Deploy a MERN Backend on Vercel for Free
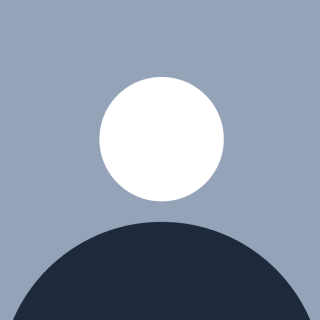
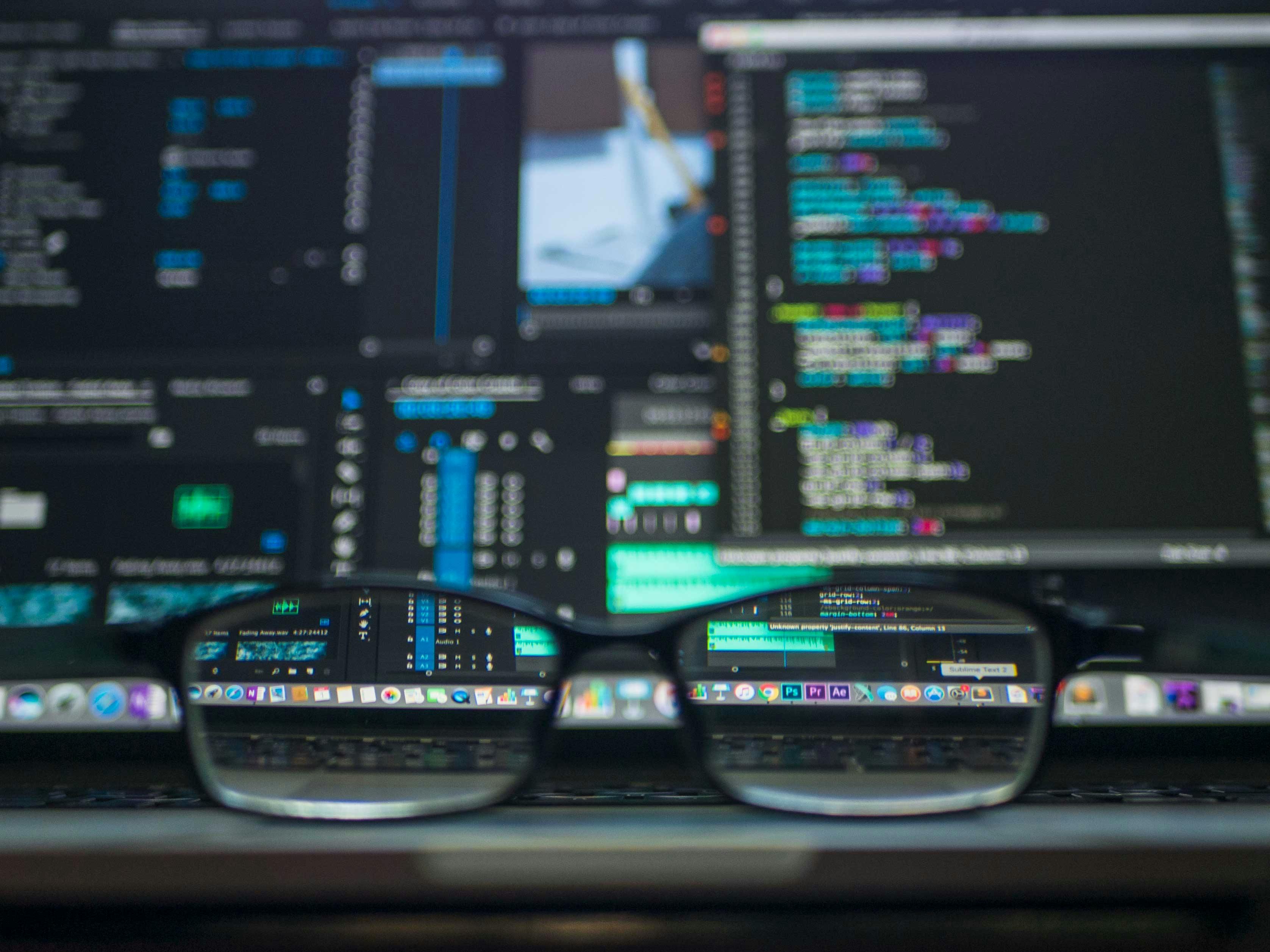
Learning to deploy your backend is an essential part of becoming a full-stack developer. In this tutorial, we’ll create a simple Express API and deploy it to Vercel step-by-step.
Step 1: Create a Simple Express API
Let’s start by setting up a basic Node.js API.
Initialize a Node.js Project
Run the following commands to create a new project:mkdir simple-api cd simple-api npm init -y
Install Express
Install Express to handle HTTP requests:npm install express
Create the API
Create a file namedindex.js
and add the following code:const express = require("express"); const app = express(); // Simple API endpoint app.get("/", (req, res) => { res.send({ message: "Hello, Vercel!" }); }); // Start the server (for local testing) const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
Test your API locally by running:
node index.js
Open
http://localhost:3000
in your browser to confirm it’s working.
Step 2: Prepare for Deployment
Add a
vercel.json
File
In the root folder, create avercel.json
file:{ "version": 2, "builds": [{ "src": "index.js", "use": "@vercel/node" }], "routes": [{ "src": "/(.*)", "dest": "/" }] }
Push Code to GitHub
Push your project to a GitHub repository using these commands:git init git add . git commit -m "Initial commit" git branch -M main git remote add origin <your-repo-url> git push -u origin main
Step 3: Deploy on Vercel
Log in to Vercel
Go to vercel.com and log in.Import Your Project
Click "Import Project."
Select your GitHub repository.
Configure environment variables if needed.
Deploy
Click "Deploy," and Vercel will build and host your project.
Step 4: Test Your API
Once deployed, you’ll get a URL like https://your-project-name.vercel.app
.
Access your API by visiting:
https://your-project-name.vercel.app/
You should see:
{ "message": "Hello, Vercel!" }
Why Vercel?
Deploying with Vercel is fast, free, and beginner-friendly. It allows you to learn real-world deployment practices with minimal setup.
Go ahead and deploy your first API today! 🚀
Subscribe to my newsletter
Read articles from Naved Ahmed khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Naved Ahmed khan
Naved Ahmed khan
Hello, I'm Naved—a seasoned Full Stack MERN Developer with expertise in crafting seamless, scalable web and mobile solutions.