HackerRank Bugs, a Rejection, and Building a Resume Parser
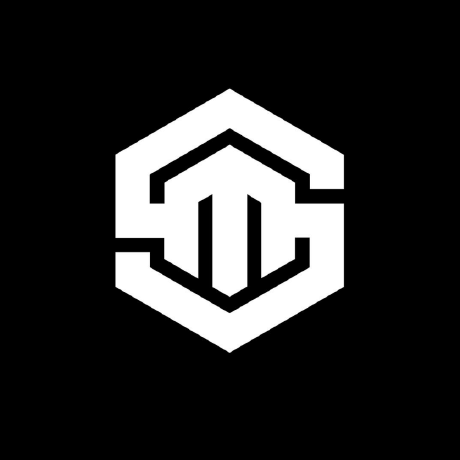
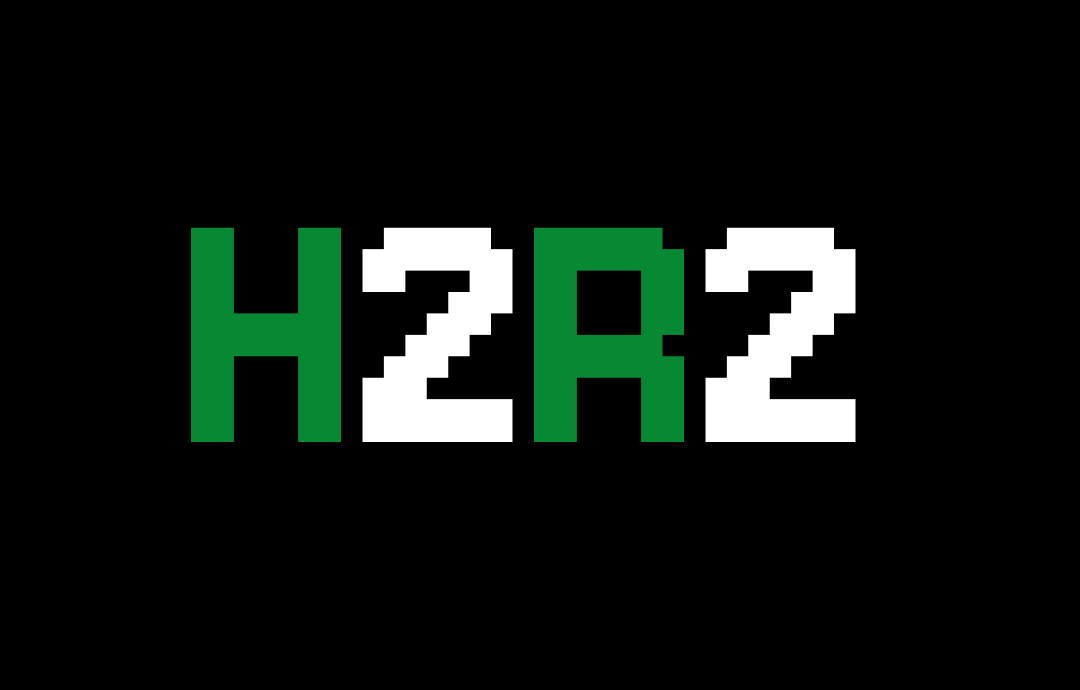
Recently, I came across a job posting for HackerRank's SDE Intern role, and I was really excited to apply. One of my senior had previously worked at HackerRank, and from all the articles I had read, it was clear that the company had an amazing work culture. While applying for the role, I came across a blog that caught my attention it was Summer Internship Experience '21 written by Ishan Bhaiya. It resonated with me deeply, as Ishaan Bhaiya’s situation was strikingly similar to mine. Much like Ishaan Bhaiya, I too began to dream of seeing my name on that TV screen.
During my application process, I was introduced to HackerRank's resume builder also coincidentally built by Ishan Bhaiya during his internship. As I used the platform, I encountered a couple of bugs that were quite frustrating. These bugs not only highlighted areas for improvement but also inspired me to build my own tools to enhance the experience. Interestingly, I felt a sense of excitement when I discovered these issues. Why? Because just like Ishaan Bhaiya, who was noticed by the HackerRank team after mailing them about a custom HTTP header, I felt like I too could contribute something valuable to the platform for them to notice me.
