Why 78% of Rails Upgrades Fail: And How to Ensure Yours Doesn't

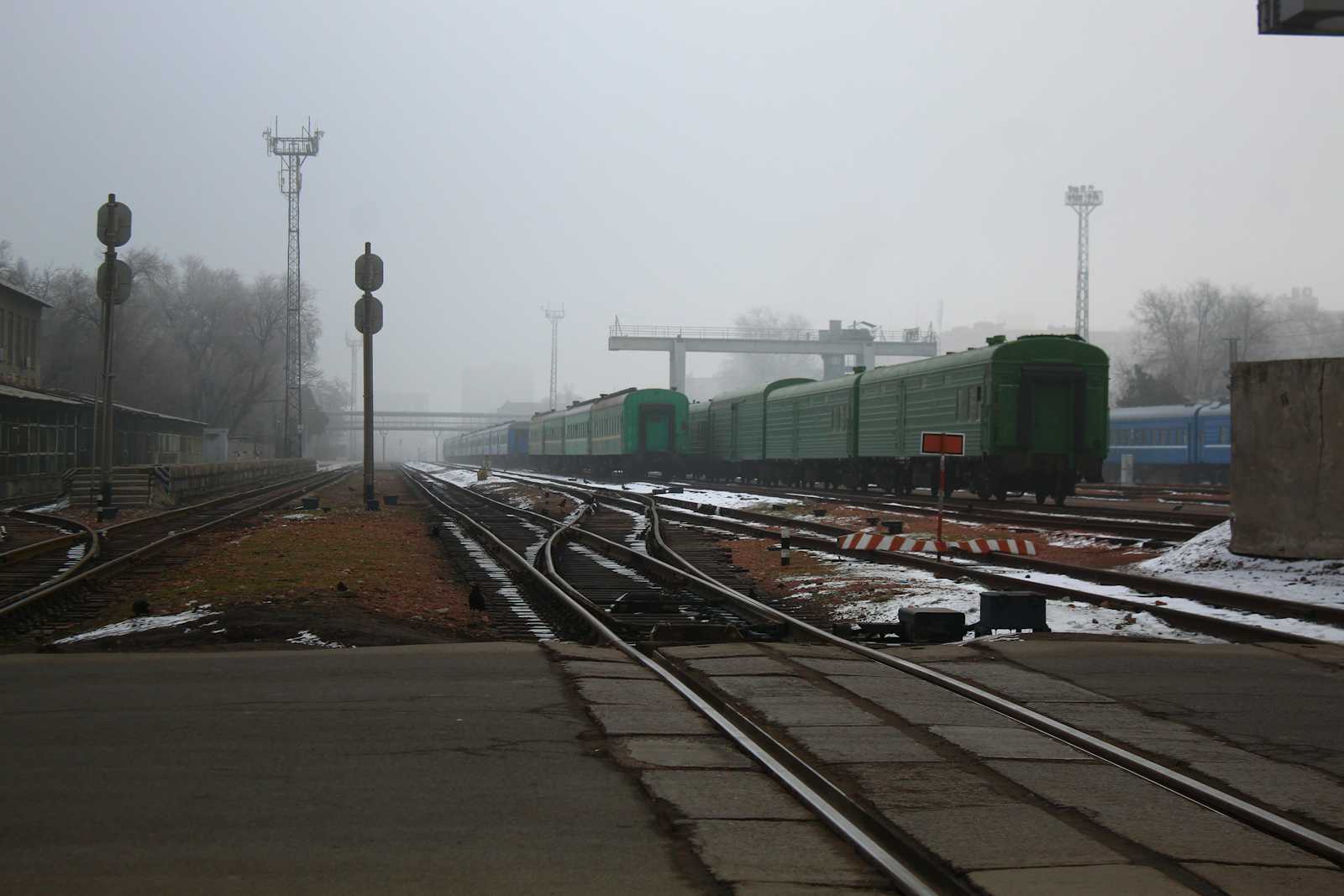
1. Understanding the Upgrade Landscape
Rails upgrades represent a significant technical challenge for development teams.
Major version upgrades often introduce breaking changes, deprecations, and new architectural patterns that can impact every layer of your application.
To navigate these challenges successfully, teams need to understand the key factors that affect upgrade success.
