Enums in Java

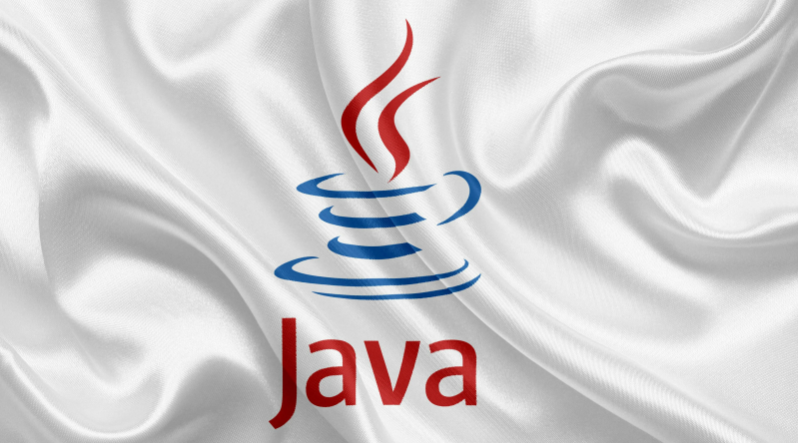
In Java, an enum (short for enumeration) is a special type of class that represents a collection of constants (unchangeable variables, like final
variables). Enums are used to define a set of named constants that are often related and can be used to represent fixed values in a program.
Enums provide a type-safe way of handling fixed sets of constants, ensuring that the values are limited to a specific set and reducing the risk of errors that can arise from using arbitrary values.
Why Use Enums in Java?
Type Safety: Enums restrict the values to predefined constants, reducing the chances of introducing invalid values.
Readability: Using enums makes the code more readable, as the named constants clearly convey their meaning.
Built-in Methods: Enums come with several built-in methods that make working with them easier, such as
values()
,valueOf()
, andordinal()
.Flexibility: Enums can have fields, methods, and constructors, making them more flexible than simple constants.
Defining an Enum in Java
An enum in Java is defined using the enum
keyword. Here's a simple example:
Example: Basic Enum
enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
public class TestEnum {
public static void main(String[] args) {
Day today = Day.MONDAY;
switch (today) {
case MONDAY:
System.out.println("Start of the week!");
break;
case FRIDAY:
System.out.println("End of the week!");
break;
case SUNDAY:
System.out.println("Rest day!");
break;
default:
System.out.println("Work day!");
}
}
}
Explanation:
The
Day
enum defines seven constants representing the days of the week.The
switch
statement is used to check the current day and perform actions based on it.
Methods in Enum
Java provides several useful methods for working with enums:
values()
: This method returns all the constants of the enum in the order they are declared.valueOf(String name)
: This method returns the enum constant corresponding to the specified name (case-sensitive).ordinal()
: This method returns the position of the enum constant, starting from0
.name()
: This method returns the name of the enum constant.
Example: Using values()
, valueOf()
, and ordinal()
enum Color {
RED, GREEN, BLUE;
}
public class EnumExample {
public static void main(String[] args) {
// Using values() to get all constants
for (Color color : Color.values()) {
System.out.println(color);
}
// Using valueOf() to get a specific enum constant
Color myColor = Color.valueOf("GREEN");
System.out.println("Selected color: " + myColor);
// Using ordinal() to get the index of an enum constant
System.out.println("Index of RED: " + Color.RED.ordinal());
}
}
Explanation:
The
values()
method returns all enum constants (RED
,GREEN
,BLUE
).valueOf("GREEN")
returns theGREEN
constant.ordinal()
shows the index of the constant (e.g.,RED
is at index0
).
Enums with Fields and Methods
Enums can also have fields, methods, and constructors. You can define behavior within an enum, which is especially useful when you want to associate specific properties with each constant.
Example: Enums with Fields and Methods
enum Planet {
MERCURY(3.303e+23, 2.4397e6), // Mass and radius for Mercury
VENUS(4.869e+24, 6.0518e6), // Mass and radius for Venus
EARTH(5.974e+24, 6.3781e6); // Mass and radius for Earth
private final double mass; // in kilograms
private final double radius; // in meters
// Constructor to initialize mass and radius for each planet
Planet(double mass, double radius) {
this.mass = mass;
this.radius = radius;
}
// Method to calculate the surface gravity of the planet
public double surfaceGravity() {
final double G = 6.67430e-11; // gravitational constant
return G * mass / (radius * radius);
}
// Method to return the gravitational force between two planets
public double gravitationalForce(Planet other) {
final double G = 6.67430e-11; // gravitational constant
return G * this.mass * other.mass / Math.pow(this.radius - other.radius, 2);
}
}
public class TestEnum {
public static void main(String[] args) {
Planet earth = Planet.EARTH;
System.out.println("Surface gravity on Earth: " + earth.surfaceGravity() + " m/s²");
}
}
Explanation:
Each planet in the
Planet
enum hasmass
andradius
fields.The constructor is used to initialize the values of
mass
andradius
for each constant.We define a method
surfaceGravity()
to calculate the gravitational force based on the planet's mass and radius.
Enum in Switch
Statements
Enums are often used in switch
statements to handle multiple conditions based on the enum constants. Since enums are type-safe, they ensure that the values used in the switch
case are valid constants, providing an error-free and easy-to-read solution.
Example: Using Enums with Switch
enum TrafficLight {
RED, YELLOW, GREEN;
}
public class TrafficControl {
public static void main(String[] args) {
TrafficLight light = TrafficLight.RED;
switch (light) {
case RED:
System.out.println("Stop");
break;
case YELLOW:
System.out.println("Get Ready");
break;
case GREEN:
System.out.println("Go");
break;
}
}
}
Key Benefits of Enums
Type Safety: You can't assign an arbitrary value to an enum, as it only allows predefined constants.
Code Clarity: Enums make the code more readable and meaningful by using descriptive names for constant values.
Efficiency: Enums can be used with
switch
statements, making it easier to handle multiple conditions without the risk of invalid values.Extensibility: Enums can have fields, methods, and constructors, enabling you to add behavior to constants.
Conclusion
Enums in Java are a powerful tool to represent a fixed set of related constants. They help in improving the readability, maintainability, and safety of the code by providing type-safe values. Enums can also have fields, methods, and constructors, making them more versatile than simple constant values. Understanding how to use enums effectively can make your code more organized, especially when dealing with fixed sets of values like days of the week, months, colors, and more.
Subscribe to my newsletter
Read articles from Mohammed Shakeel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohammed Shakeel
Mohammed Shakeel
I'm Mohammed Shakeel, an aspiring Android developer and software engineer with a keen interest in web development. I am passionate about creating innovative mobile applications and web solutions that are both functional and aesthetically pleasing.