The bugs →
The GitHub Import Pagination Bug
The first issue I stumbled upon was related to the GitHub import feature in the resume builder. When navigating through the pagination of repositories, I noticed that despite clicking on the "Next" button, the API request URL remained the same, preventing any new data from loading.
For example, the request URL stayed as:
https://www.hackerrank.com/resume/api/github/repositories/xxxxxxxx?limit=14&page=1
No matter what button I clicked, the same API was called, which resulted in the inability to load further repositories.
Solution:
Resolving this, is quite easy we just need to ensure the pagination logic includes proper query parameter updates when the "Next" button is clicked. Specifically, the page parameter should increment with each subsequent request to ensure that the correct repositories for the next page are fetched. Additionally, logging and error handling on the client side should also be added to alert users when such issues arise, improving user experience.
The CORS Issue
I initially attempted to report this bug through HackerRank's support page, but I faced another issue one of the nightmares of new devs — CORS (Cross-Origin Resource Sharing) errors. I couldn't submit my bug report because my request to the support page was blocked due to CORS policy restrictions. Here's the exact error I encountered:
Access to XMLHttpRequest at 'https://metrics.hackerrank.com/app_metrics' from origin 'https://www.hackerrank.com' has been blocked by CORS policy.
At this point, I felt stuck, as I was unable to report the issue effectively.
Solution:
Well this one also has a very easy solution it would be to allow CORS requests from authorized domains.
Resume Matcher Bugs
Further investigation revealed another bug in the resume matcher tool. When I tried to match my resume to the SDE intern job listing on HackerRank's site, I noticed that the system made multiple backend requests, all resulting in 404 errors. After several failed attempts, it displayed a "no data found" message, unlike for other job links where the data was fetched after a few retries. The issue here appeared to be that if there are no Requirements listed on the page that is being parsed the requests just errors out.
Solution:
Well the easy solution is to return a more meaningful error message something like “No skill requirements found on the page“. The slightly costlier solution is to maybe use a LLM model to ingest the page’s data and compare it against the user’s resume to generate the matching result. (One of the things I want to implement in H2R2).
The Placeholder URL Bug
I encountered an issue with the GitHub project link placeholder in the HackerRank resume builder. The builder suggests entering a GitHub URL like github.com/Username/project
, but when used, it generates a link like:
https://www.hackerrank.com/resume/builder/github.com/AvaterClasher/zexd-backend
This happens because the <a>
tag treats the entered URL as a relative URL and appends it to the base URL of the platform (https://www.hackerrank.com/resume/builder
). This causes the URL to break, leading to a 404 page. Once the resume is downloaded, the URL is recognized correctly as an external link, but the issue occurs while using the builder.
Solution:
The placeholder should be updated to prompt users to enter the full, absolute URL with the https://
protocol, like https://github.com/Username/project
. This will prevent the builder from mistakenly treating it as a relative path and ensure correct redirection in the preview.
The Overflow problem? (Recently found)
While exploring how HackerRank handles overflow in its resume preview and splits content across pages, I discovered an interesting behavior regarding line breaks. If a user types a very long word without spaces, it simply overflows the container instead of breaking properly. I understand this is not a bug. However, I thought it was worth pointing out in case the team wants to address it—or even better, let me work on fixing it during my internship!
Reporting the Bugs
Unable to reach the HackerRank support team directly, I decided to explore other channels like email and LinkedIn. Through this, I connected with Apoorve Bhaiya, an SDE2 at HackerRank. He appreciated my efforts in reporting the bugs, and mentioned that he would take the issues to the team to get them resolved as soon as possible. However, shortly after our conversation, I received an email informing me that I had not been selected.
This setback only motivated me further. I asked myself: “Why should HackerRank hire me? How can I improve their product?”
That’s when I identified a significant pain point in their Resume Builder: the lack of an import button for recurring information, like a candidate’s name, education, and other constant details. Determined to address this, I set out to build a solution.
