Step-by-Step Guide to Integrating mdsvex, SvelteKit v5, and Tailwind CSS for Best Performance

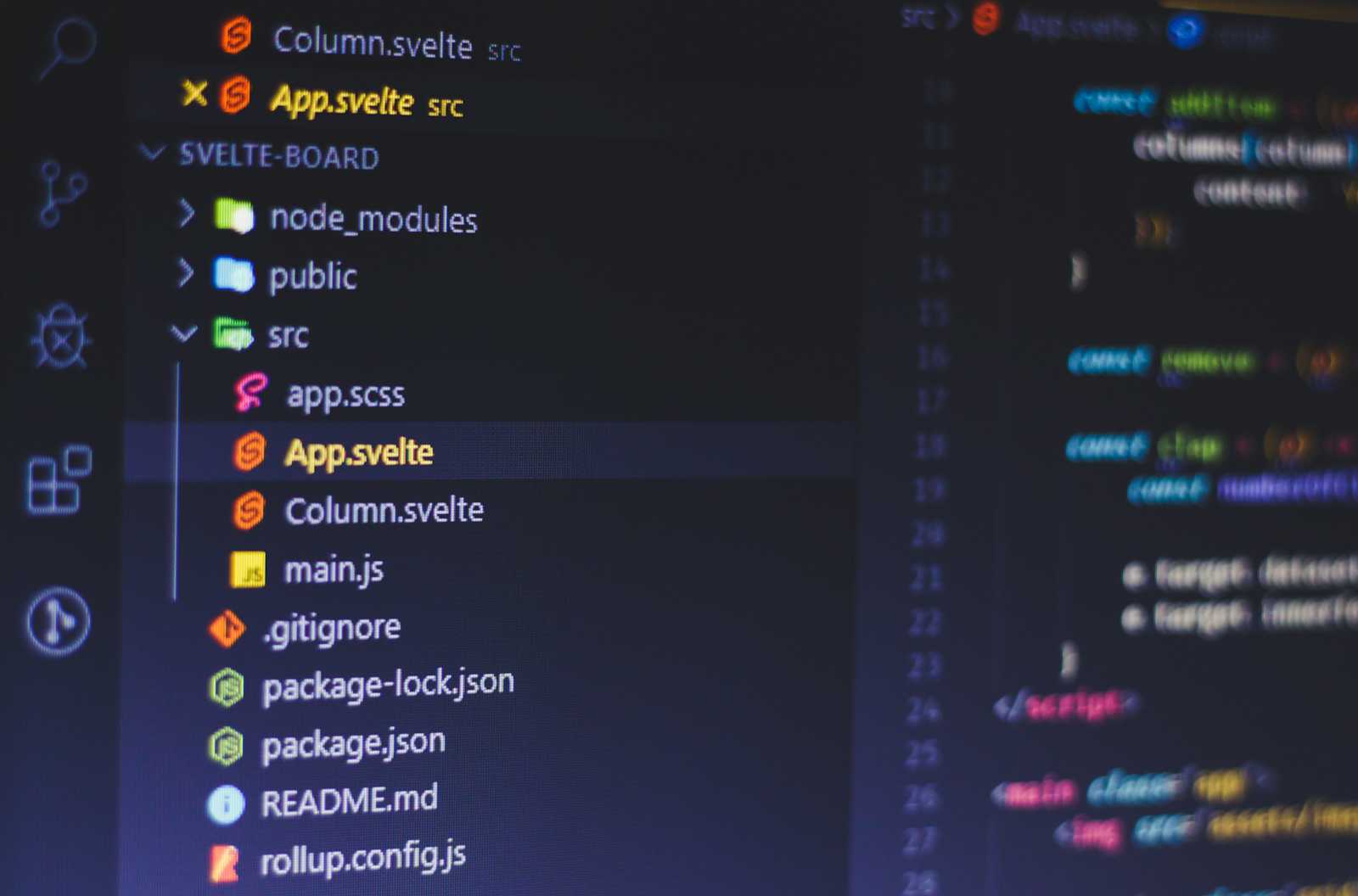
Setting Up mdsvex with SvelteKit v5 and Tailwind CSS: A Developer's Guide
As developers, we love tools that make our workflow easier. One such tool is mdsvex
, which allows us to seamlessly use Markdown within our Svelte components. When combined with SvelteKit v5 and Tailwind CSS, you get a powerful and flexible setup for building content-driven websites.
File Structure Overview
Here’s what your basic project structure should look like after setting up mdsvex
and Tailwind CSS:
/myapp
/src
/routes
/first-post
+page.svx
/app.css
svelte.config.js
package.json
The
src/routes/first-post/+page.svx
file will represent your route for thefirst-post
page with markdown content.app.css
includes Tailwind's setup and your custom styles.
Step 1: Installing the Packages
If you're starting fresh with SvelteKit v5, create a new project:
npx sv create myapp
Then navigate into your project directory:
cd myapp
To add mdsvex
, use the following command:
npx sv add mdsvex
This will automatically install and configure mdsvex
for your project, so you don't need to touch svelte.config.js
manually.
Step 2: Installing Tailwind CSS
Adding Tailwind CSS to your SvelteKit project has never been easier. Simply run:
npx sv add tailwindcss
This will automatically configure Tailwind CSS for your project without needing to manually edit any files. You’ll have Tailwind set up and ready to go.
