BeautifulSoup with Sample Output
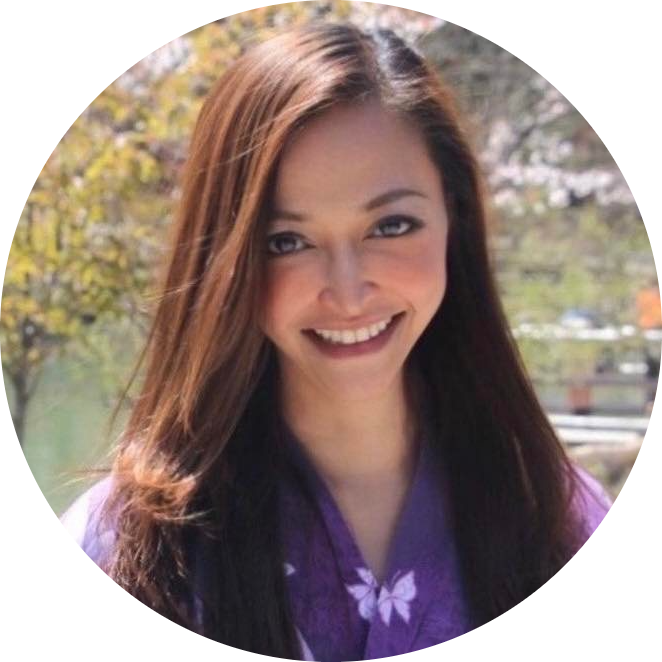
Table of contents
- Introduction to Web Scraping with BeautifulSoup
- Using BeautifulSoup to Parse HTML
- Understanding the DOM (Document Object Model)
- Setting Up Python for Web Scraping
- Basics of BeautifulSoup
- Extracting Data with BeautifulSoup
- Advanced BeautifulSoup Techniques
- Pagination and Multi-Page Scraping
- Handling Forms and Logins
- 1. Understanding Web Forms (Inputs, Buttons, Hidden Fields)
- 2. Submitting Forms with requests
- 3. Handling Authentication (Cookies, Sessions, and Headers)
- 4. Handling Headers and CSRF Tokens
- Key Tips for Handling Forms and Logins
- Scraping Job Listings
- 1. Analyzing Job Websites (Structure and Patterns)
- 2. Extracting Job Titles, Companies, Locations, and Descriptions
- 3. Extracting Links and Handling Redirections
- 4. Handling Structured and Unstructured Job Data
- 5. Putting It All Together: Multi-Page Job Scraper
- Tips for Scraping Job Listings
- Data Cleaning and Storage
- Handling Dynamic and JavaScript-Rendered Content
- Testing and Debugging Scrapers
- Automating Scraping for Job Websites
- Deploying Scrapers
- Ethical and Scalable Scraping
Introduction to Web Scraping with BeautifulSoup
Using BeautifulSoup to Parse HTML
Here’s how you can fetch and parse a webpage using requests
and BeautifulSoup
:
from bs4 import BeautifulSoup
import requests
# Step 1: Fetch the HTML content
url = "https://quotes.toscrape.com/"
response = requests.get(url)
if response.status_code == 200:
# Step 2: Parse the HTML content with BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
# Step 3: Extract the page title
print("Page Title:", soup.title.string)
# Step 4: Extract and display the first quote on the page
first_quote = soup.find('span', class_='text').text
print("First Quote:", first_quote)
else:
print(f"Failed to fetch the page, Status Code: {response.status_code}")
Sample Output:
Page Title: Quotes to Scrape
First Quote: “The world as we have created it is a process of our thinking. It cannot be changed without changing our thinking.”
Extracting Specific HTML Elements
BeautifulSoup allows you to locate and extract specific elements using tags.
Example: Extracting Links (<a>
tags):
from bs4 import BeautifulSoup
import requests
url = "https://example.com"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# Find all <a> tags and print their href attributes
links = soup.find_all('a')
for link in links:
print("Link Text:", link.text)
print("Link URL:", link['href'])
else:
print(f"Failed to fetch the page, Status Code: {response.status_code}")
Sample Output:
Link Text: More information...
Link URL: https://www.iana.org/domains/example
Extracting Text Content
You can extract text from tags using .text
or .get_text()
.
Example: Extracting Main Content Text:
from bs4 import BeautifulSoup
import requests
url = "https://example.com"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
# Extracting the text inside the <p> tag
paragraph = soup.find('p').get_text()
print("Paragraph Text:", paragraph)
else:
print(f"Failed to fetch the page, Status Code: {response.status_code}")
Sample Output:
Paragraph Text: This domain is for use in illustrative examples in documents.
Combining BeautifulSoup with robots.txt
You can combine BeautifulSoup and requests to inspect the robots.txt
file of a website to check scraping permissions.
Example: Checking robots.txt
with BeautifulSoup:
import requests
from bs4 import BeautifulSoup
url = "https://example.com/robots.txt"
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'html.parser')
print("Robots.txt Content:")
print(soup.get_text())
else:
print(f"Could not retrieve robots.txt, Status Code: {response.status_code}")
Sample Output:
Robots.txt Content:
User-agent: *
Disallow: /private/
Disallow: /admin/
Allow: /
Why BeautifulSoup is Powerful for Web Scraping
Ease of Use: Simple methods to navigate and extract HTML content.
Flexible Parsers: Use
html.parser
,lxml
, or other parsers for different needs.Integration: Works seamlessly with
requests
and other Python libraries.Dynamic Queries: Search elements using tags, attributes, and text.
Introduction to HTTP and HTML
Let’s go block by block, with code and examples wherever applicable.
1. Basics of HTTP (Requests, Responses, Status Codes)
HTTP (Hypertext Transfer Protocol) is the foundation of web communication. When scraping, we interact with websites using requests and handle responses.
Key Concepts:
Request: Sent to a server to fetch resources (e.g., HTML, JSON, or images).
Response: The server’s reply to your request, containing the resource and metadata (status, headers).
Example: Using Python to Make HTTP Requests
import requests
url = "https://example.com"
response = requests.get(url)
print("Status Code:", response.status_code) # 200 means success
print("Headers:", response.headers) # Metadata about the response
print("First 100 Characters of Content:", response.text[:100])
Sample Output:
Status Code: 200
Headers: {'Content-Type': 'text/html; charset=UTF-8', ...}
First 100 Characters of Content: <!doctype html><html><head><title>Example Domain</title>...
Common HTTP Status Codes:
200 OK: Request succeeded.
301 Moved Permanently: Resource moved to a new URL.
403 Forbidden: Access denied.
404 Not Found: Resource not found.
500 Internal Server Error: Server-side issue.
Understanding the DOM (Document Object Model)
The DOM is a hierarchical representation of an HTML document. It allows us to interact with elements programmatically.
Example HTML Structure:
<!DOCTYPE html>
<html>
<head>
<title>Example Page</title>
</head>
<body>
<h1>Welcome to Web Scraping</h1>
<p id="intro">Learn to scrape data from the web.</p>
<a href="https://example.com/contact">Contact Us</a>
</body>
</html>
Key Concepts:
Nodes: Each HTML element is a node in the DOM tree (e.g.,
<html>
,<head>
,<body>
).Attributes: Elements can have attributes (e.g.,
id
,class
,href
).Text Nodes: Contain visible content (e.g., "Learn to scrape data from the web.").
Navigating the DOM with BeautifulSoup:
from bs4 import BeautifulSoup
html_content = """
<!DOCTYPE html>
<html>
<head>
<title>Example Page</title>
</head>
<body>
<h1>Welcome to Web Scraping</h1>
<p id="intro">Learn to scrape data from the web.</p>
<a href="https://example.com/contact">Contact Us</a>
</body>
</html>
"""
soup = BeautifulSoup(html_content, 'html.parser')
print("Title:", soup.title.string) # Accessing <title>
print("Header:", soup.h1.string) # Accessing <h1>
print("Paragraph:", soup.find('p').string) # Accessing <p>
print("Link URL:", soup.a['href']) # Accessing <a href>
Sample Output:
Title: Example Page
Header: Welcome to Web Scraping
Paragraph: Learn to scrape data from the web.
