Method Overloading and Overriding in Java

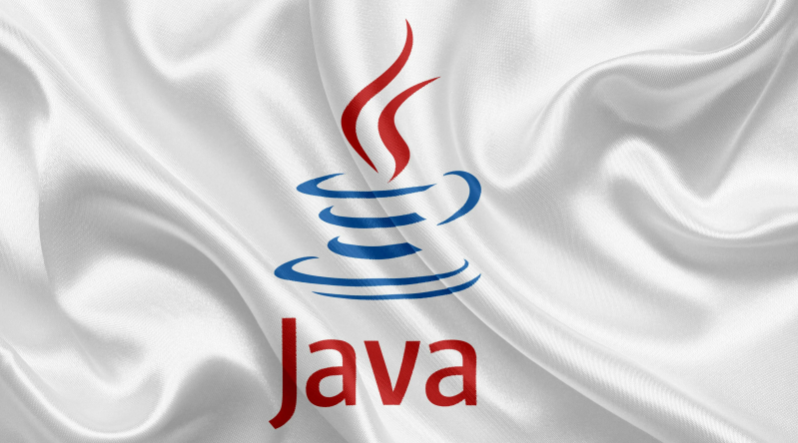
In Java, two important concepts related to methods are Method Overloading and Method Overriding. Both concepts allow developers to use the same method name, but they work in different contexts and serve different purposes. Understanding the distinction between these two can help developers write cleaner and more efficient code while enhancing flexibility in object-oriented programming.
Method Overloading in Java
Method Overloading is a concept where multiple methods within a class share the same name but have different parameters (either a different number of parameters or parameters of different types). It is a way to provide multiple implementations of the same method, allowing the same method name to perform similar but slightly different tasks depending on the parameters passed.
Key Points about Method Overloading:
Overloading happens within the same class.
Methods can have the same name, but they must differ in the number or type of parameters.
The return type of overloaded methods can be the same or different.
Overloading provides flexibility and improves the readability of the code, making it easier to handle different input scenarios.
Syntax of Method Overloading:
class Calculator {
// Method to add two integers
int add(int a, int b) {
return a + b;
}
// Overloaded method to add three integers
int add(int a, int b, int c) {
return a + b + c;
}
// Overloaded method to add two doubles
double add(double a, double b) {
return a + b;
}
}
In this example, the method add()
is overloaded three times with different parameter combinations:
add(int a, int b)
— adds two integers.add(int a, int b, int c)
— adds three integers.add(double a, double b)
— adds two double values.
By overloading methods, you avoid using multiple method names for similar operations, improving code readability and maintainability.
Why is Method Overloading Important?
Improved Code Clarity: By using the same method name for operations that are conceptually similar, code readability is improved.
Flexibility: Method overloading allows a class to handle different input types or numbers without requiring additional method names.
Code Reduction: Overloading eliminates the need for multiple method names for similar tasks, thus reducing the overall codebase size.
Method Overriding in Java
Method Overriding occurs when a subclass defines a method that has the same name and signature (method name, return type, and parameters) as a method in its superclass. The subclass method overrides the superclass method to provide a specific implementation. This is a key feature of polymorphism, as it allows an object of the subclass to invoke the overridden method.
Key Points about Method Overriding:
Method overriding happens between a superclass and a subclass.
The method signature in the subclass must be the same as the method signature in the superclass.
The @Override annotation is often used to indicate that a method is intended to override a superclass method (though it's optional).
In overriding, the return type must be the same or a subtype of the return type in the superclass (known as covariant return types).
Syntax of Method Overriding:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
// Overriding the sound() method of Animal
@Override
void sound() {
System.out.println("Dog barks");
}
}
In this example:
The
Dog
class overrides thesound()
method from theAnimal
class to provide a more specific implementation.When we create an object of the
Dog
class and callsound()
, it will print "Dog barks", not "Animal makes a sound".
Why is Method Overriding Important?
Polymorphism: Method overriding is essential for achieving runtime polymorphism, allowing the method to behave differently depending on the object (whether it's a superclass or subclass object).
Dynamic Method Dispatch: Overriding ensures that the method called is resolved at runtime, making it possible to invoke the subclass method when working with superclass references.
Specialization: It allows a subclass to provide a specific implementation of a method that is defined in the superclass, tailoring the behavior to its needs.
Key Differences Between Method Overloading and Method Overriding
Aspect | Method Overloading | Method Overriding |
Definition | Defining multiple methods with the same name but different parameters. | Defining a method in a subclass with the same signature as the method in the superclass. |
Type of Inheritance | Occurs within the same class. | Occurs between a superclass and subclass. |
Parameters | Must differ in the number or type of parameters. | Must have the same method signature (name, return type, parameters). |
Return Type | Can have the same or different return type. | Must have the same return type or a subtype (covariant return). |
Purpose | Provides multiple versions of the same method to handle different inputs. | Provides a specific implementation of a method inherited from a superclass. |
Polymorphism | Does not support polymorphism. | Supports runtime polymorphism. |
Method Resolution | Resolved at compile-time (static polymorphism). | Resolved at runtime (dynamic polymorphism). |
Conclusion
Both method overloading and method overriding are powerful features of Java that allow for flexibility, reusability, and extensibility in code. Overloading helps when you want to define methods with the same name but different input parameters, improving code clarity. On the other hand, overriding allows subclasses to define their specific behavior for inherited methods, supporting runtime polymorphism and enabling more dynamic and flexible software designs. By leveraging both overloading and overriding, developers can write efficient and maintainable object-oriented code that is adaptable to changing requirements.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.