Boost Your Link Previews: Implementing Open Graph Images in Next.js

Table of contents
- Introduction
- Fallback: Implementing Open Graph Images in Plain HTML
- Implementing Open Graph Images in Next.js
- Diving Deeper: Platform-Specific Open Graph Implementation
- Example: Open Graph Image in My Portfolio
- Testing Open Graph Images
- Troubleshooting Common Issues
- Enhancing the Open Graph Experience
- Conclusion: Showcasing Your Work with Open Graph
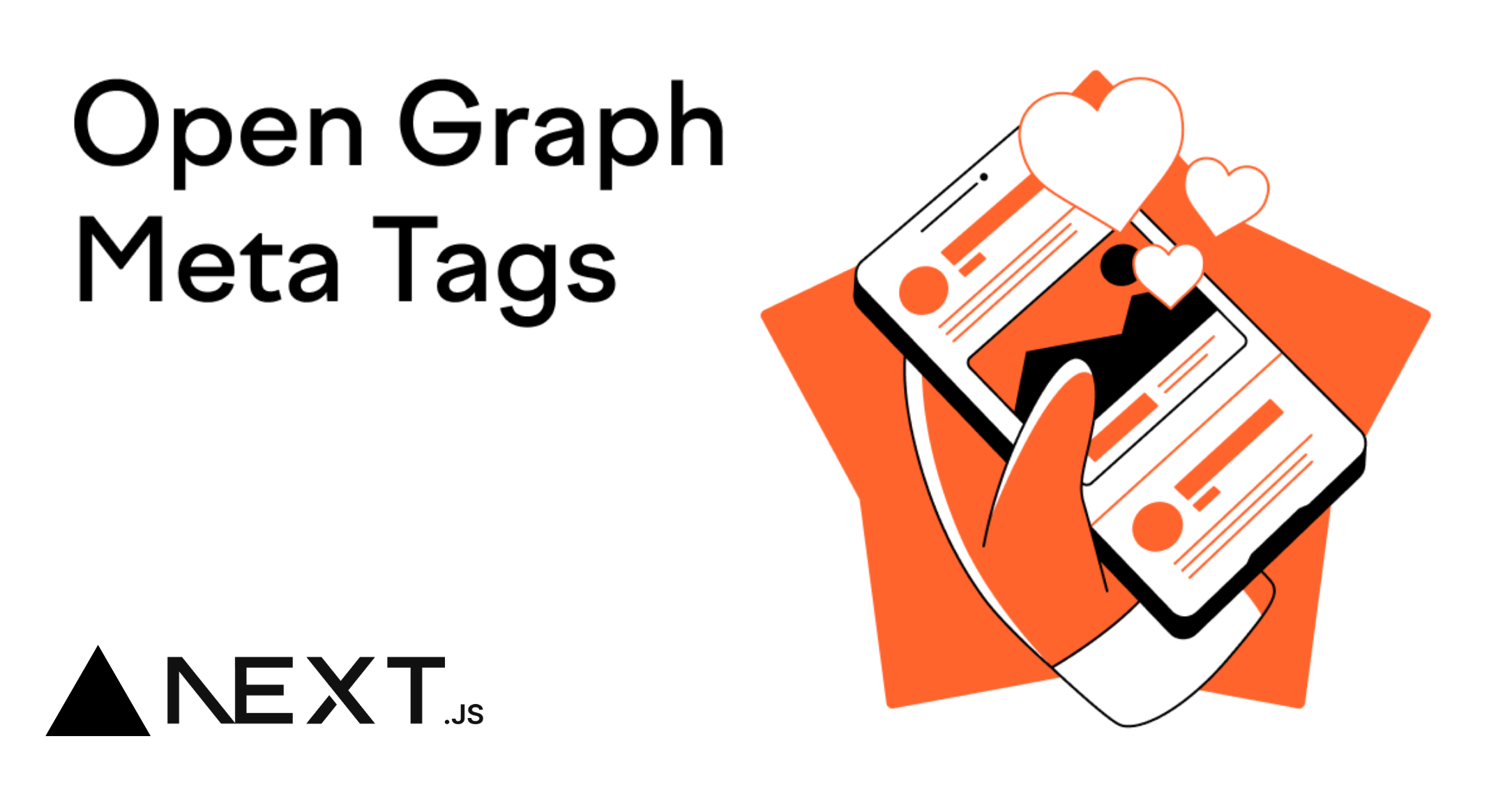
Introduction
In today's digital playground, having a social media presence is like having a megaphone for your awesomeness. One of the secret ingredients to making your content pop on platforms like Facebook, Twitter, LinkedIn and event WhatsApp is the Open Graph (OG) image. This little gem gives your posts a visual flair, making them way more shareable and clickable.