Link URL: https://example.com/contact
3. Common HTML Elements Used in Scraping
Here are the most commonly used tags when scraping:
<div>
: Used as a container for content.<span>
: Inline content.<a>
: Hyperlinks.<ul>
and<li>
: Lists.<table>
,<tr>
,<td>
: Tabular data.<form>
and<input>
: Web forms for search or login.
Example: Extracting Job Listings
<div class="job-listing">
<h2>Software Engineer</h2>
<span>Company: ExampleCorp</span>
<a href="/apply">Apply Here</a>
</div>
Using BeautifulSoup:
from bs4 import BeautifulSoup
html_content = """
<div class="job-listing">
<h2>Software Engineer</h2>
<span>Company: ExampleCorp</span>
<a href="/apply">Apply Here</a>
</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Extract data
job_title = soup.find('h2').string
company_name = soup.find('span').string
apply_link = soup.find('a')['href']
print(f"Job Title: {job_title}")
print(f"Company: {company_name}")
print(f"Application Link: {apply_link}")
Sample Output:
Job Title: Software Engineer
Company: Company: ExampleCorp
Application Link: /apply
4. Tools to Inspect Web Pages
Chrome Developer Tools
You can use Chrome’s DevTools to inspect a web page and understand its structure.
Steps:
Right-click on a webpage and select "Inspect".
Use the Elements tab to view the DOM structure.
Hover over elements to locate their corresponding tags in the DOM.
Copy CSS selectors or XPath for elements:
Right-click an element in the DOM panel.
Choose "Copy" > "Copy selector".
Example: Inspecting an Element Inspecting a job title on a website might reveal this structure:
<div class="job-card">
<h2 class="job-title">Data Scientist</h2>
<span class="company-name">TechCorp</span>
</div>
Extracting Job Title and Company Using DevTools:
from bs4 import BeautifulSoup
html_content = """
<div class="job-card">
<h2 class="job-title">Data Scientist</h2>
<span class="company-name">TechCorp</span>
</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
job_title = soup.select_one('.job-title').string # CSS Selector
company_name = soup.select_one('.company-name').string
print(f"Job Title: {job_title}")
print(f"Company: {company_name}")
Sample Output:
Job Title: Data Scientist
Company: TechCorp
Setting Up Python for Web Scraping
1. Installing Necessary Libraries
To get started with web scraping, you need a few essential libraries:
requests
: For sending HTTP requests to websites.beautifulsoup4
: For parsing and extracting data from HTML/XML.lxml
: A fast parser for BeautifulSoup.pandas
: For organizing scraped data into structured formats like CSV or Excel.
Install these libraries:
pip install requests beautifulsoup4 lxml pandas
Sample Output:
Successfully installed requests beautifulsoup4 lxml pandas
2. Setting Up a Virtual Environment (Optional but Recommended)
Virtual environments allow you to manage dependencies for each project independently, avoiding conflicts.
Step 1: Create a Virtual Environment
python -m venv webscraping_env
Step 2: Activate the Virtual Environment
On Windows:
webscraping_env\Scripts\activate
On macOS/Linux:
source webscraping_env/bin/activate
Step 3: Install Required Libraries in the Virtual Environment
pip install requests beautifulsoup4 lxml pandas
Deactivate the Environment
deactivate
3. Writing Your First Web Scraper
Here’s a simple web scraper that fetches and parses data from a webpage.
Goal: Extract the title and a paragraph from example.com.
Code:
from bs4 import BeautifulSoup
import requests
# Step 1: Fetch the web page
url = "https://example.com"
response = requests.get(url)
# Step 2: Check the response status
if response.status_code == 200:
print("Successfully fetched the page!")
else:
print(f"Failed to fetch the page. Status Code: {response.status_code}")
exit()
# Step 3: Parse the HTML content
soup = BeautifulSoup(response.text, 'html.parser')
# Step 4: Extract specific data
title = soup.title.string # Extract the title of the page
paragraph = soup.find('p').get_text() # Extract the first paragraph
# Step 5: Print the extracted data
print("Page Title:", title)
print("First Paragraph:", paragraph)
Sample Output:
Successfully fetched the page!
Page Title: Example Domain
First Paragraph: This domain is for use in illustrative examples in documents.
4. Saving the Scraped Data
You can save the extracted data to a CSV file for later use.
Code:
import pandas as pd
# Example scraped data
data = {
"Title": [title],
"Paragraph": [paragraph],
}
# Save to CSV
df = pd.DataFrame(data)
df.to_csv("scraped_data.csv", index=False)
print("Data saved to scraped_data.csv")
Sample Output:
Data saved to scraped_data.csv
Basics of BeautifulSoup
Let’s break it down step by step with code and examples.
1. Installing BeautifulSoup
The beautifulsoup4
library provides tools for parsing HTML and XML.
Installation:
pip install beautifulsoup4 lxml
Check Installation:
pip show beautifulsoup4
2. Creating a BeautifulSoup
Object
To use BeautifulSoup, you need to create an object from the HTML content you want to parse.
Example:
from bs4 import BeautifulSoup
html_content = """
<!DOCTYPE html>
<html>
<head><title>Sample Page</title></head>
<body>
<h1>Hello, BeautifulSoup!</h1>
<p>This is an example paragraph.</p>
</body>
</html>
"""
# Create a BeautifulSoup object
soup = BeautifulSoup(html_content, 'html.parser')
# Print the parsed content
print(soup.prettify())
Sample Output:
<!DOCTYPE html>
<html>
<head>
<title>
Sample Page
</title>
</head>
<body>
<h1>
Hello, BeautifulSoup!
</h1>
<p>
This is an example paragraph.
</p>
</body>
</html>
3. Parsing HTML (Parsers: html.parser
, lxml
, etc.)
BeautifulSoup supports multiple parsers. The most common ones are:
html.parser
: Built-in parser, slower but requires no extra installation.lxml
: Faster, requires installing thelxml
library.
Example: Comparing Parsers
from bs4 import BeautifulSoup
html_content = "<html><body><h1>Hello, Parser!</h1></body></html>"
# Using html.parser
soup_html_parser = BeautifulSoup(html_content, 'html.parser')
print("Using html.parser:", soup_html_parser.h1.string)
# Using lxml
soup_lxml = BeautifulSoup(html_content, 'lxml')
print("Using lxml:", soup_lxml.h1.string)
Sample Output:
Using html.parser: Hello, Parser!
Using lxml: Hello, Parser!
When to Use lxml
:
When speed is critical.
When dealing with poorly formatted HTML.
4. Navigating the HTML Tree
BeautifulSoup allows you to traverse the HTML structure using parent, children, and siblings.
HTML Structure Example:
<html>
<body>
<div id="main">
<h1>Welcome!</h1>
<p>This is a sample paragraph.</p>
<p>Another paragraph.</p>
</div>
</body>
</html>
Example Code:
from bs4 import BeautifulSoup
html_content = """
<html>
<body>
<div id="main">
<h1>Welcome!</h1>
<p>This is a sample paragraph.</p>
<p>Another paragraph.</p>
</div>
</body>
</html>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Accessing parent
h1_tag = soup.h1
print("Parent of <h1>:", h1_tag.parent.name)
# Accessing children
div_tag = soup.find('div', id='main')
print("Children of <div>:", [child.name for child in div_tag.children if child.name])
# Accessing siblings
first_p = soup.find('p')
print("Next Sibling:", first_p.find_next_sibling('p').string)
Sample Output:
Parent of <h1>: div
Children of <div>: ['h1', 'p', 'p']
Next Sibling: Another paragraph.
Key Methods for Navigation
.parent
: Accesses the parent of an element..children
: Returns all children of an element as an iterator..find_next_sibling()
: Finds the next sibling of an element..find_previous_sibling()
: Finds the previous sibling of an element.
Extracting Data with BeautifulSoup
Let’s explore how to extract specific data using BeautifulSoup, with examples for each method.
1. Finding Elements (find
, find_all
)
find
: Returns the first matching element.find_all
: Returns a list of all matching elements.