The key challenges teams typically face include:
Dependency conflicts between gems that previously worked together
Breaking changes in core Rails APIs and functionality
Database compatibility issues, particularly with Active Record changes
Performance regressions from changes in Rails internals
Security updates that require application-level changes
Testing coverage gaps that fail to catch edge cases
Legacy code patterns that become incompatible
2. Common Causes of Failed Upgrades
2.1 Insufficient Test Coverage
Many teams discover their test suites fail to catch critical issues during upgrades.
Tests that worked perfectly in older versions may miss problems introduced by new Rails behaviors, especially around edge cases and integration points.
2.2 Dependency Hell
Version conflicts between gems create complex dependency chains that can seem impossible to resolve.
This often occurs when different gems rely on conflicting versions of shared dependencies.
2.3 Custom Middleware and Engines
Applications with custom middleware or Rails engines frequently encounter compatibility issues during upgrades, especially when they rely on internal Rails APIs that have changed between versions.
2.4 Database-Specific Code
Code that interfaces directly with the database or uses database-specific features often breaks during upgrades due to changes in Active Record or database adapter implementations.
3. Pre-Upgrade Assessment and Preparation
Before starting an upgrade to Rails 8, it’s crucial to perform an in-depth assessment of your existing application.
This pre-upgrade audit helps identify areas that might require attention, ensuring a smoother transition.
The automated audit tool provided below creates a comprehensive compatibility report to uncover potential blockers before the migration begins.
3.1 The Upgrade Audit Tool
The Upgrade Audit Tool is a Rake task designed to automate the assessment of your application’s compatibility with Rails 8.
This tool is crucial for catching compatibility issues early on, as manual inspection can often miss intricate details or introduce errors.
Using this tool enables a systematic review of deprecated methods, middleware, database-specific code, and test coverage, helping prevent unexpected issues during or after the upgrade.
Upgrade Audit Task Code
Here’s the code for the audit tool, which resides in lib/tasks/upgrade_audit.rake
:
# lib/tasks/upgrade_audit.rake
namespace :upgrade do
desc "Audit application for Rails upgrade compatibility"
task audit: :environment do
class UpgradeAuditor
def self.run
new.run
end
def run
audit_results = {
deprecated_methods: find_deprecated_methods,
custom_middleware: audit_middleware,
database_specifics: audit_database_code,
test_coverage: calculate_test_coverage
}
generate_report(audit_results)
end
private
def find_deprecated_methods
deprecated_calls = []
Rails.root.glob("app/**/*.rb").each do |file|
content = File.read(file)
deprecated_calls << {
file: file,
calls: scan_for_deprecated_methods(content)
}
end
deprecated_calls
end
def audit_middleware
Rails.application.middleware.map(&:inspect)
end
def audit_database_code
ActiveRecord::Base.connection.schema_cache.columns.keys
end
def calculate_test_coverage
coverage_files = Dir.glob("coverage/.resultset.json")
return "No coverage data found" if coverage_files.empty?
JSON.parse(File.read(coverage_files.first))
end
def generate_report(results)
File.open("tmp/upgrade_audit.json", "w") do |f|
f.write(JSON.pretty_generate(results))
end
end
end
UpgradeAuditor.run
puts "Audit complete. Results saved to tmp/upgrade_audit.json"
end
end
What the Upgrade Audit Tool Covers
This audit tool systematically scans various parts of your application and generates a detailed report saved as tmp/upgrade_audit.json
.
Here’s a breakdown of what it checks:
Deprecated Method Calls: The tool searches for deprecated methods across all Ruby files in the application (typically in
app/**/*.rb
). Deprecated methods may no longer be supported in Rails 8, so identifying them in advance allows you to update or refactor these methods to ensure compatibility.Custom Middleware Configurations: Custom middleware might need adjustments or replacements in Rails 8 due to changes in middleware handling. The audit tool retrieves a list of all middleware currently configured in the application, which you can then review for compatibility or potential deprecations.
Database-Specific Code: The audit tool scans the database schema and queries to identify areas that might be affected by ActiveRecord changes in Rails 8. This includes checking columns, indexes, and any database-specific customizations that may need modification to work seamlessly in the upgraded environment.
