Mastering the for Loop in Go: The Only Loop You Need

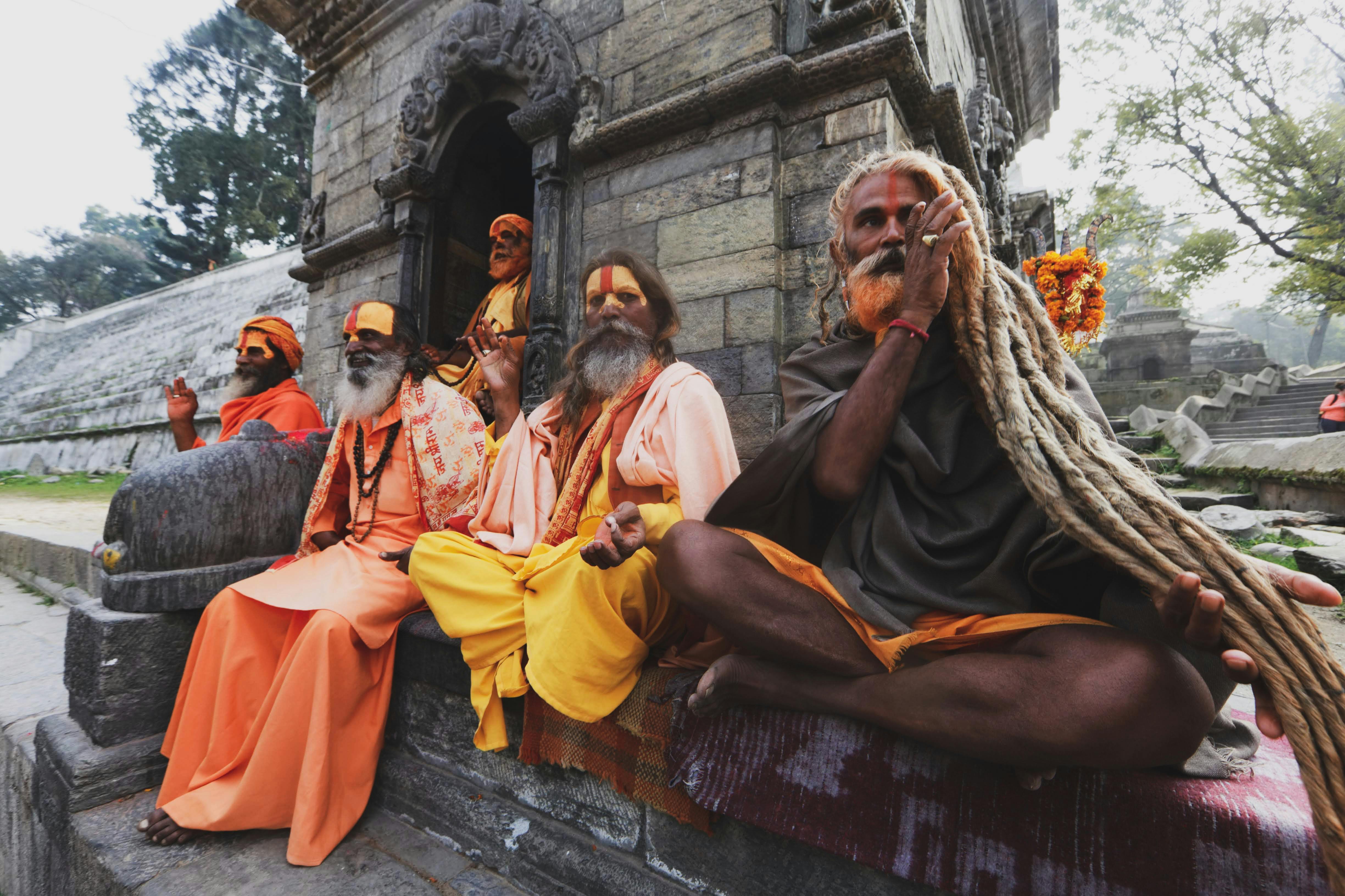
In Go, the for
loop is the only looping construct you need. Unlike many other programming languages that have multiple types of loops (such as while
and do-while
), Go simplifies things by using the versatile for
loop to handle all iterative tasks. By understanding the different ways to use for
loops, you can solve a wide range of programming problems efficiently.
In this article, we will cover:
Basic
for
loopCondition-based
for
loop (similar towhile
loop)Infinite
for
loopUsing
for
loop withrange
Nested
for
loopsBreaking out of a loop and using
continue
Let’s explore each concept with examples and detailed explanations.
1. Basic for
Loop
The most common form of the for
loop includes an initialization, a condition, and a post-statement.
Syntax
for initialization; condition; post {
// Code to execute in each iteration
}
Example 1: Basic for
Loop
package main
import "fmt"
func main() {
for i := 0; i < 5; i++ {
fmt.Println("Iteration:", i)
}
}
Code Explanation
Line 5: The
for
loop starts with the initializationi := 0
, sets the conditioni < 5
, and incrementsi
withi++
in each iteration.Line 6: Inside the loop, we print the value of
i
for each iteration.Line 7: The loop ends when
i
is no longer less than5
.