Example HTML:
<html>
<body>
<h1>Main Title</h1>
<div class="content">
<p id="intro">Introduction paragraph.</p>
<p id="info">Additional information.</p>
</div>
</body>
</html>
Code Example:
from bs4 import BeautifulSoup
html_content = """
<html>
<body>
<h1>Main Title</h1>
<div class="content">
<p id="intro">Introduction paragraph.</p>
<p id="info">Additional information.</p>
</div>
</body>
</html>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Find the first <p> tag
first_p = soup.find('p')
print("First <p> Tag:", first_p)
# Find all <p> tags
all_p = soup.find_all('p')
print("All <p> Tags:", all_p)
Sample Output:
First <p> Tag: <p id="intro">Introduction paragraph.</p>
All <p> Tags: [<p id="intro">Introduction paragraph.</p>, <p id="info">Additional information.</p>]
2. Using CSS Selectors (select
, select_one
)
CSS selectors are a powerful way to locate elements based on IDs, classes, or tag relationships.
select_one
: Returns the first matching element.select
: Returns a list of all matching elements.
Example Code:
# Select elements with CSS selectors
intro_paragraph = soup.select_one('#intro') # By ID
all_paragraphs = soup.select('.content p') # All <p> inside class "content"
print("Intro Paragraph:", intro_paragraph.text)
print("All Paragraphs in .content:", [p.text for p in all_paragraphs])
Sample Output:
Intro Paragraph: Introduction paragraph.
All Paragraphs in .content: ['Introduction paragraph.', 'Additional information.']
3. Extracting Attributes (get
, attrs
)
You can extract specific attributes (like id
, href
, src
) from HTML elements.
Example HTML:
<a href="https://example.com" id="link">Visit Example</a>
Code Example:
html_content = '<a href="https://example.com" id="link">Visit Example</a>'
soup = BeautifulSoup(html_content, 'html.parser')
# Extracting attributes
link_tag = soup.find('a')
href_value = link_tag.get('href') # Extract href attribute
id_value = link_tag.attrs['id'] # Extract id attribute
print("Href:", href_value)
print("ID:", id_value)
Sample Output:
Href: https://example.com
ID: link
4. Extracting Text (.text
, .get_text()
)
Extract the visible text content of an element using .text
or .get_text()
.
Example HTML:
<div class="text-block">
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
Code Example:
html_content = """
<div class="text-block">
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Extract text from a single element
first_paragraph = soup.find('p').text
print("First Paragraph:", first_paragraph)
# Extract text from all <p> elements
all_text = soup.get_text()
print("All Text:", all_text)
Sample Output:
First Paragraph: Paragraph 1
All Text:
Paragraph 1
Paragraph 2
Summary of Methods
Method | Description | Example |
find | Finds the first matching element | soup.find('p') |
find_all | Finds all matching elements | soup.find_all('p') |
select_one | Finds the first element matching CSS selector | soup.select _one('.class_name') |
select | Finds all elements matching CSS selector | soup.select ('.class_name') |
.get(attr) | Gets the value of a specific attribute | tag.get('href') |
.attrs | Returns all attributes as a dictionary | tag.attrs['id'] |
.text /.get_text() | Extracts text content | tag.text / tag.get_text() |
Advanced BeautifulSoup Techniques
1. Searching with Regular Expressions
BeautifulSoup supports searching for elements using Python's re
(regular expression) module. This is useful when you need to match patterns in tag names, attributes, or text content.
Example HTML:
<div class="item">Item 1</div>
<div class="product">Product 2</div>
<div class="item">Item 3</div>
Code Example:
from bs4 import BeautifulSoup
import re
html_content = """
<div class="item">Item 1</div>
<div class="product">Product 2</div>
<div class="item">Item 3</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Find all <div> tags with class starting with "item" or "product"
matching_divs = soup.find_all('div', class_=re.compile(r'^(item|product)'))
for div in matching_divs:
print("Content:", div.text)
Sample Output:
Content: Item 1
Content: Product 2
Content: Item 3
2. Using Filters and Lambda Functions
Filters and lambda functions provide flexible ways to locate elements with specific conditions.
Example HTML:
<ul>
<li data-id="101">Apple</li>
<li data-id="102">Banana</li>
<li data-id="103">Cherry</li>
</ul>
Code Example:
html_content = """
<ul>
<li data-id="101">Apple</li>
<li data-id="102">Banana</li>
<li data-id="103">Cherry</li>
</ul>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Find <li> elements where data-id > 101
filtered_items = soup.find_all('li', attrs=lambda tag: int(tag['data-id']) > 101)
for item in filtered_items:
print("Item:", item.text)
Sample Output:
Item: Banana
Item: Cherry
3. Handling Dynamic Content (JavaScript-Rendered Pages)
BeautifulSoup cannot scrape content generated dynamically by JavaScript. For such pages, use Selenium to render the page and pass the HTML to BeautifulSoup.
Example: Using Selenium with BeautifulSoup
from selenium import webdriver
from bs4 import BeautifulSoup
# Set up Selenium WebDriver
driver = webdriver.Chrome()
# Load the page
driver.get('https://example.com')
# Get the rendered HTML
html = driver.page_source
driver.quit()
# Parse with BeautifulSoup
soup = BeautifulSoup(html, 'html.parser')
print("Page Title:", soup.title.string)
Note: You need to install Selenium and a browser driver like ChromeDriver.
4. Handling Large HTML Documents Efficiently
For large documents, use BeautifulSoup’s SoupStrainer
to parse only the parts of the document you need.
Example HTML:
<div>
<h1>Main Header</h1>
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
Code Example:
from bs4 import BeautifulSoup, SoupStrainer
html_content = """
<div>
<h1>Main Header</h1>
<p>Paragraph 1</p>
<p>Paragraph 2</p>
</div>
"""
# Use SoupStrainer to extract only <p> tags
only_p_tags = SoupStrainer('p')
# Parse with BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser', parse_only=only_p_tags)
for p in soup:
print("Paragraph:", p.get_text())
Sample Output:
Paragraph: Paragraph 1
Paragraph: Paragraph 2
Summary of Techniques
Technique | Description | Use Case |
Regular Expressions | Match patterns in tag names, attributes, or text | Finding elements with partial or dynamic values. |
Filters and Lambda | Apply custom logic to search for elements | Complex attribute-based filtering. |
Handling Dynamic Content | Use Selenium to handle JavaScript-rendered pages | Scraping SPAs (e.g., React, Angular). |
SoupStrainer | Parse specific parts of a large HTML document | Speed up parsing by limiting data processing. |
Pagination and Multi-Page Scraping
1. Identifying Pagination Patterns
Pagination is often implemented using links or query parameters. Common patterns include:
Links with
page
numbers in the URL:
https://example.com/jobs?page=1
Relative links:
<a href="/jobs?page=2">Next Page</a>
Infinite scrolling: Requires JavaScript (use Selenium).
Example HTML:
<div class="pagination">
<a href="/jobs?page=1">1</a>
<a href="/jobs?page=2">2</a>
<a href="/jobs?page=3">3</a>
</div>
Code Example: Identifying Patterns
from bs4 import BeautifulSoup
html_content = """
<div class="pagination">
<a href="/jobs?page=1">1</a>
<a href="/jobs?page=2">2</a>
<a href="/jobs?page=3">3</a>
</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Extract all page links
pagination_links = [a['href'] for a in soup.find_all('a')]
print("Pagination Links:", pagination_links)
Sample Output:
Pagination Links: ['/jobs?page=1', '/jobs?page=2', '/jobs?page=3']
2. Scraping Multiple Pages with Loops
To scrape multiple pages, iterate through the page numbers or extracted links.
Code Example: Scraping a Paginated Website
import requests
from bs4 import BeautifulSoup
base_url = "https://example.com/jobs"
for page in range(1, 4): # Example: 3 pages
url = f"{base_url}?page={page}"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract job titles (assume jobs are in <h2> tags)
job_titles = [h2.text for h2 in soup.find_all('h2')]
print(f"Page {page} Job Titles:", job_titles)
Sample Output:
Page 1 Job Titles: ['Job A', 'Job B']
Page 2 Job Titles: ['Job C', 'Job D']
Page 3 Job Titles: ['Job E', 'Job F']
3. Handling Relative and Absolute URLs
Relative URLs (e.g., /jobs?page=2
) need to be converted to absolute URLs using the base URL.
