Unlocking the Power of Functions in Go: A Beginner's Journey to Mastery

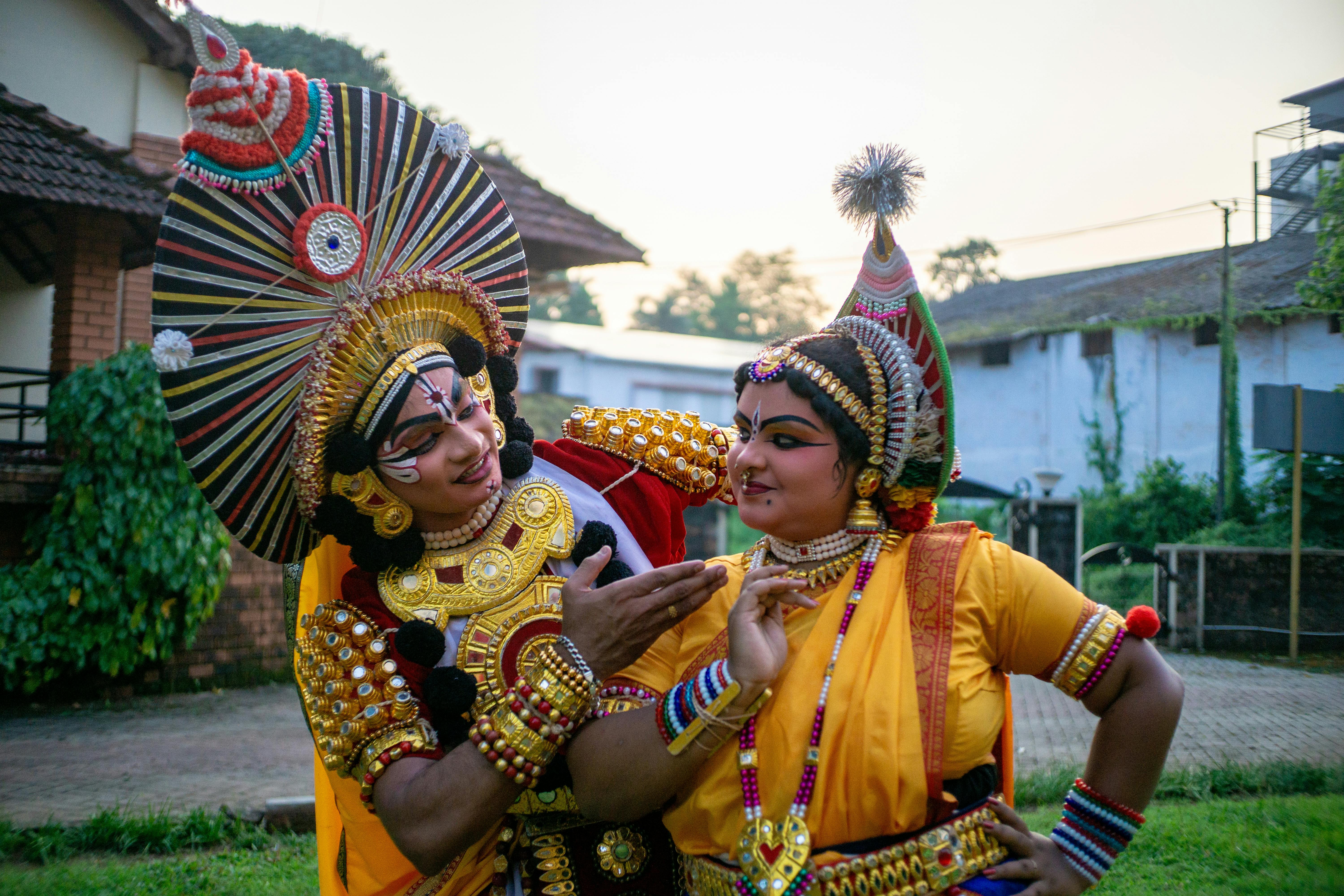
Functions are the building blocks of any programming language, and Go is no exception. Functions allow us to organize code, reduce redundancy, and make our programs easier to read and maintain. In this article, we will explore:
Defining and calling functions
Function parameters and return values
Named return values
Variadic functions
Anonymous functions and closures
We will dive into each concept with detailed explanations and examples, perfect for beginners.
1. Defining and Calling Functions
A function is a reusable block of code that performs a specific task. In Go, you define a function using the func
keyword, followed by the function name, parameters (if any), and the function body.
Example 1: Defining and Calling a Function
package main
import "fmt"
// Defining a function named greet
func greet() {
fmt.Println("Hello, Go!")
