The Call Stack Chronicles: Understanding JavaScript Execution!
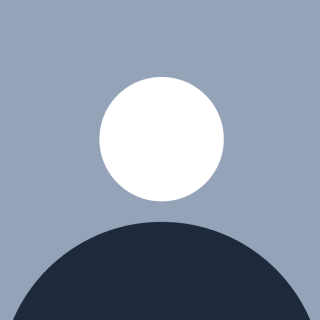
Table of contents
- What is the Call Stack? Let’s Simplify It!
- How the Call Stack Works — One Step at a Time
- Enter the Event Loop — How JavaScript Deals with Waiting
- The Call Stack in Simple Terms
- Diving Deeper into the Event Loop
- Challenge — Create a Visual Representation of the Call Stack
- Demystifying JavaScript Execution and Concurrency
- Get ready for Classes and Objects!
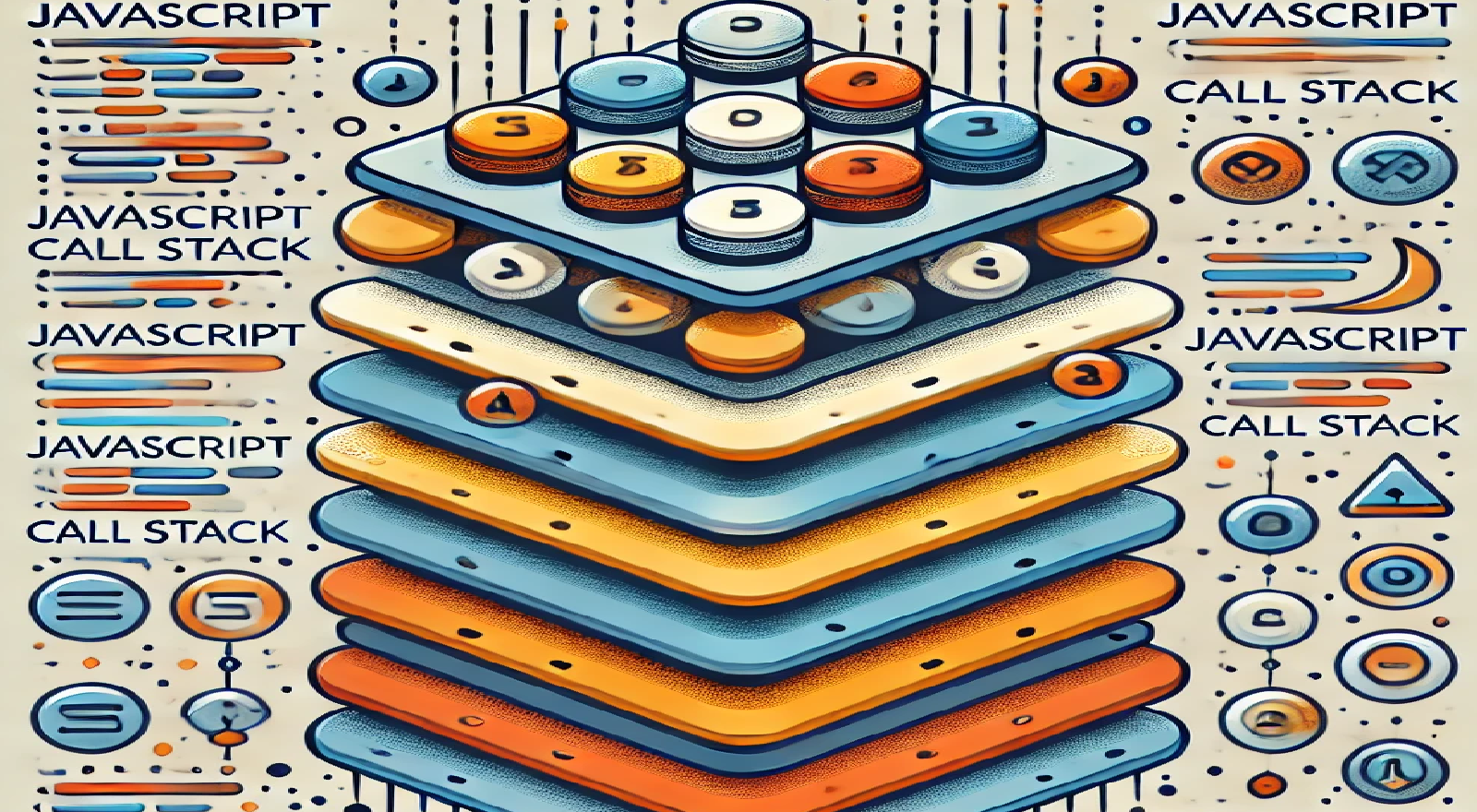
Think of the call stack in JavaScript as a stack of storybooks. Each function in your code is like a new chapter of the story. When a function starts running, it's like opening a new chapter. But you can only read one chapter at a time. Once you finish a chapter, you close it and move on to the previous chapter. The call stack works exactly the same way: one function at a time, starting at the top and moving back down when each function finishes.