Once that’s done, you can start using Tailwind’s utility classes right away in your .svx
and .svelte
files. For instance, you can add some basic styles in src/app.css
like so:
@tailwind base;
@tailwind components;
@tailwind utilities;
@layer base {
h1 {
@apply text-2xl font-bold;
}
p {
@apply text-base;
}
}
Step 3: Configure SvelteKit with mdsvex
Now, update svelte.config.js
to ensure mdsvex
is set up correctly. The file should look something like this:
import { mdsvex } from 'mdsvex';
import adapter from '@sveltejs/adapter-auto';
import { vitePreprocess } from '@sveltejs/vite-plugin-svelte';
const config = {
preprocess: [vitePreprocess(), mdsvex()],
kit: {
adapter: adapter(),
},
extensions: ['.svelte', '.svx'],
};
export default config;
This configuration tells SvelteKit to process .svx
files as Svelte components, which is the key for getting Markdown support.
Step 4: Using mdsvex for Routes
Now you can start writing your routes using Markdown inside .svx
files. For example, let's create a post route at src/routes/first-post/+page.svx
.
The content of +page.svx
could look like this:
# First Post
This is my first blog post created with **mdsvex** and **SvelteKit**.
## Introduction
In this post, I’ll show how easy it is to integrate markdown in SvelteKit with `mdsvex`. Here's a simple list of cool things we can do:
- Markdown inside `.svx` files
- Tailwind CSS for styling
- SvelteKit for routing and SSR
- And much more!
SvelteKit will automatically treat this .svx
file as a route, and the path /first-post
will render this content.
Step 5: Adding Styles
You can add custom styles to your markdown-based pages in two ways:
Option 1: Adding Styles in the +layout.svelte
of the Route
If you want to wrap your route with a custom layout, you can define it in +layout.svelte
within the same route folder. Here’s an example:
<script lang="ts">
import type { Snippet } from 'svelte';
import type { LayoutData } from './$types';
let { data, children }: { data: LayoutData; children: Snippet } = $props();
</script>
<div class="container">
{@render children()}
</div>
In this layout, you're rendering the child content (like your markdown file) and wrapping it with the class container
, where you can apply any Tailwind classes or custom styles.
Option 2: Adding Styles Directly in the .svx
File
You can also include custom styles directly inside your .svx
file. For example, to style headings and paragraphs in the markdown, you could add the following <style>
block:
<style>
/* Headings */
h1 {
font-size: 2.5rem;
font-weight: 700;
margin-bottom: 1rem;
padding: 0.5rem 1rem; /* Add padding top/bottom and left/right */
}
h2 {
font-size: 2rem;
font-weight: 600;
margin-bottom: 0.75rem;
padding: 0.375rem 1rem; /* Add padding top/bottom and left/right */
}
h3 {
font-size: 1.5rem;
font-weight: 500;
margin-bottom: 0.5rem;
padding: 0.25rem 1rem; /* Add padding top/bottom and left/right */
}
h4 {
font-size: 1.25rem;
font-weight: 400;
margin-bottom: 0.25rem;
padding: 0.125rem 1rem; /* Add padding top/bottom and left/right */
}
h5 {
font-size: 1rem;
font-weight: 400;
margin-bottom: 0.25rem;
padding: 0.125rem 1rem; /* Add padding top/bottom and left/right */
}
h6 {
font-size: 0.875rem;
font-weight: 400;
margin-bottom: 0.25rem;
padding: 0.125rem 1rem; /* Add padding top/bottom and left/right */
}
/* Paragraphs */
p {
font-size: 1rem;
line-height: 1.5;
margin-bottom: 1rem;
}
/* Lists */
ul,
ol {
margin-bottom: 1rem;
padding-left: 2rem;
}
li {
margin-bottom: 0.5rem;
}
/* Code */
code {
font-family: monospace;
background-color: #f5f5f5;
padding: 0.25rem 0.5rem;
border-radius: 4px;
}
pre {
background-color: #f5f5f5;
padding: 1rem;
border-radius: 4px;
overflow-x: auto;
}
pre code {
padding: 0;
background-color: transparent;
}
/* Links */
a {
color: blue;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
/* Blockquotes */
blockquote {
border-left: 4px solid #ddd;
padding-left: 1rem;
margin-bottom: 1rem;
}
/* Horizontal Rules */
hr {
border: 0;
border-top: 1px solid #ddd;
margin: 2rem 0;
}
</style>
This will style all the markdown elements (headings, paragraphs, code blocks, etc.) directly within your .svx
file.
Step 6: Importing Scripts and Components
Just like in a regular Svelte component, you can import other scripts, components, or libraries into your .svx
file. Here’s an example:
<script>
import MyComponent from '$lib/MyComponent.svelte';
let message = 'Hello from Markdown!';
</script>
<MyComponent {message} />
This allows you to enhance your markdown content with dynamic Svelte components and logic.
Step 7: Troubleshooting—No Styles Applied?
If your Tailwind styles are not being applied to your markdown content, check the following:
Ensure that the
@tailwind
directives are correctly added tosrc/app.css
.Make sure you’ve set up
content
intailwind.config.js
to include.svx
files.Restart the dev server after changes to ensure everything is recompiled.
Step 8: Best Practices
Here are a few tips for working with mdsvex
:
Use Tailwind for Global Styling: Tailwind is perfect for utility-first styling. Apply it in your
app.css
file to keep things consistent.Keep Markdown Simple: If you
need heavy customization, you may want to switch to Svelte components instead of relying on markdown.
- Leverage Svelte Components: Always feel free to import Svelte components into your markdown for dynamic content rendering.
By combining mdsvex
, SvelteKit v5, and Tailwind CSS, you now have a powerful, flexible toolset for building content-rich websites with ease. Happy coding!
Subscribe to my newsletter
Read articles from Michael Amachree directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