Building Nresume:
A Resume Parser for HackerResume 2.0
Inspired by the bugs I encountered, I created nresume — a resume parser designed to extract structured information from resume PDFs, such as job titles, companies, dates, and descriptions. This tool aims to fill the gap in HackerRank’s Resume Builder, making it more efficient for users.
To test nresume, I quickly built a prototype website called H2R2 at h2r2.vercel.app using Next.js—all in a single night. While the site has rough edges due to its rapid development, it provided a functional environment to showcase nresume’s capabilities.
I wanted a solution that didn't rely on a backend, as that would mean either extracting text from the PDF on the frontend and sending it to the server or uploading the file to S3 and then parsing it in the backend-side. My thought was, why not handle the entire parsing process on the frontend? That’s when I found pdfjs, a library by Mozilla that allows text extraction directly in the browser.
My second challenge was that I’d never built a JavaScript package before, which opened up a whole new world of learning about bundling and packaging. Then came another big roadblock: deploying it on Vercel. I expected a smooth React package integration, but instead, I encountered multiple issues during deployment. After a lot of reading, searching for fixes, and diving into discussions on react-pdf issues, I finally got it working (p.s. — I do write meaningful commit messages, this one’s just for fun, no judgments!).
Nresume is designed to work within the browser, making it flexible for various environments. It uses a combination of regular expressions and feature scoring to identify the most relevant information from resumes.
However, the parser has some limitations:
It currently only supports single-column resumes.
It does not yet handle project sections, as they often vary too much in structure to process reliably.
Future Upgrades →
Resume Preview and Download:
Working out how to properly display the Resume’s preview and download the same without breaking the layout. Currently the layout breaks.
Named Entity Recognition (NER):
Use AI models to identify and classify key entities such as job titles, skills, certifications, and companies, making the parser more context-aware.
Customizable LLM Queries:
Allow users to define their own prompts, models, and keys to generate personalized resume insights or summaries using LLMs like GPT.
Semantic Search for Resumes:
Implement AI models to enable semantic matching of resumes against job descriptions, identifying the best-fit candidates for specific roles.
Resume Optimization Recommendations:
Add AI-powered suggestions to reword or optimize bullet points, ensuring they align with ATS (Applicant Tracking System) best practices.
Conclusion
Building nresume has been one of the most rewarding projects I’ve undertaken. The idea was born out of the frustrations I experienced while using HackerRank’s resume builder. Encountering multiple bugs was certainly challenging, but those challenges ultimately inspired me to create my own solution to the problem.
Is nresume perfect? Not at all. Does it need significant improvements? Absolutely. So, why am I publishing it now? With HackerRank’s internships starting in January and my end-semester exams lasting until November 25th, this is my best opportunity to showcase my work before it’s too late.
If any HackerRank team member happens to read this, I’d love the chance to prove my skills—be it through an interview or even a take-home assignment. I’m confident that my abilities align with what HackerRank is looking for, and I’m eager to demonstrate my potential!Thank you for reading!
Also attaching my resume just in case :) →
Subscribe to my newsletter
Read articles from Soumyadip Moni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
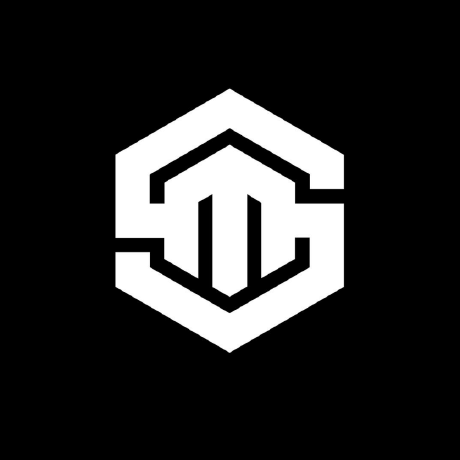
Soumyadip Moni
Soumyadip Moni
👋 Hey, I'm Soumyadip Moni, a fullstack engineer with a passion for web development. Currently, I'm diving into Go, Rust, and WebAssembly to create high-performance web apps. My tech toolbox includes JavaScript, React, Node.js, Go, Rust, and more. Join me on my coding journey here . Let's build a better digital future together !