritize client satisfaction, delivering tailor-made solutions with a focus on quality. Currently expanding my skills with Vue.js. Let's connect and explore how I can bring my passion and experience to your projects! Reach out to discuss collaborations or learn more about my skills. Excited to build something amazing together! If you like my blogs, buy me a coffee here https://www.buymeacoffee.com/asfiaaiman