Constructors in Java

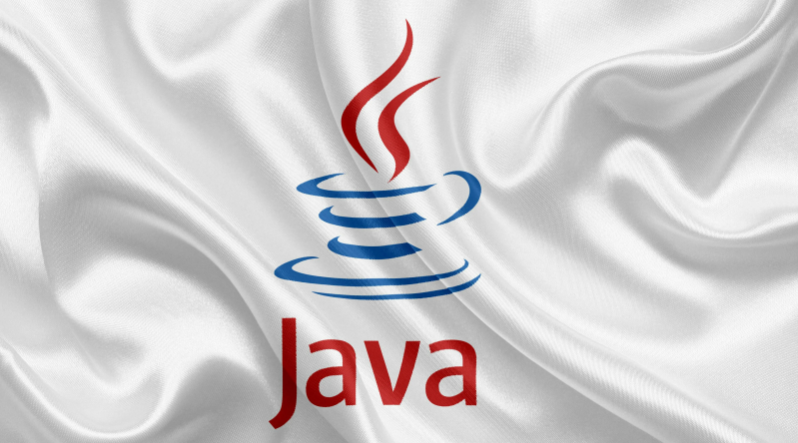
In Java, constructors are special methods used to initialize objects. When you create an object, the constructor is automatically called, setting up the object with specific values or default configurations. Constructors are essential for providing initial values to the object’s attributes and ensuring it’s ready to use from the start.
What is a Constructor?
A constructor in Java is a block of code similar to a method, but with some unique characteristics:
Same Name as the Class: A constructor must have the same name as the class in which it is defined.
No Return Type: Constructors do not have a return type, not even
void
.Called Automatically: When you create an object with the
new
keyword, the constructor is automatically invoked.
Purpose of a Constructor
The main purpose of a constructor is to initialize the object’s fields with specific values, which helps prevent uninitialized or invalid states in objects. Constructors often allow you to set values for an object’s attributes right when it’s created.
Types of Constructors
Java supports two primary types of constructors:
Default Constructor: A constructor with no parameters. If you don’t define any constructor, Java automatically provides a default constructor that initializes the object with default values.
Parameterized Constructor: A constructor that accepts parameters, allowing you to provide initial values for the object’s fields when you create the object.
Example of Constructors
Consider a Car
class with constructors:
public class Car {
String color;
String model;
int year;
// Default Constructor
public Car() {
color = "Black";
model = "Generic Model";
year = 2020;
}
// Parameterized Constructor
public Car(String color, String model, int year) {
this.color = color;
this.model = model;
this.year = year;
}
public void displayDetails() {
System.out.println("Car Color: " + color + ", Model: " + model + ", Year: " + year);
}
}
In this example:
The default constructor assigns generic values to
color
,model
, andyear
.The parameterized constructor allows you to specify
color
,model
, andyear
when creating an object.
Creating Objects with Constructors
Here’s how to create objects using the constructors defined in the Car
class:
public class Main {
public static void main(String[] args) {
// Using Default Constructor
Car defaultCar = new Car();
defaultCar.displayDetails(); // Outputs default values
// Using Parameterized Constructor
Car customCar = new Car("Red", "Toyota", 2022);
customCar.displayDetails(); // Outputs specified values
}
}
In this code:
defaultCar
is created using the default constructor, which assigns default values.customCar
is created using the parameterized constructor, which allows custom values.
this
Keyword in Constructors
In parameterized constructors, you often see the this
keyword. this
refers to the current instance of the object, helping distinguish between instance variables and parameters with the same name.
Example:
public Car(String color, String model, int year) {
this.color = color; // 'this.color' refers to the instance variable, 'color' refers to the parameter
this.model = model;
this.year = year;
}
Constructor Overloading
In Java, you can define multiple constructors in the same class, each with different parameter lists. This is known as constructor overloading. Overloading allows you to create objects in various ways by providing different sets of initial values.
Example:
public class Car {
String color;
String model;
int year;
// No-argument constructor
public Car() {
this("Black", "Generic Model", 2020); // Calls the parameterized constructor
}
// One-argument constructor
public Car(String color) {
this(color, "Generic Model", 2020);
}
// Full-parameter constructor
public Car(String color, String model, int year) {
this.color = color;
this.model = model;
this.year = year;
}
}
In this example:
The
Car
class has three constructors, each allowing different levels of customization.The
this
keyword can also be used to call another constructor within the same class, which is known as constructor chaining.
Importance of Constructors
Initialization: Constructors ensure that objects start in a valid state, with all necessary fields initialized.
Flexibility: Overloaded constructors provide multiple ways to create an object, allowing for flexibility and customization.
Data Integrity: By requiring essential fields to be set upon creation, constructors help maintain the consistency and integrity of the object’s data.
Conclusion
Constructors are vital components of Java programming, enabling structured and predictable object creation. By initializing objects right from the start, constructors ensure reliability and flexibility in how Java applications are built and maintained. Understanding constructors and how they function is a key skill for creating efficient, stable Java applications.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.