Part 4: Data Flow and State Management Basics
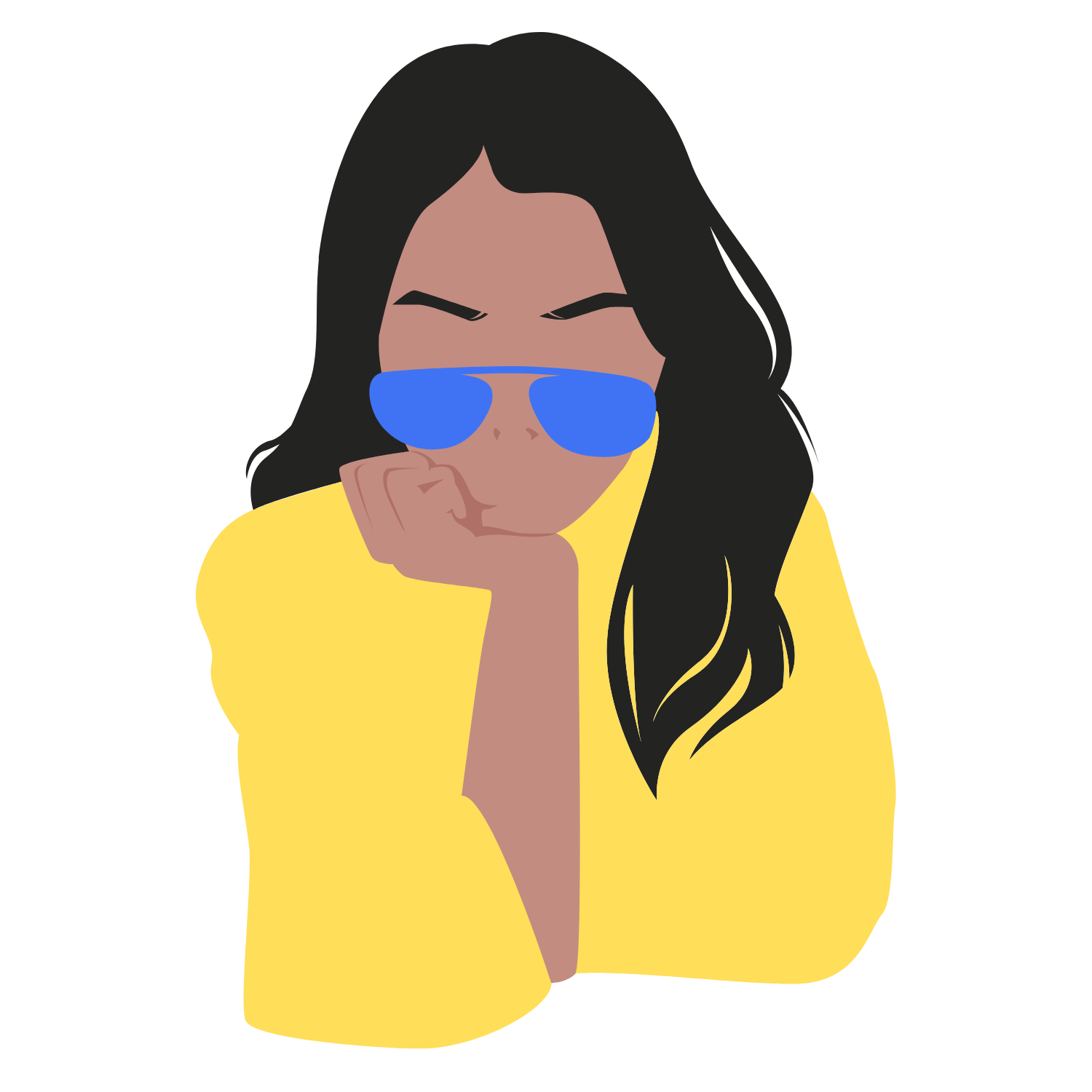
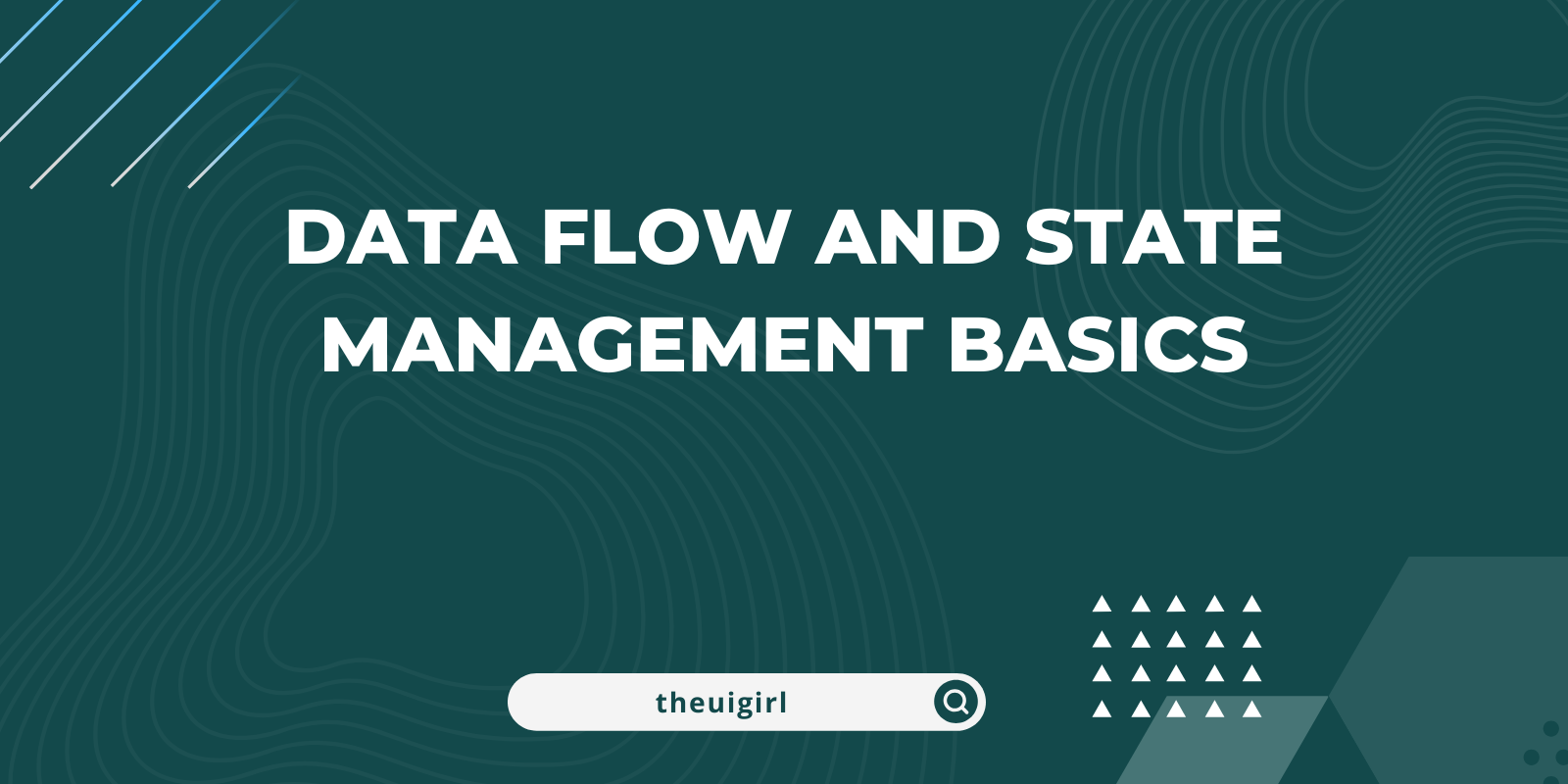
Hello, frontend devs! Today, we’re diving into one of the most important concepts in frontend development: data flow and state management. If you’re working on projects where you need to keep track of user inputs, API data, or any other kind of dynamic information, understanding data flow and state management will make your life much easier.
This guide will introduce you to the basics of data flow in frontend applications, the concept of state, and some simple ways to manage it. We'll focus on React, but these principles can apply to other frameworks as well.
What is "State" in Frontend Development?