Test Coverage Analysis: Ensuring adequate test coverage before an upgrade is crucial for catching regressions and issues. The audit tool checks for test coverage data (typically located in
coverage/.resultset.json
) and includes it in the report. This allows you to identify gaps in test coverage and add tests as needed, reducing the risk of encountering untested errors post-upgrade.
Why This Audit Tool is Essential
Upgrading a Rails application can introduce breaking changes, especially in a major version like Rails 8.
Manually identifying compatibility issues is not only time-consuming but also prone to human error, especially in large applications.
By automating this audit:
Reduces the chance of unexpected failures by catching compatibility issues early.
Increases efficiency by automating repetitive tasks, allowing you to focus on resolving any identified issues.
Supports better planning by providing a structured report that helps gauge the scale of the work involved in the upgrade.
This Upgrade Audit Tool is a critical first step in the Rails 8 upgrade process, providing a solid foundation for a smoother and more predictable upgrade path.
Running the Pre-Upgrade Assessment
The Upgrade Audit Tool helps identify potential blockers before the upgrade by scanning your app for deprecated methods, middleware configurations, database-specific code, and test coverage.
Steps
Save the code in a file located at
lib/tasks/upgrade_audit.rake
.Run the Rake task in your terminal:
bin/rake upgrade:audit
Check the generated report in
tmp/upgrade_audit.json
:This report will detail any deprecated methods, middleware configurations, database schema information, and test coverage data.
Review this report to identify compatibility issues and areas needing code adjustments before proceeding with the upgrade.
Resolve flagged issues as per the report before moving forward with the upgrade process.
4. Step-by-Step Upgrade Process
Upgrading a Rails application, especially for a major release, involves managing multiple interdependent tasks, from resolving deprecations to handling migrations and verifying compatibility with other dependencies.
The RailsUpgrader
module is designed to simplify this complex process by providing essential tools that allow you to capture deprecation warnings, manage database migrations safely, and verify gem compatibility.
Rails Upgrader Module
This module includes three key components to streamline and safeguard the upgrade process.
It helps to systematically track deprecations, handle migrations with rollback options, and ensure that all dependencies meet the requirements of the new Rails version.
Upgrade Module Code
The following code, located in lib/rails_upgrader.rb
, defines each component of the RailsUpgrader
module:
module RailsUpgrader
class DeprecationHandler
def self.track_deprecations
deprecations = []
ActiveSupport::Deprecation.behavior = ->(message, callstack) {
deprecations << {
message: message,
location: callstack.first,
timestamp: Time.current
}
}
yield
deprecations
ensure
ActiveSupport::Deprecation.behavior = :stderr
end
end
class DatabaseMigrator
def self.validate_migrations
pending = ActiveRecord::Migration.check_pending!
true
rescue ActiveRecord::PendingMigrationError
false
end
def self.safe_migrate
return false unless validate_migrations
ActiveRecord::Base.transaction do
begin
ActiveRecord::Migrator.migrate(ActiveRecord::Migrator.migrations_paths)
true
rescue => e
ActiveRecord::Base.connection.rollback_transaction
Rails.logger.error "Migration failed: #{e.message}"
false
end
end
end
end
class DependencyResolver
def self.check_compatibility
compatibility_issues = []
Bundler.definition.specs.each do |spec|
next unless spec.respond_to?(:required_ruby_version)
unless spec.required_ruby_version.satisfied_by?(Gem::Version.new(RUBY_VERSION))
compatibility_issues << {
gem: spec.name,
version: spec.version,
required_ruby: spec.required_ruby_version.to_s
}
end
end
compatibility_issues
end
end
end
Key Components of the Rails Upgrader Module
DeprecationHandler:
Purpose: Automatically tracks and logs deprecation warnings.
How it works: The
track_deprecations
method temporarily overrides Rails’ deprecation logging behavior, capturing all deprecation messages and their stack traces in a structured format. These details are crucial for identifying specific methods or features that need refactoring before the Rails 8 upgrade.
DatabaseMigrator:
Purpose: Manages database migrations with built-in safety mechanisms.
How it works: The
validate_migrations
method checks if there are pending migrations, while thesafe_migrate
method attempts to run migrations within a transaction. If a migration fails, it rolls back all changes, ensuring that your database remains consistent. This mechanism prevents half-completed migrations, reducing the risk of data inconsistencies.
DependencyResolver:
Purpose: Identifies gems that may be incompatible with Rails 8.
