Inner Classes and Anonymous Classes in Java

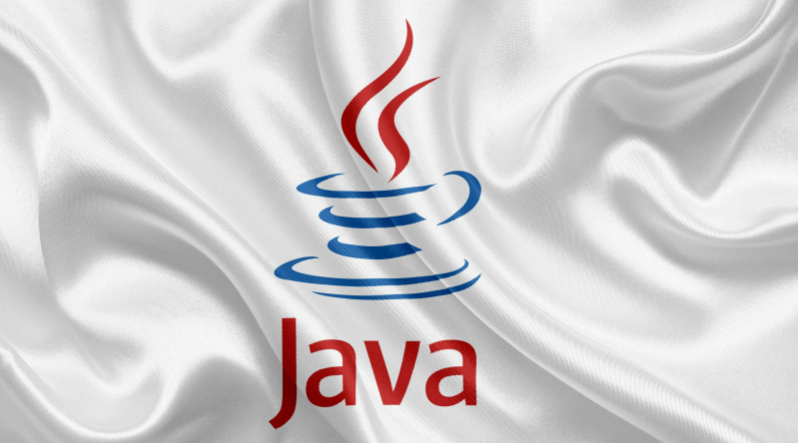
In Java, inner classes and anonymous classes are mechanisms that allow you to define classes within other classes. These classes provide significant advantages in terms of readability, encapsulation, and flexibility in various scenarios. While both types of classes are defined inside other classes, they serve different purposes and are used in distinct situations.
What are Inner Classes?
Inner classes are classes defined within another class. They are part of the outer class and can access its members, including private fields and methods. Inner classes allow for grouping related classes together, making the code more readable and organized.
Types of Inner Classes:
Non-static Inner Class (Member Inner Class):
A member inner class is associated with an instance of the outer class. It can access all fields and methods of the outer class, including private ones.
You must instantiate the outer class before you can create an instance of the member inner class.
Example:
class OuterClass {
private String outerField = "Outer class field";
class InnerClass {
void display() {
System.out.println(outerField); // Accessing outer class field
}
}
}
public class TestInnerClass {
public static void main(String[] args) {
OuterClass outer = new OuterClass();
OuterClass.InnerClass inner = outer.new InnerClass(); // Creating inner class instance
inner.display();
}
}
Static Inner Class:
A static inner class is not associated with an instance of the outer class. It can only access the static members of the outer class.
It behaves like a regular static member of the outer class.
Example:
class OuterClass {
static String outerField = "Static Outer class field";
static class StaticInnerClass {
void display() {
System.out.println(outerField); // Accessing static outer class field
}
}
}
public class TestStaticInnerClass {
public static void main(String[] args) {
OuterClass.StaticInnerClass inner = new OuterClass.StaticInnerClass();
inner.display();
}
}
Local Inner Class:
A local inner class is defined inside a method, constructor, or block, and is used for local computations.
It can access local variables of the enclosing block, but only if they are declared final or effectively final.
Example:
class OuterClass {
void methodWithInnerClass() {
final String localVar = "Local variable";
class LocalInnerClass {
void display() {
System.out.println(localVar); // Accessing local variable
}
}
LocalInnerClass localInner = new LocalInnerClass();
localInner.display();
}
}
public class TestLocalInnerClass {
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.methodWithInnerClass();
}
}
Anonymous Inner Class:
An anonymous inner class is a special type of inner class with no name. It is used to instantiate a class and define its behavior in a single expression.
It is often used to create instances of classes that implement interfaces or extend other classes without needing a separate class definition.
What are Anonymous Classes?
An anonymous class is a local inner class with no name, and it is used to instantiate objects of either a class or an interface. Anonymous classes are typically used when you need a one-time implementation of an interface or class, often for event handling, callbacks, or short-lived objects.
Key Features of Anonymous Classes:
They do not have a constructor or a class name.
They are defined and instantiated at the same time.
They are often used with interfaces and abstract classes, providing a quick implementation for one-off uses.
Example: Using Anonymous Class with an Interface
interface Greet {
void sayHello();
}
public class TestAnonymousClass {
public static void main(String[] args) {
Greet greet = new Greet() {
public void sayHello() {
System.out.println("Hello from Anonymous Class!");
}
};
greet.sayHello();
}
}
In this example, the Greet
interface is implemented using an anonymous class. The class is instantiated and the sayHello()
method is provided within the curly braces {}
.
Key Differences Between Inner Classes and Anonymous Classes
Aspect | Inner Classes | Anonymous Classes |
Naming | Have a name (except for local inner classes) | Do not have a name |
Usage | Used for multiple instances, can have multiple methods and constructors | Used for single-use implementations |
Scope | Can be used as a full class, can be instantiated multiple times | Typically used in situations requiring a one-time use |
Constructor | Can have a constructor | Cannot have a constructor |
Flexibility | More flexible, can be used for reusable logic | Less flexible, intended for short-lived use |
Purpose | Grouping related classes together and adding structure | Providing quick implementation of an interface or abstract class |
When to Use Inner Classes vs Anonymous Classes?
Use Inner Classes:
When you need to define a class that logically belongs to another class and needs multiple instances.
When you want to access members of the outer class and need to define methods or constructors.
Use Anonymous Classes:
When you need a one-off implementation of an interface or abstract class.
When you are dealing with event handling, callbacks, or other short-lived use cases where defining a full class is unnecessary.
Conclusion
Both inner classes and anonymous classes offer unique ways to structure code in Java. Inner classes allow you to group related classes together, making your code more organized and modular, while anonymous classes provide a quick and convenient way to implement interfaces or abstract classes for single-use cases. Choosing between them depends on the specific requirements of your code, such as whether you need a reusable class or just a one-off implementation.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.