Abstract Classes and Interfaces in Java

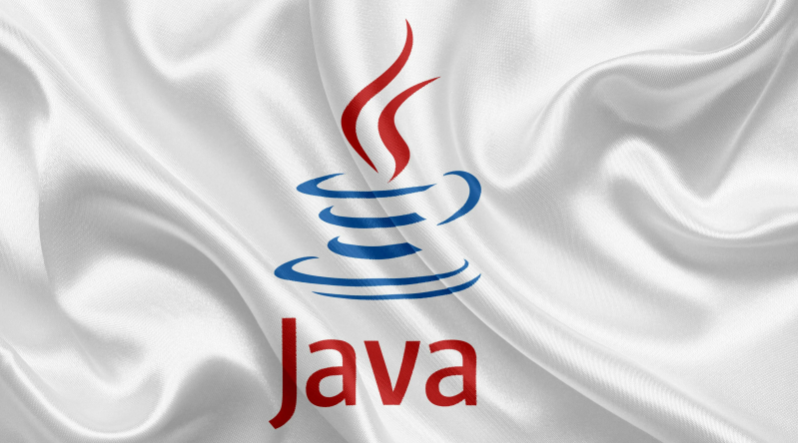
Abstract Classes and Interfaces are two fundamental concepts in Java that are crucial for achieving abstraction in object-oriented programming (OOP). These concepts allow you to define classes and methods that are meant to be implemented by other classes, enabling you to create more flexible and scalable systems. While both abstract classes and interfaces serve the purpose of defining methods that must be implemented by subclasses, they are used in different scenarios and have distinct characteristics.
In this article, we will explore Abstract Classes and Interfaces, their differences, when to use them, and how they contribute to Java's object-oriented paradigm.
What is an Abstract Class?
An abstract class is a class that cannot be instantiated directly, meaning you cannot create objects of an abstract class. It is used to define a common base class with shared functionality that must be implemented by its subclasses. An abstract class can have both abstract methods (methods without implementation) and concrete methods (methods with implementation).
Key Characteristics of Abstract Classes:
Abstract Methods: These are methods without any implementation. Subclasses must provide their implementation.
abstract class Animal { abstract void sound(); // abstract method }
Concrete Methods: These are methods that have a full implementation. Subclasses can inherit these methods directly without needing to override them unless desired.
abstract class Animal { void eat() { System.out.println("This animal eats food"); } }
Constructors: Abstract classes can have constructors, which can be called by the subclasses when they are instantiated.
Instance Variables: Like regular classes, abstract classes can have instance variables, and these variables can be inherited by subclasses.
Cannot be instantiated: You cannot create an object of an abstract class directly. Instead, you must instantiate a subclass that provides implementations for the abstract methods.
Example of Abstract Class:
abstract class Animal {
abstract void sound(); // Abstract method
void eat() { // Concrete method
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void sound() {
System.out.println("Dog barks");
}
}
public class TestAbstract {
public static void main(String[] args) {
Animal dog = new Dog();
dog.sound(); // Output: Dog barks
dog.eat(); // Output: Animal is eating
}
}
What is an Interface?
An interface in Java is a reference type, similar to a class, but it is used to specify a set of methods that must be implemented by a class. Unlike abstract classes, interfaces only contain method signatures (without any implementation) and constants. A class that implements an interface must provide implementations for all of the methods defined in the interface.
Key Characteristics of Interfaces:
Method Signatures: An interface only provides method signatures, without any implementation. The implementing class provides the method implementations.
No Constructors: Interfaces cannot have constructors, as they cannot be instantiated directly.
Multiple Inheritance: A class can implement multiple interfaces, providing a form of multiple inheritance in Java (which is not possible with classes).
Constants: All variables in an interface are implicitly
public
,static
, andfinal
. They are constants and must be initialized when declared.No Instance Variables: Interfaces do not contain instance variables, but they can have constants.
Example of Interface:
interface Animal {
void sound(); // Method signature
void eat(); // Method signature
}
class Dog implements Animal {
public void sound() {
System.out.println("Dog barks");
}
public void eat() {
System.out.println("Dog eats food");
}
}
public class TestInterface {
public static void main(String[] args) {
Animal dog = new Dog();
dog.sound(); // Output: Dog barks
dog.eat(); // Output: Dog eats food
}
}
Key Differences Between Abstract Classes and Interfaces
Aspect | Abstract Class | Interface |
Inheritance | A class can inherit from only one abstract class. | A class can implement multiple interfaces. |
Methods | Can have both abstract and concrete methods. | Can only have abstract methods (until Java 8, which introduced default methods). |
Constructor | Can have constructors. | Cannot have constructors. |
Access Modifiers | Methods can have any access modifier (private, protected, public). | All methods are implicitly public . |
Instance Variables | Can have instance variables. | Cannot have instance variables. |
Purpose | Used when classes share a common base but need some differences. | Used to define common behavior for unrelated classes. |
Multiple Inheritance | Not supported (only single inheritance). | Supports multiple inheritance (a class can implement multiple interfaces). |
When to Use Abstract Classes and Interfaces?
Use an Abstract Class When:
You want to provide a common base class with shared behavior for subclasses but still enforce the implementation of some methods.
You want to provide both abstract methods (which subclasses must implement) and concrete methods (which are inherited).
You want to allow subclasses to inherit common state and functionality (instance variables and methods).
Use an Interface When:
You want to define a contract for classes without enforcing how they implement the methods.
You want to enable a class to inherit behavior from multiple sources (Java allows a class to implement multiple interfaces).
You are defining a common set of methods that are shared across completely unrelated classes (i.e., classes that don’t share a common ancestor).
Default and Static Methods in Interfaces (Java 8 and Above)
Starting from Java 8, interfaces can also include default methods and static methods.
Default Methods: These methods have a default implementation and can be called directly on objects that implement the interface, even if the implementing class does not provide an implementation.
Static Methods: These methods belong to the interface and can be invoked on the interface itself, not the objects implementing the interface.
Example of Default and Static Methods:
interface Animal {
void sound();
default void sleep() {
System.out.println("Animal is sleeping");
}
static void eat() {
System.out.println("Animal is eating");
}
}
class Dog implements Animal {
public void sound() {
System.out.println("Dog barks");
}
}
public class TestInterface {
public static void main(String[] args) {
Animal dog = new Dog();
dog.sound(); // Output: Dog barks
dog.sleep(); // Output: Animal is sleeping (default method)
Animal.eat(); // Output: Animal is eating (static method)
}
}
Conclusion
Abstract Classes and Interfaces are both essential components in Java’s OOP model, but they serve different purposes. Abstract classes are suitable when classes share a common base and need some degree of implementation. On the other hand, interfaces are ideal for defining common behavior across unrelated classes and allowing multiple inheritance.
Understanding the differences and when to use each will help you design more flexible, maintainable, and extensible Java applications. By leveraging abstract classes and interfaces effectively, you can achieve abstraction, ensure modularity, and improve the scalability of your systems.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.