What is the Call Stack? Let’s Simplify It!
At its core, the call stack is just a way for JavaScript to keep track of what’s currently happening in your code. It helps JavaScript know which function to run next.
Think of it like this:
Story starts: You call a function, it gets added to the stack.
Another function is called inside the first one: that gets added on top.
Once the top function finishes, JavaScript goes back to the previous one, and the stack shrinks.
It’s like stacking storybooks: the new book goes on top, and you finish one before going back to the previous book.
A Simple Example
Let’s say you’re writing a short story with three actions. In JavaScript, those actions might look like this:
function startAdventure() {
console.log("You begin your journey.");
}
function findTreasure() {
console.log("You found a hidden treasure!");
}
function endAdventure() {
startAdventure();
findTreasure();
console.log("Adventure over!");
}
endAdventure();
Here’s what happens, step by step:
endAdventure()
is called. This function is added to the top of the stack.Inside
endAdventure()
, thestartAdventure()
function is called. So,startAdventure()
is added on top of the stack.startAdventure()
finishes (logs "You begin your journey.") and is removed from the stack.Now,
findTreasure()
is called insideendAdventure()
, so it’s added to the stack.findTreasure()
finishes (logs "You found a hidden treasure!") and is removed.Finally,
endAdventure()
logs "Adventure over!" and is removed from the stack.
So, the order of events in the call stack would look like this:
// Flowchart for function calls:
1. [ endAdventure() ] <-- endAdventure() is called, added to the stack.
2. [ startAdventure() ] <-- startAdventure() is called, added to the stack.
3. [ startAdventure() finishes ] <-- startAdventure() finishes, removed from the stack.
4. [ endAdventure() ] <-- endAdventure() continues.
5. [ findTreasure() ] <-- findTreasure() is called, added to the stack.
6. [ findTreasure() finishes ] <-- findTreasure() finishes, removed from the stack.
7. [ endAdventure() finishes ] <-- endAdventure() is done, removed from the stack.
Each function waits its turn to be run, and once it finishes, the stack shrinks.
How the Call Stack Works — One Step at a Time
Let’s break it down even further:
New function call: When you call a function, it gets added to the top of the call stack.
Function finishes: Once that function is done, JavaScript removes it from the stack and returns to whatever was running before.
One thing at a time: JavaScript can only run one function at a time, so it keeps adding and removing functions from the stack as they start and finish.
It’s like taking notes during a meeting. You write down one point at a time, and once you’ve written down a point (or finished a task), you can move on to the next. But you can only focus on one thing at a time.
Flowchart: Visualizing the Call Stack
Here’s a simpler way to visualize what happens in the call stack as functions are called and finished:
// Flowchart for function calls:
1. [ endAdventure() ] <-- endAdventure() starts, added to the stack.
2. [ startAdventure() ] <-- startAdventure() is called, added to the stack.
3. [ startAdventure() finishes ] <-- startAdventure() finishes, removed from the stack.
4. [ findTreasure() ] <-- findTreasure() is called, added to the stack.