And let’s be honest, you can look pretty cool when you share your website with your friends, and it pops up with a slick image instead of just a bland link. 😎
In this blog, we're diving into how to effortlessly add Open Graph images to your Next.js app. We'll walk you through creating dynamic OG images that match your brand's vibe and make your social media game strong. Whether you're working on a personal project, a blog, or a business site, these images can seriously amp up your content's visibility.
Fallback: Implementing Open Graph Images in Plain HTML
Before we jump into Next.js wizardry, let's take a quick detour to understand how Open Graph images work in the good ol' static HTML world. You know, the kind of HTML that doesn’t make you feel like a wizard every time you hit refresh.
In the simplest form, Open Graph images are defined using the <meta>
tags within the <head>
section of your HTML document. Here’s a quick example of how to manually add an Open Graph image:
<head>
<meta property="og:title" content="Your Page Title" />
<meta property="og:description" content="A brief description of your content." />
<meta property="og:image" content="https://example.com/path/to/your/image.jpg" />
<meta property="og:url" content="https://example.com" />
</head>
Now, let's break it down:
og:title
: The title that will appear when the content is shared.og:description
: A short description of the page's content.og:image
: The URL of the image you want to show as the thumbnail on social media.og:url
: The canonical URL for the page.
In this example, the og:image
tag points to a specific image URL, which will be displayed when the page link is shared. You could use any valid image URL, whether it’s hosted on your server, an external CDN, or a cloud storage service. The key is to make sure that the image is publicly accessible.
That’s it! It’s like the cherry on top of your HTML sundae. When you share a link to your page on Facebook or Twitter, this metadata ensures your page shows up with a neat image and description instead of just a sad, boring link. 🍒
But while this works perfectly for static pages, once you dive into the dynamic, ever-changing world of Next.js, we’ll need to step things up a notch. Plus, if you want to fine-tune things for specific platforms like Twitter or LinkedIn, there’s a whole other layer of Open Graph fun to explore. But don’t worry, we’ll cover that in a bit!
Implementing Open Graph Images in Next.js
Now that we’ve got the static HTML basics covered, let’s take it to the next level with Next.js. With Next.js, you’re no longer dealing with plain, static pages. You’re working with dynamic content, and that means your Open Graph tags need to be more flexible, adapting to different pages and content.
To set up Open Graph metadata dynamically in Next.js, you’ll be working with the <Head>
component, which is a part of Next.js’s next/head
package. This allows you to modify the <head>
section of each page dynamically — pretty handy, right?
Why Next.js Makes OG Images More Awesome
Before we jump into the code (don't worry, we'll get there!), let's talk about why Next.js is like your social media's best friend:
Dynamic Generation: Your OG images can change based on content (magic, right?)
Built-in Optimization: Next.js comes with image optimization that's smoother than a fresh jar of skippy
Server-Side Rendering: Your OG tags are ready before social media platforms even peek at them
API Routes: Create custom OG images on the fly (like a pizza, but for your links)
To set up Open Graph metadata dynamically in Next.js, you’ll be working with the <Head>
component, which is a part of Next.js’s next/head
package. This allows you to modify the <head>
section of each page dynamically — pretty handy, right?
