Classes and Objects in Java

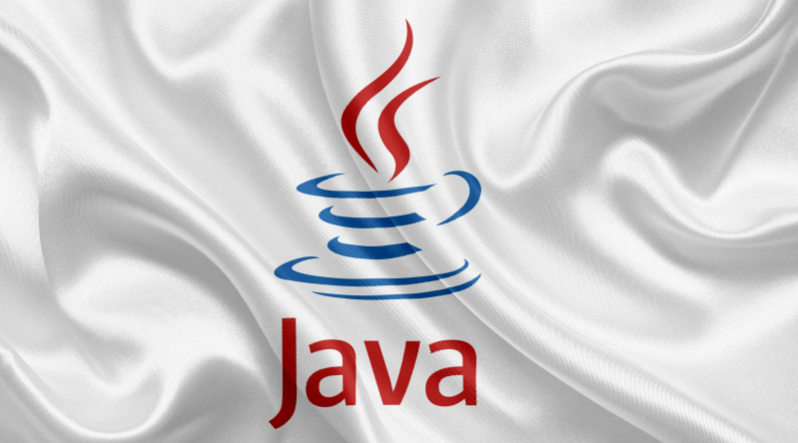
In Java, classes and objects form the core of object-oriented programming (OOP). These two concepts help organize code in a structured way that represents real-world entities and interactions. Let’s delve into what each term means and why they’re important.
What is a Class?
A class is a blueprint or template for creating objects. It defines the properties (data) and behaviors (methods) that the objects created from it can have. Think of a class as a recipe that specifies the ingredients and instructions to make a dish; in programming, it defines what an object will contain and what it can do.
Components of a Class
Fields (Attributes): These are variables defined within a class to store the object’s data. For example, in a
Car
class, fields might include properties likecolor
,model
, andyear
.Methods: These are functions within a class that define behaviors or actions that objects of the class can perform. For a
Car
class, methods might include actions likedrive()
orstop()
.
Example of a Simple Class
Here’s an example of a basic Java class called Car
:
public class Car {
String color;
String model;
int year;
public void drive() {
System.out.println("The car is driving.");
}
}
In this Car
class, color
, model
, and year
are fields, and drive()
is a method.
What is an Object?
An object is an instance of a class. When you create an object, you’re making a specific item based on the class blueprint, with actual values assigned to the properties defined in the class.
To create an object in Java, use the new
keyword, which allocates memory and initializes it as per the class structure.
Example of Creating an Object
Using the Car
class from above, let’s create an object:
public class Main {
public static void main(String[] args) {
Car myCar = new Car(); // Creating an object of Car class
myCar.color = "Red";
myCar.model = "Toyota";
myCar.year = 2021;
System.out.println("Color: " + myCar.color);
myCar.drive(); // Calling the method
}
}
Here, myCar
is an instance (object) of the Car
class. It has specific values for color
, model
, and year
and can perform actions defined by the Car
class, like drive()
.
Difference Between Classes and Objects
Class: A class is a template or blueprint that defines attributes and methods.
Object: An object is a concrete instance created from a class, with actual data values.
For example, Car
is a class, while myCar
is an object of that class, with specific attribute values assigned to it.
Why are Classes and Objects Important?
Encapsulation: Classes help group related data and methods together, creating self-contained code that is easier to manage and understand.
Modularity: Each class can represent a distinct component or entity in a program, promoting modularity and simplifying debugging.
Real-World Modeling: Classes and objects allow programmers to model real-world entities and scenarios, making programs more intuitive and realistic.
Code Reusability: Once a class is defined, you can create multiple objects from it without rewriting the class code.
Conclusion
Classes and objects are fundamental concepts in Java that provide the foundation for organizing and structuring code. Understanding how to use classes and create objects is essential for building robust, modular, and scalable Java applications.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.