Code Example: Converting Relative URLs
from urllib.parse import urljoin
base_url = "https://example.com"
relative_url = "/jobs?page=2"
absolute_url = urljoin(base_url, relative_url)
print("Absolute URL:", absolute_url)
Sample Output:
Absolute URL: https://example.com/jobs?page=2
Implementation in Pagination:
pagination_links = [urljoin(base_url, link) for link in pagination_links]
print("Full Links:", pagination_links)
4. Managing Delays to Avoid Bans (Rate Limiting)
To avoid being blocked, respect the website’s server by:
Adding delays between requests.
Rotating user agents and IP addresses (using proxies).
Code Example: Adding Delays
import time
import requests
from bs4 import BeautifulSoup
base_url = "https://example.com/jobs"
for page in range(1, 4):
url = f"{base_url}?page={page}"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(f"Scraped Page {page}")
# Delay between requests
time.sleep(2) # Wait for 2 seconds
Sample Output:
Scraped Page 1
Scraped Page 2
Scraped Page 3
5. Handling Infinite Scrolling
If the website uses infinite scrolling (loads data via JavaScript), use Selenium to scroll and load additional content.
Code Example: Selenium for Infinite Scrolling
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
from bs4 import BeautifulSoup
driver = webdriver.Chrome()
driver.get("https://example.com/jobs")
# Scroll down to load more content
for _ in range(3): # Adjust for the number of scrolls
driver.find_element(By.TAG_NAME, 'body').send_keys(Keys.END)
time.sleep(2) # Wait for content to load
# Parse loaded content with BeautifulSoup
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
# Extract job titles
job_titles = [h2.text for h2 in soup.find_all('h2')]
print("Job Titles:", job_titles)
driver.quit()
Key Tips for Pagination
Use delays: Always respect server load.
Check robots.txt: Ensure scraping complies with the website’s rules.
Rotate headers/IPs: Use tools like
fake-useragent
and proxy services to avoid detection.
Handling Forms and Logins
1. Understanding Web Forms (Inputs, Buttons, Hidden Fields)
Web forms typically consist of <input>
fields, <button>
tags, and often hidden fields for additional data.
Example HTML Form:
<form action="/login" method="POST">
<input type="text" name="username" placeholder="Enter username">
<input type="password" name="password" placeholder="Enter password">
<input type="hidden" name="csrf_token" value="123456789">
<button type="submit">Login</button>
</form>
Key Components:
Action: The URL where the form is submitted (
/login
in the example above).Method: HTTP method (
POST
for sensitive data).Inputs: Fields for data entry.
Hidden Fields: Invisible fields often used for security (e.g., CSRF tokens).
To scrape and submit such forms, we first inspect the form fields.
Using BeautifulSoup to Inspect Form Fields:
from bs4 import BeautifulSoup
html_content = """
<form action="/login" method="POST">
<input type="text" name="username" placeholder="Enter username">
<input type="password" name="password" placeholder="Enter password">
<input type="hidden" name="csrf_token" value="123456789">
<button type="submit">Login</button>
</form>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Extract all input fields
inputs = soup.find_all('input')
for input_tag in inputs:
print(f"Name: {input_tag.get('name')}, Type: {input_tag.get('type')}, Value: {input_tag.get('value')}")
Sample Output:
Name: username, Type: text, Value: None
Name: password, Type: password, Value: None
Name: csrf_token, Type: hidden, Value: 123456789
2. Submitting Forms with requests
Once the form fields are identified, we can use the requests
library to submit the form programmatically.
Code Example: Submitting a Login Form
import requests
# Step 1: Define form data
form_data = {
"username": "myusername",
"password": "mypassword",
"csrf_token": "123456789", # Hidden field value
}
# Step 2: Submit the form
url = "https://example.com/login"
response = requests.post(url, data=form_data)
# Step 3: Check the response
if response.status_code == 200:
print("Login successful!")
print("Response:", response.text[:200]) # Show first 200 characters of the response
else:
print(f"Login failed. Status Code: {response.status_code}")
Sample Output:
Login successful!
Response: <html>...Welcome, myusername...</html>
3. Handling Authentication (Cookies, Sessions, and Headers)
To maintain an authenticated session after login, you need to handle cookies and use a session object.
Using a requests.Session
A session object allows you to persist cookies across requests, making it ideal for handling logins.
Code Example: Using Sessions for Login and Authenticated Requests
import requests
# Step 1: Create a session
session = requests.Session()
# Step 2: Login
login_url = "https://example.com/login"
form_data = {
"username": "myusername",
"password": "mypassword",
}
response = session.post(login_url, data=form_data)
if response.status_code == 200:
print("Logged in successfully!")
else:
print(f"Login failed. Status Code: {response.status_code}")
exit()
# Step 3: Make an authenticated request
dashboard_url = "https://example.com/dashboard"
response = session.get(dashboard_url)
print("Dashboard Response:", response.text[:200]) # Display part of the dashboard page
4. Handling Headers and CSRF Tokens
Some forms may require custom headers (e.g., User-Agent) or dynamically fetched CSRF tokens.
Dynamically Fetching CSRF Tokens
Code Example:
from bs4 import BeautifulSoup
import requests
# Step 1: Fetch the login page to extract CSRF token
login_page_url = "https://example.com/login"
response = requests.get(login_page_url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract CSRF token from the hidden input field
csrf_token = soup.find('input', {'name': 'csrf_token'})['value']
# Step 2: Submit login form with the token
form_data = {
"username": "myusername",
"password": "mypassword",
"csrf_token": csrf_token,
}
login_url = "https://example.com/login"
response = requests.post(login_url, data=form_data)
if response.status_code == 200:
print("Logged in successfully with CSRF token!")
Key Tips for Handling Forms and Logins
Inspect Forms Using DevTools:
- Use Chrome DevTools to locate form fields, hidden inputs, and headers.
Use Sessions:
- Always use
requests.Session
for login flows to persist cookies.
- Always use
Dynamic CSRF Tokens:
- Scrape and include CSRF tokens or other hidden fields dynamically.
Headers:
- Add custom headers like
User-Agent
to mimic browser behavior.
- Add custom headers like
Scraping Job Listings
1. Analyzing Job Websites (Structure and Patterns)
To scrape job websites effectively:
Inspect the HTML Structure: Use browser dev tools (e.g., Chrome DevTools) to locate job titles, companies, locations, and links.
Identify Patterns: Check for repeating structures like
<div>
or<li>
containers for each job.Pagination: Look for links or parameters for navigating multiple pages.
Example HTML:
<div class="job-card">
<h2 class="job-title">Software Engineer</h2>
<span class="company">TechCorp</span>
<span class="location">San Francisco, CA</span>
<a class="job-link" href="/job/software-engineer">View Job</a>
</div>
2. Extracting Job Titles, Companies, Locations, and Descriptions
Use BeautifulSoup to extract specific elements.
Code Example: Extracting Job Listings
from bs4 import BeautifulSoup
html_content = """
<div class="job-card">
<h2 class="job-title">Software Engineer</h2>
<span class="company">TechCorp</span>
<span class="location">San Francisco, CA</span>
<a class="job-link" href="/job/software-engineer">View Job</a>
</div>
<div class="job-card">
<h2 class="job-title">Data Scientist</h2>
<span class="company">DataCorp</span>
<span class="location">New York, NY</span>
<a class="job-link" href="/job/data-scientist">View Job</a>
</div>
"""
soup = BeautifulSoup(html_content, 'html.parser')
# Find all job cards
job_cards = soup.find_all('div', class_='job-card')
# Extract job details
for job in job_cards:
title = job.find('h2', class_='job-title').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
link = job.find('a', class_='job-link')['href']
print(f"Title: {title}, Company: {company}, Location: {location}, Link: {link}")
Sample Output:
Title: Software Engineer, Company: TechCorp, Location: San Francisco, CA, Link: /job/software-engineer
Title: Data Scientist, Company: DataCorp, Location: New York, NY, Link: /job/data-scientist
3. Extracting Links and Handling Redirections
Links are often relative, and you’ll need to convert them to absolute URLs.
Using urllib.parse.urljoin
:
from urllib.parse import urljoin
base_url = "https://example.com"
relative_link = "/job/software-engineer"
absolute_url = urljoin(base_url, relative_link)
print("Absolute URL:", absolute_url)
Sample Output:
Absolute URL: https://example.com/job/software-engineer
Integrating into the Scraper:
base_url = "https://example.com"
for job in job_cards:
link = job.find('a', class_='job-link')['href']
absolute_url = urljoin(base_url, link)
print(f"Job Link: {absolute_url}")
4. Handling Structured and Unstructured Job Data
Structured Data: Jobs with consistent patterns, e.g.,
<div>
with classes for titles, companies, etc.Unstructured Data: Varying HTML structures that require more flexible extraction methods (e.g., regex or fuzzy matching).