5. [ findTreasure() finishes ] <-- findTreasure() finishes, removed from the stack.
6. [ endAdventure() finishes ] <-- endAdventure() finishes, removed from the stack.
At each step, functions get added to the stack and are removed when they finish. The stack grows when new functions are called, and shrinks as each function finishes its work.
Enter the Event Loop — How JavaScript Deals with Waiting
Now, here’s where things get interesting. So far, we’ve been looking at functions that run one after the other. But what happens when JavaScript has to wait for something, like fetching data from an API or waiting for a timer?
This is where the event loop comes in. The event loop is like a manager making sure that everything runs smoothly. When JavaScript hits a task that takes time (like waiting for a network request), it sends that task to the task queue and moves on to the next thing in the call stack.
Once the call stack is empty, the event loop checks the task queue to see if there are any tasks ready to be processed.
Code Example: Understanding the Event Loop
Here’s a simplified example that shows how the event loop works:
console.log("Start");
setTimeout(() => {
console.log("This happens later");
}, 2000);
console.log("End");
What happens here:
"Start" is logged immediately.
The
setTimeout()
function is called, but instead of waiting for 2 seconds before moving on, JavaScript sends it off to the task queue and keeps going."End" is logged next because JavaScript didn’t wait for the 2-second timer.
After the call stack is empty, the event loop checks the task queue, sees that the 2-second timer is done, and "This happens later" is logged.
Flowchart: How the Event Loop and Task Queue Work Together
Here’s a simple flow of what happens with the event loop and task queue:
// Flowchart for event loop:
1. [ console.log("Start") ] <-- console.log("Start") runs, added to the stack.
2. [ setTimeout() ] <-- setTimeout() is called, added to the stack, and sent to task queue.
3. [ console.log("End") ] <-- console.log("End") runs next, added to the stack.
4. [ console.log("End") finishes ] <-- console.log("End") finishes, removed from the stack.
5. Event loop checks the task queue after the call stack is empty.
6. [ Task from task queue runs ] <-- Task from the queue is moved to the stack and logs "This happens later".
The event loop makes sure that long-running tasks don’t block the rest of your code from running. Instead, it waits until the main story is finished before dealing with things like timers or network requests.
The Call Stack in Simple Terms
In this first part of the Call Stack Chronicles, we’ve looked at the call stack as a way to organize and manage function calls, one step at a time. By thinking of each function as a chapter in a story, we’ve seen how JavaScript keeps track of what’s happening. We’ve also introduced the event loop, which helps manage tasks that take time without blocking the flow of the story.
In the next part, we’ll dive deeper into how the event loop, task queue, and the call stack work together to handle asynchronous tasks in JavaScript.
Diving Deeper into the Event Loop
Previously, we introduced the event loop, which works like a manager, handling tasks that take time while letting other functions run. Now, let’s look a bit more closely at how it helps JavaScript handle asynchronous operations like timers, network requests, and user interactions.
What’s Happening Behind the Scenes?
Here’s the big idea: JavaScript can only do one thing at a time because it’s single-threaded. But what if you need to wait for data from an API or respond to a button click? That’s where the event loop and task queue come into play.
When JavaScript hits an asynchronous task (like a timer or API request), it moves that task to a different place called the task queue. This way, the rest of your code keeps running, and once everything else is done, JavaScript checks the task queue to see if any long-running tasks are ready to be processed.
Code Snippet: Event Loop in Action
Let’s see how this works with a more interactive example:
console.log("A");
setTimeout(() => {
console.log("B");
}, 1000);
console.log("C");
Here’s how it works step by step:
"A" is logged immediately because it’s synchronous (it runs right away).
setTimeout()
is called, but instead of blocking the code, it schedules the function to run after 1 second and moves on."C" is logged next, immediately after "A," because
setTimeout()
didn’t block the code.After 1 second, the task queue signals that the
setTimeout()
callback is ready, and "B" is logged.
Flowchart: How the Event Loop Handles Async Tasks
Here’s a step-by-step flowchart showing how the event loop works with asynchronous tasks:
// Flowchart for event loop example:
1. [ console.log("A") ] <-- "A" is logged right away, added to the stack.
