Comprehensive guide to learning Hangfire in .NET Core
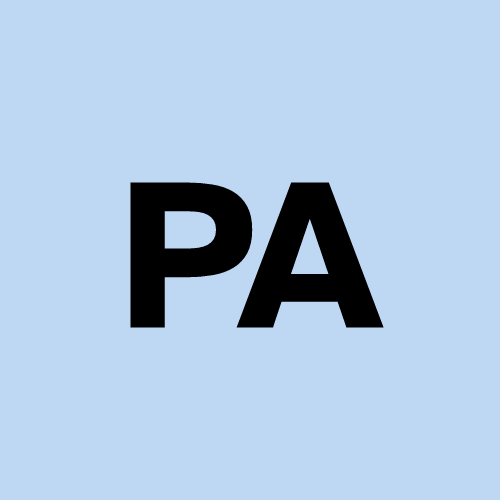
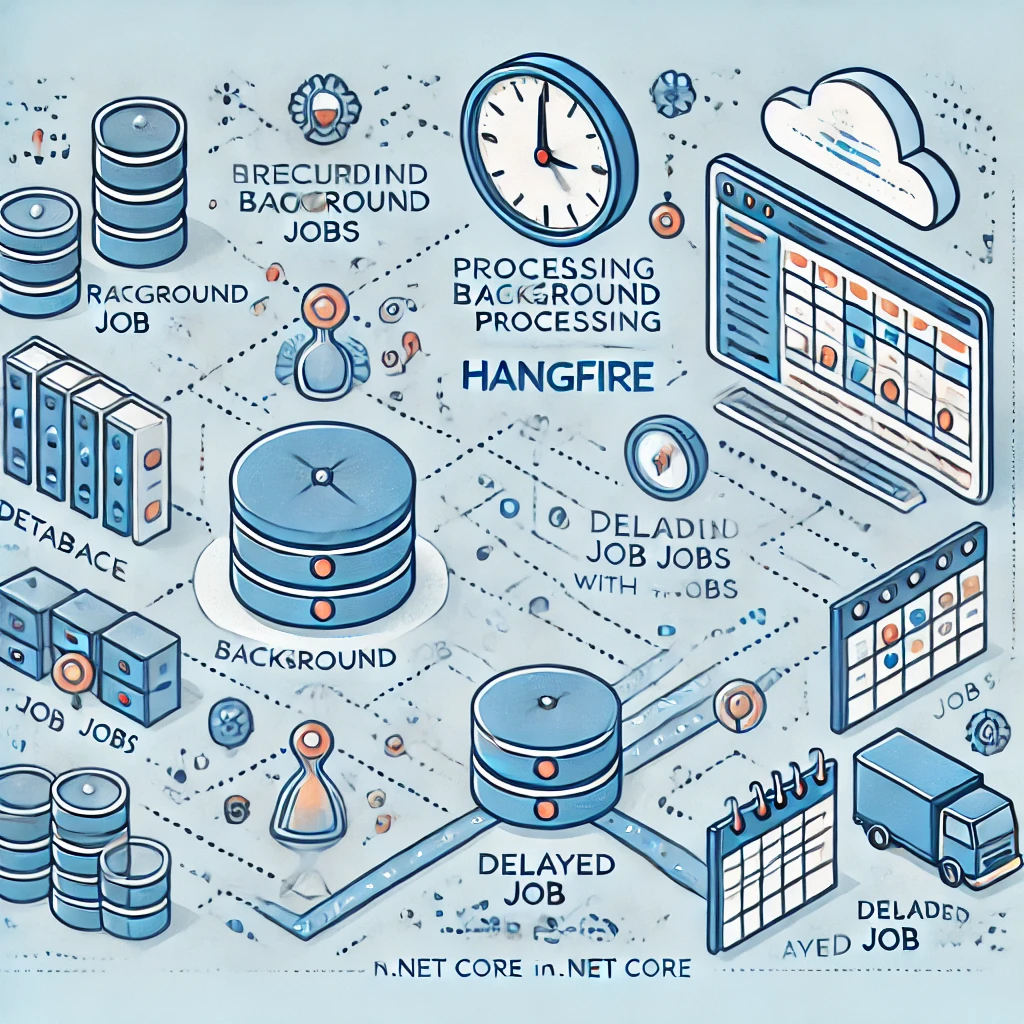
In today’s fast-paced digital world, smooth and efficient background processing can make or break the performance of your applications. Enter Hangfire, the powerhouse library for handling background jobs in .NET Core. Whether you’re building a high-load web application or automating routine tasks, Hangfire provides a simple yet powerful way to offload work, improve scalability, and boost performance.
In this guide, we’ll unravel Hangfire’s core concepts, explore its features with hands-on examples, and equip you with best practices to ensure your applications run seamlessly. Ready to take your .NET Core skills to the next level? Let’s dive in!
Hangfire is a robust library for managing background jobs in .NET applications. It allows developers to schedule and manage tasks outside the main request-response lifecycle, enhancing performance and enabling asynchronous job processing. Below is a comprehensive guide to learning Hangfire in .NET Core, including foundational concepts, best practices, and references to official documentation.
What is Hangfire?
Hangfire is an open-source library that simplifies the creation, scheduling, and execution of background jobs. It supports:
Fire-and-forget jobs: Execute a task once after the current request completes.
