Mastering Flow Control in Go: Break, Continue, and Goto Explained

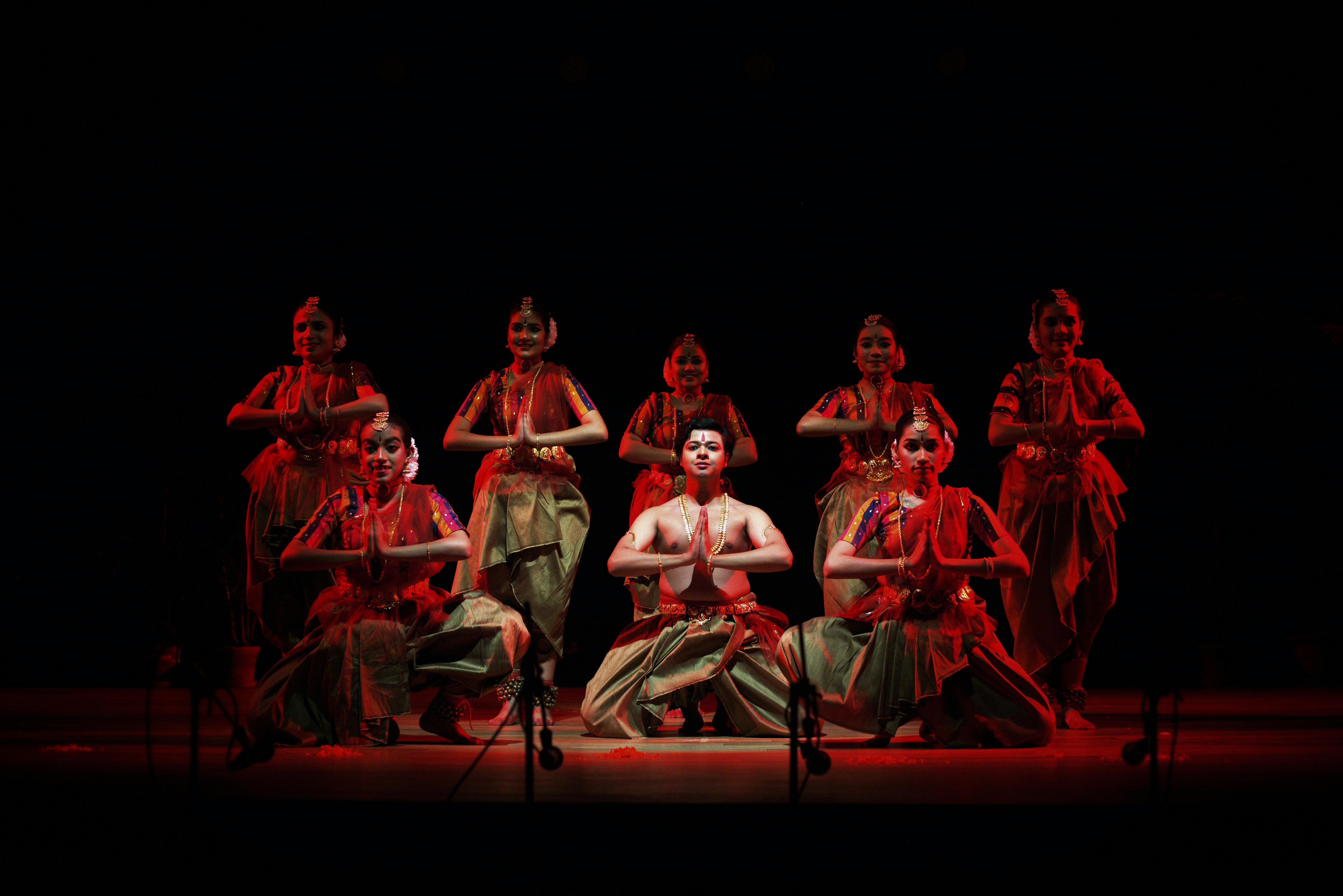
In Go, controlling the flow of loops and blocks of code is essential for writing efficient programs. Go provides three key keywords for this purpose:
break
: Exits a loop or switch statement prematurely.continue
: Skips the current iteration of a loop and moves to the next iteration.goto
: Jumps to a labeled statement in the code.
Understanding how to use these keywords can help you write more flexible and efficient code. In this article, we’ll explore these concepts with examples and detailed explanations.
1. Using break
in Go
The break
keyword is used to exit a loop or a switch statement immediately. It’s useful when you need to stop the loop based on a certain condition.