How it works: The
check_compatibility
method inspects each gem’s required Ruby version and flags those that don’t meet the Ruby version used by Rails 8. This helps you proactively identify dependency issues, so you can update or replace incompatible gems before the upgrade.
Together, these tools help manage and track critical aspects of the upgrade, providing the structure needed to detect issues early and resolve them efficiently.
Running the Step-by-Step Upgrade Process
The RailsUpgrader module in lib/rails_upgrader.rb
provides utilities to help you manage deprecations, migrations, and dependency compatibility as you upgrade.
Follow the Steps as below:
Save the code in a file located at
lib/rails_upgrader.rb
.Use the RailsUpgrader Module to perform specific upgrade tasks:
Deprecation Tracking: Wrap upgrade code within
DeprecationHandler.track_deprecations
to capture all deprecation warnings.RailsUpgrader::DeprecationHandler.track_deprecations do # Your code here, e.g., initialize or run the application in a sandbox environment end
This will log deprecation warnings with timestamps and locations, which can be reviewed to guide refactoring efforts.
Database Migration Check and Rollback:
if RailsUpgrader::DatabaseMigrator.safe_migrate puts "Migration completed successfully" else puts "Migration failed and was rolled back" end
This ensures that migrations will rollback if any issues are encountered, keeping your database in a consistent state.
Gem Dependency Compatibility:
compatibility_issues = RailsUpgrader::DependencyResolver.check_compatibility puts "Compatibility issues found:" if compatibility_issues.any? compatibility_issues.each do |issue| puts "#{issue[:gem]} (#{issue[:version]}): Requires Ruby #{issue[:required_ruby]}" end
This command checks for gems that may have compatibility issues with the new Rails version and prints the details for each incompatible gem.
Review Output and Logs:
- Resolve any issues related to deprecations, database migrations, and gem dependencies based on the outputs of each component.
5. Testing Strategies
Testing is an essential part of the upgrade process.
To verify that your application behaves consistently before and after the upgrade, you need comprehensive tests that cover critical functionality and user flows.
The UpgradeTestHelpers
module provides testing helpers that allow you to compare application behavior across versions, check for consistent API responses, and detect breaking changes.
Upgrade Test Helpers Code
The following code, located in spec/support/upgrade_test_helpers.rb
, defines the UpgradeTestHelpers
module, which supports automated comparison testing and compatibility verification.
module UpgradeTestHelpers
extend ActiveSupport::Concern
class_methods do
def verify_backwards_compatibility(&block)
around do |example|
old_behavior = capture_old_behavior(&block)
example.run
new_behavior = capture_new_behavior(&block)
expect(new_behavior).to eq(old_behavior)
end
end
end
def capture_old_behavior
result = nil
ActiveSupport::BacktraceCleaner.silence do
Rails.logger.silence do
result = yield
end
end
result
end
def capture_new_behavior
capture_old_behavior { yield }
end
end
RSpec.configure do |config|
config.include UpgradeTestHelpers
end
Example Usage in Tests
The following example demonstrates how to use verify_backwards_compatibility
to compare old and new behaviors for a critical feature.
In this case, it ensures that user score calculations remain consistent after the upgrade:
RSpec.describe "API Compatibility" do
verify_backwards_compatibility {
# Test critical functionality
User.find_each.map(&:calculated_score)
}
it "maintains response format" do
expect {
get api_endpoint_path
}.not_to change {
response.content_type
}
end
end
Key Benefits of Upgrade Test Helpers
Automated Behavior Comparison:
Purpose: Helps verify that core behaviors remain consistent after the upgrade.
How it works: The
verify_backwards_compatibility
method captures the pre-upgrade behavior (old behavior) and compares it to the post-upgrade behavior (new behavior), allowing you to identify any unexpected changes in critical functionalities.
API Response Verification:
Purpose: Ensures that API responses maintain a consistent format and structure.
How it works: Tests can verify that response formats, content types, and other response attributes remain unchanged. This is especially important for applications with external clients that rely on stable APIs.
Early Detection of Breaking Changes:
Purpose: Identifies potential breaking changes early in the testing phase, allowing you to address them before production deployment.
How it works: Since
verify_backwards_compatibility
captures both old and new behavior, any mismatch between the two signals a breaking change, which can then be addressed in the codebase.