State is the current status or condition of an application at a given time. Think of it as data that may change over time. For example, in a to-do list app:
The list of tasks would be part of the app’s state.
Whether a task is marked as "completed" or not would also be part of the state.
Managing this changing data can be tricky as applications grow. That's where state management techniques and tools come into play.
Understanding Data Flow
In most frontend frameworks, data typically flows in a unidirectional way, meaning it goes from parent components to child components. Here’s what that looks like in a React example:
Parent Component: Holds the main state and passes it down as props.
Child Component: Receives the state (or parts of it) via props and can use it to display data or trigger functions that update the parent’s state.
This is sometimes called top-down or one-way data flow.
Setting Up State with React’s useState
React provides a built-in hook called useState
for managing local state in functional components. Let’s see how it works in a simple counter app.
import React, { useState } from 'react';
function Counter() {
// Declaring state using useState
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
return (
<div>
<h1>Counter: {count}</h1>
<button onClick={increment}>Increase</button>
</div>
);
}
export default Counter;
Explanation:
useState(0)
creates a state variable calledcount
, initialized to 0, and a functionsetCount
to update it.When the button is clicked,
increment
is called, which updates the count by 1.
This is a very basic example of state management for a single component. However, in a larger app, you’ll often need to manage state across multiple components.
Passing State to Child Components
Let's say you have a parent component that keeps track of a username and passes it down to a child component to display it.
// ParentComponent.js
import React, { useState } from 'react';
import ChildComponent from './ChildComponent';
function ParentComponent() {
const [username, setUsername] = useState("John Doe");
return (
<div>
<h1>Welcome, {username}</h1>
<ChildComponent username={username} />
</div>
);
}
export default ParentComponent;
// ChildComponent.js
import React from 'react';
function ChildComponent({ username }) {
return <p>Hello, {username}! Nice to see you here.</p>;
}
export default ChildComponent;
Here, ParentComponent
passes username
as a prop to ChildComponent
, which receives it as a parameter and displays it.
Note on Data Flow:
Data flows down from
ParentComponent
toChildComponent
.ChildComponent
can use the data but cannot change it directly.
Introducing State Management Tools: React Context API
In more complex applications, you might have state that many components need to access. Passing props down through multiple components is known as "prop drilling" and can become messy. This is where the React Context API can help.
The Context API allows us to create a global state that any component can access, no matter where it is in the component tree.
Setting Up Context for Global State
Create a Context: Define a context and a provider component.
Wrap Your Components with the Provider: So they have access to the context.
Use the Context: In any component that needs access to the state.
Let’s see this in action with a theme toggle example:
// ThemeContext.js
import React, { createContext, useState } from 'react';
export const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState("light");
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === "light" ? "dark" : "light"));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
Explanation:
ThemeContext
holds the theme state andtoggleTheme
function.ThemeProvider
is a wrapper component that makes the theme data available to all children components.
Using Context in a Component
To use this context in a component, use the useContext
hook:
// App.js
import React, { useContext } from 'react';
import { ThemeContext, ThemeProvider } from './ThemeContext';
function ThemeButton() {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div>
<button onClick={toggleTheme}>
Switch to {theme === "light" ? "dark" : "light"} theme
</button>
</div>
);
}
function App() {
return (
<ThemeProvider>
<div className="App">
<h1>Welcome to the App</h1>
<ThemeButton />
</div>
</ThemeProvider>
);
}
export default App;
In this setup:
The
ThemeProvider
wraps the entire app, allowing any child component to access thetheme
state andtoggleTheme
function.ThemeButton
usesuseContext(ThemeContext)
to access and update the theme without prop drilling.
Key Takeaways
Local State: Use
useState
to manage component-specific data.Prop Drilling: Pass data from parent to child as props, but beware of passing data through too many layers.
Global State: For larger apps, use the React Context API or another state management tool to manage state across multiple components.
Understanding these concepts will lay a solid foundation for building scalable and maintainable frontend applications. Happy coding!