Recurring jobs: Execute tasks on a schedule (e.g., cron expressions).
Delayed jobs: Execute a task at a specified future time.
Continuations: Execute a job only after a parent job completes successfully.
Core Features
Built-in Dashboard for monitoring and managing jobs.
Persistent storage options (SQL Server, Redis, etc.).
Easy-to-use, fluent API.
Support for distributed processing and multiple servers.
Setup Hangfire in .NET Core
1. Install Hangfire
Add Hangfire to your project using NuGet:
dotnet add package Hangfire
dotnet add package Hangfire.AspNetCore
2. Configure Hangfire in Program.cs
In .NET Core, configure Hangfire during application startup:
using Hangfire;
using Hangfire.SqlServer;
var builder = WebApplication.CreateBuilder(args);
// Add Hangfire services
builder.Services.AddHangfire(config =>
config.SetDataCompatibilityLevel(CompatibilityLevel.Version_170)
.UseSimpleAssemblyNameTypeSerializer()
.UseDefaultTypeSerializer()
.UseSqlServerStorage(builder.Configuration.GetConnectionString("HangfireConnection")));
builder.Services.AddHangfireServer();
var app = builder.Build();
// Configure Hangfire dashboard
app.UseHangfireDashboard();
// Add a sample recurring job
RecurringJob.AddOrUpdate(() => Console.WriteLine("Recurring Job Executed!"), Cron.Daily);
app.Run();
3. Database Setup
Ensure you have a connection string for Hangfire in your appsettings.json
:
"ConnectionStrings": {
"HangfireConnection": "Server=.;Database=HangfireDb;Trusted_Connection=True;"
}
Run the app to let Hangfire create the necessary database schema automatically.
Creating Background Jobs
1. Fire-and-Forget Jobs
Used for tasks that need to run once, immediately after the request ends.
BackgroundJob.Enqueue(() => Console.WriteLine("Fire-and-forget job executed!"));
2. Delayed Jobs
Used for tasks scheduled to execute in the future.
BackgroundJob.Schedule(() => Console.WriteLine("Delayed job executed!"), TimeSpan.FromMinutes(30));
3. Recurring Jobs
Run tasks at regular intervals, defined by cron expressions.
RecurringJob.AddOrUpdate("daily-job",
() => Console.WriteLine("Recurring job executed!"),
Cron.Daily);
4. Continuations
Run tasks after a parent job completes.
var jobId = BackgroundJob.Enqueue(() => Console.WriteLine("Parent job executed!"));
BackgroundJob.ContinueWith(jobId, () => Console.WriteLine("Continuation job executed!"));
Dashboard
The Hangfire Dashboard provides a user-friendly interface to monitor jobs, retry failed tasks, and inspect logs. Enable it by calling:
app.UseHangfireDashboard();
Access it at /hangfire
(e.g., http://localhost:5000/hangfire
).
Best Practices
Use Persistent Storage Wisely:
Choose storage (SQL Server, Redis) that aligns with your scalability and reliability requirements.
Clean up old jobs to prevent database bloat.
Secure the Dashboard:
Protect the dashboard with authentication/authorization middleware to prevent unauthorized access.
app.UseHangfireDashboard("/hangfire", new DashboardOptions { Authorization = new[] { new MyAuthorizationFilter() } });
Optimize Job Retries:
Configure retry policies to handle transient errors gracefully.
GlobalJobFilters.Filters.Add(new AutomaticRetryAttribute { Attempts = 3 });
Avoid Heavy Tasks in Jobs:
- Use smaller, lightweight tasks for better performance and scalability.
Monitor Job Performance:
- Use Hangfire metrics and logs to identify bottlenecks and optimize.
Distributed Processing:
- Deploy multiple Hangfire servers for better throughput in high-load environments.
By understanding the core principles and adhering to best practices, you can efficiently use Hangfire to manage background jobs in .NET Core applications. Let me know in the comment section if you'd like a hands-on example.
Thank you for taking the time to explore the world of Hangfire with me! Whether you’re a seasoned .NET developer or just starting with background job processing, I hope this guide has been insightful and empowers you to harness Hangfire's capabilities effectively.
If you found this blog valuable, don’t forget to share it with your colleagues and fellow developers—it might just be the resource they need! Let’s grow and learn together; feel free to leave your thoughts, feedback, or questions in the comments below. Your engagement helps us all become better at what we do. 🚀
Together, let’s build efficient, scalable, and reliable .NET applications! 💻✨
Subscribe to my newsletter
Read articles from Pragyanshu Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
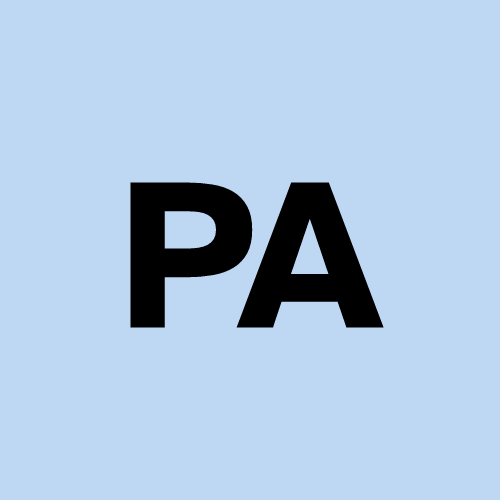