Garbage Collection: How It Works and Why It Matters
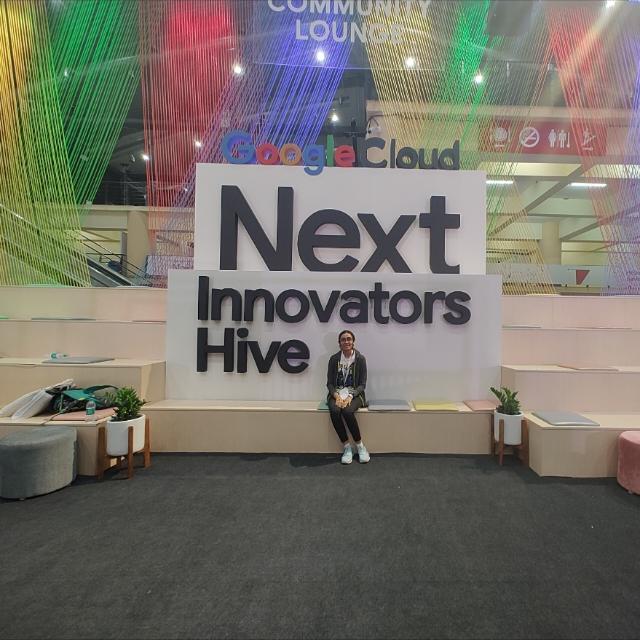
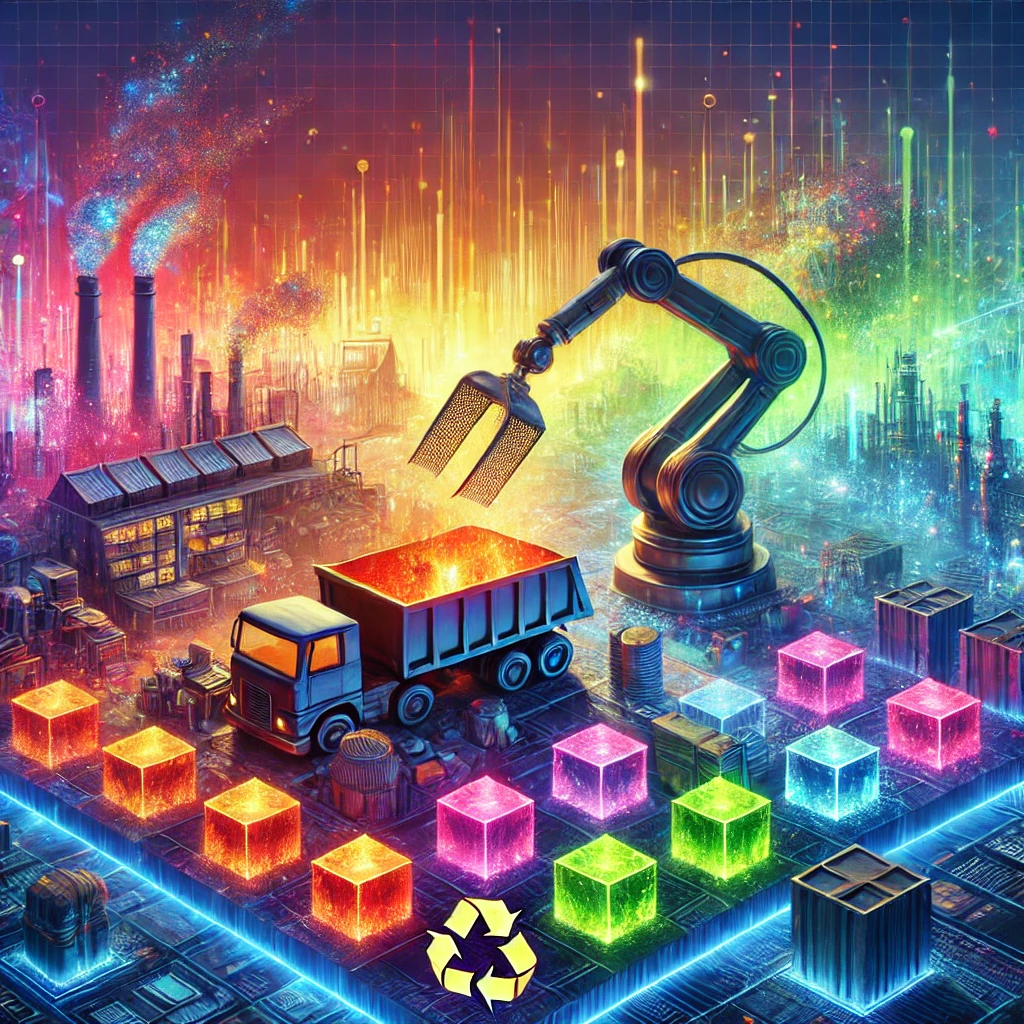
Garbage collection is one of the really important processes of programming languages as it collects vacant memory quite efficiently with the help of automatic memory reclamation. If not for this, developers would have to manually allocate as well as deallocate memory, which might increase the chances of occurrence of memory leaks and even crashes. In this blog, we will dive into the concept of garbage collection and understand the different kinds, along with how numerous programming languages implement it.
