Encapsulation and Access Modifiers in Java

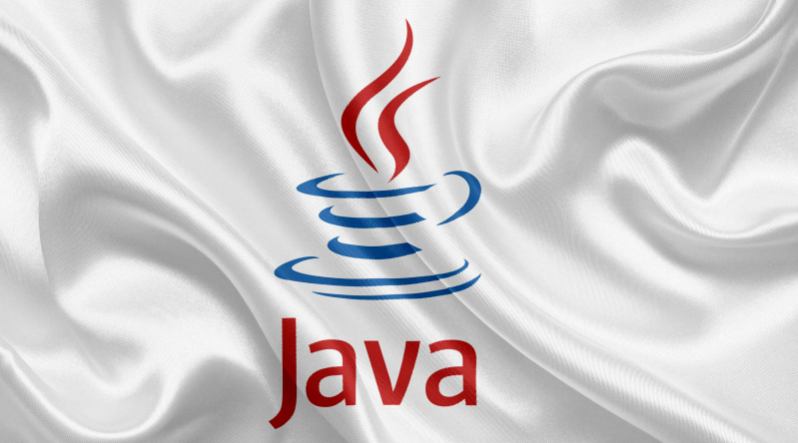
Encapsulation is one of the fundamental principles of Object-Oriented Programming (OOP), and it plays a vital role in ensuring the proper structure and organization of code. In Java, encapsulation is closely related to access modifiers, which define the visibility and accessibility of class members (fields, methods, constructors) from other parts of the program. This article will discuss encapsulation, how it is implemented in Java, and the different access modifiers used to control access to class members.
What is Encapsulation?
Encapsulation refers to the concept of bundling the data (variables) and methods that operate on the data into a single unit or class. It also involves restricting direct access to some of an object's components, which is why we often say "encapsulation hides the internal workings of an object." This is achieved by using access modifiers to control access to the fields and methods.
The main idea behind encapsulation is to protect the internal state of an object from unwanted or inappropriate modifications, ensuring that it can only be changed in controlled ways.
Benefits of Encapsulation:
Data Hiding: Internal details of the object are hidden from the outside world. The outside world interacts with the object only through its public methods (getters and setters), thus providing better security.
Code Maintainability: Changes to the internal implementation of a class can be made without affecting the code that uses the class, as long as the public interface (methods) remains the same.
Increased Flexibility and Control: Encapsulation allows the developer to modify the internal state of the object and control how it is accessed, improving control over the object’s behavior.
How to Achieve Encapsulation in Java?
In Java, encapsulation is typically implemented by making the fields (variables) of a class private and then providing public getter and setter methods to access and update these fields.
Example of Encapsulation:
class Employee {
// Private fields
private String name;
private int age;
private double salary;
// Getter method for name
public String getName() {
return name;
}
// Setter method for name
public void setName(String name) {
this.name = name;
}
// Getter method for age
public int getAge() {
return age;
}
// Setter method for age
public void setAge(int age) {
if (age > 18) { // Validation before setting age
this.age = age;
} else {
System.out.println("Age must be greater than 18.");
}
}
// Getter method for salary
public double getSalary() {
return salary;
}
// Setter method for salary
public void setSalary(double salary) {
this.salary = salary;
}
}
In this example:
The fields
name
,age
, andsalary
are declared asprivate
. This ensures that these fields cannot be directly accessed or modified by any other class.The public getter and setter methods allow controlled access to these fields. For example, the setter method for
age
includes validation to ensure that the age is greater than 18 before it is set.
By using this approach, the internal state of the Employee
class is protected, while still allowing other classes to interact with it through controlled methods.
Access Modifiers in Java
In Java, access modifiers define the level of access control for classes, methods, and variables. They determine where a member can be accessed from within the program. Java provides four types of access modifiers:
public
private
protected
default (no modifier)
Each access modifier has a different level of access control, from least restrictive to most restrictive.
1. public
The public
access modifier allows the widest level of access. Any other class, whether in the same package or a different package, can access the public members (fields, methods, etc.) of the class.
Public Access: Accessible from any other class.
Usage: Typically used for methods or variables that need to be accessed by any other class or outside package.
Example:
public class Car {
public String model;
}
In this case, the model
field can be accessed from any class, whether it's in the same package or a different package.
2. private
The private
access modifier restricts access to the member (fields, methods, etc.) only to the own class. No other class, even in the same package, can access the private members.
Private Access: Accessible only within the class it is declared in.
Usage: Typically used for fields and methods that should be hidden from other classes to maintain encapsulation and data security.
Example:
class Car {
private String model; // Only accessible within the Car class
public String getModel() {
return model;
}
public void setModel(String model) {
this.model = model;
}
}
Here, the model
field is private, and it can only be accessed and modified through the public getModel()
and setModel()
methods.
3. protected
The protected
access modifier allows access to the member from the same package or subclasses (including subclasses in different packages).
Protected Access: Accessible within the same package or subclasses (even if they are in different packages).
Usage: Typically used for fields and methods that should be accessible to subclasses but hidden from other classes.
Example:
class Animal {
protected String species;
}
Here, the species
field can be accessed by classes in the same package or by any subclass, even if the subclass is in a different package.
4. default (Package-Private)
If no access modifier is specified, the default access level is package-private. This means that the member is accessible only within classes that are in the same package. It is not accessible from outside the package.
Package-Private Access: Accessible only within classes in the same package.
Usage: Typically used for classes or methods that are only relevant within the same package.
Example:
class Bike {
String model; // Default access (package-private)
}
Here, the model
field is accessible only within classes in the same package.
Why Encapsulation and Access Modifiers Matter?
Data Protection: Encapsulation helps in protecting the internal state of objects, reducing the chances of unintentional or unauthorized data modification.
Code Maintenance: By controlling access to class members, encapsulation allows developers to change the internal implementation without affecting external code that uses the class.
Security: Access modifiers provide the ability to specify the level of access to fields, methods, and classes, ensuring that critical code is shielded from unwanted access.
Readability and Maintainability: Using encapsulation and access modifiers effectively makes code easier to understand and maintain, as developers can better define what parts of the code are allowed to interact with each other.
Conclusion
Encapsulation and access modifiers work together to control the visibility and accessibility of class members, ensuring that data is protected from unintended access or modification. By using private fields and public getter/setter methods, Java programmers can maintain control over how data is accessed and modified. Access modifiers provide a fine-grained approach to define access levels, from the most restrictive private
to the least restrictive public
. Together, these features are crucial in promoting good software design practices such as security, maintainability, and readability in object-oriented programming.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.