Example 1: Using break
in a for
Loop
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i == 5 {
fmt.Println("Breaking the loop at i =", i)
break
}
fmt.Println("i =", i)
}
}
Code Explanation
Line 5: The loop iterates from
1
to10
.Line 6: We check if
i
equals5
.Line 7: If
i
is5
, thebreak
statement is executed, exiting the loop.Line 9: We print the value of
i
for each iteration until the loop breaks.
Output
i = 1
i = 2
i = 3
i = 4
Breaking the loop at i = 5
Key Points:
The
break
statement immediately exits the loop when the condition is met.No further iterations occur once the loop is broken.
2. Using continue
in Go
The continue
keyword is used to skip the current iteration of a loop and move on to the next iteration. It’s useful when you want to ignore certain cases without exiting the entire loop.
Example 2: Using continue
in a for
Loop
package main
import "fmt"
func main() {
for i := 1; i <= 5; i++ {
if i%2 == 0 {
fmt.Println("Skipping even number:", i)
continue
}
fmt.Println("Odd number:", i)
}
}
Code Explanation
Line 5: The loop iterates from
1
to5
.Line 6: We check if
i
is even using the conditioni%2 == 0
.Line 7: If
i
is even, thecontinue
statement is executed, skipping the rest of the loop body.Line 10: We print the value of
i
only if it is odd.
Output
Odd number: 1
Skipping even number: 2
Odd number: 3
Skipping even number: 4
Odd number: 5
Key Points:
The
continue
statement skips the current iteration and moves to the next one.It’s useful for skipping unwanted values or cases in a loop.
3. Using goto
in Go
The goto
keyword allows you to jump to a labeled statement in your code. While it can be useful in certain scenarios, it should be used sparingly because it can make the code harder to read and maintain.
Syntax
goto labelName
labelName
is a user-defined label that marks a position in the code.
Example 3: Using goto
to Jump to a Label
package main
import "fmt"
func main() {
i := 0
start:
if i >= 5 {
fmt.Println("End of loop with goto")
return
}
fmt.Println("i =", i)
i++
goto start
}
Code Explanation
Line 5: We initialize
i
to0
.Line 7: The
start
label marks the beginning of the loop.Line 8: We check if
i
is greater than or equal to5
. If true, the program prints a message and exits usingreturn
.Line 10: The value of
i
is printed.Line 11: We increment
i
by1
.Line 12: The
goto start
statement jumps back to thestart
label, creating a loop.
Output
i = 0
i = 1
i = 2
i = 3
i = 4
End of loop with goto
Key Points:
The
goto
statement allows you to jump to a labeled part of the code.Overusing
goto
can make your code complex and hard to follow. It’s generally best to use loops and functions for control flow.
4. Combining break
, continue
, and goto
Let’s see an example that uses all three keywords together.
Example 4: Using break
, continue
, and goto
package main
import "fmt"
func main() {
i := 0
loop:
for {
if i == 10 {
fmt.Println("Breaking the loop at i =", i)
break
}
if i%2 == 0 {
fmt.Println("Even number, skipping:", i)
i++
continue
}
if i == 7 {
fmt.Println("Jumping to the loop label at i =", i)
i++
goto loop
}
fmt.Println("Odd number:", i)
i++
}
}
Code Explanation
Line 5: We initialize
i
to0
.Line 7: The
loop
label is defined, marking the start of the main loop.Line 8: An infinite
for
loop is started.Line 9: If
i
is10
, thebreak
statement exits the loop.Line 12: If
i
is even, thecontinue
statement skips to the next iteration after printing a message.Line 16: If
i
is7
, thegoto
statement jumps back to theloop
label.Line 20: If
i
is odd and not equal to7
, the value ofi
is printed.
Output
Even number, skipping: 0
Odd number: 1
Even number, skipping: 2
Odd number: 3
Even number, skipping: 4
Odd number: 5
Even number, skipping: 6
Jumping to the loop label at i = 7
Odd number: 7
Even number, skipping: 8
Odd number: 9
Breaking the loop at i = 10
Key Points:
The
break
statement stops the loop wheni
is10
.The
continue
statement skips the current iteration wheni
is even.The
goto
statement jumps back to the labeled loop wheni
is7
.
Conclusion
In this article, we explored:
break
: Used to exit a loop or switch statement.continue
: Skips the current iteration and moves to the next one.goto
: Jumps to a labeled statement in the code.
These keywords provide powerful control over the flow of your programs. However, use them wisely, especially goto
, as improper usage can make your code difficult to read and maintain.
Stay tuned for upcoming tutorials, where we’ll dive into more advanced topics like functions, error handling, and concurrency in Go.
Happy coding with Go, and see you in the next article!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
