Object-Oriented Programming (OOP) in Java

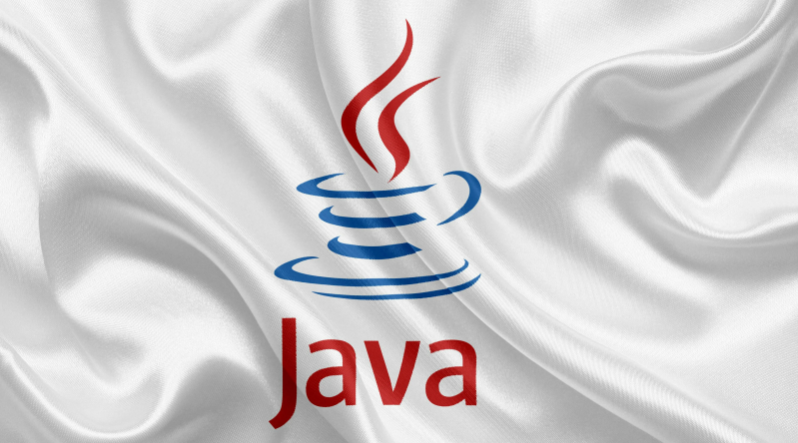
Object-Oriented Programming (OOP) is a programming paradigm centered around the concept of "objects," which represent real-world entities and data. Java, as a fully object-oriented language, leverages OOP principles to help developers build modular, reusable, and organized code. Here, we’ll explore the core concepts of OOP in Java—encapsulation, inheritance, polymorphism, and abstraction—and discuss their importance in modern software development.
Here’s a brief description of each of the topics related to Object-Oriented Programming in Java:
Classes and Objects
A class is a blueprint for creating objects, defining the properties and behaviors that the objects will have. Objects are instances of classes that represent real-world entities in programming. Classes provide structure, and objects use this structure to store data and perform actions defined by the class.
Constructors
Constructors are special methods in a class used to initialize objects when they are created. A constructor has the same name as the class and does not have a return type. It is called automatically when an object is instantiated, allowing the programmer to set initial values for object properties.
this
and super
Keywords
The this
keyword refers to the current instance of a class and is commonly used to distinguish between instance variables and parameters in a constructor or method. The super
keyword refers to the superclass (parent class) and allows access to its methods and constructors. It is often used in inheritance to invoke a superclass method or constructor.
Inheritance and Types of Inheritance
Inheritance is a core OOP concept allowing a class (subclass) to inherit properties and methods from another class (superclass). Java supports single inheritance (a class inherits from one superclass) and multi-level inheritance (a class inherits from a subclass that is itself a subclass of another class). However, Java does not support multiple inheritance (inheriting from more than one class), though it can be achieved using interfaces.
Method Overloading and Overriding
Method Overloading: This is when multiple methods in the same class have the same name but different parameters. It allows methods to perform similar operations with different types or numbers of parameters.
Method Overriding: This is when a subclass provides a specific implementation of a method already defined in its superclass. Overriding is key to polymorphism, as it allows subclass objects to use subclass-specific behavior when invoked through a superclass reference.
Encapsulation and Access Modifiers
Encapsulation is the practice of bundling data (fields) and methods that operate on the data into a single unit, or class, and restricting access to them from outside. Java’s access modifiers (private
, protected
, public
, and package-private) control the visibility of class members, ensuring that data is secure and that internal class details are hidden from other classes.
Polymorphism (Dynamic Method Dispatch)
Polymorphism enables objects to be treated as instances of their parent class rather than their actual class. Dynamic method dispatch is the process Java uses to select which method to call at runtime, based on the object’s actual type, not the reference type. This enables flexible and reusable code, as a single interface can be used with various underlying implementations.
Abstract Classes and Interfaces
Abstract Classes: These are classes that cannot be instantiated and are intended to be subclassed. They can include abstract methods (without implementations) and concrete methods (with implementations).
Interfaces: An interface defines a contract for classes to follow. It only contains method declarations (prior to Java 8) and constants. Interfaces allow Java to support a form of multiple inheritance, as a class can implement multiple interfaces.
Composition vs Inheritance
Composition and inheritance are two ways of establishing relationships between classes:
Composition: A design principle where a class is composed of one or more objects from other classes to reuse functionality.
Inheritance: A design principle where a class derives properties and behaviors from another class, establishing an “is-a” relationship.
Composition is often preferred over inheritance as it allows for more flexibility and less tightly coupled code.
Inner Classes and Anonymous Classes
Inner Classes: These are classes defined within another class. They can access the outer class’s fields and methods, which helps logically group related classes.
Anonymous Classes: These are a type of inner class with no name, created on the fly for one-time use. Anonymous classes are often used for quick implementation of interfaces or abstract classes.
Object Cloning
Object cloning is the process of creating a duplicate of an existing object. Java provides a clone()
method in the Object
class for this purpose. The Cloneable
interface must be implemented for an object to be cloned. Cloning is often used to create a copy of an object without going through the construction process.
Enums in Java
Enums (Enumerations) in Java are a special data type that defines a collection of constants. Enums are often used to represent a fixed set of related values, like days of the week or months of the year. They provide a type-safe way to handle a collection of related constants and can include fields, methods, and constructors, making them more versatile than basic constants.
Why OOP is Important in Java Development
OOP principles are foundational to Java and crucial for effective software development. Here’s why OOP matters:
Modularity: OOP allows developers to break down complex programs into simpler, manageable parts or modules (classes). This makes the codebase easier to understand and debug.
Reusability: By enabling code reuse through inheritance and polymorphism, OOP reduces redundancy, saving time and effort in development.
Maintainability: OOP’s structure and design principles make code more maintainable. Code changes can be made in one part of the program without needing to rewrite the entire codebase.
Scalability: OOP facilitates scalability, allowing developers to extend existing code smoothly. This is critical for growing projects or applications that may need to adapt to new requirements.
Improved Collaboration: OOP is particularly beneficial in team environments, as it establishes a clear code structure. Developers can work on different classes independently without impacting the entire system.
In summary, Object-Oriented Programming is at the heart of Java’s design and is essential for creating organized, reusable, and efficient code. It mirrors real-world interactions, making code more intuitive, which ultimately enhances productivity and maintains software quality over time.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.