Composition vs Inheritance in Java

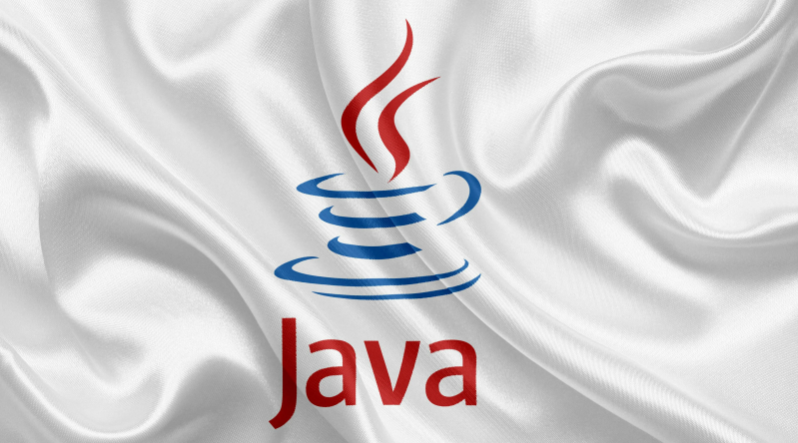
In Java, Composition and Inheritance are two fundamental concepts used to create relationships between classes, and they each have distinct advantages and use cases. While both allow for code reuse and the creation of flexible systems, choosing between them requires understanding their core differences and the impact on design, maintainability, and scalability of an application.
What is Inheritance?
Inheritance is a mechanism in object-oriented programming that allows one class to inherit fields and methods from another class. This allows for creating a new class based on an existing class, where the new class can extend the functionality of the parent class without re-writing the existing code.
Key Features of Inheritance:
Inheritance represents an "is-a" relationship. For example, a
Dog
"is a" type ofAnimal
.It allows for code reuse, meaning that a subclass can use methods and fields of its superclass.
Java supports single inheritance, meaning a class can inherit from only one direct superclass.
Example:
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog barks");
}
}
public class TestInheritance {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat(); // Inherited method from Animal class
dog.bark(); // Method of Dog class
}
}
What is Composition?
Composition is an object-oriented design principle where one class contains instances of other classes as fields, thereby using their functionality. It represents a "has-a" relationship. Composition allows for the construction of complex types by combining objects of different classes, rather than inheriting from a parent class.
Key Features of Composition:
Composition represents a "has-a" relationship. For example, a
Car
"has a"Engine
.It enables greater flexibility and decoupling, as objects can be composed of different components.
Composition avoids the limitations of inheritance, such as tight coupling and the issue of single inheritance in Java.
Example:
class Engine {
void start() {
System.out.println("Engine starting");
}
}
class Car {
private Engine engine;
Car() {
engine = new Engine();
}
void drive() {
engine.start();
System.out.println("Car is driving");
}
}
public class TestComposition {
public static void main(String[] args) {
Car car = new Car();
car.drive(); // Engine functionality is composed into Car class
}
}
Key Differences Between Composition and Inheritance
Aspect | Inheritance | Composition |
Relationship | Represents "is-a" relationship | Represents "has-a" relationship |
Flexibility | Inflexible, as it is based on a rigid class hierarchy | Highly flexible, as classes can be composed dynamically |
Coupling | Tightly coupled between superclass and subclass | Loosely coupled, classes are more independent |
Reusability | Inheriting methods and fields from a parent class | Reuse functionality by including objects of other classes |
Change Impact | Changes in the superclass may affect all subclasses | Changes in components do not affect the containing class |
Multiple Inheritance | Java allows only single inheritance | Java allows composition of multiple components |
Suitability | Best suited for representing hierarchies | Best suited for creating complex objects with different behaviors |
When to Use Inheritance?
When you have a clear hierarchical relationship between the classes, such as "Dog is a type of Animal".
When subclassing adds additional behavior to an existing class while maintaining the core functionality.
When the functionality in the parent class is generalized and needs to be extended by the child class.
When to Use Composition?
When you need to represent relationships where one class "has" other classes as parts, such as "Car has an Engine".
When you want to avoid tight coupling and need flexibility to change the behavior or structure of objects.
When the behavior of a class should be dynamically composed from different components, enabling greater code reuse.
Conclusion
Both Composition and Inheritance play important roles in object-oriented design, but they are suited for different situations. Inheritance provides a simpler mechanism for code reuse when there is a clear "is-a" relationship, whereas Composition offers more flexibility and reduces the risks associated with tightly coupled class hierarchies. In general, composition is often preferred for creating more modular, maintainable, and scalable systems, but there are cases where inheritance remains the best option, especially when you are working with naturally hierarchical structures.
Ultimately, understanding the difference between these two concepts and knowing when to use each one is crucial to designing effective object-oriented applications.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.