What is Garbage Collection?
Garbage collection is a process to identify and reclaim memory no longer used by a program. This allows the system to reuse memory to optimize resource utilization and prevent memory-related problems.
Why is Garbage Collection Important?
Prevents Memory Leaks: The unused objects automatically get cleaned.
Simplifies Development: Developers do not have to venture into details about the manual memory management.
Improves Stability: It limits the chances of crashes due to the errors caused in memory. Garbage Collection Techniques Reference Counting It keeps track of references to an object. When the count goes zero, de-allocate that object.
Backward: Cannot appropriately handle cyclic reference Mark-and-Sweep Marks objects which are accessible and sweeps away those unreferenced. It occurs in two phases: Mark phase and Sweep phase.
Generational GC Divide the memory into generations (young, old, permanent). The majority of objects live for a lesser time and collected early to improve performance Concurrent GC Runs parallel to the application and reduces pause times. Pervasive in real-time systems. Copying GC
It copies reachable objects into new memory space and then reclaims the old one. This makes it effective for young-generation object management.
Mark Down and Sweep:
The Mark phase identifies and marks all the in-use objects, starting from some set of "root" references, such as global variables, stack variables, or static fields, and traversing the object graph to find all reachable objects. Each discovered object is "marked", indicating that it is active and should not be reclaimed. All objects which are still reachable by the program remain in memory during this phase. However, this might insert some pauses in the execution of programs, at least in medium to big applications, because it has to trace all live references .
It further sweeps through the memory allocated to objects not in use anymore. After marking phase, garbage collector scans through the heap identifying the objects which had not been reached, marked as reachable. The objects are therefore now identified as garbage, and thus removed from the memory creating room for future allocation. This phase, though efficient, often causes memory fragmentation as it does not necessarily compact the memory layout, which can be filled with holes between active objects. More advanced garbage collectors will often add a compact phase after sweeping.
Garbage Collection in Java vs Python:
Python | Java |
Combines Reference Counting and Cyclic Garbage Collection. | Uses Generational Garbage Collection with Mark-and-Sweep, Compact, and other strategies. |
Automatic and explicit via the gc module (e.g., gc.collect()). | Automatic or explicit via System.gc() (though explicit calls are discouraged). |
Reference counting for immediate cleanup; Cyclic GC for circular references. | Typically Mark-and-Sweep combined with Generational GC for efficiency. |
Managed by Python's heap allocator and the built-in garbage collector. | Managed by the JVM (Java Virtual Machine). |
Special cyclic garbage collector to detect and resolve reference cycles. | Handles cycles naturally through graph traversal during the Mark phase. |
Partial control via the gc module for enabling/disabling GC or tuning thresholds. | Limited control; JVM decides when to run GC, though tunable via JVM options (-xxflags). |
Garbage collection, often the unsung hero of memory management, plays a pivotal role in ensuring the smooth operation of modern programming languages.
Subscribe to my newsletter
Read articles from Laasya Kandukuri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
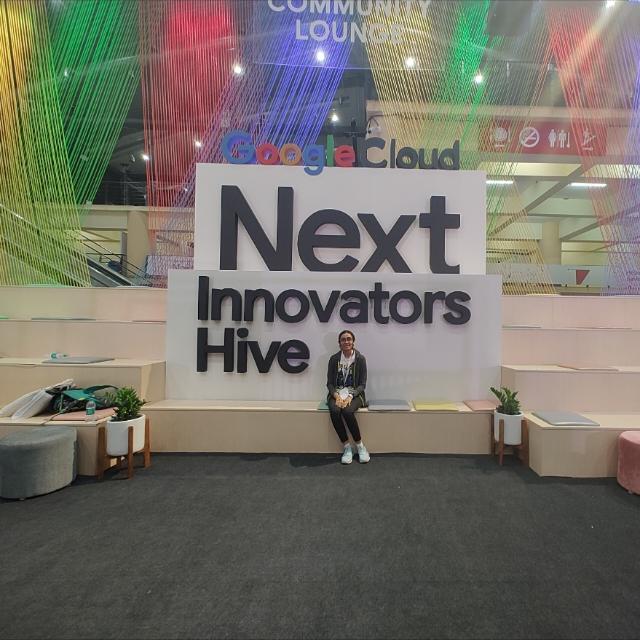
Laasya Kandukuri
Laasya Kandukuri
Hello, I'm Laasya, a Computer Science Engineering graduate passionate about Java development. Skilled in building efficient backend systems and solving complex problems through object-oriented programming. Eager to explore new challenges in software development and backend technologies.