Inheritance and Types of Inheritance in Java

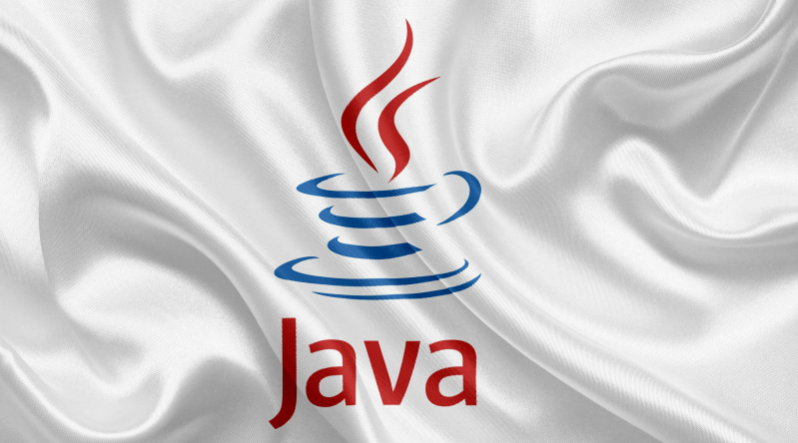
Inheritance is one of the core concepts of Object-Oriented Programming (OOP), and Java, being an object-oriented language, fully supports inheritance. Inheritance allows a class to inherit properties (fields) and methods from another class, promoting reusability and extensibility of code. This article will dive into the concept of inheritance in Java, explain its importance, and explore the different types of inheritance supported by the language.
What is Inheritance in Java?
In Java, inheritance enables a new class to derive properties and behaviors (methods) from an existing class. The class that inherits the properties is called the subclass (or child class), and the class from which the properties are inherited is the superclass (or parent class).
Key Benefits of Inheritance:
Code Reusability: Inheritance allows you to reuse the methods and variables of the superclass in the subclass, which minimizes code duplication.
Method Overriding: Subclasses can override superclass methods to provide specific implementations while keeping the same method signature.
Extensibility: Inheritance allows you to extend the functionality of existing classes without modifying them, making your code easier to maintain and scale.
Syntax of Inheritance in Java
To implement inheritance in Java, you use the extends
keyword, which establishes a relationship between a superclass and a subclass.
Example:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
In this example, the Dog
class inherits the sound()
method from the Animal
class but overrides it to make a specific sound.
Types of Inheritance in Java
Java supports several types of inheritance based on how classes are related to each other. Let’s discuss these types in detail:
1. Single Inheritance
Single inheritance refers to the scenario where a subclass inherits from a single superclass. This is the most common and straightforward type of inheritance.
Example:
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
Here, Dog
inherits from the Animal
class, gaining the eat()
method. In single inheritance, a subclass can inherit from only one parent class, maintaining simplicity and clarity.
2. Multilevel Inheritance
In multilevel inheritance, a class is derived from another derived class, creating a chain of inheritance. This allows multiple levels of classes to inherit from each other.
Example:
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
class Puppy extends Dog {
void sleep() {
System.out.println("Sleeping...");
}
}
In this case:
The
Puppy
class inherits fromDog
, which in turn inherits fromAnimal
.Puppy
can access both thebark()
method fromDog
and theeat()
method fromAnimal
.
3. Hierarchical Inheritance
Hierarchical inheritance occurs when multiple subclasses inherit from a single superclass. This type of inheritance allows multiple derived classes to share common functionality from the same parent class.
Example:
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
class Cat extends Animal {
void meow() {
System.out.println("Meowing...");
}
}
Here, both the Dog
and Cat
classes inherit from the Animal
class, meaning both classes can use the eat()
method from Animal
but also have their own unique methods (bark()
and meow()
).
4. Multiple Inheritance (Through Interfaces)
Java does not support multiple inheritance through classes, meaning a class cannot directly inherit from multiple classes. However, Java allows multiple inheritance through interfaces. A class can implement multiple interfaces, inheriting the abstract methods from each interface.
Example:
interface Animal {
void eat();
}
interface Bird {
void fly();
}
class Eagle implements Animal, Bird {
public void eat() {
System.out.println("Eagle eats");
}
public void fly() {
System.out.println("Eagle flies");
}
}
Here, the Eagle
class implements two interfaces, Animal
and Bird
. Even though Java doesn’t support multiple inheritance through classes, interfaces allow a class to inherit behavior from multiple sources.
5. Hybrid Inheritance (Combining Different Types)
Hybrid inheritance occurs when different types of inheritance are combined. This can involve multiple classes, interfaces, or both, although it can introduce some complexity. Java doesn't directly support hybrid inheritance, but a combination of interfaces and classes can mimic this type.
Example:
interface Animal {
void eat();
}
interface Walkable {
void walk();
}
class Dog implements Animal, Walkable {
public void eat() {
System.out.println("Dog eats");
}
public void walk() {
System.out.println("Dog walks");
}
}
In this case, the Dog
class implements both Animal
and Walkable
interfaces, inheriting the methods from both.
Importance of Inheritance in Java
Promotes Code Reusability: Inheritance allows developers to reuse code from existing classes rather than writing new code from scratch. This leads to less duplication and more maintainable code.
Enhances Flexibility: By allowing subclasses to modify or extend the behavior of superclass methods, inheritance increases the flexibility of the code.
Encourages the Use of Interfaces: In Java, multiple inheritance is achieved through interfaces. This encourages the use of interfaces, which is considered a best practice for defining abstract behavior in Java programs.
Simplifies Code Maintenance: Inheritance makes it easier to manage and maintain code by centralizing common logic in base classes. Changes in the base class automatically reflect in derived classes, making updates simpler.
Conclusion
Inheritance is a foundational concept in object-oriented programming, and Java provides several ways to implement it. From single inheritance to the use of interfaces for multiple inheritance, Java offers flexibility in how classes can relate to each other. Inheritance simplifies code reuse, increases flexibility, and encourages cleaner, more maintainable code. By understanding and applying inheritance in Java, developers can create more robust, modular, and efficient software systems.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.