A Comprehensive Guide to Conditional Logic with `switch-case`

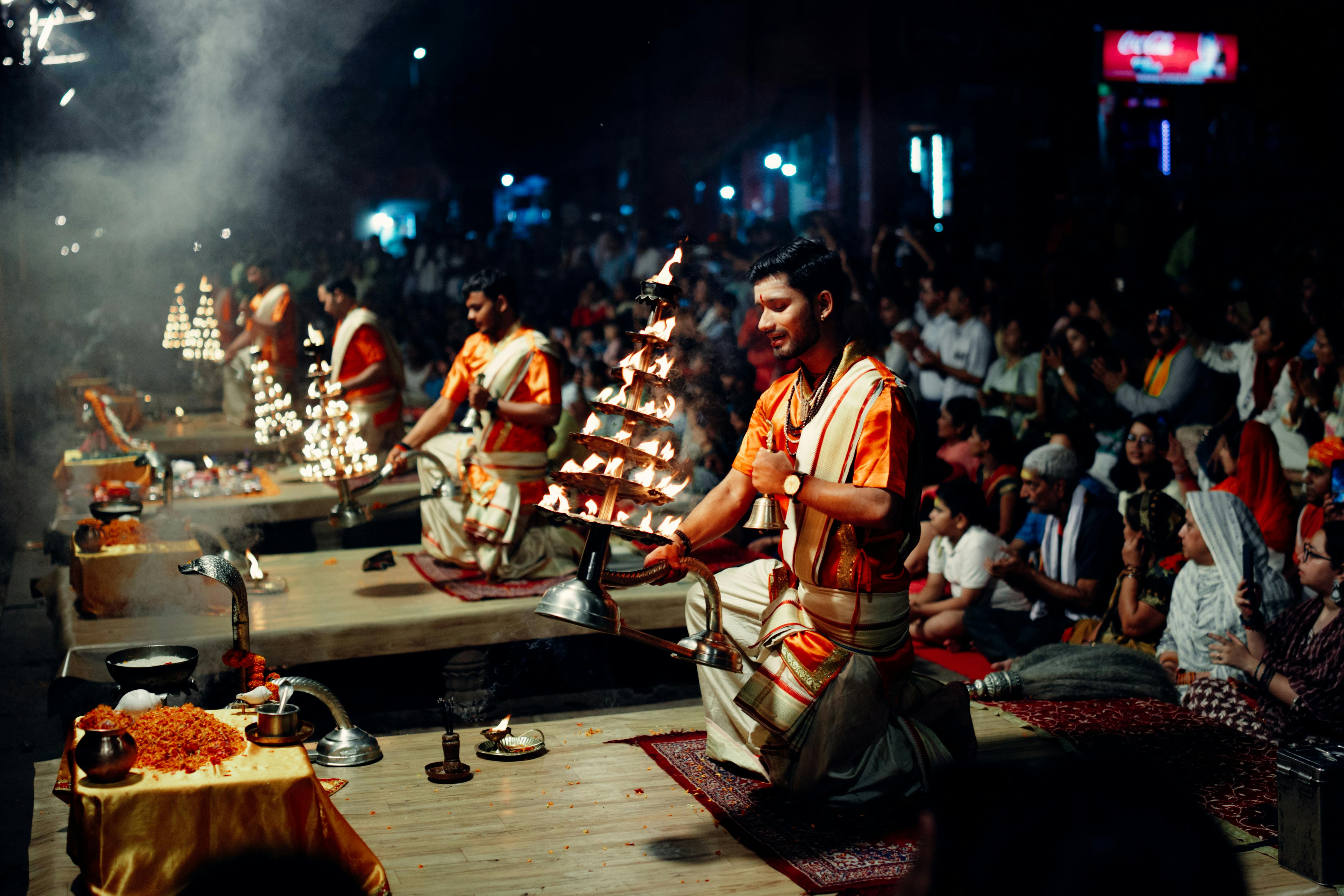
The switch
statement in Go is a powerful control structure that allows you to execute one block of code among many based on the value of a variable or expression. It's an alternative to using multiple if-else
statements, making your code more readable and easier to maintain.
In this article, we will cover:
Basic
switch
statementUsing multiple cases
Default case
Switch with expressions
Fallthrough in
switch
Type switch
By the end of this article, you’ll be able to use the switch
statement effectively in your Go programs.
1. Basic switch
Statement
The basic switch
statement checks the value of an expression against several cases and executes the block of code that matches the case.
Syntax
switch expression {
case value1:
// Code to execute if expression == value1
case value2:
// Code to execute if expression == value2
default:
// Code to execute if no case matches
}
Example 1: Basic switch
Statement
package main
import "fmt"
func main() {
day := "Monday"
switch day {
case "Monday":
fmt.Println("Start of the work week.")
case "Friday":
fmt.Println("End of the work week.")
default:
fmt.Println("It's a regular day.")
