Nested Routing in React JS
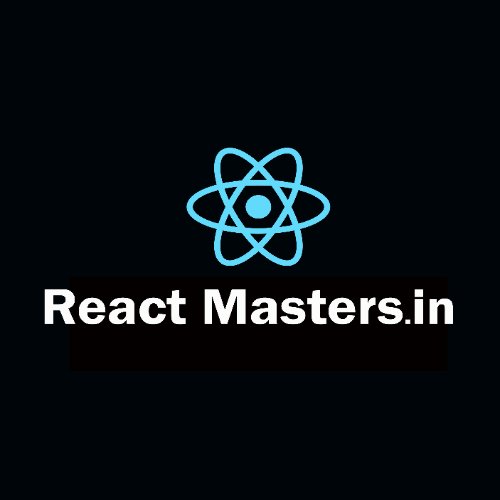
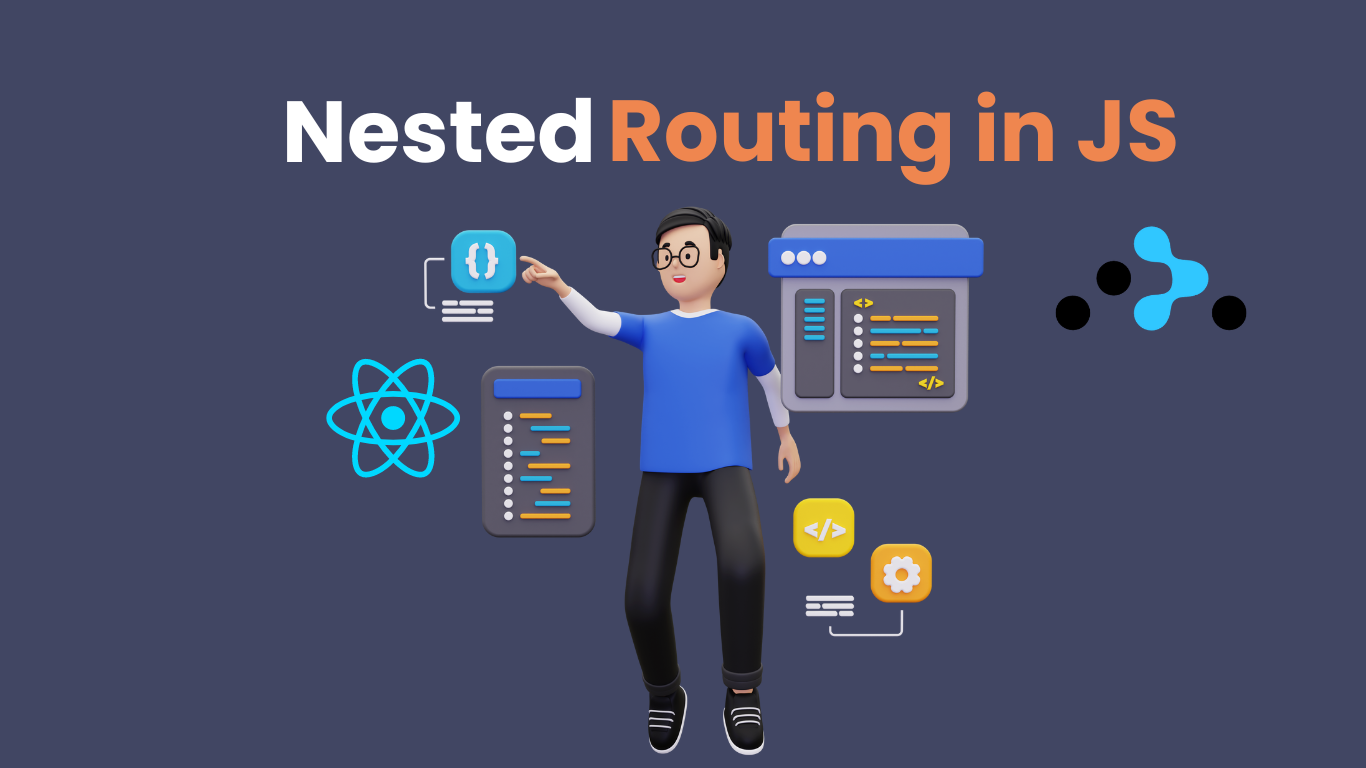
Nested routing is a powerful feature in modern web applications, enabling the development of complex UI hierarchies while maintaining modularity and clarity in the codebase. In React JS, libraries such as React Router are widely used for routing implementation.