}
func main() {
// Calling the greet function
greet()
}
Code Explanation
Line 5: We define a function named
greet
using thefunc
keyword. It does not take any parameters and does not return a value.Line 6: Inside the function, we use
fmt.Println
to print a message.Line 10: We call the
greet
function inside themain
function to execute its code.
Output
Hello, Go!
Key Points:
The
greet
function is defined once but can be called multiple times.Every Go program must have a
main
function, which is the entry point of the program.
2. Function Parameters and Return Values
Functions in Go can take parameters (inputs) and return values (outputs). Parameters are specified inside the parentheses after the function name.
Example 2: Function with Parameters and Return Value
package main
import "fmt"
// Defining a function that takes two integers and returns their sum
func add(a int, b int) int {
sum := a + b
return sum
}
func main() {
result := add(5, 10)
fmt.Println("Sum:", result)
}
Code Explanation
Line 5: The
add
function takes two parameters,a
andb
, both of typeint
, and returns an integer.Line 6: We calculate the sum of
a
andb
and store it in thesum
variable.Line 7: The
return
statement sends the value ofsum
back to the caller.Line 11: We call the
add
function with arguments5
and10
and store the result inresult
.
Output
Sum: 15
Key Points:
Function parameters allow us to pass values to functions.
The
return
statement is used to send a value back to the caller.
3. Named Return Values
In Go, you can assign names to return values, making your functions more readable and allowing you to omit explicit return values in some cases.
Example 3: Named Return Values
package main
import "fmt"
// Defining a function with named return value
func multiply(a int, b int) (result int) {
result = a * b
return
}
func main() {
product := multiply(3, 4)
fmt.Println("Product:", product)
}
Code Explanation
Line 5: The
multiply
function has a named return value,result
, of typeint
.Line 6: We directly assign the value to
result
without using a local variable.Line 7: Since
result
is named, we can simply usereturn
without specifying a value.
Output
Product: 12
Key Points:
Named return values make the code more self-explanatory.
The
return
statement can be used without specifying a value if the return value is named.
4. Variadic Functions
A variadic function is a function that can accept a variable number of arguments. It’s useful when you don’t know the exact number of arguments in advance.
Example 4: Variadic Function
package main
import "fmt"
// Defining a variadic function that calculates the sum of multiple integers
func sum(nums ...int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
func main() {
result := sum(1, 2, 3, 4, 5)
fmt.Println("Total Sum:", result)
}
Code Explanation
Line 5: The
sum
function takes a variable number of integer arguments using...int
.Line 6: We initialize a variable
total
to store the sum.Line 7: We use a
for
loop withrange
to iterate over all the arguments.Line 8: The sum of the numbers is calculated by adding each
num
tototal
.Line 12: We call the
sum
function with multiple arguments.
Output
Total Sum: 15
Key Points:
The
...
syntax allows a function to accept any number of arguments.The arguments are treated as a slice inside the function.
Note: Here, we have used some uncovered topics like slice and the
_
syntax. We will cover these topics in our upcoming tutorials, so you don’t need to worry. We will worry for you!
5. Anonymous Functions and Closures
In Go, you can define functions without names, called anonymous functions. Anonymous functions can be used as closures, capturing and using variables from their surrounding scope.
Example 5: Anonymous Function
package main
import "fmt"
func main() {
// Defining and calling an anonymous function
func() {
fmt.Println("Hello from an anonymous function!")
}()
}
Code Explanation
Line 5: We define an anonymous function and call it immediately using
()
.Line 6: The anonymous function prints a message.
Output
Hello from an anonymous function!
Key Points:
- Anonymous functions are useful for short, simple tasks that don’t need a separate named function.
Example 6: Closure
package main
import "fmt"
func main() {
counter := 0
increment := func() {
counter++
fmt.Println("Counter:", counter)
}
increment()
increment()
}
Code Explanation
Line 5: We define a variable
counter
in themain
function.Line 7: We define an anonymous function
increment
that accesses and modifiescounter
.Line 11: We call
increment
twice, increasing the value ofcounter
each time.
Output
Counter: 1
Counter: 2
Key Points:
The anonymous function captures the
counter
variable from its surrounding scope.This behavior is called a closure.
Conclusion
In this article, we covered:
How to define and call functions in Go.
Using function parameters and return values for flexible code.
Named return values for improved readability.
Variadic functions for handling a variable number of arguments.
Anonymous functions and closures, which are powerful features for writing concise and flexible code.
Functions are an essential part of Go programming, providing structure and reusability to your code. In the next article, we’ll dive deeper into advanced function topics like recursion, methods, and higher-order functions.
Happy coding, and stay tuned for more Go tutorials!
Subscribe to my newsletter
Read articles from Shivam Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
