Avoiding Performance Pitfalls in SPFx Development: How to Keep Your SharePoint Customizations Fast and Efficient 🚀
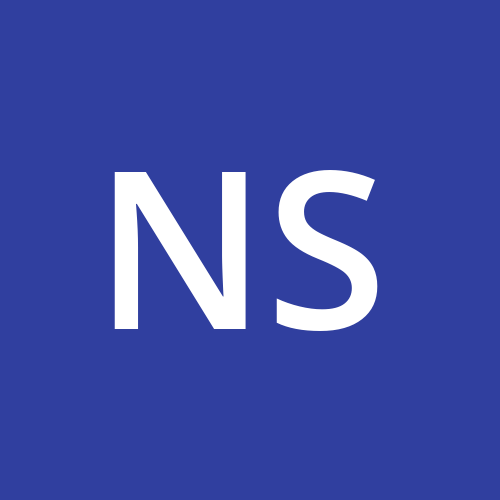
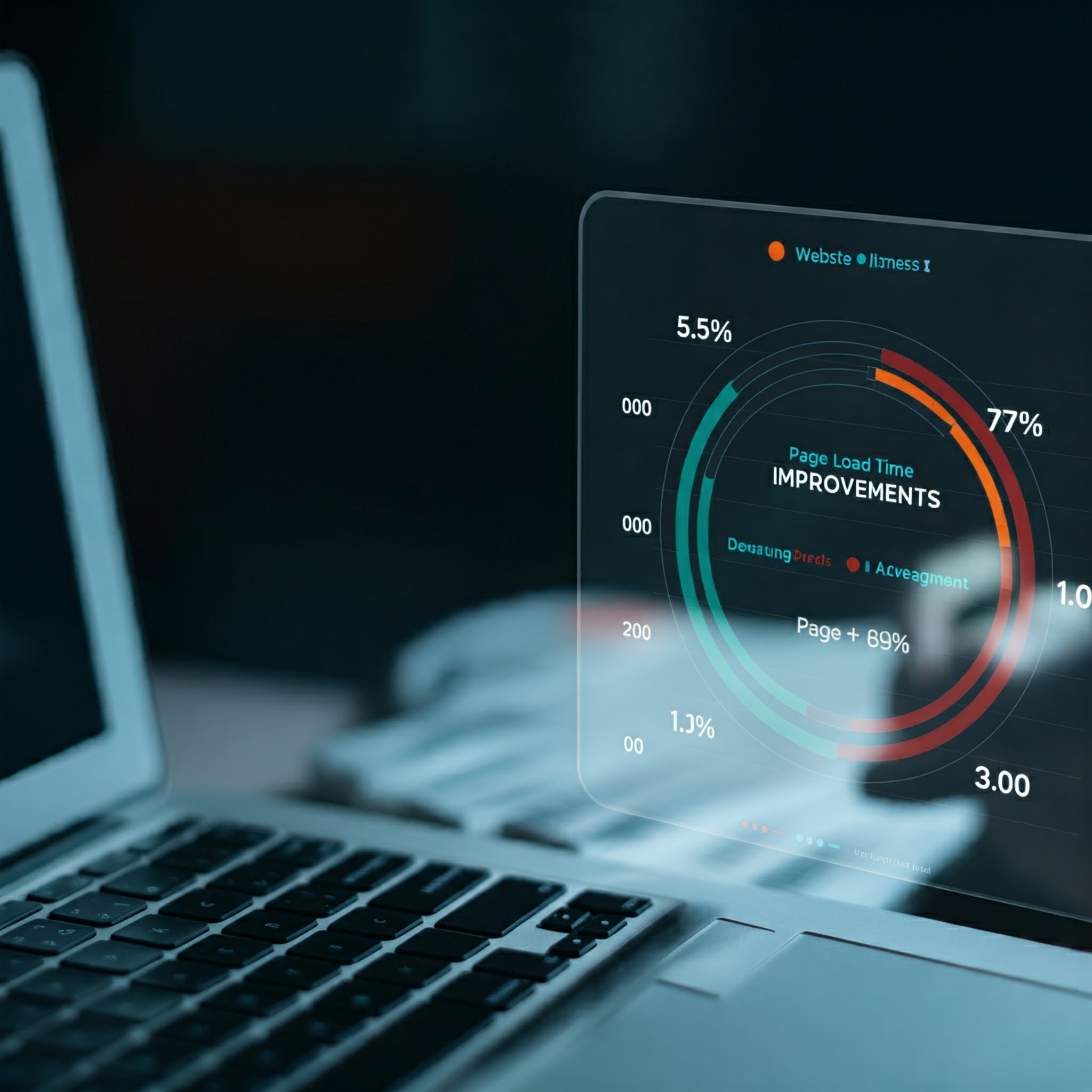
Performance is critical for user experience in SharePoint Framework (SPFx) applications, where slow load times can lead to user frustration and reduced productivity. For developers customizing SharePoint, managing performance is an ongoing challenge. In this post, we’ll dive into the common pitfalls that lead to bloated SPFx components and share best practices to optimize performance effectively.