Output
Iteration: 0
Iteration: 1
Iteration: 2
Iteration: 3
Iteration: 4
Key Points:
The loop starts with
i = 0
and continues untili
reaches5
.The value of
i
is incremented by1
after each iteration (i++
is shorthand fori = i + 1
).
2. Condition-based for
Loop (Similar to while
Loop)
You can use the for
loop in Go as a while
loop by omitting the initialization and post-statement.
Example 2: Condition-based for
Loop
package main
import "fmt"
func main() {
count := 1
for count <= 5 {
fmt.Println("Count:", count)
count++
}
}
Code Explanation
Line 5: We initialize the variable
count
to1
.Line 7: The
for
loop checks the conditioncount <= 5
before each iteration.Line 8: Inside the loop, we print the value of
count
.Line 9: We increment
count
by1
in each iteration.Line 10: The loop ends when
count
becomes greater than5
.
Output
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
Key Points:
This form of the
for
loop behaves like awhile
loop in other languages.It keeps iterating as long as the condition is
true
.
3. Infinite for
Loop
An infinite loop is a loop that runs forever until it is explicitly stopped using a break
statement.
Example 3: Infinite for
Loop
package main
import "fmt"
func main() {
i := 0
for {
fmt.Println("Infinite loop iteration:", i)
i++
if i >= 3 {
break
}
}
}
Code Explanation
Line 5: We initialize the variable
i
to0
.Line 7: The
for
loop has no condition, making it an infinite loop.Line 9: We increment
i
and check if it is greater than or equal to3
.Line 10: The
break
statement exits the loop wheni
is3
.
Output
Infinite loop iteration: 0
Infinite loop iteration: 1
Infinite loop iteration: 2
Key Points:
Infinite loops can be useful in certain situations, such as server applications that need to run continuously.
Always include a
break
statement or a condition to exit the loop to prevent it from running indefinitely.
4. Using for
Loop with range
The range
keyword is used to iterate over elements of arrays, slices, maps, or strings.
Example 4: Iterating Over an Array with range
package main
import "fmt"
func main() {
numbers := []int{10, 20, 30, 40, 50}
for index, value := range numbers {
fmt.Println("Index:", index, "Value:", value)
}
}
Code Explanation
Line 5: We define an array
numbers
with 5 integers.Line 7: The
for
loop usesrange
to iterate overnumbers
.Line 8:
index
is the index of the current element, andvalue
is its value.Line 9: We print both the index and value in each iteration.
Output
Index: 0 Value: 10
Index: 1 Value: 20
Index: 2 Value: 30
Index: 3 Value: 40
Index: 4 Value: 50
Key Points:
The
range
keyword simplifies looping through collections like arrays and slices.You can use
_
as a placeholder if you don’t need the index or value.
5. Nested for
Loops
You can use a for
loop inside another for
loop to iterate through multidimensional data structures.
Example 5: Nested for
Loop
package main
import "fmt"
func main() {
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
fmt.Println("i:", i, "j:", j)
}
}
}
Code Explanation
Line 5: The outer loop iterates with
i
from1
to3
.Line 6: The inner loop iterates with
j
from1
to3
for each value ofi
.Line 7: We print the values of
i
andj
in each iteration.
Output
i: 1 j: 1
i: 1 j: 2
i: 1 j: 3
i: 2 j: 1
i: 2 j: 2
i: 2 j: 3
i: 3 j: 1
i: 3 j: 2
i: 3 j: 3
Key Points:
Nested loops are useful for working with multidimensional data structures like matrices.
Be careful with nested loops as they can become computationally expensive.
6. Breaking Out of a Loop and Using continue
You can use the break
statement to exit a loop early and the continue
statement to skip to the next iteration.
Example 6: Using break
and continue
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i%2 == 0 {
continue
}
if i > 7 {
break
}
fmt.Println("Odd number:", i)
}
}
Code Explanation
Line 5: The loop iterates from
1
to10
.Line 6: If
i
is even (i%2 == 0
), thecontinue
statement skips the rest of the loop body.Line 8: If
i
is greater than7
, thebreak
statement exits the loop.Line 10: We print the value of
i
if it is odd and less than or equal to7
.
Output
Odd number: 1
Odd number: 3
Odd number: 5
Odd number: 7
Conclusion
In this article, we covered:
The basic
for
loop and its components.Using the
for
loop as a condition-based loop (like awhile
loop).Infinite loops and how to break out of them.
Iterating through collections using
range
.Nested loops for handling complex data structures.
Using
break
andcontinue
for controlling loop flow.
The for
loop in Go is versatile and powerful, making it the only loop you need to master. In upcoming articles, we’ll dive deeper into functions, error handling, and more advanced Go programming concepts.
Happy coding with Go, and stay tuned for more tutorials!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