Here’s an example of how you can include Open Graph tags in your Next.js pages:
import Head from 'next/head';
export default function HomePage() {
return (
<>
<Head>
<meta property="og:title" content="Awesome Next.js Page" />
<meta property="og:description" content="This is the best Next.js page ever!" />
<meta property="og:image" content="https://example.com/path/to/your/dynamic-image.jpg" />
<meta property="og:url" content="https://yourwebsite.com" />
</Head>
<div>
<h1>Welcome to the Awesome Next.js Page</h1>
</div>
</>
);
}
What’s happening here:
<Head>
: This is the key to adding meta tags to your Next.js pages. It allows you to inject any HTML element, including<meta>
, into the head of your page.Dynamic Content: The cool part of Next.js is that you can make these tags dynamic based on the content of the page. So if you’re displaying different products, blog posts, or anything unique, you can tailor the
og:title
,og:description
, andog:image
for each page.
Dynamic Open Graph Image
For a more advanced touch, let’s say you want to generate an Open Graph image that is unique to each page, like a personalized greeting or product image. In Next.js, you can use a server-side solution (such as a serverless function or an external API) to generate these images on the fly.
This is super helpful when you don’t want to manually upload and link a new image for every page — instead, you generate it dynamically!
This is how you can start implementing Open Graph metadata in a Next.js app. But we’re just scratching the surface. As mentioned earlier, if you want to target specific platforms (like Twitter, LinkedIn, etc.), there are some additional tweaks and tags you’ll need to add, which we’ll explore next.
Diving Deeper: Platform-Specific Open Graph Implementation
So far, we’ve set up the basics with Open Graph images in Next.js, but here’s where things get interesting. Different platforms like Facebook, Twitter, LinkedIn, and others each have their own quirks when it comes to how they display content. While Open Graph tags work across platforms, each one has its own set of meta tags that can fine-tune the way your content looks.
Here are some platform-specific docs reference:
Example: Open Graph Image in My Portfolio
To demonstrate the process, let's look at how I added an Open Graph image to my portfolio website. For the image, I used Canva (which is super easy and fun, by the way) to create a banner that best represents my portfolio. Once I designed the image, I uploaded it to my Next.js project and placed it in the public
folder.
Why the public
folder?
Good question! If you want to include assets like images, styles, or scripts that need to be publicly accessible, they must be placed in the public
folder. This is where Next.js looks for static files that you can directly link to in your HTML. So, if you have an Open Graph image (or any other asset), make sure it’s in the public
folder.
Here’s how I added the Open Graph image to my portfolio code:
// src/app/layout.tsx
export default function RootLayout({
children,
}: Readonly<{
children: React.ReactNode;
}>) {
return (
<html lang="en" suppressHydrationWarning className="light">
<head>
<meta
name="description"
content="Pulkit - Developer. Portfolio and projects showcasing my skills and work in web development."
/>
<meta
name="keywords"
content="Pulkit, Developer, Web Development, React, Portfolio"
/>
<meta name="author" content="Pulkit" />
<meta name="robots" content="index, follow" />
{/* Open Graph Tags */}
<meta property="og:title" content="Pulkit - Developer" />
<meta
property="og:description"
content="Pulkit - Developer. Portfolio and projects showcasing my skills and work in web development."
/>
<meta property="og:image" content="https://devpulkit.in/banner.png" />
<meta property="og:url" content="https://devpulkit.in" />
<meta property="og:type" content="website" />
<meta property="og:site_name" content="Pulkit - Developer" />
{/* Twitter Cards */}
<meta name="twitter:card" content="summary_large_image" />
<meta name="twitter:title" content="Pulkit - Developer" />
<meta
name="twitter:description"
content="Pulkit - Developer. Portfolio and projects showcasing my skills and work in web development."
/>
<meta name="twitter:image" content="https://devpulkit.in/banner.png" />
<meta name="twitter:site" content="@devpulkitt" />
{/* Canonical Tag */}
<link rel="canonical" href="https://devpulkit.in" />
{/* Facebook Tag */}
<meta property="og:url" content="https://devpulkit.in" />
<meta property="og:type" content="website" />
<meta property="og:title" content="Pulkit - Developer" />
<meta
property="og:description"
content="Pulkit - Developer. Portfolio and projects showcasing my skills and work in web development."
/>
<meta property="og:image" content="https://devpulkit.in/banner.png" />
{/* Title Tag */}
<title>Pulkit - Developer</title>
<link
rel="icon"
href="/icon?<generated>"
type="image/<generated>"
sizes="<generated>"
/>
</head>
<body>
......