Subscribe to my newsletter
Read articles from Anisha Swain | The UI Girl directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
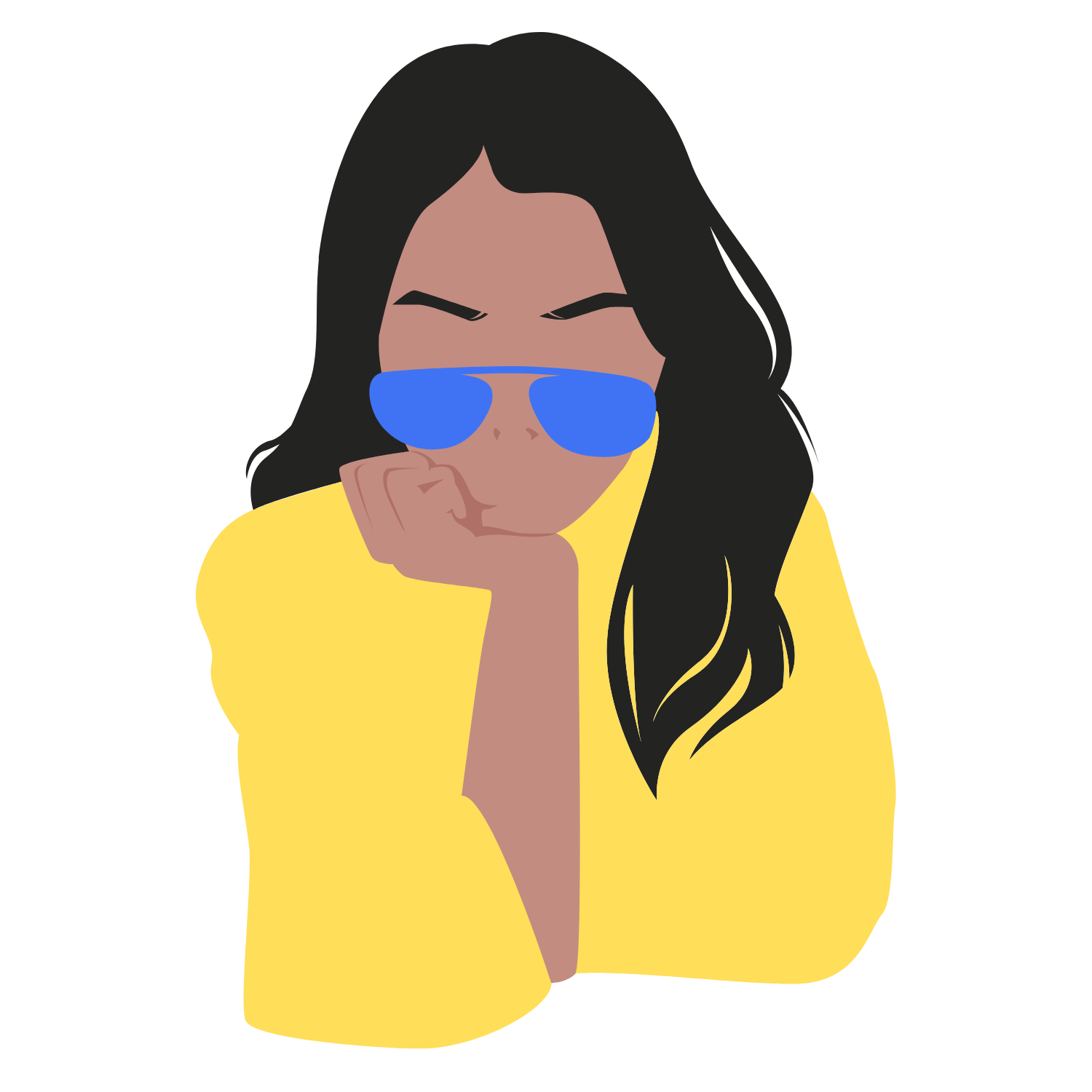
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl Hello world! Anisha this side👋 💪Making @theuigirl 💻 speaker http://t.ly/9D22 🍀 1:1 https://topmate.io/anishaswain 🎙️ podcast host http://t.ly/_MUml ✏️ blog https://medium.com/the-ui-girl 🧳 Travel story http://t.ly/Xa-5v https://bento.me/anishaswain Let's connect 🤝