2. [ setTimeout() ] <-- setTimeout() is called, added to the stack, and moves task to the task queue.
3. [ console.log("C") ] <-- "C" is logged next, added to the stack.
4. [ console.log("C") finishes ] <-- "C" finishes, removed from the stack.
5. Event loop checks the task queue after the call stack is empty.
6. [ Task from task queue runs ] <-- Timer is up, task moves to the stack and logs "B".
The event loop ensures that JavaScript can do things like waiting for a timer or an API request without stopping the rest of your code from running. The key point is that the call stack must be empty before any tasks from the task queue can be processed.
Challenge — Create a Visual Representation of the Call Stack
Now that we’ve explored how the call stack, event loop, and task queue work together, it’s time for a challenge! Your task is to create a visual representation of how the call stack handles function calls and asynchronous tasks.
Challenge Instructions:
Create a webpage that simulates the call stack in action.
Use buttons to trigger different types of function calls and timers to simulate asynchronous tasks.
Create a visual queue that shows how tasks are added to and removed from the call stack and task queue.
Here’s a basic starter idea to help you get going:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Call Stack Visualizer</title>
<style>
#callStack, #taskQueue {
border: 1px solid black;
padding: 10px;
margin: 20px;
width: 200px;
min-height: 100px;
}
.stack-item, .queue-item {
background-color: lightblue;
padding: 5px;
margin: 5px;
}
</style>
</head>
<body>
<h1>Call Stack and Task Queue</h1>
<button onclick="runSynchronous()">Run Synchronous Function</button>
<button onclick="runAsynchronous()">Run Asynchronous Function</button>
<div id="callStack">
<h3>Call Stack</h3>
</div>
<div id="taskQueue">
<h3>Task Queue</h3>
</div>
<script>
function addToStack(functionName) {
let stack = document.getElementById("callStack");
let stackItem = document.createElement("div");
stackItem.classList.add("stack-item");
stackItem.textContent = functionName;
stack.appendChild(stackItem);
}
function removeFromStack() {
let stack = document.getElementById("callStack");
stack.removeChild(stack.lastChild);
}
function runSynchronous() {
addToStack("runSynchronous");
setTimeout(removeFromStack, 1000); // Simulate function execution time
}
function runAsynchronous() {
addToStack("setTimeout (Async Task)");
setTimeout(() => {
let queue = document.getElementById("taskQueue");
let queueItem = document.createElement("div");
queueItem.classList.add("queue-item");
queueItem.textContent = "Task Completed!";
queue.appendChild(queueItem);
removeFromStack();
}, 2000);
}
</script>
</body>
</html>
Explanation:
This simple webpage simulates the call stack and task queue visually.
Clicking the “Run Synchronous Function” button shows how a function is added to the call stack and removed after it finishes.
Clicking the “Run Asynchronous Function” button shows how a task is added to the stack and, after a delay, moves to the task queue when complete.
You can experiment with this basic structure by adding more functions, timing delays, and other interactions to better understand how JavaScript processes tasks.
Demystifying JavaScript Execution and Concurrency
By now, you’ve learned that JavaScript’s call stack processes functions one by one, while the event loop and task queue handle asynchronous operations without blocking the main thread. This setup ensures that your program can handle complex tasks, such as making API requests or waiting for timers, without slowing down or freezing.
Key Takeaways:
The call stack keeps track of which functions are running, adding and removing them as they start and finish.
The event loop ensures that asynchronous tasks are handled without interrupting the main program flow.
The task queue stores tasks that need to be run once the call stack is empty, and the event loop moves these tasks into the stack when ready.
This system allows JavaScript to handle concurrency, making sure everything runs smoothly even when tasks take time.
Get ready for Classes and Objects!
In the next article, we’ll be diving into classes and objects—a key part of object-oriented programming in JavaScript. Get ready to explore how to create blueprints for your data and how objects can help you organize complex applications!
Subscribe to my newsletter
Read articles from gayatri kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by