Mastering Git: A Complete Guide to Essential Concepts, Commands, and Practical Applications
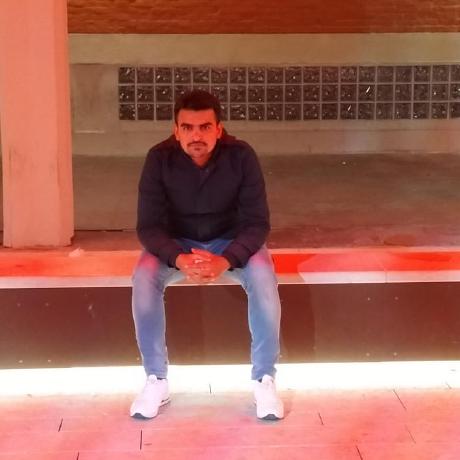
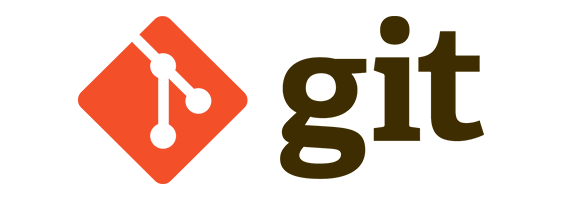
Git is a powerful version control system widely used for tracking changes in code, managing collaborative workflows, and ensuring code quality and stability across projects. This guide covers fundamental and advanced Git commands, practical applications, and real-world scenarios where Git becomes indispensable.
Why Use Git?
Git offers several advantages for managing code:
Tracking Changes: Every change in a project is stored, allowing easy reversion if necessary.
Branching and Merging: Git enables branching, allowing isolated development without affecting the main codebase. Once changes are tested, branches can be merged.
Collaboration: Developers can work on the same project concurrently without overwriting each other’s work.
Integration: Git integrates with other tools like GitHub, CI/CD pipelines, and project management systems.
Core Git Commands with Examples
Initialize a Repository
Creates a new Git repository in the current directory.git init
Clone a Repository
Copies an existing repository to your local machine.git clone <repository-url>
Add Files to Staging Area
Stages changes for the next commit.git add <file>
Commit Changes
Saves changes in the local repository, with a message describing them.git commit -m "Your commit message here"
Check Repository Status
Shows the current state of the working directory and staging area.git status
Pull Changes from Remote
Fetches and merges changes from a remote repository.git pull
Push Changes to Remote
Uploads local commits to a remote repository.git push
Advanced Git Commands and Use Cases
Branching
Branches allow development to occur separately without affecting the main project.git branch <branch-name> # Create a new branch git checkout <branch-name> # Switch to the branch git checkout -b <branch-name> # Create and switch to a branch in one command
Merging Branches
Merges a specified branch into the current one.git merge <branch-name>
Rebase
Rebases one branch onto another, often used for cleaning up commit history.git rebase <base-branch>
Stashing Changes
Temporarily saves changes without committing, ideal for quick switches between tasks.git stash # Stash current changes git stash apply # Apply stashed changes git stash list # List all stashes
Reset and Revert
Reset: Discards changes either in the working directory or staging area.
Revert: Undoes changes from a specific commit while retaining commit history.
git reset --hard <commit-hash> # Resets working directory to a specific commit
git revert <commit-hash> # Creates a new commit to undo specific changes
Cherry-Picking Commits
Selectively applies changes from a specific commit to the current branch.git cherry-pick <commit-hash>
Real-World Scenarios Using Git
Collaborating on a Team Project
For multi-developer projects, each contributor works on separate branches for various parts of a feature. After testing, these branches are merged into the main branch to integrate each developer's work without interference.Handling Hotfixes on a Live Site
When a critical bug is discovered on a live application, a developer can create a hotfix branch from the main branch, apply the necessary fixes, and then deploy it without disrupting ongoing work on new features in other branches.Rolling Back a Buggy Release
If a release contains errors, Git allows rolling back to the previous stable version. Developers can either revert specific buggy commits or reset the repository to an earlier, stable commit.Experimenting with New Features
When testing new features, developers often create isolated branches (e.g.,feature/new-login
) to experiment without impacting the main project. If the feature is successful, it can be merged back.Maintaining a Clean Commit History
In projects with multiple contributors, keeping a clean commit history is essential. Developers can use interactive rebase to condense multiple smaller commits into a single one, which makes the project history more readable.
Tips for Effective Git Workflow
Commit Frequently and Meaningfully
Make regular, descriptive commits. This helps in understanding the history and finding bugs.Use Branches for Isolated Development
Always create a branch for new features or fixes to keep the main codebase stable.Use Git Aliases
Create custom shortcuts for commonly used Git commands to save time.git config --global alias.co checkout git config --global alias.st status
Review and Stage Changes Carefully
Usegit status
andgit diff
to verify staged changes before committing.Leverage Git Remotes
Regularly push local changes to remote repositories like GitHub to safeguard your work and facilitate team collaboration.Properly Configure .gitignore
Use.gitignore
to exclude unnecessary files (e.g., build artifacts, dependencies) from the repository, ensuring the project stays clean and efficient.
Conclusion
Mastering Git helps developers maintain code quality, collaborate seamlessly, and recover from errors swiftly. By implementing Git best practices and understanding both fundamental and advanced commands, you can streamline your development workflow and contribute to more robust projects.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
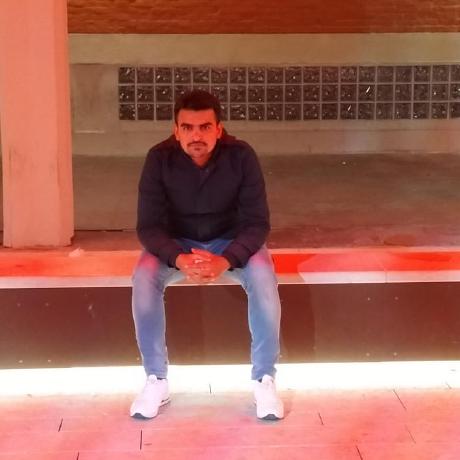
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.