Why Performance Matters in SPFx Customization
When SPFx solutions grow in complexity, it’s easy for bundle sizes to increase and load times to suffer. Large bundle sizes and unoptimized resources can lead to long waits, creating friction that hinders productivity and engagement. A slow site not only impacts the user experience but can also lower adoption rates within organizations that rely on SharePoint for daily tasks.
Large Bundle Size
In SPFx, every component you add contributes to the overall size of your solution bundle. As a bundle grows, load times increase, affecting users’ ability to access information quickly. Often, the culprit is the inclusion of heavy libraries or the absence of lazy loading strategies, causing unnecessary code to load on the initial page load.
Best Practices for Optimizing Bundle Sizes
Lazy Loading Components and Libraries
Why: Loading components and libraries only when they are needed reduces initial load times.
How: Use
React.lazy
orimport()
for conditional loading of components and libraries. For example, load a complex reporting component only when the user navigates to the Reports section.Using External Dependencies Effectively
Why: SPFx natively includes common libraries like
React
andReact-DOM
, so bundling them within your code is redundant and increases bundle size.How: Reference these external libraries directly from SPFx instead of importing them. In your project’s
config.json
, define popular libraries as external dependencies to keep them out of your bundled code.
Analyze and Optimize Bundle Size with Webpack Tools
Why: Understanding which components and libraries contribute the most to your bundle size is key to targeted optimization.
How: Use Webpack Bundle Analyzer to visualize and analyze the size of your components and dependencies. This can help identify areas to optimize, like replacing large libraries or rethinking how assets are structured. To analyze bundle sizes in an SPFx project, you can use the Webpack Bundle Analyzer with Gulp. Here’s a step-by-step guide to set it up and use it to gain insights into what’s contributing to your bundle size:
Setting Up Webpack Bundle Analyzer in Your SPFx Project
- Install Webpack Bundle Analyzer
First, navigate to your SPFx project directory and install Webpack Bundle Analyzer as a development dependency:
- Install Webpack Bundle Analyzer
npm install --save-dev webpack-bundle-analyzer
Update Gulpfile.js
Next, you’ll need to modify the gulpfile.js
in your project to integrate Webpack Bundle Analyzer. This configuration adds a custom Gulp task that will use Webpack Bundle Analyzer to display a visual representation of your bundle.
Open gulpfile.js
and add the following code:
'use strict';
const gulp = require('gulp');
const build = require('@microsoft/sp-build-web');
const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');
// Create a custom task to analyze the bundle
build.configureWebpack.mergeConfig({
additionalConfiguration: (generatedConfiguration) => {
if (!generatedConfiguration.plugins) {
generatedConfiguration.plugins = [];
}
// Add the Bundle Analyzer Plugin
generatedConfiguration.plugins.push(new BundleAnalyzerPlugin({
analyzerMode: 'static', // Generates an HTML file with the report
reportFilename: 'bundle-report.html', // Output file
openAnalyzer: true, // Automatically open the report after build
}));
return generatedConfiguration;
}
});
module.exports = build.initialize(gulp);
Explanation:
analyzerMode: 'static'
: This creates a standaloneHTML
file with the bundle report, which is more accessible for reviewing and sharing.reportFilename: 'bundle-report.html'
: Specifies the output file name for the report.openAnalyzer: true
: Automatically opens the report in the default browser once the build completes.Run the Analyzer
To generate the bundle analysis report, use the following Gulp command:gulp bundle --ship
By regularly running Webpack Bundle Analyzer, you can keep a close watch on your bundle size and make optimizations as your project evolves
moment.js
, which can significantly increase bundle size, consider using a lighter alternative like date-fns
. This simple change can drastically reduce the amount of JavaScript loaded by your solution.Subscribe to my newsletter
Read articles from Nitesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
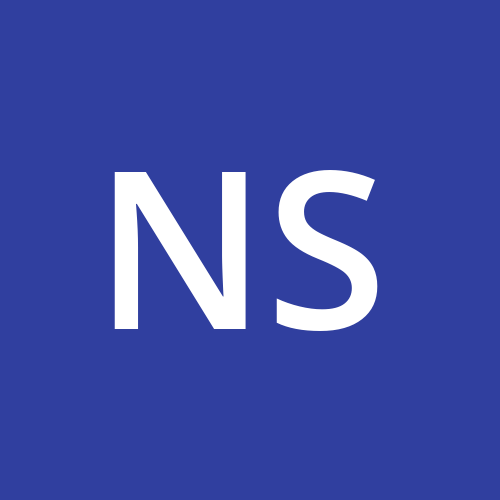
Nitesh Singh
Nitesh Singh
M365 Full Stack Developer - Experience in Azure, SharePoint SPFX, .NET, and React Full Stack Development, Driving Innovation and Digital Transformation Across Diverse Industries