</body>
</html>
);
}
In the code above, you can see how I’ve added the Open Graph tags using the <head>
tag in Next.js. The image URL, https://devpulkit.in/banner.png
, points directly to the image placed in the public
folder of my project. This image will be shown when someone shares my portfolio on social media.
Image Storage: As I mentioned, the
banner.png
file is stored in thepublic
folder of my Next.js project. This ensures that it’s publicly accessible and can be fetched without any issues.OG Tags: I’ve added the standard Open Graph tags like
og:title
,og:description
, andog:image
to specify the title, description, and image that should appear when the site is shared.
You can check out the full implementation on GitHub.
Testing Open Graph Images
Once you've added the Open Graph image and related meta tags to your project, it's time to test if everything works as expected. Fortunately, both Facebook and Twitter offer tools to preview how your Open Graph image will look when shared.
Facebook Sharing Debugger
Facebook provides a Sharing Debugger tool that allows you to test how your content will appear when shared. Simply enter the URL of your page, and it will fetch the metadata, including the Open Graph image. If there’s an issue, it’ll also show any errors in the metadata.Twitter Card Validator
Similarly, Twitter has its own Card Validator tool. You can paste your URL into the tool to see how the card (including the Open Graph image) will appear when shared on Twitter. It helps verify that your image, description, and title are correctly displayed.Browser Testing
You can also test how your Open Graph image appears by simply sharing the link in various messaging platforms or in the address bar of a new tab (sometimes social media platforms automatically fetch the metadata when the link is pasted).
After testing, if everything looks good, congratulations! You're ready to share your site with the world, and your Open Graph image will make sure it looks sleek when shared on social media.
Troubleshooting Common Issues
It’s not uncommon to run into a few hiccups when setting up Open Graph images. Here are some things to look out for:
Image Not Showing Up:
If the Open Graph image isn’t showing up on social media platforms, make sure the image is publicly accessible (i.e., it's in thepublic
folder in Next.js). Also, check the image size – social platforms often have specific size recommendations. For Facebook, a 1200x630px image works well.Caching Issues:
Both Facebook and Twitter cache your Open Graph data. If you change the image or other meta information, you might need to refresh their cache. Use the aforementioned Debugger and Validator tools to clear cached data.Incorrect Image Dimensions:
If the image is too small or the aspect ratio doesn’t fit, it could appear stretched or squished. Always refer to platform-specific documentation (like Facebook or Twitter) for image dimension guidelines.
Enhancing the Open Graph Experience
While we’ve covered the basics of Open Graph images, you can also enhance the experience by including:
Custom Open Graph Images for Different Pages:
You might want to set a unique Open Graph image for different pages of your website (e.g., one for the homepage, one for the portfolio page, etc.). This can help make your links even more engaging when shared on social media.More Platform-Specific Tags:
As we mentioned earlier, you can add tags specific to platforms like Twitter, LinkedIn, or Pinterest. These can control things like card types or the way images are cropped. By diving deeper into the documentation, you can unlock even more customization options.
Conclusion: Showcasing Your Work with Open Graph
By using Open Graph, you're not just adding a simple image to your site. You’re creating a more engaging experience for anyone who shares or links to your website. Think of it like your website’s business card – but way cooler!
As a developer, learning how to work with Open Graph tags gives you more control over how your content is displayed on social media. Whether you’re sharing a blog post, portfolio, or a product page, Open Graph ensures that your content stands out and looks professional.
Now, take the time to refine your Open Graph setup to make sure your site shines when shared. By following these steps, you’re well on your way to mastering this essential aspect of SEO and social media sharing, ensuring your content always makes a great impression.
And remember, in the digital world, first impressions matter. So, go ahead and make your content shine like a superstar! 🌟
Subscribe to my newsletter
Read articles from Pulkit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pulkit
Pulkit
Hi there! 👋 I'm Pulkit, a passionate web and app developer specializing in MERN and React Native technologies. With a keen interest in open-source contributions and competitive programming, I love building innovative solutions and sharing my knowledge with the community. Follow along for insights, tutorials, and updates on my coding journey!