By combining automated comparison testing with comprehensive API checks, the UpgradeTestHelpers
module helps ensure a smooth transition to Rails 8 with minimal risk to your application’s stability and reliability.
These structured upgrade processes and testing strategies offer a detailed and organized approach to handling a Rails 8 upgrade, helping to ensure compatibility and performance while reducing unexpected issues.
Running Testing Strategies
Testing your application thoroughly before, during, and after the upgrade is essential.
The UpgradeTestHelpers
module provides methods for comparing old and new behaviors, which helps detect breaking changes and maintain API consistency.
Follow the Steps as below:
Save the code in a file located at
spec/support/upgrade_test_helpers.rb
.Configure RSpec to include
UpgradeTestHelpers
:In
spec/rails_helper.rb
orspec/spec_helper.rb
, add:require_relative 'support/upgrade_test_helpers'
Use Backwards Compatibility Tests in Specs:
In your specs, wrap critical functionality in
verify_backwards_compatibility
blocks to compare old vs. new behavior.Example: To verify backwards compatibility for a specific API response:
RSpec.describe "API Compatibility" do verify_backwards_compatibility do # Test critical functionality, such as score calculation User.find_each.map(&:calculated_score) end it "maintains response format" do expect { get api_endpoint_path }.not_to change { response.content_type } end end
Run the Tests:
bin/rspec
Review Test Results:
Use the test output to identify any failing tests, which may indicate breaking changes introduced by the upgrade.
Address any issues by refactoring code, updating dependencies, or adjusting database migrations as needed.
6. General Recommendations
Isolate the Upgrade: Use a separate branch or environment to run these upgrade processes so you can identify and resolve issues without affecting production.
Commit Changes Incrementally: Each time you complete a step and resolve a set of issues, commit your changes. This will make it easier to track progress and revert if necessary.
Document Fixes: Keep a log of deprecated methods, updated database migrations, or replaced dependencies for future reference.
7. Post-Upgrade Optimization
After completing the Ruby on Rails version 8 upgrade, the focus shifts to enhancing application performance, maintaining code quality, and ensuring seamless functionality.
7.1 Performance Monitoring
Monitor and analyze application performance to ensure optimal functionality post-upgrade:
Application response times: Measure the time taken to process and respond to requests, ensuring faster user experiences.
Memory usage patterns: Identify and optimize memory-intensive operations to prevent excessive resource consumption.
Database query performance: Monitor and fine-tune database queries to minimize latency and improve data retrieval speeds.
CPU utilization: Track and balance CPU usage to prevent server overloads and maintain stable performance.
Background job processing: Ensure smooth and efficient execution of background tasks such as email sending or data processing.
7.2 Code Cleanup
Address technical debt and modernize the codebase introduced during the upgrade process:
Delete unused code and dependencies: Remove obsolete code and libraries to streamline the application.
Update outdated patterns: Replace old coding styles with modern, efficient practices to align with Rails 8 standards.
Remove temporary compatibility layers: Eliminate stopgap measures used to maintain compatibility during the upgrade.
Modernize deprecated code patterns: Refactor code to utilize new Rails features and best practices.
Clean up custom patches: Replace temporary fixes with long-term, stable solutions integrated into Rails 8.
8. Case Studies and Solutions
8.1 Common Issues and Resolutions
Address typical challenges encountered during Rails upgrades:
ActiveRecord Query Interface Changes:
Modernize query syntax to comply with Rails 8 standards.
Implement query abstraction layers for flexibility and maintainability.
Update custom scopes and relations to ensure compatibility with the latest ActiveRecord updates.
Asset Pipeline Changes:
Migrate to modern asset-handling systems like Webpacker or CSS bundling.
Update asset compilation configurations to meet new requirements.
Restructure asset organization for improved maintainability and performance.
Cookie Serialization:
Update serialization formats to enhance security and compatibility.
Implement data migration strategies to safely transition existing cookies.
Handle legacy cookie formats to ensure seamless user sessions.
Authentication Changes:
Update authentication mechanisms to align with new security standards in Rails 8.
Implement new security features, such as multi-factor authentication (MFA).
Migrate user sessions to updated storage and handling mechanisms.