While Hansnode is not a core library in the React ecosystem, it is a concept or utility (depending on the context) that can complement nested routing in a React project.
This article explains the role of nested routing in React JS and highlights how Hansnode can enhance its capabilities.
What is Nested Routing in React.js?
Nested routing allows developers to define routes within routes, enabling the creation of UI components that display different layouts or functionality based on the route hierarchy. For example, a blog application might have the following route structure:
/blog
- Displays the list of all blogs./blog/:id
- Displays a specific blog post./blog/:id/comments
- Displays comments for the specific blog post.
React Router makes it easy to implement such structures using components like <Routes>
, <Route>
, and <Outlet>
.
Role of Hansnode in Nested Routing
If you're working with Hansnode
as part of your nested routing strategy in React, it's likely a custom solution or middleware designed to streamline routing-related operations. Here's how Hansnode might fit into this context:
Dynamic Route Resolution: Hansnode can dynamically resolve nested routes, making it easier to handle complex route trees.
Data Management: With nested routing, components often rely on data passed down through routes. Hansnode could serve as a data-fetching layer, ensuring components in nested routes receive the necessary data.
Reusable Components: It may enable reusable route definitions by abstracting repetitive route logic into a simpler configuration format.
Enhanced State Synchronization: If your application requires syncing state with route changes, Hansnode can act as a bridge, reducing boilerplate code.
Implementing Nested Routing with React Router
To understand how Hansnode might integrate with React, let’s first look at how nested routing is typically implemented:
Install React Router:
npm install react-router-dom
Define Nested Routes:
import { BrowserRouter, Routes, Route } from 'react-router-dom';
import Blog from './pages/Blog';
import BlogPost from './pages/BlogPost';
import Comments from './pages/Comments';
function App() {
return (
<BrowserRouter>
<Routes>
<Route path="blog" element={<Blog />}>
<Route path=":id" element={<BlogPost />}>
<Route path="comments" element={<Comments />} />
</Route>
</Route>
</Routes>
</BrowserRouter>
);
}
export default App;
- Use
<Outlet>
for Nested Rendering: Inside theBlogPost
component, use the<Outlet>
to render the nestedComments
component.
import { Outlet } from 'react-router-dom';
const BlogPost = () => {
return (
<div>
<h1>Blog Post</h1>
<Outlet /> {/* Nested routes will render here */}
</div>
);
};
export default BlogPost;
Integrating Hansnode
To integrate Hansnode into the nested routing structure:
Setup Hansnode for Dynamic Routes: If Hansnode enables dynamic route configurations, use it to generate or load routes.
Data Loading with Hansnode: Integrate Hansnode’s APIs to fetch data for components in nested routes. For example:
import { useParams } from 'react-router-dom';
import hansnode from 'hansnode';
const BlogPost = () => {
const { id } = useParams();
const [post, setPost] = React.useState(null);
React.useEffect(() => {
hansnode.fetch(`/api/posts/${id}`).then(setPost);
}, [id]);
return post ? <div>{post.title}</div> : <p>Loading...</p>;
};
export default BlogPost;
- Route Middleware: Hansnode might act as middleware to validate or transform route data before rendering the components.
Benefits of Using Hansnode in Nested Routing
Simplified Configuration: Makes complex routing structures more manageable.
Dynamic Updates: Allows changes in route definitions without hardcoding.
Improved Data Flow: Ensures seamless data fetching and synchronization with routes.
Conclusion
Nested routing in React JS is essential for building feature-rich web applications, and tools like Hansnode can simplify route management and enhance functionality.
While Hansnode might not be a widely recognized library, its principles can inspire solutions tailored to your project’s needs.
Integrating Hansnode effectively requires understanding its capabilities and aligning them with your application's routing architecture.
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
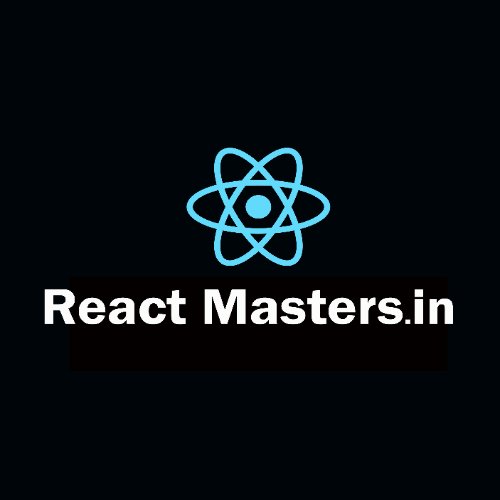
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.