Structured Data
# Example of structured extraction
title = job.find('h2', class_='job-title').text
company = job.find('span', class_='company').text
Unstructured Data (Fallbacks and Defaults)
Code Example:
# Use .get() with default values for unstructured data
title = job.find('h2', class_='job-title').get_text(strip=True) if job.find('h2', class_='job-title') else "N/A"
description = job.find('p', class_='description').get_text(strip=True) if job.find('p', class_='description') else "No description available"
print(f"Title: {title}, Description: {description}")
Handling Missing Attributes:
link = job.find('a', class_='job-link')
link_url = link['href'] if link and 'href' in link.attrs else "No link available"
print("Link:", link_url)
5. Putting It All Together: Multi-Page Job Scraper
Code Example:
import requests
from bs4 import BeautifulSoup
from urllib.parse import urljoin
import time
base_url = "https://example.com/jobs"
headers = {'User-Agent': 'Mozilla/5.0'}
# Scrape multiple pages
for page in range(1, 4): # Example: 3 pages
url = f"{base_url}?page={page}"
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
job_cards = soup.find_all('div', class_='job-card')
for job in job_cards:
title = job.find('h2', class_='job-title').text
company = job.find('span', class_='company').text
location = job.find('span', class_='location').text
relative_link = job.find('a', class_='job-link')['href']
job_link = urljoin(base_url, relative_link)
print(f"Title: {title}, Company: {company}, Location: {location}, Link: {job_link}")
# Add delay to avoid being banned
time.sleep(2)
Sample Output:
Page 1
Title: Software Engineer, Company: TechCorp, Location: San Francisco, CA, Link: https://example.com/job/software-engineer
Title: Data Scientist, Company: DataCorp, Location: New York, NY, Link: https://example.com/job/data-scientist
Page 2
...
Tips for Scraping Job Listings
Inspect HTML Structure:
- Use browser DevTools to locate job attributes.
Handle Pagination:
- Automate navigation through
page
parameters or next-page links.
- Automate navigation through
Respect Rate Limits:
- Add delays (
time.sleep
) between requests.
- Add delays (
Save Data:
- Store extracted data in CSV or a database for analysis.
Saving Data to CSV:
import csv
# Save job data
with open('jobs.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(["Title", "Company", "Location", "Link"]) # Header
for job in job_cards:
writer.writerow([title, company, location, job_link])
print("Data saved to jobs.csv")
Data Cleaning and Storage
1. Cleaning Scraped Data with Python
Scraped data often contains extra whitespace, special characters, or missing values that need cleaning.
Code Example: Cleaning with pandas
import pandas as pd
# Example scraped data
data = {
"Title": [" Software Engineer ", "Data Scientist", None],
"Company": ["TechCorp", "DataCorp", "CodeLab"],
"Location": ["San Francisco, CA ", "New York, NY", None],
}
# Create a DataFrame
df = pd.DataFrame(data)
# Clean the data
df["Title"] = df["Title"].str.strip() # Remove leading/trailing spaces
df["Location"] = df["Location"].str.strip() # Clean Location column
df["Title"].fillna("Unknown Title", inplace=True) # Fill missing titles
df["Location"].fillna("Unknown Location", inplace=True) # Fill missing locations
print(df)
Output:
Title Company Location
0 Software Engineer TechCorp San Francisco, CA
1 Data Scientist DataCorp New York, NY
2 Unknown Title CodeLab Unknown Location
Other String Manipulations
Remove special characters:
df["Title"] = df["Title"].str.replace(r"[^a-zA-Z0-9 ]", "", regex=True)
Convert to lowercase:
df["Company"] = df["Company"].str.lower()
2. Storing Data in CSV, Excel, or JSON
Data can be exported for sharing or further analysis.
Save as CSV
df.to_csv("jobs.csv", index=False)
print("Data saved to jobs.csv")
Save as Excel
df.to_excel("jobs.xlsx", index=False)
print("Data saved to jobs.xlsx")
Save as JSON
df.to_json("jobs.json", orient="records", lines=True)
print("Data saved to jobs.json")
3. Storing Data in a Database (SQLite)
Using SQLite allows querying and storing large datasets efficiently.
Code Example: Storing in SQLite
import sqlite3
# Connect to SQLite database (or create one if it doesn't exist)
conn = sqlite3.connect("jobs.db")
# Store the DataFrame in a table
df.to_sql("job_listings", conn, if_exists="replace", index=False)
# Query the data back
query = "SELECT * FROM job_listings"
retrieved_data = pd.read_sql(query, conn)
print("Retrieved Data:")
print(retrieved_data)
conn.close()
Output (Sample Query Result):
Title Company Location
0 Software Engineer TechCorp San Francisco, CA
1 Data Scientist DataCorp New York, NY
2 Unknown Title CodeLab Unknown Location
4. Storing Data in PostgreSQL
PostgreSQL allows managing structured data with advanced querying capabilities.
Code Example: Storing in PostgreSQL
import psycopg2
from sqlalchemy import create_engine
# PostgreSQL connection settings
engine = create_engine("postgresql+psycopg2://username:password@localhost:5432/mydatabase")
# Store DataFrame in a PostgreSQL table
df.to_sql("job_listings", engine, if_exists="replace", index=False)
# Verify by querying
retrieved_data = pd.read_sql("SELECT * FROM job_listings", engine)
print("Retrieved Data:")
print(retrieved_data)
5. Exporting to Visualization Tools (Tableau, Power BI)
Save as CSV for Tableau/Power BI
Both Tableau and Power BI can ingest CSV files directly:
df.to_csv("jobs_for_visualization.csv", index=False)
print("Data saved to jobs_for_visualization.csv")
Direct Connection to Databases
Tableau: Connect directly to SQLite/PostgreSQL from Tableau using the database connector.
Power BI: Use Power BI’s built-in SQLite/PostgreSQL connectors to fetch data.
Basic Visualization Insights
Analyze job distributions by location.
Visualize top hiring companies by frequency.
6. Full Example: Scraping, Cleaning, and Storing
Code Example: End-to-End Job Scraper
import requests
from bs4 import BeautifulSoup
import pandas as pd
from urllib.parse import urljoin
import sqlite3
# Step 1: Scrape Data
base_url = "https://example.com/jobs"
headers = {'User-Agent': 'Mozilla/5.0'}
data = []
for page in range(1, 3): # Example: 2 pages
url = f"{base_url}?page={page}"
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
job_cards = soup.find_all('div', class_='job-card')
for job in job_cards:
title = job.find('h2', class_='job-title').get_text(strip=True)
company = job.find('span', class_='company').get_text(strip=True)
location = job.find('span', class_='location').get_text(strip=True)
relative_link = job.find('a', class_='job-link')['href']
link = urljoin(base_url, relative_link)
data.append({"Title": title, "Company": company, "Location": location, "Link": link})
# Step 2: Clean Data
df = pd.DataFrame(data)
df["Title"] = df["Title"].str.strip()
df["Location"] = df["Location"].str.strip()
df.fillna("Unknown", inplace=True)
# Step 3: Store Data in SQLite
conn = sqlite3.connect("jobs.db")
df.to_sql("job_listings", conn, if_exists="replace", index=False)
# Step 4: Save as CSV and Excel
df.to_csv("jobs.csv", index=False)
df.to_excel("jobs.xlsx", index=False)
print("Scraping, cleaning, and storage complete.")
Summary
Task | Tool/Method | Purpose |
Cleaning Data | pandas , string methods | Remove noise, handle missing data |
Save to CSV/Excel | df.to _csv , df.to _excel | Export for analysis or sharing |
Store in SQLite/PostgreSQL | sqlite3 , psycopg2 | Store data for querying and large datasets |
Export for Visualization | CSV, database connectors | Tableau/Power BI integration |
Handling Dynamic and JavaScript-Rendered Content
1. Introduction to Dynamic Content
Dynamic content is generated on the client-side using JavaScript, meaning the HTML you receive from a server might not contain the data you're looking for. Instead, the content is rendered dynamically in the browser.