}
}
Code Explanation
Line 5: We declare the variable
day
with a value of"Monday"
.Line 7: The
switch
statement checks the value ofday
.Line 8: The
case "Monday"
matches the value ofday
, so this block is executed, printing"Start of the work week."
.Line 12: The
default
block would be executed if no cases matched.
Output
Start of the work week.
Key Points:
The
switch
statement checks each case from top to bottom.The first matching case is executed, and then the
switch
statement exits.The
default
case is optional but is executed if no other case matches.
2. Using Multiple Cases
You can list multiple values for a single case by separating them with commas.
Example 2: Using Multiple Cases
package main
import "fmt"
func main() {
day := "Saturday"
switch day {
case "Saturday", "Sunday":
fmt.Println("It's the weekend!")
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday":
fmt.Println("It's a weekday.")
default:
fmt.Println("Unknown day.")
}
}
Code Explanation
Line 5: We declare the variable
day
with a value of"Saturday"
.Line 7: The
switch
statement checks the value ofday
.Line 8: The
case "Saturday", "Sunday"
matches becauseday
is"Saturday"
, so the block prints"It's the weekend!"
.
Output
It's the weekend!
Key Points:
You can list multiple values for a single case, making the code concise.
The case block is executed if any of the listed values match.
3. Default Case
The default
case is used when none of the specified cases match. It acts as a fallback.
Example 3: Using Default Case
package main
import "fmt"
func main() {
color := "purple"
switch color {
case "red":
fmt.Println("The color is red.")
case "blue":
fmt.Println("The color is blue.")
default:
fmt.Println("Color not recognized.")
}
}
Code Explanation
Line 5: We declare the variable
color
with a value of"purple"
.Line 7: The
switch
statement checks the value ofcolor
.Line 12: Since
"purple"
does not match any case, thedefault
block is executed.
Output
Color not recognized.
Key Points:
The
default
block runs if no cases match.It’s a good practice to include a
default
case for unexpected values.
4. Switch with Expressions
In Go, you can use an expression in the switch
statement instead of a variable.
Example 4: Switch with Expressions
package main
import "fmt"
func main() {
number := 15
switch {
case number%2 == 0:
fmt.Println("The number is even.")
case number%2 != 0:
fmt.Println("The number is odd.")
}
}
Code Explanation
Line 5: We declare the variable
number
with a value of15
.Line 7: The
switch
statement does not use a variable but checks conditions directly.Line 8: The
case
checks ifnumber
is even. Since15
is odd, this block is skipped.Line 10: The next
case
checks ifnumber
is odd. This condition istrue
, so the block prints"The number is odd."
.
Output
The number is odd.
Key Points:
Using expressions in
switch
makes it flexible for condition-based checks.The
switch
statement exits after the first matching case.
5. Fallthrough in switch
By default, Go’s switch
does not automatically fall through to the next case like in some other languages. However, you can explicitly specify fallthrough
if needed.
Example 5: Using Fallthrough
package main
import "fmt"
func main() {
grade := "B"
switch grade {
case "A":
fmt.Println("Excellent!")
fallthrough
case "B":
fmt.Println("Good job!")
fallthrough
case "C":
fmt.Println("You passed.")
default:
fmt.Println("Better luck next time.")
}
}
Code Explanation
Line 5: We declare the variable
grade
with a value of"B"
.Line 7: The
switch
statement checks the value ofgrade
.Line 11: The
fallthrough
keyword makes the execution continue to the next case, even if it doesn’t match.Line 13: The
fallthrough
after"B"
makes it execute the"C"
case as well.
Output
Good job!
You passed.
Key Points:
By default, Go does not fall through to the next case.
Using
fallthrough
can make your code less clear, so use it carefully.
6. Type Switch
A type switch is used to compare the type of a variable rather than its value.
Example 6: Using Type Switch
package main
import "fmt"
func main() {
var value interface{} = "Hello, Go!"
switch v := value.(type) {
case string:
fmt.Println("The value is a string:", v)
case int:
fmt.Println("The value is an integer:", v)
default:
fmt.Println("Unknown type.")
}
}
Code Explanation
Line 5: We declare
value
as an empty interface, which can hold any type.Line 7: The type switch checks the type of
value
.Line 8: If
value
is astring
, it prints the string value.Line 10: If
value
is anint
, it prints the integer value.Line 12: If the type does not match any case, the
default
block is executed.
Output
The value is a string: Hello, Go!
Key Points:
Type switches are useful when dealing with variables of unknown types (e.g., interfaces).
They help in handling different types safely.
Conclusion
In this article, we covered:
The basic
switch
statement for value-based checks.Using multiple cases and the
default
case.Advanced usage with expressions and type switches.
The
fallthrough
keyword for manual case continuation.
Understanding the switch
statement is essential for writing clear and concise Go programs. In upcoming articles, we’ll dive into loops, functions, and more advanced topics in Go programming.
Happy coding with Go, and stay tuned for more tutorials!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
