Polymorphism in Java

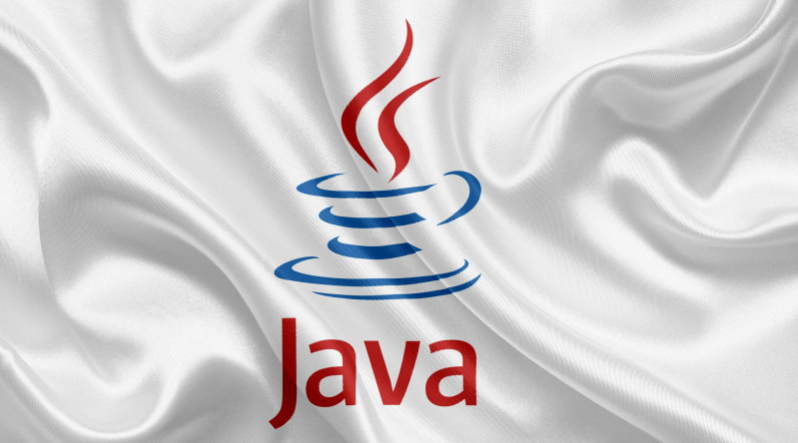
Polymorphism is one of the core concepts of Object-Oriented Programming (OOP) and is a feature that allows one interface to be used for a general class of actions. In Java, polymorphism enables the use of objects of different classes through a common interface, typically in the context of method overriding. This article will focus on Polymorphism, specifically Dynamic Method Dispatch, and explain its importance and how it works in Java.
What is Polymorphism?
Polymorphism comes from the Greek words “poly” (meaning many) and “morph” (meaning form), and it refers to the ability of a single function, method, or operator to behave in different ways depending on the context. In the context of Java, polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables methods to operate on objects of different types, and the behavior can vary based on the object’s actual class type.
There are two types of polymorphism in Java:
Compile-time Polymorphism (also known as Method Overloading)
Runtime Polymorphism (also known as Dynamic Method Dispatch)
This article focuses on runtime polymorphism, which is achieved through dynamic method dispatch.
Dynamic Method Dispatch (Runtime Polymorphism)
Dynamic Method Dispatch is the mechanism in Java by which a call to an overridden method is resolved at runtime, rather than at compile-time. This means that the method that gets called is determined at runtime based on the actual object type (not the reference type).
In Java, this occurs when a method in a subclass overrides a method in a superclass. At runtime, Java determines which version of the method (superclass or subclass) should be called based on the type of the object being referenced, not the type of the reference variable itself.
This process allows Java to achieve runtime polymorphism, where the same method name can behave differently based on the object that it is acting upon.
How Dynamic Method Dispatch Works?
The core of dynamic method dispatch lies in method overriding. In Java, when a subclass provides its own implementation of a method that is already defined in the superclass, the method in the subclass is said to override the method in the superclass.
Here’s how dynamic method dispatch works:
A reference variable of the superclass type is created.
An object of the subclass is assigned to this reference.
When a method is called on this reference, the Java Virtual Machine (JVM) determines which version of the method to execute at runtime based on the actual object type.
This allows Java to support method overriding and polymorphic behavior, making the language more flexible and scalable.
Example of Dynamic Method Dispatch
Let's consider the following example to better understand how dynamic method dispatch works:
// Superclass Animal
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
// Subclass Dog
class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
// Subclass Cat
class Cat extends Animal {
@Override
void sound() {
System.out.println("Cat meows");
}
}
public class TestPolymorphism {
public static void main(String[] args) {
// Creating references of Animal
Animal animal;
// Assigning Dog object to Animal reference
animal = new Dog();
animal.sound(); // Output: Dog barks
// Assigning Cat object to Animal reference
animal = new Cat();
animal.sound(); // Output: Cat meows
}
}
Breakdown:
The
sound()
method is overridden in both theDog
andCat
subclasses.In the
main()
method, anAnimal
reference is used to point toDog
andCat
objects.Even though the reference variable
animal
is of typeAnimal
, the methodsound()
called onanimal
invokes the overridden methods inDog
andCat
based on the actual object type (at runtime).
Thus, at runtime:
When
animal
references aDog
object, theDog
version ofsound()
is invoked.When
animal
references aCat
object, theCat
version ofsound()
is invoked.
This is dynamic method dispatch, where the method call is resolved dynamically at runtime.
Importance of Dynamic Method Dispatch (Runtime Polymorphism)
Dynamic method dispatch is a powerful concept in Java, as it offers several benefits in the context of OOP:
Flexibility and Extensibility: Dynamic method dispatch allows new subclasses to be added without changing the code that uses the superclass reference. This helps in extending the behavior of existing systems with minimal changes, improving maintainability and scalability.
Loose Coupling: It enables loose coupling between classes. The caller does not need to know the specific type of the object being referenced, as the correct method is determined at runtime. This promotes the use of generic code and interfaces.
Code Reusability: It facilitates the reuse of code. The same method name can be used for different object types, leading to cleaner and more concise code.
Design Patterns: Many design patterns, such as the Strategy and State patterns, rely heavily on polymorphism. They allow different behaviors to be executed based on the actual object type without changing the client code.
Implementing Interfaces: Dynamic method dispatch is fundamental in implementing interfaces and abstract classes in Java. It helps achieve polymorphism and facilitates implementing multiple versions of an interface or abstract class.
Key Points to Remember
Method overriding is essential for dynamic method dispatch in Java.
The method call is resolved at runtime based on the actual object type, not the reference type.
Dynamic method dispatch enables runtime polymorphism, where multiple classes can share the same method name but have different implementations.
It makes Java more flexible and extensible, reducing code redundancy and promoting better software design.
Conclusion
Polymorphism, specifically dynamic method dispatch, is a cornerstone of Object-Oriented Programming (OOP) in Java. It allows for more flexible and reusable code by letting the same method behave differently depending on the object type. This feature is essential in designing scalable and maintainable Java applications, and it is widely used in frameworks and libraries for implementing runtime polymorphism. By understanding and using dynamic method dispatch, developers can create more adaptable and efficient systems, making Java a powerful language for building large, complex applications.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.