How to Identify Dynamic Content
Use browser DevTools:
Inspect the element containing the data.
Check the "Network" tab to see if data is fetched via an API.
If the data doesn't appear in the HTML source but is visible in the browser, it's dynamically rendered.
Approaches for Scraping Dynamic Content
Use
selenium
for rendering JavaScript.Look for underlying APIs serving the data.
Handle CAPTCHA and bot detection mechanisms.
2. Using selenium
for Scraping JavaScript-Heavy Websites
Installation:
pip install selenium
Set Up WebDriver: Download the appropriate WebDriver (e.g., ChromeDriver) and add it to your system PATH.
Code Example: Using Selenium
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
# Initialize WebDriver
driver = webdriver.Chrome() # Or webdriver.Firefox()
# Navigate to a JavaScript-heavy page
driver.get("https://example.com/jobs")
# Wait for the page to load
time.sleep(5) # Use explicit waits in production
# Extract content
job_titles = driver.find_elements(By.CLASS_NAME, "job-title")
for job in job_titles:
print("Job Title:", job.text)
# Close the browser
driver.quit()
Sample Output:
Job Title: Software Engineer
Job Title: Data Scientist
Best Practices:
Use explicit waits instead of
time.sleep()
:from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.CLASS_NAME, "job-title")) )
Use headless mode to avoid opening a browser window:
options = webdriver.ChromeOptions() options.add_argument("--headless") driver = webdriver.Chrome(options=options)
3. Understanding APIs for Data Retrieval
Many websites use APIs to fetch dynamic data. Instead of scraping rendered HTML, you can directly query the API.
How to Identify APIs
Open the "Network" tab in browser DevTools.
Filter requests by
XHR
(XMLHttpRequest) to see API calls.Inspect the API response for the required data.
Code Example: API-Based Scraping
Suppose an API endpoint is https://example.com/api/jobs
.
import requests
# API endpoint
url = "https://example.com/api/jobs"
# Send GET request
response = requests.get(url)
if response.status_code == 200:
data = response.json()
for job in data["jobs"]:
print(f"Title: {job['title']}, Company: {job['company']}")
else:
print("Failed to fetch data. Status Code:", response.status_code)
Sample Output:
Title: Software Engineer, Company: TechCorp
Title: Data Scientist, Company: DataCorp
Advantages of Using APIs
Faster and more efficient than rendering HTML.
Reduces reliance on third-party tools like Selenium.
4. Handling CAPTCHA and Bot Protection
CAPTCHAs and bot detection mechanisms can block automated scraping attempts.
Types of Bot Protection
CAPTCHAs: Human-verification tests.
Headers/User-Agent Checking: Websites block non-browser user agents.
IP Rate Limits: Blocking requests from the same IP.
Strategies for Bypassing Protection
Use Proxies:
Rotate IPs using proxy services.
Example:
proxies = {"http": "http://your-proxy.com:8080"} response = requests.get("https://example.com", proxies=proxies)
Rotate User-Agent Strings:
Mimic a browser by sending appropriate headers.
Example:
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)"} response = requests.get("https://example.com", headers=headers)
Solve CAPTCHAs with External Services:
Use services like 2Captcha or Anti-Captcha.
Example:
import requests captcha_solution = requests.post( "https://2captcha.com/in.php", data={"key": "your-api-key", "method": "base64", "body": "captcha-image-base64"} )
Use Selenium with CAPTCHA Solvers:
- Selenium combined with automated CAPTCHA solving tools can bypass some challenges.
Identify CAPTCHA-Free APIs:
- Look for APIs not protected by CAPTCHAs to avoid solving them altogether.
End-to-End Example: Scraping JavaScript Content with CAPTCHA Handling
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
# Set up Selenium WebDriver
options = webdriver.ChromeOptions()
options.add_argument("--headless")
driver = webdriver.Chrome(options=options)
try:
# Navigate to the site
driver.get("https://example.com/jobs")
# Wait for job cards to load
WebDriverWait(driver, 10).until(
EC.presence_of_all_elements_located((By.CLASS_NAME, "job-card"))
)
# Extract job data
job_cards = driver.find_elements(By.CLASS_NAME, "job-card")
for card in job_cards:
title = card.find_element(By.CLASS_NAME, "job-title").text
company = card.find_element(By.CLASS_NAME, "company").text
print(f"Title: {title}, Company: {company}")
# Optional: Screenshot CAPTCHA for manual solving
# driver.save_screenshot("captcha.png")
finally:
# Close the driver
driver.quit()
Summary
Challenge | Solution |
Dynamic Content | Use selenium for JavaScript-rendered pages or inspect APIs for direct access. |
APIs for Data Retrieval | Use API endpoints directly if they are accessible (faster than scraping). |
CAPTCHA Challenges | Solve using external services, proxies, or identify CAPTCHA-free endpoints. |
Bot Detection | Rotate IPs, User-Agent strings, and avoid aggressive scraping patterns. |
Testing and Debugging Scrapers
1. Debugging Common Errors
Common HTTP Status Codes and Their Causes
404 Not Found: URL doesn’t exist or is incorrect.
403 Forbidden: Server blocks access, possibly due to bot detection.
500 Internal Server Error: Issue with the server; retry later or inspect the request payload.
Approaches to Debugging
404 Debugging:
Check if the URL is correct.
Inspect the webpage structure using browser DevTools to ensure the scraper targets the right elements.
403 Debugging:
Use appropriate headers (e.g., User-Agent).
Rotate proxies and IP addresses.
500 Debugging:
- Retry after some time using a delay or exponential backoff.
Code Example: Handling Errors Gracefully
import requests
url = "https://example.com/jobs"
try:
response = requests.get(url)
response.raise_for_status() # Raise HTTPError for bad responses (4xx and 5xx)
print("Page Content:", response.text[:100])
except requests.exceptions.HTTPError as e:
print(f"HTTP Error: {e}")
except requests.exceptions.RequestException as e:
print(f"Error: {e}")
Sample Output:
HTTP Error: 404 Client Error: Not Found for url: https://example.com/jobs
2. Logging for Scrapers
Logging provides a way to track the scraper’s behavior, debug issues, and ensure smooth operation.
Setting Up Logging
Code Example: Logging in a Scraper
import requests
import logging
# Configure logging
logging.basicConfig(
filename="scraper.log",
level=logging.INFO,
format="%(asctime)s - %(levelname)s - %(message)s",
)
def scrape_page(url):
try:
response = requests.get(url)
response.raise_for_status()
logging.info(f"Successfully fetched {url}")
return response.text
except requests.exceptions.HTTPError as e:
logging.error(f"HTTP Error for {url}: {e}")
except requests.exceptions.RequestException as e:
logging.error(f"Error for {url}: {e}")
# Example usage
scrape_page("https://example.com/jobs")
scrape_page("https://example.com/404")
Sample Log File (scraper.log
):
2024-11-16 12:00:00 - INFO - Successfully fetched https://example.com/jobs
2024-11-16 12:01:00 - ERROR - HTTP Error for https://example.com/404: 404 Client Error: Not Found for url
3. Testing Scrapers with Mock Data
Testing scrapers using live websites can be unreliable due to changes in page structure. Mock libraries like httpretty
or responses
allow testing against predefined data.
3.1 Using responses
for Mocking
Install responses
:
pip install responses
Code Example: Mocking Requests
import responses
import requests
@responses.activate
def test_scraper():
# Mock API response
url = "https://example.com/api/jobs"
responses.add(
responses.GET,
url,
json={"jobs": [{"title": "Software Engineer", "company": "TechCorp"}]},
status=200,
)
# Test scraper
response = requests.get(url)
data = response.json()
print("Job Title:", data["jobs"][0]["title"])
print("Company:", data["jobs"][0]["company"])
# Run test
test_scraper()
Output:
Job Title: Software Engineer
Company: TechCorp
3.2 Using httpretty
for Mocking
Install httpretty
:
pip install httpretty
Code Example: Mocking with httpretty
import httpretty
import requests
@httpretty.activate
def test_scraper():
# Mock HTTP response
url = "https://example.com/jobs"
httpretty.register_uri(
httpretty.GET,
url,
body="<html><body><h1>Software Engineer</h1></body></html>",
content_type="text/html",
)
# Test scraper
response = requests.get(url)
print("Response Content:", response.text)
# Run test
test_scraper()
Output:
Response Content: <html><body><h1>Software Engineer</h1></body></html>
Best Practices for Testing Scrapers
Mock External Dependencies:
- Use libraries like
responses
orhttpretty
to avoid hitting live servers.