9. Tools and Resources
9.1 Essential Tools
Utilize these tools to facilitate the upgrade process and maintain code quality:
RuboCop Rails:
Enforces Rails-specific best practices.
Identifies and suggests fixes for upgrade-related code issues.
Automates code modernization for consistent improvements.
Bundler-audit:
Scans for known security vulnerabilities in gems.
Verifies gem compatibility with Rails 8.
Suggests safe version updates for dependencies.
Rails Upgrade Guide:
Provides official migration paths tailored for Rails 8.
Details breaking changes and how to address them.
Offers community-driven solutions and recommendations.
9.2 Monitoring Tools
Ensure application stability and catch issues early using these monitoring solutions:
Performance Monitoring:
New Relic: Tracks application metrics, including response times and error rates.
Scout: Monitors server performance for bottlenecks and resource issues.
Datadog: Provides infrastructure insights for comprehensive system health checks.
Error Tracking:
Rollbar: Captures exceptions and provides detailed diagnostics for resolution.
Sentry: Offers real-time error tracking and alerting for immediate action.
Airbrake: Monitors deployments and flags potential issues early.
Conclusion
Upgrading a Rails application to a new major version is a complex process that can greatly enhance the application’s performance, security, and maintainability. However, without a structured approach, upgrades can introduce unexpected issues and disrupt functionality. A well-planned and methodical upgrade strategy is essential for ensuring a smooth transition.
Key Elements of a Successful Rails Upgrade
Thorough Preparation and Auditing
- Conducting a comprehensive pre-upgrade assessment is crucial. Tools like the upgrade audit (introduced in section 3) help identify potential blockers early on, such as deprecated methods, custom middleware, and database-specific code. These tools save time and effort by pinpointing areas requiring adjustments before the upgrade process even begins.
Incremental Upgrade Steps
- Upgrading Rails version by version, rather than jumping multiple versions, reduces the risk of compatibility issues and makes it easier to identify the source of any problems. The
RailsUpgrader
module (section 4) provides helpful tools to assist with managing this incremental process, including tracking deprecations, safely handling database migrations, and verifying gem dependencies. By isolating each step, you can focus on fixing issues gradually and confidently.
- Upgrading Rails version by version, rather than jumping multiple versions, reduces the risk of compatibility issues and makes it easier to identify the source of any problems. The
Comprehensive Testing
- Thorough testing is at the core of a successful upgrade. The
UpgradeTestHelpers
module (section 5) helps verify that critical functionalities behave as expected before and after the upgrade, minimizing the risk of introducing breaking changes. By conducting tests at every stage, teams can identify any issues early, ensure backward compatibility, and verify API response consistency, ultimately preserving the integrity of the application.
- Thorough testing is at the core of a successful upgrade. The
Careful Performance Monitoring
- After completing the upgrade, closely monitor application performance to detect and resolve any potential slowdowns or memory usage issues. Tools like New Relic, Datadog, and Scout provide insights into response times, CPU utilization, database query efficiency, and background job processing, enabling you to identify and address any bottlenecks introduced during the upgrade.
Systematic Code Cleanup
- With the upgrade completed, it’s important to remove any temporary compatibility layers, unused code, or outdated dependencies that were necessary during the transition. Regular code cleanup helps reduce technical debt, enhance code readability, and improve maintainability, ensuring that your application remains efficient and easy to develop in the future.
Updated Documentation
- Documenting changes made during the upgrade, including updated dependencies, configuration changes, and modified functionalities, is essential for knowledge sharing within the team. Updated documentation aids onboarding and provides a reference point for future upgrades, helping maintain long-term application stability.
Final Thoughts
By following these guidelines and utilizing the provided tools and modules, teams can approach Rails upgrades with confidence and precision.
A well-planned upgrade process not only ensures compatibility with the latest Rails features but also enhances the application’s performance, security, and maintainability.
With careful planning, comprehensive testing, and systematic cleanup, teams can significantly reduce upgrade-related disruptions and lay a solid foundation for future improvements.
Subscribe to my newsletter
Read articles from Chetan Mittal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chetan Mittal
Chetan Mittal
I stumbled upon Ruby on Rails beta version in 2005 and has been using it since then. I have also trained multiple Rails developers all over the globe. Currently, providing consulting and advising companies on how to upgrade, secure, optimize, monitor, modernize, and scale their Rails apps.