- Use libraries like
Automate Tests:
- Integrate tests with CI/CD pipelines to detect issues early.
Test for Changes in Structure:
- Write unit tests to ensure scrapers adapt to changes in page structure.
Summary
Challenge | Solution |
HTTP Errors (404, 403, 500) | Gracefully handle errors with try-except and retry logic. |
Debugging Issues | Use logging to track scraper behavior and debug failures. |
Unstable Websites | Use mock libraries like httpretty or responses for reliable testing. |
Testing Data | Mock APIs or HTML responses to simulate real-world conditions. |
Automating Scraping for Job Websites
1. Building a Scraper for Indeed
Steps:
Inspect the structure of job listings using Chrome DevTools.
Identify the relevant tags for job titles, companies, locations, and URLs.
Handle pagination to scrape multiple pages.
Code Example: Scraping Indeed
import requests
from bs4 import BeautifulSoup
def scrape_indeed(base_url, num_pages=3):
jobs = []
for page in range(num_pages):
url = f"{base_url}&start={page * 10}" # Pagination for Indeed
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for card in soup.find_all('div', class_='job_seen_beacon'):
title = card.find('h2', class_='jobTitle').get_text(strip=True)
company = card.find('span', class_='companyName').get_text(strip=True)
location = card.find('div', class_='companyLocation').get_text(strip=True)
link = "https://indeed.com" + card.find('a')['href']
jobs.append({'title': title, 'company': company, 'location': location, 'link': link})
return jobs
# Example usage
base_url = "https://www.indeed.com/jobs?q=data+scientist"
jobs = scrape_indeed(base_url)
for job in jobs:
print(job)
Sample Output:
{'title': 'Data Scientist', 'company': 'TechCorp', 'location': 'San Francisco, CA', 'link': 'https://indeed.com/viewjob?jk=abc123'}
{'title': 'Machine Learning Engineer', 'company': 'DataCorp', 'location': 'New York, NY', 'link': 'https://indeed.com/viewjob?jk=def456'}
2. Building a Scraper for LinkedIn (With API if Available)
Option 1: Scraping LinkedIn (Requires Login)
LinkedIn's website uses dynamic content, requiring selenium
for scraping.
Code Example: Scraping LinkedIn
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
def scrape_linkedin(base_url):
driver = webdriver.Chrome()
driver.get(base_url)
time.sleep(5) # Allow page to load
jobs = []
job_cards = driver.find_elements(By.CLASS_NAME, 'base-card')
for card in job_cards:
title = card.find_element(By.CLASS_NAME, 'base-search-card__title').text
company = card.find_element(By.CLASS_NAME, 'base-search-card__subtitle').text
location = card.find_element(By.CLASS_NAME, 'job-search-card__location').text
link = card.find_element(By.TAG_NAME, 'a').get_attribute('href')
jobs.append({'title': title, 'company': company, 'location': location, 'link': link})
driver.quit()
return jobs
# Example usage
base_url = "https://www.linkedin.com/jobs/search?keywords=data+scientist"
jobs = scrape_linkedin(base_url)
for job in jobs:
print(job)
Option 2: Using LinkedIn APIs
If you have access to the LinkedIn API:
Follow the LinkedIn Developer API Docs.
Authenticate using OAuth2 and fetch job postings via
GET
requests.
Code Example (LinkedIn API):
import requests
url = "https://api.linkedin.com/v2/jobSearch"
headers = {
"Authorization": "Bearer your_access_token"
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(response.json())
else:
print("Error:", response.status_code)
3. Building a Scraper for AIJobs.net
Steps:
Analyze the HTML structure of job listings.
Extract job titles, companies, and links.
Code Example: Scraping AIJobs.net
import requests
from bs4 import BeautifulSoup
from urllib.parse import urljoin
def scrape_aijobs(base_url):
response = requests.get(base_url)
soup = BeautifulSoup(response.text, 'html.parser')
jobs = []
for card in soup.find_all('div', class_='job-card'):
title = card.find('h2', class_='job-title').text.strip()
company = card.find('span', class_='company-name').text.strip()
relative_link = card.find('a', href=True)['href']
link = urljoin(base_url, relative_link)
jobs.append({'title': title, 'company': company, 'link': link})
return jobs
# Example usage
base_url = "https://aijobs.net/"
jobs = scrape_aijobs(base_url)
for job in jobs:
print(job)
Sample Output:
{'title': 'AI Engineer', 'company': 'OpenAI', 'link': 'https://aijobs.net/jobs/ai-engineer'}
{'title': 'Data Scientist', 'company': 'DeepMind', 'link': 'https://aijobs.net/jobs/data-scientist'}
4. Scheduling Scrapers with cron
or APScheduler
Option 1: Scheduling with cron
(Linux/Unix)
Set Up Cron Job:
Save the scraper script as
scraper.py
.Open the crontab editor:
crontab -e
Add a job to run the script every day at midnight:
0 0 * * * /path/to/python /path/to/scraper.py
Example Script (scraper.py
):
import pandas as pd
# Call your scraper functions here
jobs = scrape_indeed("https://www.indeed.com/jobs?q=data+scientist")
df = pd.DataFrame(jobs)
df.to_csv("jobs.csv", index=False)
print("Scraper ran successfully!")
Option 2: Scheduling with APScheduler
(Python)
Install APScheduler:
pip install apscheduler
Code Example: Using APScheduler
from apscheduler.schedulers.blocking import BlockingScheduler
import pandas as pd
def scheduled_scraper():
jobs = scrape_indeed("https://www.indeed.com/jobs?q=data+scientist")
df = pd.DataFrame(jobs)
df.to_csv("jobs.csv", index=False)
print("Scraper ran successfully!")
# Schedule the scraper
scheduler = BlockingScheduler()
scheduler.add_job(scheduled_scraper, 'interval', hours=24) # Run every 24 hours
scheduler.start()
Best Practices for Automated Job Scraping
Respect Website Terms:
- Check
robots.txt
to ensure compliance.
- Check
Add Delays and Rotate Proxies:
- Avoid overwhelming servers or being detected as a bot.
Store Data Efficiently:
- Save scraped data to a database (e.g., SQLite or PostgreSQL) for querying.
Error Handling and Logging:
- Log scraper errors to debug issues.
Monitor Scheduler Jobs:
- Use tools like Airflow or Cron Monitoring services.
Deploying Scrapers
1. Running Scrapers on Cloud Platforms
1.1 Deploying on AWS
Step 1: Set up an EC2 instance.
Launch an EC2 instance and SSH into it.
Install Python and required libraries:
sudo apt update sudo apt install python3-pip pip3 install requests beautifulsoup4
Step 2: Upload the scraper.
Use
scp
to transfer your scraper:scp scraper.py ec2-user@<your-instance-ip>:/home/ec2-user/
Step 3: Schedule with
cron
or run manually:python3 scraper.py
1.2 Deploying on Google Cloud Platform (GCP)
Step 1: Create a Compute Engine instance.
Enable Compute Engine in your GCP account and set up a VM.
SSH into the instance.
Step 2: Install Python and libraries.
sudo apt update sudo apt install python3-pip pip3 install requests beautifulsoup4
Step 3: Upload the script and run it:
gcloud compute scp scraper.py instance-name:/home/ python3 scraper.py
1.3 Deploying on Heroku
Step 1: Create a Heroku app.
heroku create your-scraper-app
Step 2: Create a
Procfile
to specify the scraper:worker: python scraper.py
Step 3: Deploy the scraper:
git init git add . git commit -m "Deploy scraper" heroku git:remote -a your-scraper-app git push heroku master
Step 4: Run the worker process:
heroku ps:scale worker=1
2. Using Docker for Containerized Scraping
Docker allows you to package your scraper with all dependencies, ensuring consistent performance across environments.
2.1 Writing a Dockerfile
# Use a base Python image
FROM python:3.9-slim
# Set the working directory
WORKDIR /app
# Copy the scraper files
COPY . /app
# Install dependencies
RUN pip install -r requirements.txt
# Command to run the scraper
CMD ["python", "scraper.py"]
2.2 Building and Running the Docker Image
# Build the Docker image
docker build -t my-scraper .
# Run the Docker container
docker run -it --rm my-scraper
2.3 Deploying the Dockerized Scraper
Push the Docker image to a container registry (e.g., Docker Hub, AWS ECR).
Use cloud services like AWS ECS or Kubernetes to run the container.
3. Automating Workflows with CI/CD
3.1 Setting Up GitHub Actions
GitHub Actions can automate deploying your scraper to a cloud platform or running it periodically.
Example Workflow (.github/workflows/scraper.yml
):
name: Run Scraper
on:
schedule:
- cron: "0 0 * * *" # Run daily at midnight
push:
branches:
- main
jobs:
scraper:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: 3.9
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run scraper
run: python scraper.py
3.2 Setting Up CI/CD on Jenkins
Install Jenkins on a server.
Create a Jenkins pipeline that:
Pulls your scraper from a Git repository.
Builds a Docker image.
Deploys it to your target environment.
Example Jenkins Pipeline Script:
pipeline {
agent any
stages {
stage('Checkout') {
steps {
git 'https://github.com/your-repo/scraper.git'
}
}
stage('Build Docker Image') {
steps {
sh 'docker build -t scraper-image .'
}
}
stage('Run Scraper') {
steps {
sh 'docker run --rm scraper-image'
}
}
}
}
4. Best Practices for Deployment
Scaling Scrapers
Use cloud platforms like AWS ECS or GCP Kubernetes to manage and scale containers.
Automate retries and error handling for failed scrapers.
Security Considerations
Use secrets management tools for storing API keys and sensitive data (e.g., AWS Secrets Manager, GCP Secret Manager).
Regularly update dependencies to patch security vulnerabilities.
Monitoring and Alerts
Integrate monitoring tools like Prometheus or CloudWatch to track scraper performance.
Set up alerts for failures or unusual activity.
Summary
Task | Tools/Platforms | Key Steps |
Cloud Deployment | AWS EC2, GCP Compute Engine, Heroku | Set up VMs or use serverless platforms to run the scraper. |
Containerization | Docker | Package scraper and dependencies into a Docker container. |
Workflow Automation | GitHub Actions, Jenkins | Automate deployment and periodic scraping tasks. |
Monitoring | Prometheus, CloudWatch | Monitor and set up alerts for scraper performance. |
Ethical and Scalable Scraping
1. Respecting Website Terms of Service
Ethical web scraping involves respecting a website's rules and guidelines. Ignoring these can lead to legal issues or IP bans.
Best Practices:
Check
robots.txt
:- Websites often define scraping rules in a
robots.txt
file. While not legally binding, it’s good to respect these guidelines.
- Websites often define scraping rules in a
User-agent: *
Disallow: /api/
Code Example: Checking robots.txt
import requests
def check_robots(url):
response = requests.get(url + "/robots.txt")
if response.status_code == 200:
print(response.text)
else:
print("robots.txt not found")
check_robots("https://example.com")
Rate-Limit Requests:
Add delays between requests to avoid overwhelming the server.
Use libraries like
time.sleep
or rate-limiting tools.
Provide Identification:
- Include a clear
User-Agent
string to identify your scraper.
- Include a clear
headers = {"User-Agent": "MyScraperBot/1.0 (+http://example.com/bot)"}
requests.get("https://example.com", headers=headers)
Avoid Sensitive Data:
- Do not scrape personal or sensitive information unless explicitly allowed.
2. Using APIs Instead of Scraping (Where Applicable)
Many websites provide APIs for structured and efficient data access. APIs are often more reliable and faster than scraping.
Benefits of APIs:
Faster and easier to parse JSON/XML responses.
More robust to changes in the website layout.
Avoids legal and ethical issues.
Example: Using a Job API
import requests
url = "https://api.example.com/jobs"
headers = {"Authorization": "Bearer your_api_key"}
response = requests.get(url, headers=headers)
if response.status_code == 200:
print(response.json()) # Parsed JSON data
else:
print("Error:", response.status_code)
Find APIs:
Official APIs: Check the website's developer documentation (e.g., LinkedIn, Twitter).
Third-Party APIs: Services like RapidAPI offer APIs for many use cases.
3. Planning Scalable Scraping Solutions
Scalability ensures your scraper can handle large volumes of data without hitting performance bottlenecks.
Key Considerations:
Distribute Requests:
Use proxy pools or load balancers to distribute requests across multiple IPs.
Example with
rotating proxies
:proxies = [ "http://proxy1:8080", "http://proxy2:8080", "http://proxy3:8080" ] for i, url in enumerate(urls): proxy = proxies[i % len(proxies)] response = requests.get(url, proxies={"http": proxy, "https": proxy}) print(response.status_code)
Asynchronous Scraping:
Use
asyncio
andaiohttp
to send requests concurrently.import aiohttp import asyncio async def fetch(url, session): async with session.get(url) as response: return await response.text() async def scrape_all(urls): async with aiohttp.ClientSession() as session: tasks = [fetch(url, session) for url in urls] return await asyncio.gather(*tasks) urls = ["https://example.com/page1", "https://example.com/page2"] results = asyncio.run(scrape_all(urls)) print(results)
Store Data Efficiently:
Use databases like PostgreSQL or MongoDB for scalable storage.
Save in batches to reduce disk I/O overhead.
Monitor and Log:
Use tools like Prometheus and Grafana for performance monitoring.
Log scraper performance (e.g., request success rates, errors).
4. Future Trends in Web Scraping
4.1 Increased Use of AI for Scraping
Intelligent Parsers:
- Tools like OpenAI’s GPT models can extract structured data from unstructured HTML.
Example:
from transformers import pipeline html_content = "<html><body><h1>Title</h1><p>Description here</p></body></html>" summarizer = pipeline("summarization") summary = summarizer(html_content) print(summary)
4.2 APIs Over Scraping
- APIs are becoming the preferred method for accessing data, reducing the need for scraping.
4.3 Anti-Scraping Measures
Websites are adopting advanced anti-bot technologies, including:
CAPTCHA challenges.
Behavioral analysis (e.g., detecting mouse movements).
JavaScript obfuscation.
4.4 Ethical and Legal Frameworks
- Governments and organizations are introducing stricter regulations on web scraping.
4.5 Distributed Scraping:
- Scraping frameworks like Scrapy will incorporate distributed task management systems like Apache Kafka or RabbitMQ.
Summary
Aspect | Key Practices |
Ethical Scraping | Respect robots.txt , use headers, avoid sensitive data. |
API Usage | Use APIs for structured, reliable data access where available. |
Scalability | Implement proxies, asynchronous requests, and efficient storage. |
Future Trends | AI-based scraping, anti-scraping advancements, distributed solutions. |
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
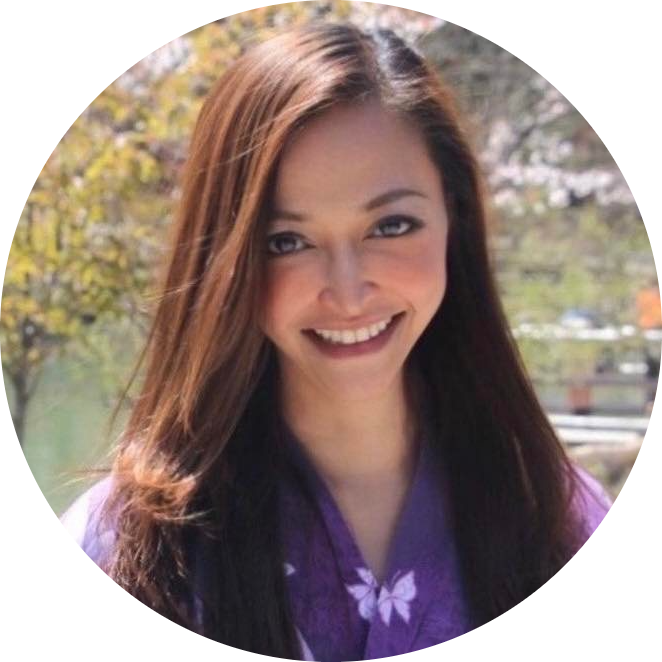